### 简单了解Django文件下载方式 在开发基于Django框架的Web应用时,文件下载是一项常见的需求。无论是用户需要下载文档、图片还是其他类型的文件,掌握Django中的文件下载方法都是非常重要的。本文将详细介绍Django中的三种文件下载方式,并通过具体的代码示例来帮助读者更好地理解和应用这些技术。 #### 方式一:使用HttpResponse 这是最基础也是最直接的方法之一。通过创建一个`HttpResponse`对象并将其与文件关联起来,可以实现简单的文件下载功能。 **步骤详解**: 1. **打开文件**:首先需要使用Python内置的`open`函数打开待下载的文件。需要注意的是,为了确保文件能够正确地传输,这里需要以二进制模式(`'rb'`)打开文件。 ```python file = open('path/to/your/file', 'rb') ``` 2. **创建HttpResponse对象**:接下来,使用打开的文件创建一个`HttpResponse`对象。 ```python response = HttpResponse(file) ``` 3. **设置响应头**:为了让浏览器识别出这是一个可下载的文件而非普通的网页内容,需要设置响应头。其中`Content-Type`表示文件类型,`Content-Disposition`用于指定文件名以及下载行为。 ```python response['Content-Type'] = 'application/octet-stream' response['Content-Disposition'] = 'attachment; filename="filename"' ``` 4. **返回响应**:最后返回创建好的`HttpResponse`对象即可。 ```python return response ``` **完整示例代码**: ```python from django.shortcuts import HttpResponse def download(request): file = open('path/to/your/file', 'rb') response = HttpResponse(file) response['Content-Type'] = 'application/octet-stream' response['Content-Disposition'] = 'attachment; filename="filename"' return response ``` #### 方式二:使用StreamingHttpResponse 这种方式相比使用`HttpResponse`更为高效,因为它采用流式传输,可以处理大文件而不会占用过多内存。`StreamingHttpResponse`允许开发者逐块读取文件内容并发送给客户端,从而降低内存消耗。 **步骤详解**: 1. **打开文件**:同样以二进制模式打开文件。 ```python file = open('path/to/your/file', 'rb') ``` 2. **创建StreamingHttpResponse对象**:与上一种方式不同的是,这里使用`StreamingHttpResponse`对象。 ```python response = StreamingHttpResponse(file) ``` 3. **设置响应头**:与使用`HttpResponse`相同。 ```python response['Content-Type'] = 'application/octet-stream' response['Content-Disposition'] = 'attachment; filename="filename"' ``` 4. **返回响应**:返回创建好的`StreamingHttpResponse`对象。 ```python return response ``` **完整示例代码**: ```python from django.http import StreamingHttpResponse def download(request): file = open('path/to/your/file', 'rb') response = StreamingHttpResponse(file) response['Content-Type'] = 'application/octet-stream' response['Content-Disposition'] = 'attachment; filename="filename"' return response ``` #### 方式三:使用FileResponse `FileResponse`是Django提供的专门用于文件下载的工具类,它内部实现了基于迭代器的数据流传输机制,因此可以高效地处理大文件。 **步骤详解**: 1. **打开文件**:以二进制模式打开文件。 ```python file = open('path/to/your/file', 'rb') ``` 2. **创建FileResponse对象**:使用`FileResponse`对象代替之前的`HttpResponse`或`StreamingHttpResponse`。 ```python response = FileResponse(file) ``` 3. **设置响应头**:同样需要设置响应头。 ```python response['Content-Type'] = 'application/octet-stream' response['Content-Disposition'] = 'attachment; filename="filename"' ``` 4. **返回响应**:返回创建好的`FileResponse`对象。 ```python return response ``` **完整示例代码**: ```python from django.http import FileResponse def download(request): file = open('path/to/your/file', 'rb') response = FileResponse(file) response['Content-Type'] = 'application/octet-stream' response['Content-Disposition'] = 'attachment; filename="filename"' return response ``` ### 总结 以上介绍了Django中实现文件下载的三种方法:使用`HttpResponse`、`StreamingHttpResponse`和`FileResponse`。这三种方式各有特点: - **HttpResponse**:简单易用,但不适合处理大文件。 - **StreamingHttpResponse**:适合处理大文件,但需要更多手动控制。 - **FileResponse**:专门针对文件下载设计,使用方便且性能优秀。 根据实际应用场景和需求,开发者可以选择最适合自己的方式来实现文件下载功能。
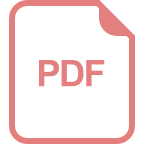
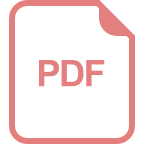
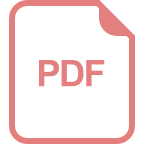
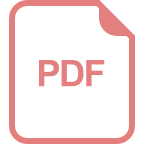
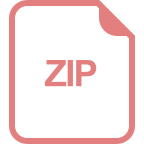
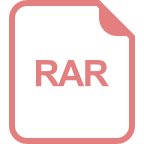
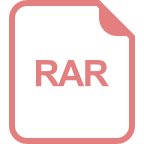
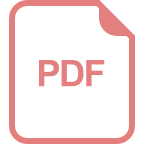
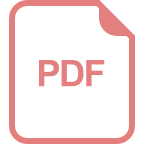
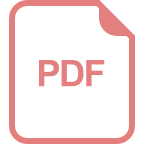
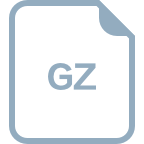
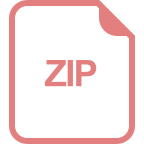
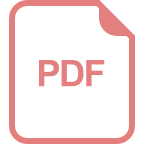
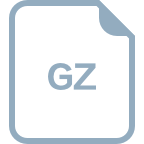
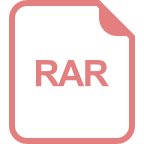
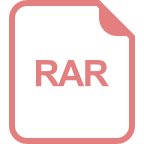
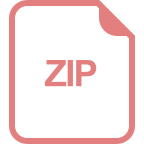
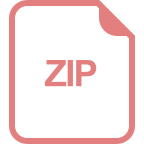
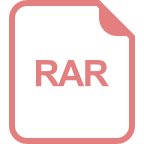
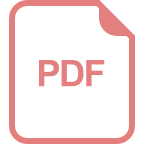
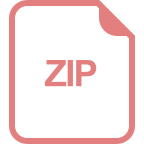
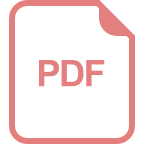
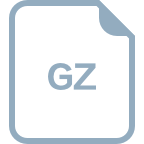
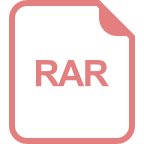
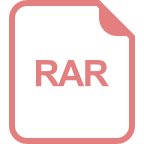
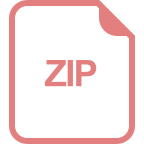
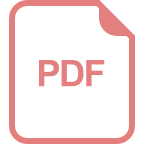
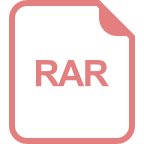
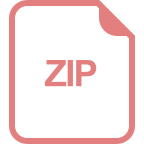
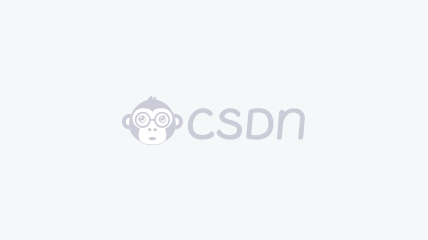

- 粉丝: 8
- 资源: 895
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

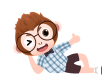
最新资源
- 编译原理大作业-简易c语言编译器.zip
- DELPHI d12 开发的温度计,没有使用第三方控件
- 简单的C语言http服务器.zip
- 简单版贪吃蛇小游戏由c语言实现.zip
- 简单、易用、稳定、高效,具有扩展和集成的,大语言模型工程化开发框架.zip
- 程序设计基础课程设计-基于C语言的简易Windows平台Dos超市管理系统.zip
- 百灵微信公众号管理平台,是一款开源、免费的微信公众号管理系 采用JAVA语言,基于Jfinal开发,支持微信公众号、微信企业号等多账号简单的模拟管理和操作,使用用户可以进行二次开发 .zip
- Python毕业设计中小微企业信贷决策模型及算法研究项目源码+论文(高分项目)
- 甲语言是一门对机器码助记语言,让人更容易理解的语言,发明目的是为了开发操作系统 .zip
- adb常用命令!!!.xmind

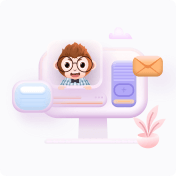
