webuploader 实现图片批量上传功能附实例代码
Webuploader 实现图片批量上传功能 Webuploader 是一个功能强大且灵活的上传组件,提供了多种上传方式,包括图片、文件、视频等。今天,我们将通过实例代码,来演示如何使用 Webuploader 实现图片批量上传功能。 标题解释 标题“webuploader 实现图片批量上传功能附实例代码”表明了本文的主要内容,即使用 Webuploader 实现图片批量上传功能,并提供了实例代码以供参考。 描述解释 描述中提到,Webuploader 实现图片批量上传功能非常不错,具有参考借鉴价值。这说明了 Webuploader 在图片批量上传方面的优势和可靠性。 标签解释 标签“webuploader 上传图片”表明了本文的主要主题,即使用 Webuploader 实现图片上传功能。 部分内容解释 在部分内容中,我们可以看到 Webuploader 的实例代码,包括 JSP 代码和 HTML 代码。这些代码展示了如何使用 Webuploader 实现图片批量上传功能。 在 JSP 代码中,我们可以看到以下几个部分: 1. 导入资源:在这里,我们需要导入 Webuploader 相关的资源,例如 JavaScript 文件和 CSS 文件。 2. JSP 代码:在这里,我们可以看到 Webuploader 的实例代码,包括上传按钮、上传进度条、上传状态等。 在 HTML 代码中,我们可以看到以下几个部分: 1. 项目名称:这里显示项目名称,并提供了文本框以供用户输入。 2. 缩略图:这里显示缩略图,并提供了上传按钮以供用户选择图片。 3. 项目发票上传:这里显示项目发票上传部分,包括上传按钮、上传进度条、上传状态等。 知识点解释 通过分析上述代码,我们可以得到以下知识点: 1. Webuploader 是一个功能强大且灵活的上传组件,提供了多种上传方式,包括图片、文件、视频等。 2. 使用 Webuploader 可以实现图片批量上传功能,提高上传效率和用户体验。 3. Webuploader 提供了多种上传方式,包括选择文件、拖拽文件、粘贴文件等。 4. 在实现图片批量上传功能时,需要导入 Webuploader 相关的资源,例如 JavaScript 文件和 CSS 文件。 5. 使用 Webuploader 需要配置上传参数,例如上传的文件类型、上传的文件大小、上传的文件数量等。 总结 本文介绍了如何使用 Webuploader 实现图片批量上传功能,并提供了实例代码以供参考。通过学习本文,读者可以更好地理解 Webuploader 的使用方法和优点,从而更好地应用于实际项目中。
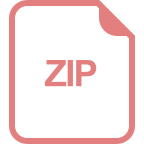
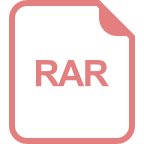
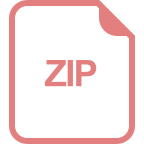
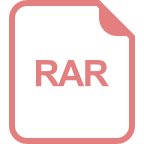
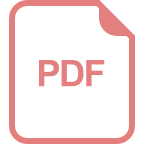
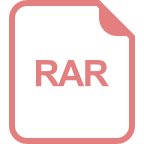
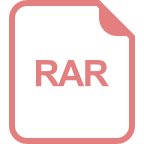
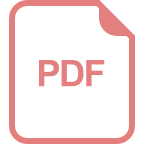
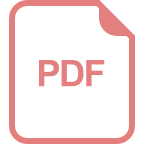
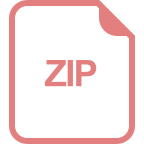
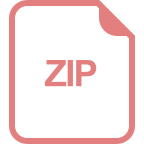
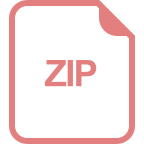
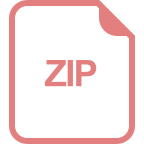
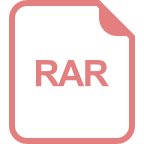
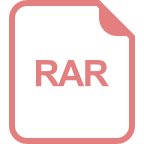
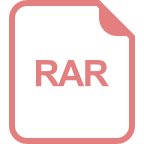
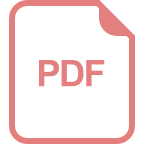
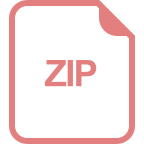
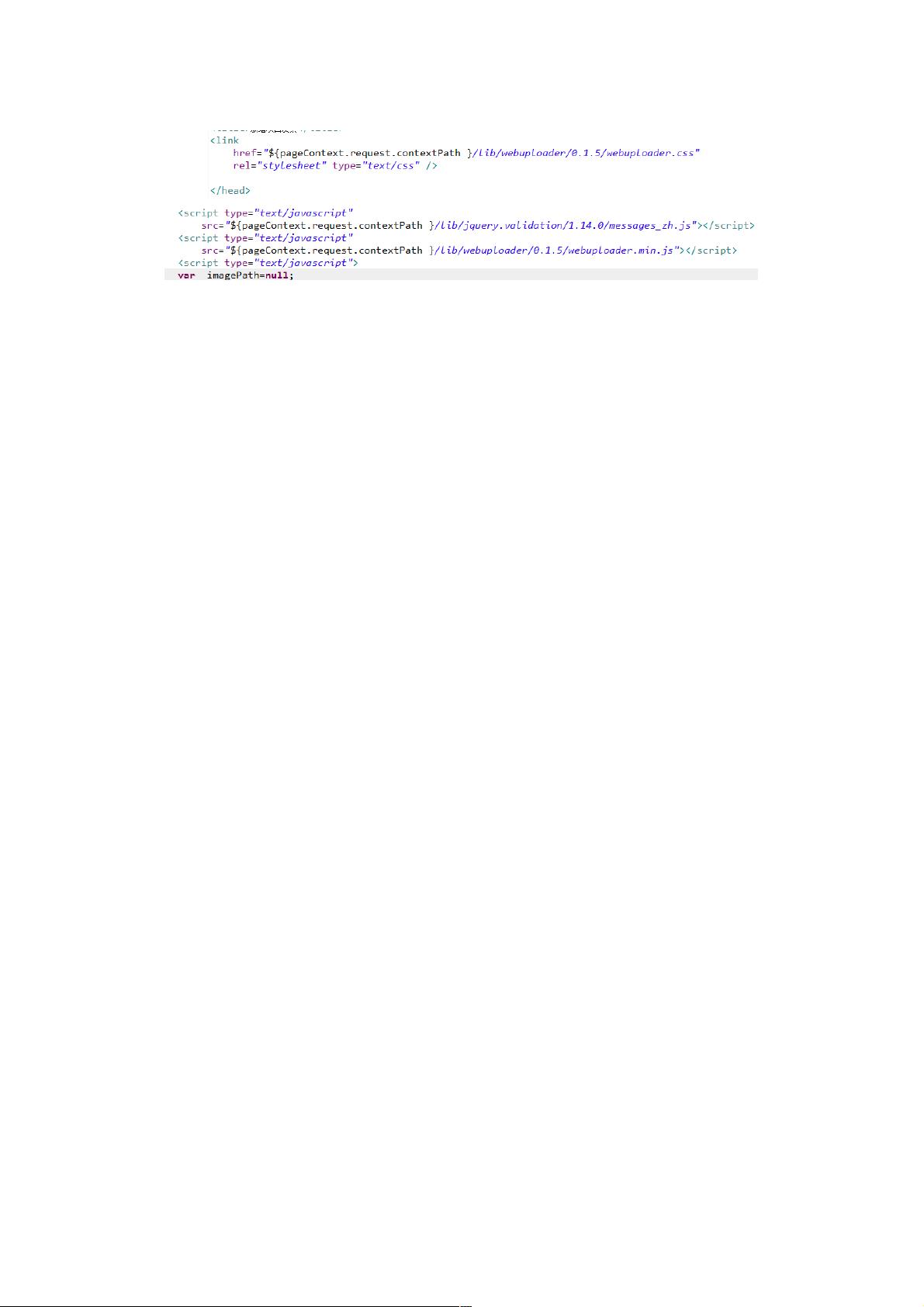
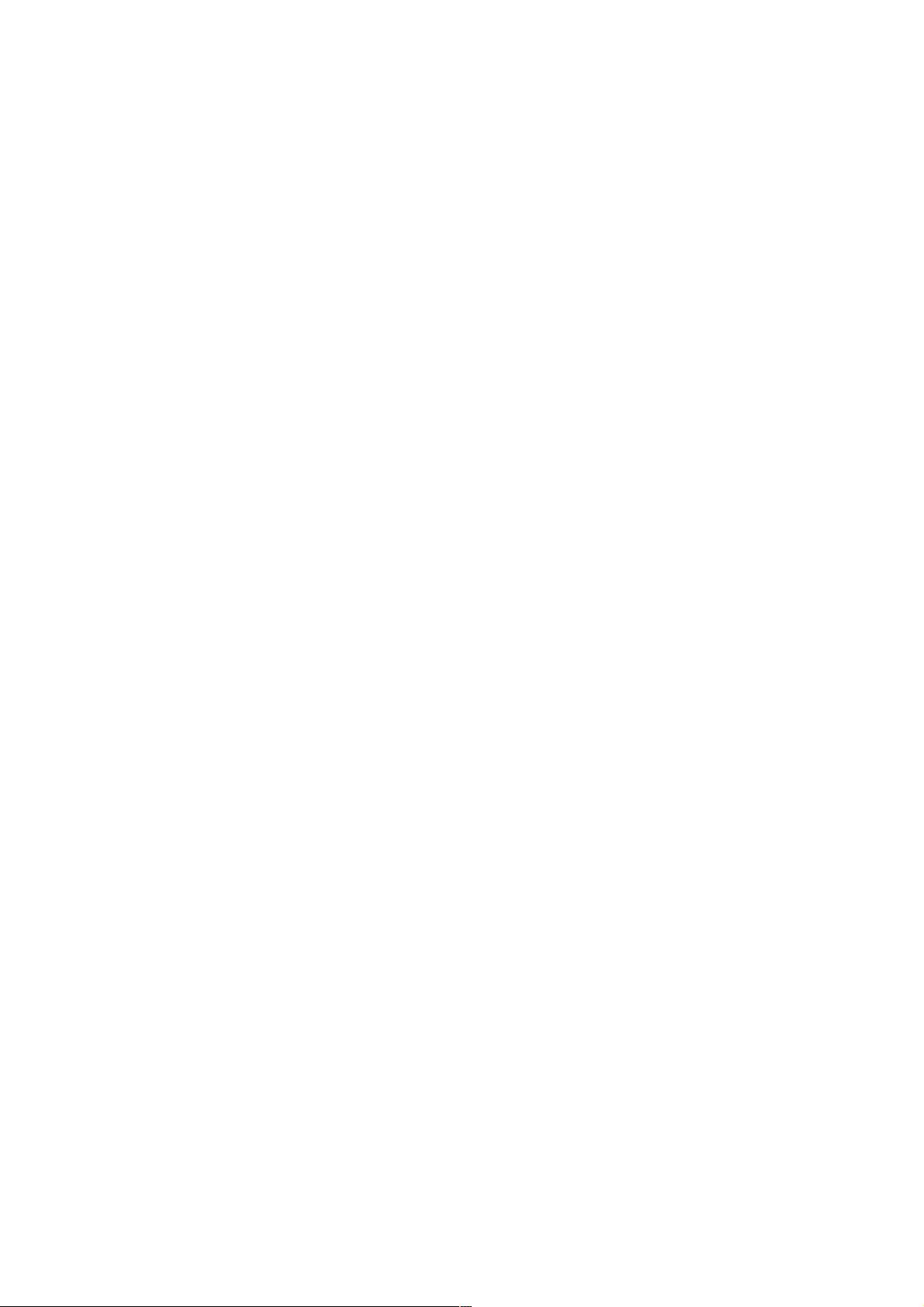
剩余8页未读,继续阅读
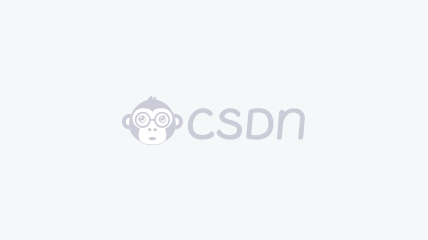

- 粉丝: 4
- 资源: 908
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

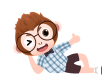
最新资源
- mmexport1731025953198.mp4
- 案例数据:标准木数据.sav
- 技术资料分享nRF24L01中文说明书很好的技术资料.zip
- 技术资料分享NRF24l01模块说明书很好的技术资料.zip
- 技术资料分享NRF24L01功能使用文档很好的技术资料.zip
- mbedtls-3.5.2的VS2015编译库
- 技术资料分享nRF24L01P(新版无线模块控制IC)很好的技术资料.zip
- 技术资料分享Nintendo Entertainment System Documentation Version 1.0
- 技术资料分享NES Specifications很好的技术资料.zip
- 技术资料分享MultiMediaCard Product Manual很好的技术资料.zip

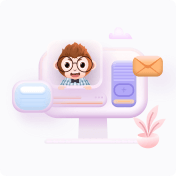
