UIAlertTableView 里进行单选和多选的代码例子
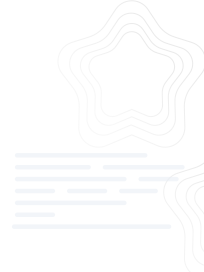

在iOS开发中,UIAlertController是苹果提供的一种用于展示警告或者操作表单的API,它可以包含一个或多个按钮,但默认情况下并不支持用户进行多选或单选操作。`UIAlertTableView`是一个自定义的解决方案,它扩展了UIAlertController的功能,允许在弹出的对话框中集成UITableView,以实现类似选择器的效果,支持单选或多选。这篇博客通过代码示例展示了如何使用UIAlertTableView来完成这一功能。 我们需要创建一个自定义的UIAlertController,继承自UIAlertController,并添加UITableView作为其子视图。在初始化方法中,我们可以设置表格的数据源和代理,以便展示数据并处理用户的交互。 ```swift class UIAlertTableView: UIAlertController { var tableView: UITableView! init(title: String?, message: String?, preferredStyle: UIAlertController.Style, data: [String], selectionHandler: @escaping ([String]) -> Void) { super.init(title: title, message: message, preferredStyle: preferredStyle) // 初始化tableView tableView = UITableView() tableView.dataSource = self tableView.delegate = self tableView.register(UITableViewCell.self, forCellReuseIdentifier: "cell") view.addSubview(tableView) // 设置数据源 self.data = data // 设置选择回调 self.selectionHandler = selectionHandler } } ``` 接下来,我们需要实现UITableViewDataSource和UITableViewDelegate协议,以便填充表格并处理用户的选中状态: ```swift extension UIAlertTableView: UITableViewDataSource { func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int { return data.count } func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell { let cell = tableView.dequeueReusableCell(withIdentifier: "cell", for: indexPath) cell.textLabel?.text = data[indexPath.row] cell.accessoryType = .none return cell } } extension UIAlertTableView: UITableViewDelegate { func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath) { tableView.deselectRow(at: indexPath, animated: true) let cell = tableView.cellForRow(at: indexPath)! cell.accessoryType = cell.isSelected ? .checkmark : .none } func tableView(_ tableView: UITableView, didDeselectRowAt indexPath: IndexPath) { let cell = tableView.cellForRow(at: indexPath)! cell.accessoryType = .none } func tableView(_ tableView: UITableView, commit editingStyle: UITableViewCell.EditingStyle, forRowAt indexPath: IndexPath) { if editingStyle == .delete { // 如果需要删除功能,这里可以处理 } } } ``` 我们可以通过这个自定义的UIAlertTableView来显示对话框,并在用户完成选择后获取结果: ```swift let alertController = UIAlertTableView(title: "请选择", message: nil, preferredStyle: .alert, data: ["选项1", "选项2", "选项3"]) { (selectedItems) in print("用户选择了:\(selectedItems)") } UIApplication.shared.keyWindow?.rootViewController?.present(alertController, animated: true, completion: nil) ``` 在上述代码中,`UIAlertTableView`类提供了一个构造函数,接受标题、消息、样式、数据数组以及一个闭包作为参数,该闭包会在用户完成选择时被调用。`UITableViewDataSource`和`UITableViewDelegate`协议的实现确保了表格的正确展示和用户交互。通过这种方式,我们可以轻松地在UIAlertController中实现单选或多选功能,而无需依赖第三方库。这个自定义组件的灵活性使得在各种场景下都可以方便地使用,如设置、权限请求等。
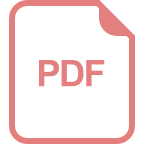
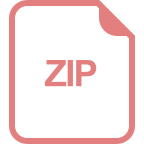
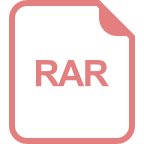
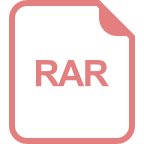
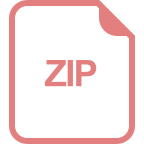
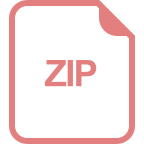
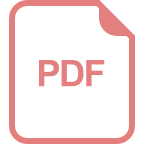
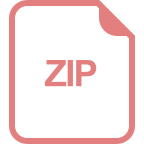
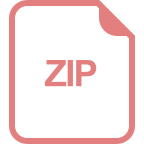
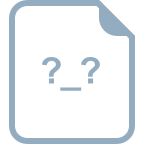
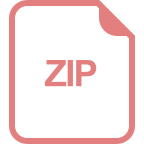
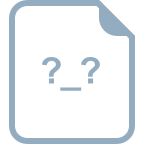
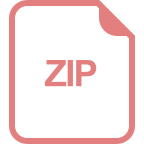
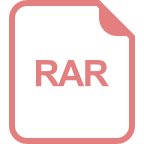
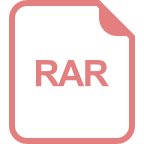

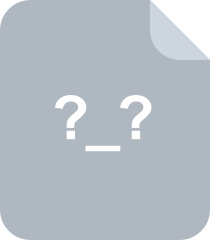
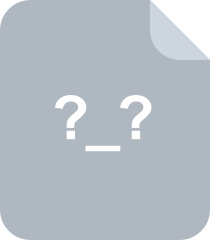
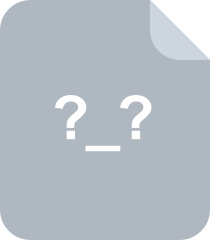
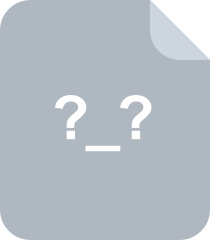
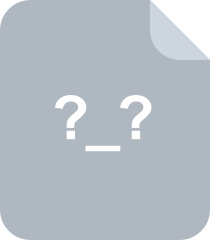
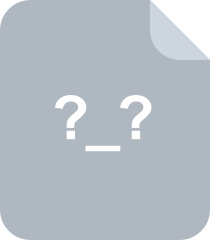
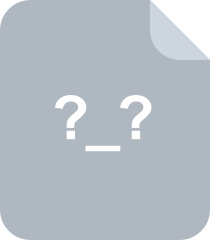
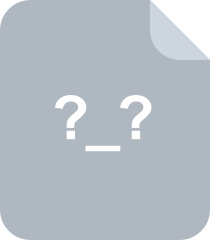
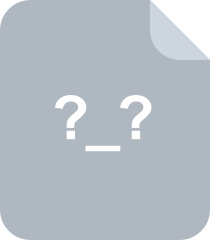
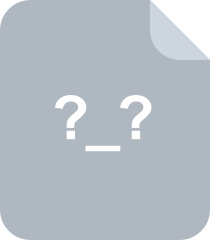
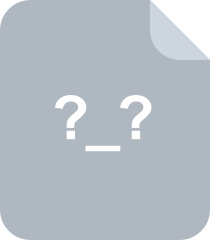
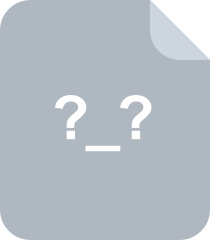
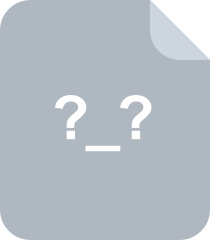
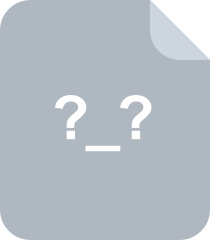
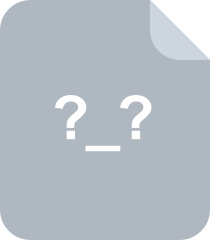
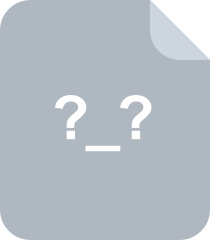
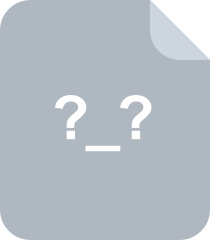
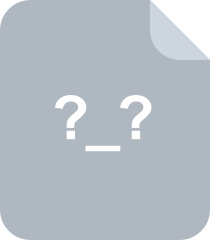
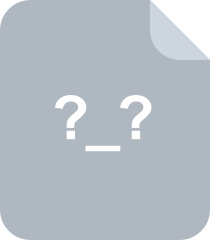
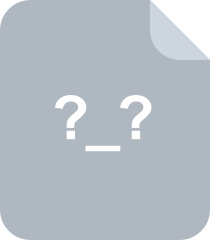
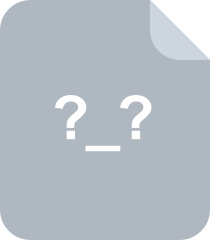
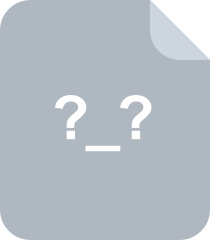
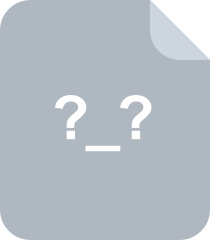
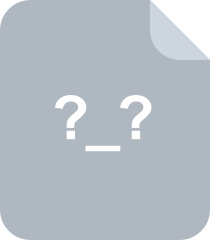
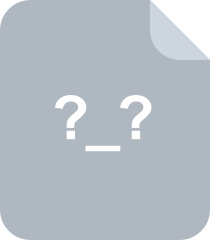
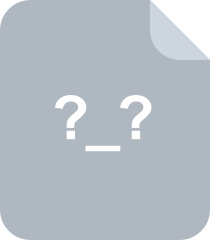
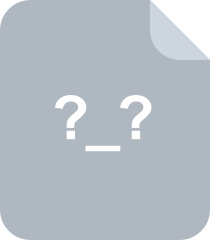
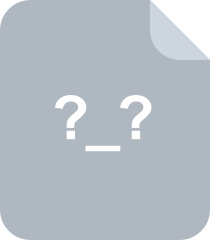
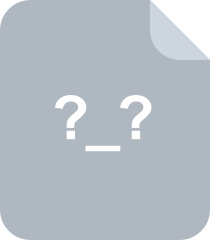
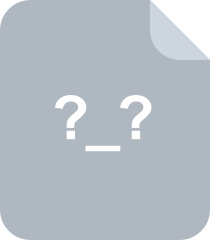
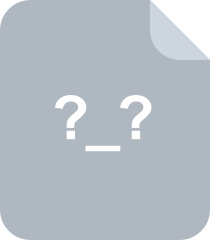
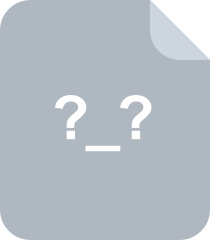
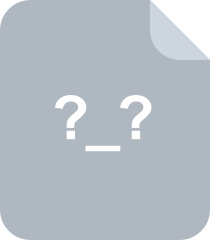
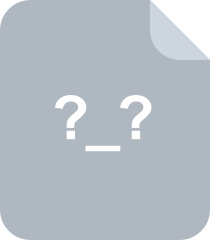
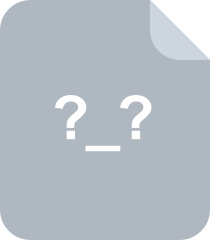
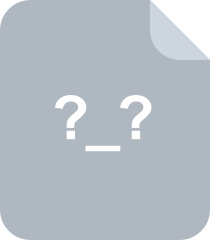
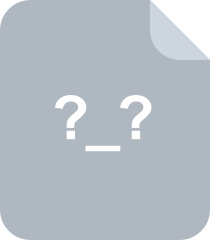
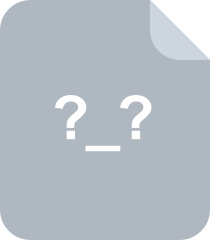
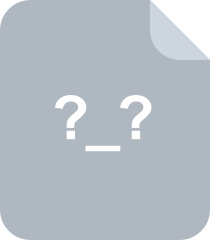
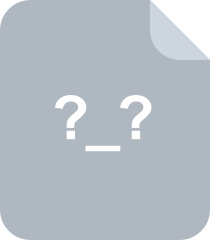
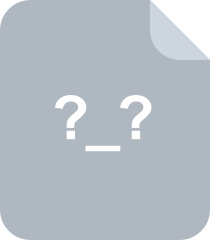
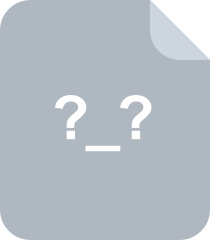
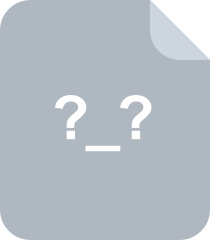
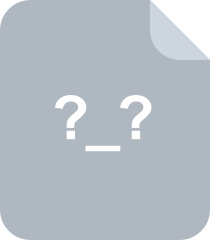
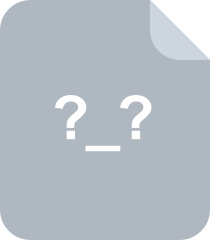
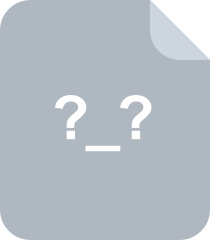
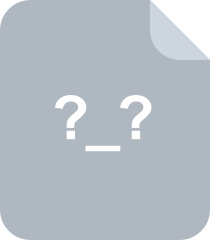
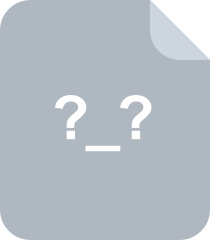
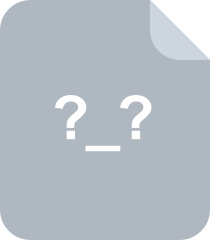
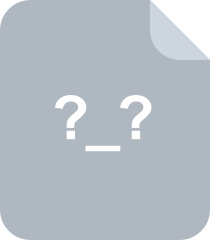
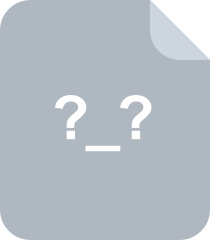
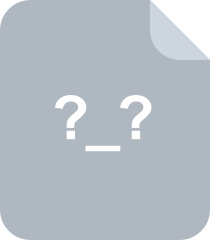
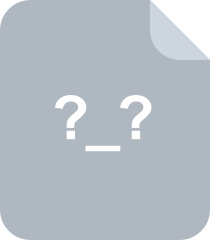
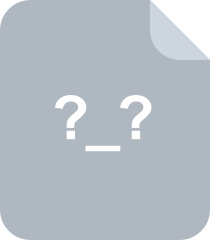
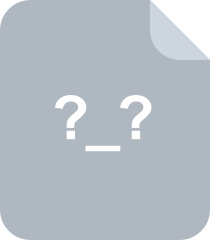
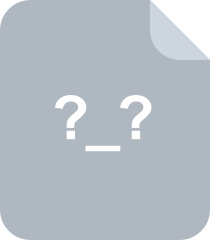
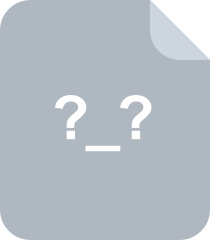
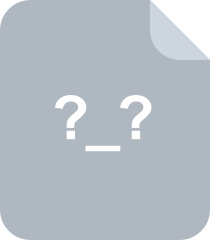
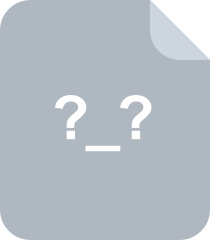
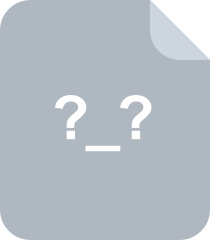
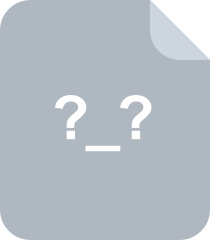
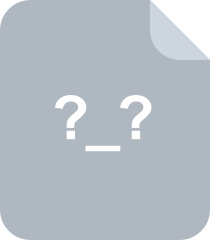
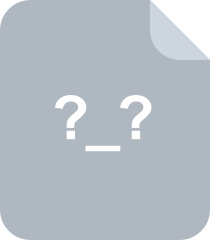
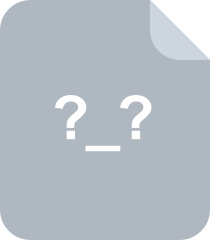
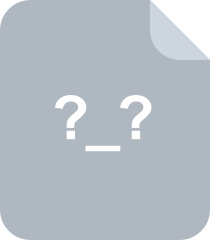
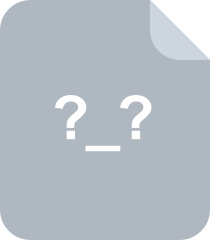
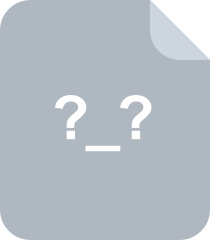
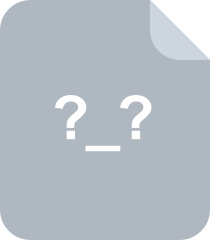
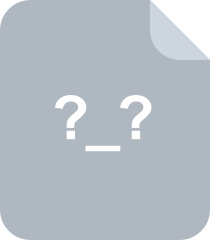
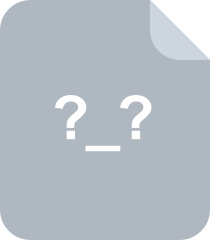
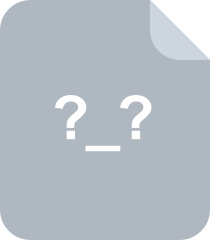
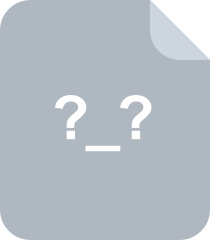
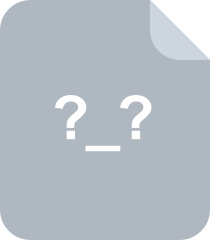
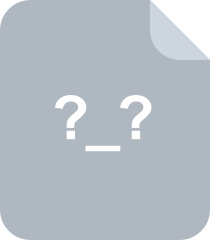
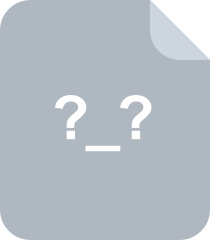
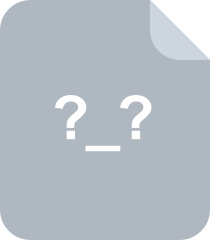
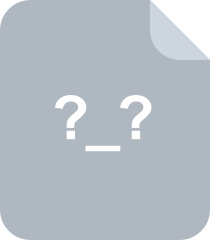
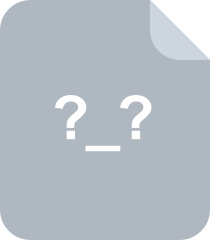
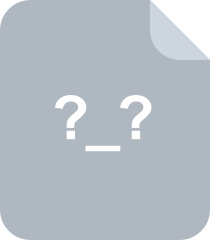
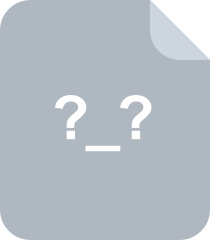
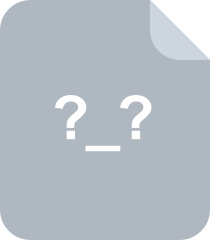
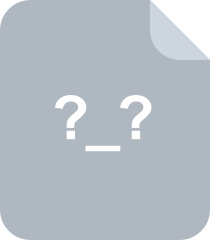
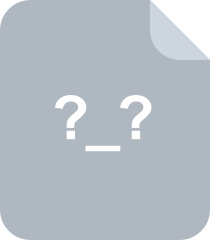
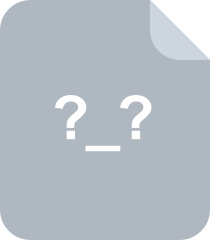
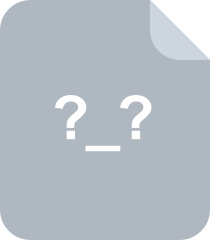
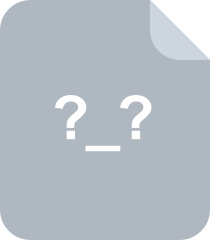
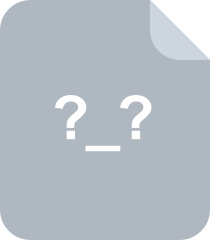
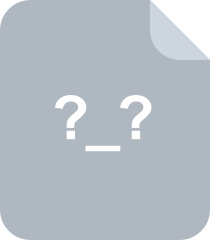
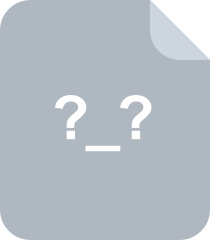
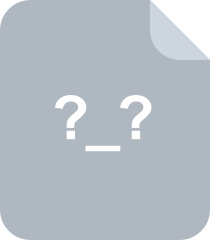
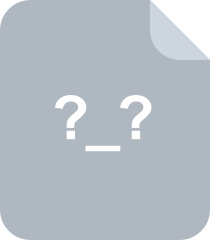
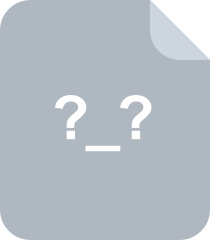
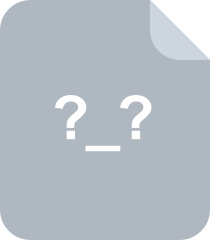
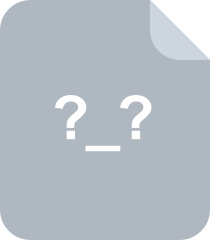
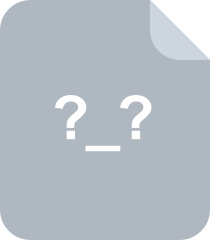
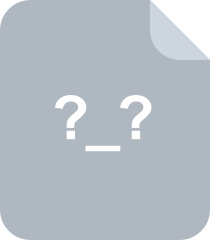
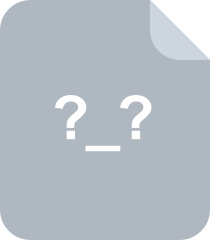
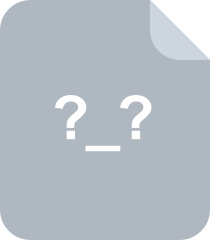
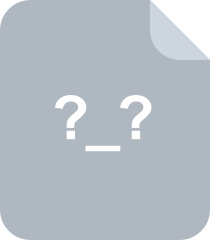
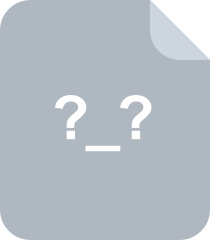
- 1
- 2
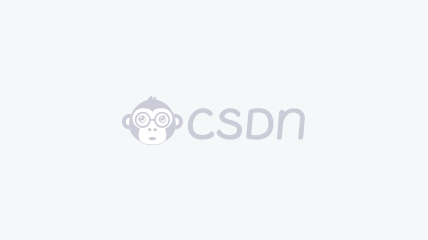

- 粉丝: 387
- 资源: 6万+
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

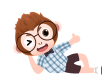
最新资源

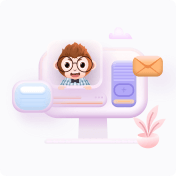
