【多线程】是计算机编程中的一个重要概念,它允许程序在同一时间内执行多个任务,从而提高了程序的执行效率。在Java中,多线程是通过创建并运行多个线程来实现的,每个线程代表着进程中的一条独立控制流。Java线程是抢占式的,意味着线程的执行是由操作系统调度,根据优先级和当前状态决定。 【进程】是一个程序或应用的实例,拥有自己的内存空间和系统资源。在同一个进程中,可以存在多个【线程】,它们共享进程的内存和文件资源,这样就减少了通信成本。创建和销毁线程相比进程来说更快,线程间的切换也更为高效,这是因为它们位于同一内存空间内,不需要像进程间通信那样通过内核。 【同步与异步】是处理并发操作的关键概念。同步操作意味着调用者必须等待方法执行完毕才能继续,保证了操作的顺序性。异步操作则相反,调用者无需等待,可以立即返回,通常由另一个线程执行后续任务。异步操作在提高系统响应速度方面有很大优势,但也可能导致控制流程的复杂性增加。 【阻塞与非阻塞】是指线程对资源的访问方式。阻塞线程在无法获取所需资源时会被挂起,等待资源释放。而非阻塞线程即使无法立即获取资源,也会尝试进行其他操作,不会导致线程挂起。非阻塞操作常用于优化并发性能,避免线程间的相互等待。 【临界资源与临界区】是并发编程中需要特别注意的部分。临界资源是多个线程可能同时访问的共享资源,为避免数据竞争,需要通过同步机制(如锁)来确保同一时间只有一个线程能访问。临界区是包含对临界资源操作的代码段,确保每次只有一个线程执行这段代码。 在Java中,【Thread】类是实现多线程的基础。它实现了Runnable接口,可以通过重写run()方法定义线程的行为。Thread类有多种构造器,允许我们创建带有不同参数的线程,例如指定线程组、Runnable目标或线程名等。通过调用start()方法,我们可以启动线程的执行,而run()方法将在此时被执行。 多线程是提高程序并发性和效率的重要手段,但同时也引入了线程安全、死锁等问题,需要开发者精心设计和管理线程的生命周期,正确使用同步和异步机制,以及处理好临界资源的访问,以保证程序的稳定和高效运行。
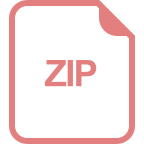
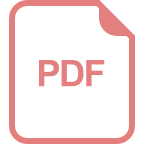
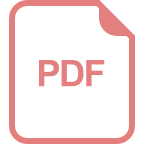
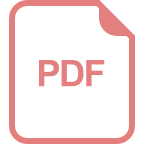
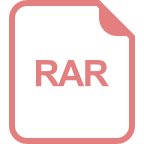
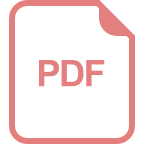
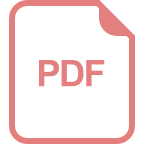
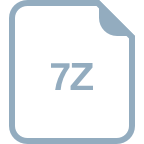
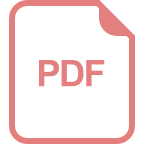
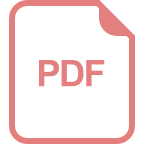
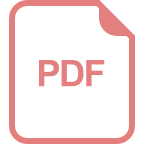
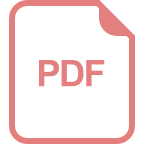
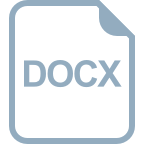
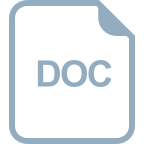
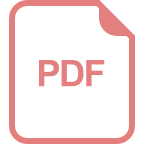
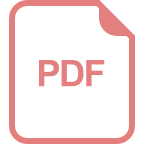
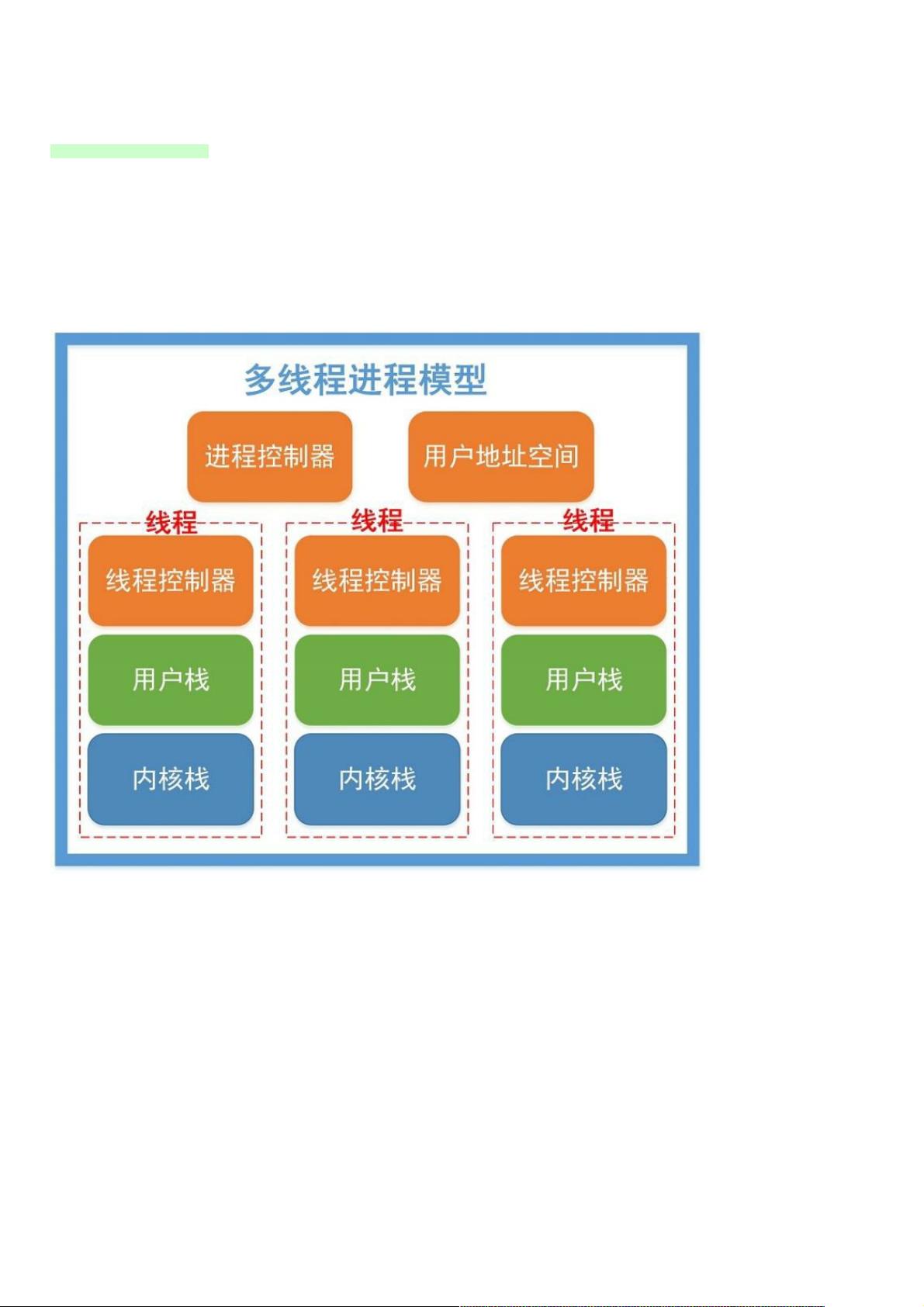
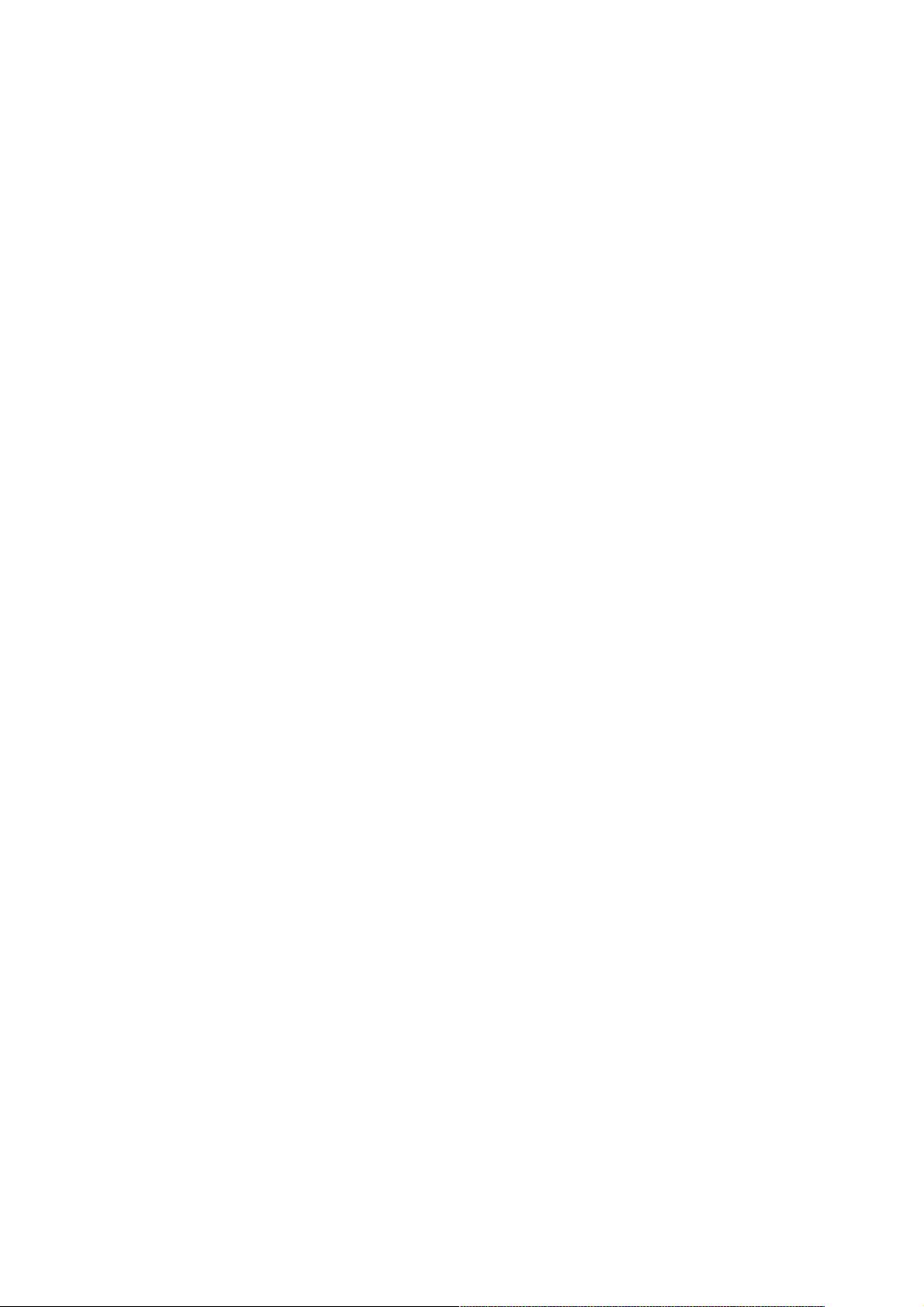
剩余8页未读,继续阅读
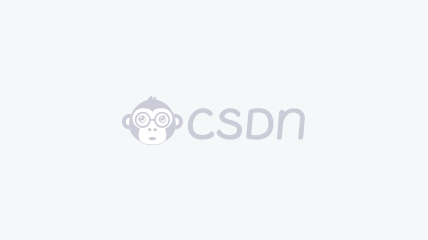

- 粉丝: 7
- 资源: 941
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

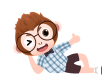
最新资源
- 毕设和企业适用springboot智能云服务平台类及物流信息平台源码+论文+视频.zip
- 毕设和企业适用springboot智能云服务平台类及用户体验优化平台源码+论文+视频.zip
- 毕设和企业适用springboot智能云服务平台类及员工管理平台源码+论文+视频.zip
- 毕设和企业适用springboot智能云服务平台类及智能农业解决方案源码+论文+视频.zip
- 毕设和企业适用springboot智能云服务平台类及智能语音助手平台源码+论文+视频.zip
- 毕设和企业适用springboot智能制造类及车联网管理平台源码+论文+视频.zip
- 毕设和企业适用springboot智能制造类及互联网金融平台源码+论文+视频.zip
- 毕设和企业适用springboot智能制造类及个性化推荐平台源码+论文+视频.zip
- 毕设和企业适用springboot智能制造类及机器学习平台源码+论文+视频.zip
- 毕设和企业适用springboot智能制造类及交通信息平台源码+论文+视频.zip
- 毕设和企业适用springboot智能制造类及健康风险评估平台源码+论文+视频.zip
- 毕设和企业适用springboot智能制造类及企业管理智能化平台源码+论文+视频.zip
- 毕设和企业适用springboot智能制造类及旅游数据平台源码+论文+视频.zip
- 毕设和企业适用springboot智能制造类及企业培训平台源码+论文+视频.zip
- 毕设和企业适用springboot智能制造类及视觉识别平台源码+论文+视频.zip
- 毕设和企业适用springboot智能制造类及数字营销平台源码+论文+视频.zip

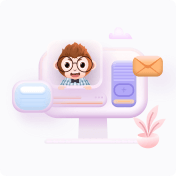
