用注解的方式进行SpringAOP开发
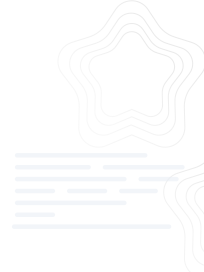

在Spring框架中,AOP(面向切面编程)是一种强大的设计模式,它允许开发者将关注点分离,将横切关注点(如日志、事务管理)从核心业务逻辑中解耦出来。本篇我们将深入探讨如何使用注解的方式来实现Spring AOP开发。 ### 一、注解基础 在Spring AOP中,主要使用以下几种注解: 1. `@Aspect`:定义一个切面类,切面是AOP的核心,包含通知(advisors)和切点(pointcuts)。 2. `@Before`:前置通知,方法在目标方法执行前运行。 3. `@After`:后置通知,无论目标方法是否正常结束,都会执行。 4. `@AfterReturning`:返回后通知,目标方法正常结束时运行。 5. `@AfterThrowing`:异常后通知,目标方法抛出异常时运行。 6. `@Around`:环绕通知,最灵活的通知类型,可以在方法调用前后自定义逻辑,甚至决定是否调用目标方法。 7. `@Pointcut`:定义切点表达式,可以匹配一系列的方法。 ### 二、创建切面 我们需要创建一个切面类,通过`@Aspect`注解标记: ```java import org.aspectj.lang.annotation.Aspect; import org.aspectj.lang.annotation.Pointcut; @Aspect public class LoggingAspect { // 定义一个切点,匹配com.example.service.*.*()的所有方法 @Pointcut("execution(com.example.service..*.*(..))") public void serviceMethods() {} } ``` ### 三、定义通知 然后,在切面类中定义通知方法,并使用相应的注解指定执行时机: ```java import org.aspectj.lang.ProceedingJoinPoint; import org.aspectj.lang.annotation.Around; import org.aspectj.lang.annotation.Aspect; import org.aspectj.lang.annotation.Before; import org.aspectj.lang.annotation.After; import org.aspectj.lang.annotation.AfterReturning; import org.aspectj.lang.annotation.AfterThrowing; @Aspect public class LoggingAspect { // 前置通知 @Before("serviceMethods()") public void beforeServiceCall() { System.out.println("Service method is about to be called."); } // 返回后通知 @AfterReturning(pointcut = "serviceMethods()", returning = "result") public void afterServiceCall(Object result) { System.out.println("Service method finished successfully. Result: " + result); } // 异常后通知 @AfterThrowing(pointcut = "serviceMethods()", throwing = "ex") public void afterServiceException(Throwable ex) { System.out.println("Service method threw an exception: " + ex.getMessage()); } // 环绕通知 @Around("serviceMethods()") public Object aroundServiceCall(ProceedingJoinPoint joinPoint) throws Throwable { System.out.println("Before the service method execution."); Object result = joinPoint.proceed(); // 调用目标方法 System.out.println("After the service method execution."); return result; } } ``` ### 四、配置Spring 在Spring配置文件中启用AOP并注册切面: ```xml <aop:config> <aop:aspect ref="loggingAspect"> <!-- 配置通知 --> </aop:aspect> </aop:config> <bean id="loggingAspect" class="com.example.aspect.LoggingAspect"/> ``` 或者,在Spring Boot应用中,通过`@EnableAspectJAutoProxy`启用注解驱动的AOP: ```java import org.springframework.context.annotation.Configuration; import org.springframework@EnableAspectJAutoProxy; @Configuration @EnableAspectJAutoProxy public class AppConfig { } ``` ### 五、运行与测试 现在,当`serviceMethods()`匹配的方法被调用时,相应的通知将会按预期执行。你可以创建一个服务类并调用其中的方法来测试AOP功能。 通过注解方式实现的Spring AOP使得代码更简洁,更易于理解和维护。这种方式避免了XML配置,使代码更加声明式,更符合现代Java开发的习惯。同时,Spring AOP提供了丰富的通知类型,可以根据实际需求灵活选择。理解并熟练掌握注解驱动的Spring AOP,对提升应用程序的可维护性和可扩展性具有重要意义。
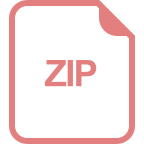
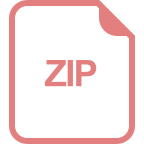
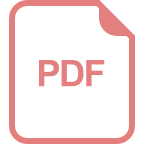
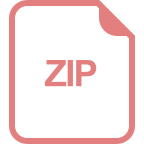
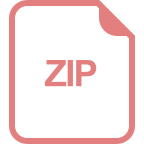
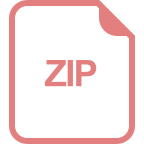
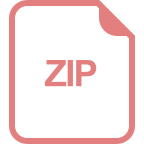
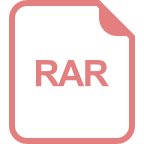
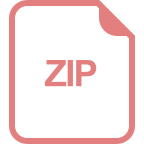
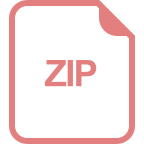
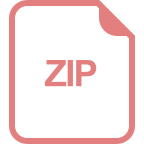
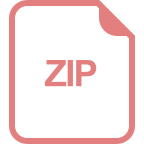
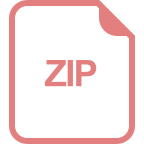
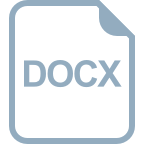
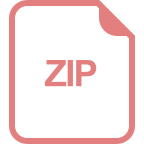
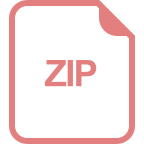
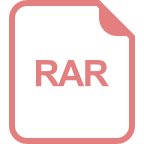
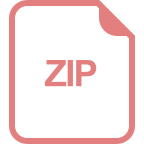
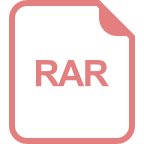


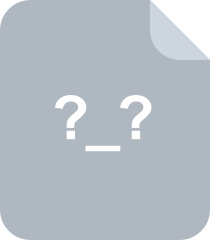
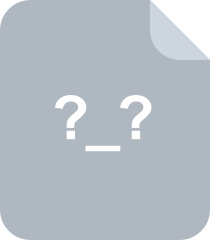
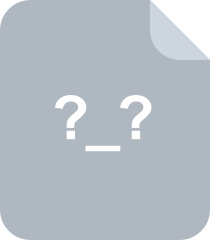

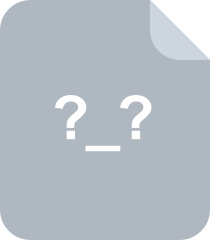


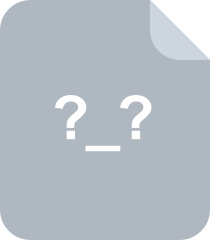



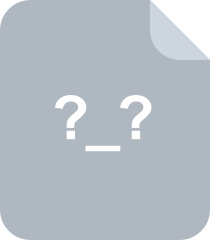

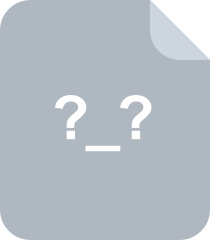

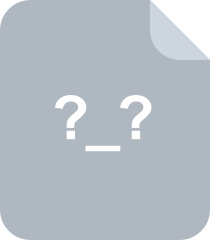



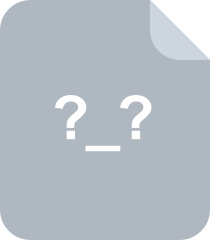


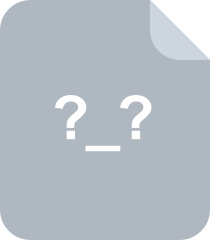



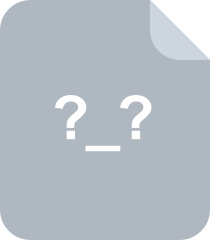

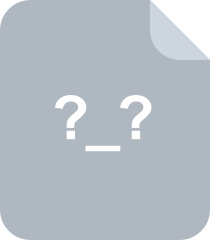

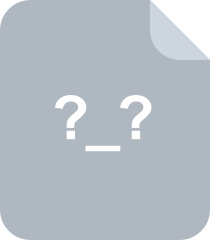

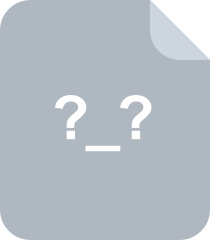
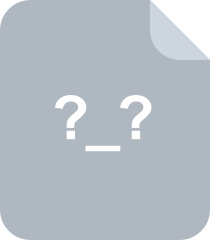

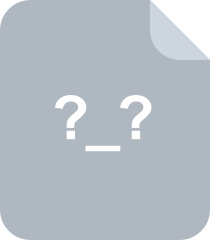

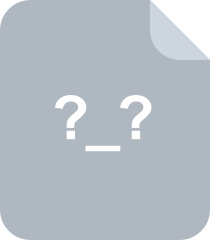
- 1
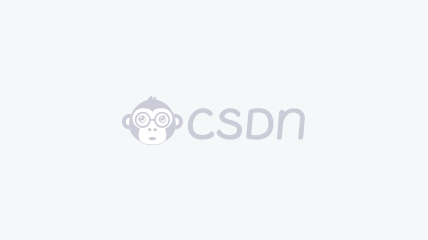

- 粉丝: 387
- 资源: 6万+
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

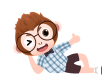
最新资源
- 東耳篮球馆会员信息管理系统(编号:98721117).zip
- 房屋系统(编号:45266146).zip
- 大学生志愿者信息管理系统(编号:96654262).zip
- 房屋租赁系统(编号:49930163).zip
- 付费自习室管理系统(编号:46724236)(1).zip
- 学术论文撰写技巧:施一公提高英文论文写作能力的六点建议
- 科研真问题从何而来-中科院院士分享
- 通过matlab语言读取csv文件.zip
- 通过Django实现用户注册和登录的简单认证系统.zip
- 通过汇编语言计算两个整数和,将结果存储在另一个变量中.zip
- Aruba%20Instant%20On_2.3.0_apk-dl.com.apk.1.1
- Ruby参考手册中文CHM版最新版本
- RubyonRails字符串处理中文最新版本
- 基于 selenium 模拟微博登录爬虫资料齐全+详细文档+源码.zip
- 基于chromeDriver+selenium蓝桥杯题库爬虫资料齐全+详细文档+源码.zip
- 基于java+selenium爬虫资料齐全+详细文档+源码.zip

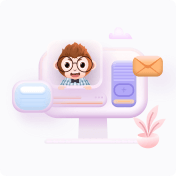
