js代码-LinkList 单向
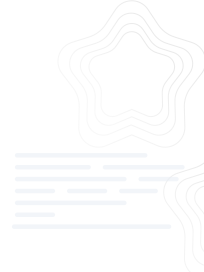

JavaScript中的链表是一种数据结构,它在内存中不连续存储元素,而是通过节点之间的引用连接。单向链表是链表的一种类型,每个节点包含数据和一个指向下一个节点的引用,但没有指回上一个节点的链接。在这个"js代码-LinkList 单向"项目中,我们主要会探讨如何在JavaScript中实现单向链表。 我们需要创建一个Node类,它代表链表中的一个节点。这个Node类通常有两个属性:`value`用于存储数据,`next`则指向链表中的下一个节点。 ```javascript class Node { constructor(value) { this.value = value; this.next = null; // 初始化为null,表示当前节点是链表的末尾 } } ``` 接下来,我们需要创建一个LinkedList类来管理这些节点。LinkedList类通常包含以下方法: 1. `constructor()`: 构造函数,初始化空链表。 2. `append(value)`: 在链表末尾添加新的节点。 3. `prepend(value)`: 在链表开头添加新的节点。 4. `insertBefore(value, newVal)`: 在指定值的节点前插入新节点。 5. `insertAfter(value, newVal)`: 在指定值的节点后插入新节点。 6. `delete(value)`: 删除具有指定值的第一个节点。 7. `search(value)`: 查找具有指定值的节点,返回找到的节点或null。 8. `print()`: 打印链表的所有节点值。 以下是LinkedList类的实现: ```javascript class LinkedList { constructor() { this.head = null; this.size = 0; } append(value) { const newNode = new Node(value); if (!this.head) { this.head = newNode; } else { let current = this.head; while (current.next) { current = current.next; } current.next = newNode; } this.size++; } prepend(value) { const newNode = new Node(value); newNode.next = this.head; this.head = newNode; this.size++; } insertBefore(value, newVal) { let newNode = new Node(newVal); let current = this.head; let found = false; while (current && !found) { if (current.value === value) { newNode.next = current; if (current === this.head) { this.head = newNode; } else { previous.next = newNode; } found = true; } else { previous = current; current = current.next; } } if (!found) { console.log("Value not found in the list."); } else { this.size++; } } insertAfter(value, newVal) { let newNode = new Node(newVal); let current = this.head; let previous = null; let found = false; while (current && !found) { if (current.value === value) { found = true; newNode.next = current.next; current.next = newNode; } else { previous = current; current = current.next; } } if (!found) { console.log("Value not found in the list."); } else { this.size++; } } delete(value) { if (!this.head) return; if (this.head.value === value) { this.head = this.head.next; this.size--; return; } let current = this.head; while (current.next && current.next.value !== value) { current = current.next; } if (current.next) { current.next = current.next.next; this.size--; } else { console.log("Value not found in the list."); } } search(value) { let current = this.head; while (current) { if (current.value === value) { return current; } current = current.next; } return null; } print() { let current = this.head; let result = []; while (current) { result.push(current.value); current = current.next; } console.log(result); } } ``` `main.js`文件可能包含了使用这个LinkedList类的一些示例代码,例如创建链表、插入节点、删除节点和打印链表。而`README.txt`文件可能包含了对项目的一些说明和使用指南。 在JavaScript中实现链表可以帮助我们理解数据结构的基础,这对于优化算法和提高代码效率至关重要。链表操作如插入和删除通常比数组快,因为它们不需要移动数组中的其他元素。然而,链表不支持随机访问,这意味着获取链表中任意位置的元素通常需要从头开始遍历。因此,在选择数据结构时,应根据具体需求和性能考虑来决定。
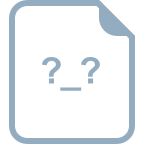
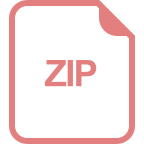
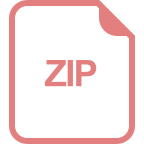
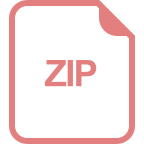
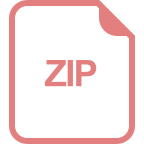
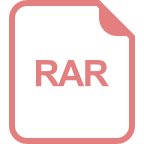
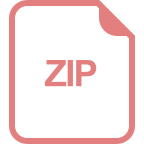
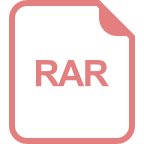
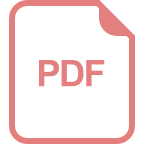
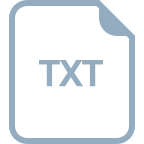
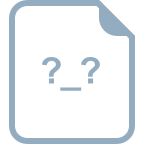
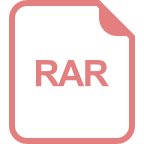
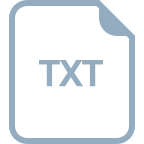
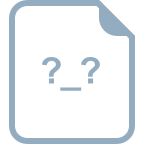
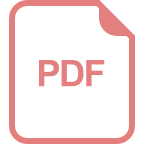
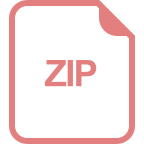

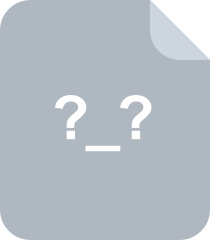
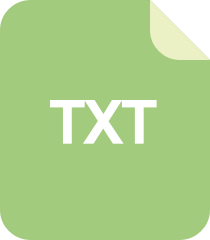
- 1
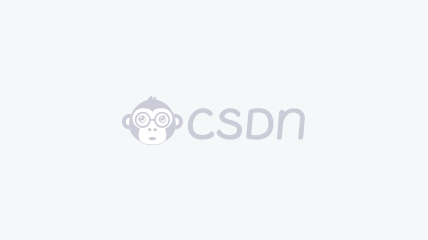

- 粉丝: 4
- 资源: 958
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

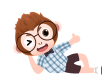
最新资源
- 基于Java技术的ASC学业支持中心并行项目开发设计源码
- 基于Java和微信支付的wxmall开源卖票商城设计源码
- 基于Java和前端技术的东软环保公众监督系统设计源码
- 基于Python、HTML、CSS的crawlerdemo软件工程实训爬虫设计源码
- 基于多智能体深度强化学习的边缘协同任务卸载方法设计源码
- 基于BS架构的Java、Vue、JavaScript、CSS、HTML整合的毕业设计源码
- 基于昇腾硬件加速的AI大模型性能优化设计源码
- 基于Plpgsql与Python FastAPI的mini-rbac-serve权限管理系统后端设计源码
- 基于SpringBoot的轻量级Java快速开发源码
- 基于Python开发的物流调度算法设计源码

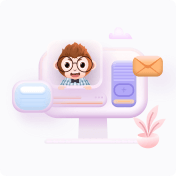
