py代码-python发邮件
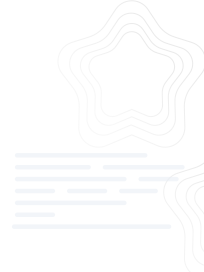

在Python编程语言中,发送电子邮件是一项常见的任务,可用于自动化通知、报告或个人通信。这篇教程将深入探讨如何使用Python编写代码来实现这个功能。我们将主要关注`smtplib`和`email`这两个标准库,它们是Python处理邮件的核心模块。 让我们了解`smtplib`库。它是用于发送简单邮件传输协议(SMTP)邮件的模块。SMTP是互联网上用于传递电子邮件的标准。通过`smtplib.SMTP`类,我们可以连接到SMTP服务器,登录(如果需要),然后发送邮件。 ```python import smtplib server = smtplib.SMTP('smtp.example.com', 587) server.starttls() # 开启加密传输 server.login('username', 'password') # 登录SMTP服务器 ``` 接下来,我们来看`email`库,它提供了一组用于创建电子邮件消息的工具。`email.mime`子模块包含了许多类,用于构建邮件消息的不同部分,如文本、HTML、附件等。 ```python from email.mime.text import MIMEText from email.mime.multipart import MIMEMultipart msg = MIMEMultipart() msg['From'] = 'sender@example.com' msg['To'] = 'receiver@example.com' msg['Subject'] = 'Python发送的邮件' body = '这是一封由Python程序发送的邮件。' msg.attach(MIMEText(body, 'plain')) # 添加附件 with open('attachment.txt', 'rb') as attachment: part = MIMEBase('application', 'octet-stream') part.set_payload(attachment.read()) encoders.encode_base64(part) part.add_header('Content-Disposition', 'attachment; filename="attachment.txt"') msg.attach(part) ``` 现在,我们可以使用`smtplib`发送邮件了: ```python server.send_message(msg) server.quit() ``` 在实际应用中,你可能需要处理更复杂的情况,比如错误处理、使用配置文件存储SMTP凭据、处理多个收件人,或者使用SSL/TLS加密。以下是一个完整的示例,展示了如何封装这些功能: ```python import smtplib from email.mime.text import MIMEText from email.mime.multipart import MIMEMultipart from email.mime.base import MIMEBase from email import encoders import os def send_email(smtp_server, port, username, password, from_addr, to_addrs, subject, body, attachments=None): msg = MIMEMultipart() msg['From'] = from_addr msg['To'] = ', '.join(to_addrs) msg['Subject'] = subject msg.attach(MIMEText(body, 'plain')) if attachments: for attachment in attachments: with open(attachment, 'rb') as file: part = MIMEBase('application', 'octet-stream') part.set_payload(file.read()) encoders.encode_base64(part) part.add_header('Content-Disposition', f'attachment; filename="{os.path.basename(attachment)}"') msg.attach(part) try: server = smtplib.SMTP(smtp_server, port) server.starttls() server.login(username, password) server.send_message(msg) print("邮件发送成功") except Exception as e: print(f"邮件发送失败:{e}") finally: server.quit() # 使用示例 smtp_config = { 'smtp_server': 'smtp.example.com', 'port': 587, 'username': 'your_username', 'password': 'your_password', } send_email(**smtp_config, from_addr='sender@example.com', to_addrs=['receiver1@example.com', 'receiver2@example.com'], subject='Python发送的邮件', body='这是一封由Python程序发送的邮件。', attachments=['attachment.txt']) ``` 在上述代码中,`main.py`可能就是实现上述功能的完整脚本,而`README.txt`可能是包含额外说明或示例用法的文档。 Python通过`smtplib`和`email`库提供了强大且灵活的邮件发送功能。你可以根据需求进行扩展,例如添加日志记录、使用环境变量管理SMTP设置,或者集成到自动化工作流中。掌握这项技能对于任何Python开发者来说都是非常有价值的。
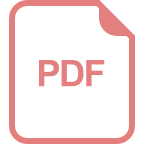
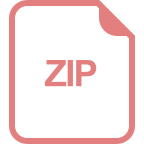
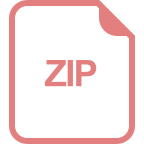
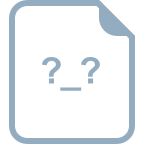
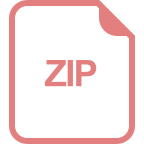
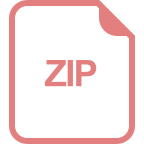
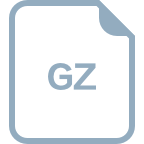
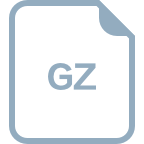
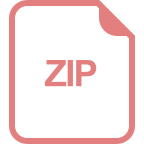
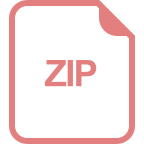
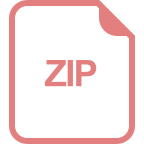
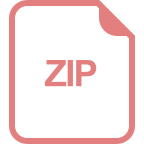
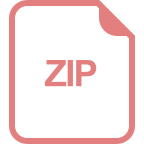
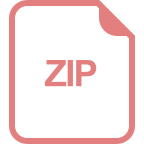
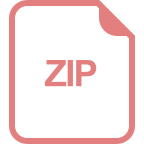
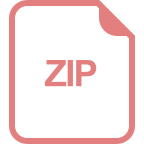
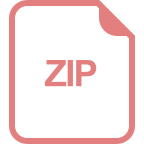
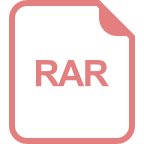
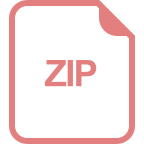
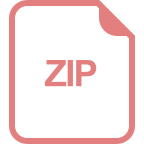
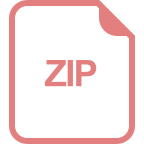
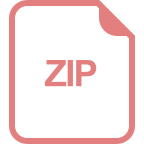
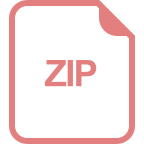
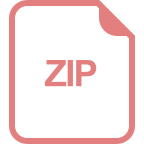
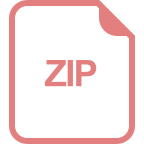
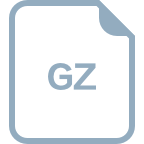

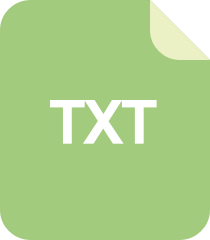
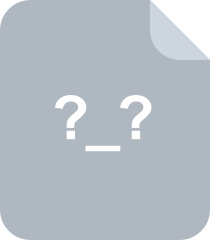
- 1
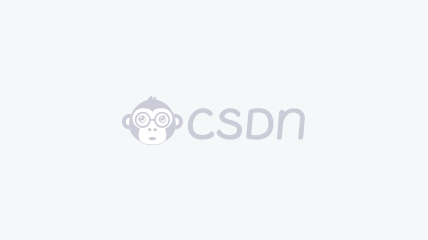

- 粉丝: 7
- 资源: 902
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

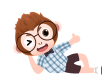
最新资源

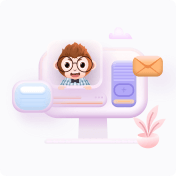
