# V2X_SJTU
## Introduction
A repository of basic codes and usage instructions for [Mokar V2X devices](https://www.huali-tec.com/project.php). The project is sponsored by Shanghai Jiaotong University.
Here are some introductions and tutorials for the V2X device. Honestly speaking, I 'm just a beginner in this field. My document and tutorial contain only some basic ways to code & run programs on the V2X device.
**Instruction about Symbols**
In this instruction, all the linux commands with "$" should be run on your own computer, and the orders with "#" should be run on device.
## Content
1. Description
2. Installation
3. Hardware Connection
4. Before Communication
5. Samples
6. Coding
7. TCP Communication
8. Other Problems
9. Contact
## 1.Description
Folder tree:
.
├── lidar_ros *--ros package for message sending*
│ ├── include
│ ├── msg
│ └── src
├── mde *--SDK provided by Mokar Technology*
│ └── mocar
│ ├── bin *--Compiled files here*
│ ├── build *--Make file here*
│ ├── docs *--Documents by Mocar*
│ ├── libs *--Library*
│ ├── license *--License*
│ └── samples *--Some sample codes by Mokar, except Lidar_send and Lidar_recv generated by Fantasticos*
├── NTP *--Clock Synchronization Software*
│ └── ntpclient-2015
└── TCP_Scripts *--TCP communication code*
├── test_tcp_1 *--Old version 1*
├── test_tcp_2 *--Old version 2*
├── test_tcp_3 *--Latest version*
└── test_tcp_3 (copy) *--Copy of old version 3*
***mde*** is a developing kit provided and copyrighted by Mokar Technology.
***TCP_Scripts*** contains some simple programs and their codes for socket TCP communication between **computer** and **Mokar V2X devices**.
***lidar_ros*** is a ros package sending messages to road side devices
***NTP*** is a software for clock synchronization
## 2. Installation
To clone the codes from Github
`$ cd /your_own_work_space`
`$ git clone https://github.com/Fantasticos/V2X_SJTU.git`
Install some essential libraries
`$ sudo apt-get install libc6:i386`
`$ sudo apt-get install lib32stdc++6`
`$ sudo apt-get install lib32z1`
**Install *arm-linux-gnueabihf* cross compiler**
Download [compiler](https://pan.baidu.com/s/1o6HW8bS#list/path=%2Fshare)
Move *.tar* to `/opt`
`$ sudo mv gcc-linaro-arm-linux-gnueabihf-4.9-2014.07_linux/ /opt`
Extract
`$ tar -xvf gcc-linaro-arm-linux-gnueabihf-4.9-2014.07_linux.tar`
Modify profile
`$ cd /etc`
`$ sudo gedit profile`
add `export PATH=$PATH:/opt/gcc-linaro-arm-linux-gnueabihf-4.9-2014.07_linux/bin`
`$ sudo gedit ~/.bashrc`
add `source /etc/profile`
reboot the terminal
## 3. Hardware Connection
Refer to the handbook provided by Huali for hardware wire connection instruction
If you want to connect your computer and v2x device by Wifi, search for Wifi AP named "IMASTERXXXX", default device ip address 192.168.10.1
If you want to connect through Ethernet, change your local ip address to be 192.168.253.1 and wire-connect directly to the Mokar device, or through a switch. Default device ip address 192.168.253.10
## 4. Before Communication
Suppose you connect through a wire, the ip address of V2X device will be 192.168.253.10. If you connect wirelessly, just replace the ip with 192.168.10.1
**IMPORTANT**
You should change your ip address according to your target address. For example, if you want to connect to 192.168.253.10 with Ethernet, you have to change your own ipv4 address to be 192.168.253.x, x can be replaced by any integer from 0 to 255 except 10 (you cannot set your ip to be identical with your device's)
Before you test the communicaiton, you have to upload the essential libraries.
```
$ cd mde/mocar/libs
$ scp libmocarv2x.so root@192.168.253.10:/usr/lib/
$ scp base/libasnhl.so root@192.168.253.10:/usr/lib/
$ scp base/libosstoed.so root@192.168.253.10:/usr/lib/
$ scp base/libLLC.so root@192.168.253.10:/usr/lib/
$ scp base/libstack.so root@192.168.253.10:/usr/lib/
$ scp base/libsmartway.so root@192.168.253.10:/usr/lib/
$ scp ../samples/mocar_log.conf root@192.168.253.10:/usr/local
```
**PASSWORD:** hL2017.moKar
## 5. Run Samples
The sample codes are in `mocar/samples`, you can compile the sample under `mocar/build`
```
$ cd mde/mocar/build
$ make
```
Upload the sample app to the v2x device, take **bsm** for example
```
$ cd ../bin
$ scp bsm root@192.168.253.10:/var
```
Open a new terminal, log into the device through SSH
`$ ssh -l root 192.168.253.10`
**PASSWORD:** hL2017.moKar
Enter `/var` and run the sample app
`# cd /var`
`# ./bsm`
If warn `undefined symbol: mde_stack_init`, just remove `usr/local` and upload the .conf file again.
`# rm -rf /usr/local/*`
`$ scp ../samples/mocar_log.conf root@192.168.253.10:/usr/local`
Now you can see the device sending bsm message at 10 HZ.
You can repeat this process and upload program to another Mokar device, run the same `./bsm`, you can definitely see the two device communicating!!!
**If you want to change the IP of device**
`# vi /etc/network/interfaces`
change the address
## 6. Coding
The coding work now takes place in `mocar/samples`
Two example are provided, *lidar_send* and *lidar_recv*. I wrote these two program for my graduation project. They combine both TCP communication and BSM message.
To generate your own code, I suggest you to copy one sample, like **bsm**, copy the folder and rename the folder. Also remember to replace the code file name and the name in makefile.
Now you can write any funcion in c++ you like!!!
## 7. TCP Communicaiton
The communication between computer and V2X device is done by TCP.
In **TCP_Scripts**, programs include *client* and *server*, client runs on the computer and server runs on the device.
In my project, the tcp server is merged into `samples/lidar_send` and `samples/lidar_recv`. *lidar_send* is for the Road Side Device, while *lidar_recv* is for the On Board device
To test, you can upload *lidar_send* or *lidar_recv* to the device, these two are both tcp servers. *lidar_send* is for road side unit while *lidar_recv* is for on board unit.
I wrote 2 clients for this project, one is road side client. It subscribe messages from rostopic and publish them through tcp to the road side v2x device.
Another client is `TCP_Scripts/test_tcp_3/TCP_Client2.c` or its executable `client2`
I also wrote a `talker.cpp` to simulate the message published by the lidar.
To fully function the communication chain, follow the steps **carefully**:
1. Move `/lidar_ros` to your own workspace i.e. `catkin_ws`
```
$ cd catkin_ws
$ catkin_make
$ roscore
```
2. Start the 2 tcp servers on the devices, take the road side device for example, you can repeat it again with OBU.
use ssh login 192.168.10.1
`# cd /var`
`# ./lidar_send`
open another terminal and ssh login again, use the ntp to synchronize the two clock
`# cd /var`
`# ./ntpclient -s -d -c 1 -i 5 -h 192.168.10.101`
**you can refer to 8. Other Problems for instruction on ntpclient**
**ATTENTION**
Some times when you run *lidar_send* or *lidar_recv* you will meet the segment fault. This is because the time should be changed to 01/01/2010 before running the program. (I'm also confused why, but this is what the engineer told me)
To fix this problem
open another terminal, ssh login
`# date 0101000010`
3. Start the clients
for road side unit, open the client to send messages
`$ rosrun lidar_ros talker`
`$ rosrun lidar_ros listener 192.168.10.1`
for on board unit
`$ cd TCP_Scripts/test_tcp_3`
`$ ./client2 192.168.253.10`
Now you can see the two device communicating messages generated by talker.
## 8. Other Problems
**Synchronization of clock**
To make sure that the clock

weixin_38722193
- 粉丝: 5
- 资源: 908
最新资源
- matlab实现四旋翼无人机自抗扰姿态容错控制-飞行器控制-四旋翼无人机-自抗扰控制-UAV-扰动识别-matlab
- 基于主从博弈的综合能源微网与共享储能优化运行研究:迭代算法探索与利益相关者的博弈策略,基于主从博弈的综合能源微网与共享储能优化运行研究:电价策略与用户行为互动,matlab 代码基于主从博弈的共享储能
- 14章RAG全栈技术从基础到精通 ,打造高精准AI应用
- MATLAB平台下的多目标粒子群算法在冷热电联供综合能源系统优化中的实践,基于多目标粒子群算法的冷热电联供综合能源系统优化调度模型,MATLAB代码:基于多目标粒子群算法冷热电联供综合能源系统运行优化
- 三相桥式全控整流及其有源逆变与Simulink仿真波形分析(含不同触发角和负载变化图),三相桥式全控整流及其有源逆变与Simulink仿真探究:触发角与负载变化下的波形图分析,三相桥式全控整流及其有源
- 中国智能制造装备产业发展机遇蓝皮书 2024.pdf
- 【毕业设计】Python的Django-html网易新闻+评论的舆情热点分析平台源码(完整前后端+mysql+说明文档+LW+PPT).zip
- 西门子1200 PLC与昆仑通态上位软件结合的新能源物料输送系统程序案例解析,V16+博图版本,RS485通讯控制托利多称重仪表模拟量读取技术实现,西门子S1200博图物料输送系统:新能源物料输送PL
- 【毕业设计】Python的Django-html图像的信息隐藏技术研究源码(完整前后端+mysql+说明文档+LW+PPT).zip
- 【毕业设计】Python的Django-html深度学习的车牌识别系统源码(完整前后端+mysql+说明文档+LW+PPT).zip
- 【毕业设计】Python的Django-html深度学习屋内烟雾检测方法源码(完整前后端+mysql+说明文档+LW+PPT).zip
- 灰狼算法在微电网优化调度中的应用:考虑风光储与需求响应的多元经济优化策略,模型可塑性强且出图丰富的实践研究,灰狼算法在微电网风光储需求响应优化调度中的应用:经济性优化与模型可塑性的研究分析图集,灰狼算
- 【毕业设计】Python的Django-html人脸识别的实验室智能门禁系统源码(完整前后端+mysql+说明文档+LW+PPT).zip
- 【毕业设计】Python的Django-html深度学习的身份证识别考勤系统源码(完整前后端+mysql+说明文档+LW+PPT).zip
- 【毕业设计】Python的Django-html时间序列分析的大气污染预测系统源码(完整前后端+mysql+说明文档+LW+PPT).zip
- matlab实现遗传算法在物流中心配送路径规划中的应用-遗传算法-路径规划-物流配送-最佳配送方案-matlab
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


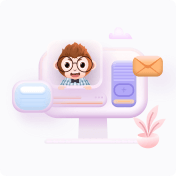
评论0