### Python 解压、复制、删除文件的实例代码详解 #### 一、Python 解压文件 在 Python 中处理文件的解压通常涉及到多种压缩格式,如 zip、rar、tar 和 tar.gz。下面详细介绍如何使用 Python 来解压这些常见的压缩文件。 ##### 1. Python 解压文件代码示例 首先来看一个通用的解压函数 `unzip_file` 的实现。这个函数支持递归解压,并且可以处理多种压缩文件类型。以下为具体的代码实现: ```python import os from zipfile import ZipFile import rarfile import tarfile def unzip_file(src_file, dst_dir=None, unzipped_files=None, del_flag=True): """ 根据指定的压缩文件类型递归解压所有指定类型的压缩文件 :param src_file: 解压的源文件路径,可以为文件夹路径也可以是文件路径 :param dst_dir: 解压后的文件存储路径 :param unzipped_files: 完成解压的文件名列表 :param del_flag: 解压完成后是否删除原压缩文件,默认删除 :return: 完成解压的文件名列表 """ # 完成解压的文件名列表初始为空 if unzipped_files is None: unzipped_files = [] # 指定的解压文件类型 zip_types = ['.zip', '.rar', '.tar', '.gz'] def exec_decompress(zip_file, dst_dir): """ 解压实现的公共代码 :param zip_file: 压缩文件全路径 :param dst_dir: 解压后文件存储路径 :return: """ file_suffix = os.path.splitext(zip_file)[1].lower() try: print(f'Start extracting the file: {zip_file}') # zip 解压 if file_suffix == '.zip': with ZipFile(zip_file, mode='r') as zf: zf.extractall(dst_dir) # rar 解压 elif file_suffix == '.rar': rf = rarfile.RarFile(zip_file) rf.extractall(dst_dir) # tar、tgz(tar.gz)解压 elif file_suffix in ['.tar', '.gz']: tf = tarfile.open(zip_file) tf.extractall(dst_dir) tf.close() print(f'Finished extracting the file: {zip_file}') except Exception as e: print(e) # 解压完成加入完成列表 unzipped_files.append(zip_file) # 根据标识执行原压缩文件删除 if del_flag and os.path.exists(zip_file): os.remove(zip_file) # 如果传入的文件路径为文件目录,则遍历目录下所有文件 if os.path.isdir(src_file): # 初始化文件目录下存在的压缩文件集合为空 zip_files = [] # 如果传入的目的文件路径为空,则取解压的原文件夹路径 dst_dir = dst_dir if dst_dir else src_file # 遍历目录下所有文件 for file in os.listdir(src_file): file_path = os.path.join(src_file, file) # 如果是文件夹则继续递归解压 if os.path.isdir(file_path): dst_path = os.path.join(dst_dir, file) unzip_file(file_path, dst_path, unzipped_files) # 如果是指定类型的压缩文件则加入到压缩文件列表 elif os.path.isfile(file_path) and os.path.splitext(file_path)[1].lower() in zip_types and file_path not in unzipped_files: zip_files.append(file_path) # 遍历压缩文件列表,执行压缩文件的解压 for zip_file in zip_files: exec_decompress(zip_file, dst_dir) else: exec_decompress(src_file, dst_dir) return unzipped_files ``` #### 二、Python 复制文件 复制文件在 Python 中可以通过多种方式实现,其中最常用的是使用 `shutil` 模块中的 `copy()` 或 `copy2()` 函数。下面是这两种方法的具体应用: ```python import shutil import os def copy_file(src, dst): """ 复制单个文件 :param src: 源文件路径 :param dst: 目标文件路径 """ shutil.copy2(src, dst) # 使用copy2()保留文件元数据 def copy_folder(src, dst): """ 复制整个文件夹及其子文件夹 :param src: 源文件夹路径 :param dst: 目标文件夹路径 """ shutil.copytree(src, dst) ``` #### 三、Python 删除文件 删除文件在 Python 中可以通过 `os` 模块中的 `remove()` 或 `rmdir()` 函数来实现。以下是具体的应用示例: ```python import os def delete_file(file_path): """ 删除单个文件 :param file_path: 文件路径 """ os.remove(file_path) def delete_folder(folder_path): """ 删除空文件夹 :param folder_path: 文件夹路径 """ os.rmdir(folder_path) def delete_non_empty_folder(folder_path): """ 删除非空文件夹及其内容 :param folder_path: 文件夹路径 """ shutil.rmtree(folder_path) ``` 以上是关于使用 Python 进行文件解压、复制和删除的详细代码实现与解释。这些代码段不仅可以帮助理解如何处理各种文件操作,而且还可以作为实际项目中的参考或直接使用。
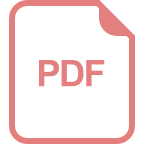
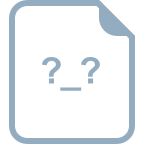
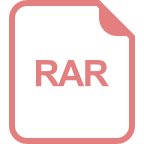
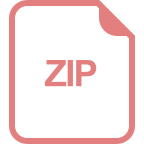
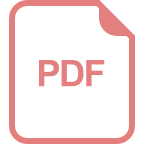
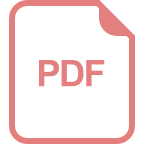
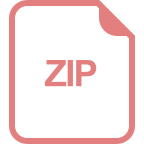
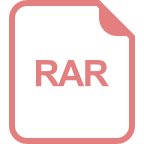
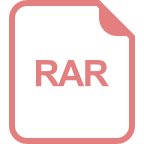
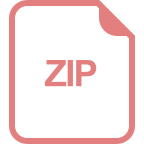
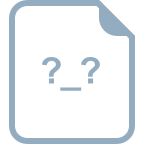
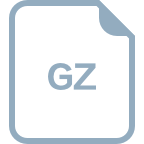
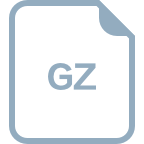
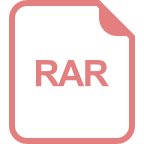
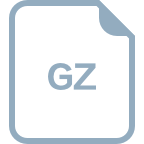
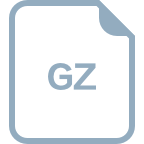
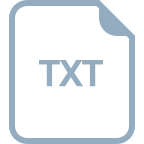
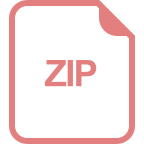
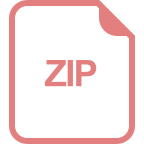
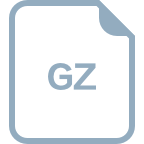
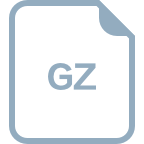
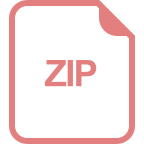
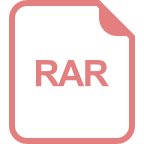
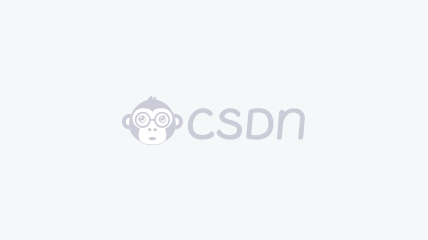

- 粉丝: 5
- 资源: 882
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

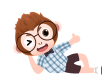
最新资源

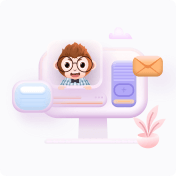
