C#在foreach遍历删除集合中元素的三种实现方法
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)

C#在foreach遍历删除集合中元素的三种实现方法 C#中,foreach遍历删除集合中元素是很常见的操作,但是删除元素时,集合的大小和元素索引值会发生变化,从而导致在foreach中删除元素时会抛出异常。下面我们将介绍三种实现方法,帮助大家更好地理解和使用C#。 方法一:采用for循环和倒序遍历 在使用foreach遍历删除集合中元素时,如果从头到尾正序遍历删除,可能会出现漏网之鱼的情况。例如,在列表中删除所有的某个元素,如果从头到尾遍历删除,可能会遗漏某些元素。解决这个问题的方法是使用for循环,并且从尾到头遍历循环。 ```csharp List<string> tempList = new List<string>() { "a","b","b","c" }; for (int i = tempList.Count-1; i>=0; i--) { if (tempList[i] == "b") { tempList.Remove(tempList[i]); } } tempList.ForEach(p => { Console.Write(p+","); }); ``` 控制台输出结果:a,c,这次删除了所有的b。 方法二:使用递归 使用递归方法可以解决foreach遍历删除集合中元素的问题。每次删除以后都从新foreach,这样就不存在这个问题了。 ```csharp static void Main(string[] args) { List<string> tempList = new List<string>() { "a","b","b","c" }; RemoveTest(tempList); tempList.ForEach(p => { Console.Write(p+","); }); } static void RemoveTest(List<string> list) { foreach (var item in list) { if (item == "b") { list.Remove(item); RemoveTest(list); return; } } } ``` 控制台输出结果:a,c,正确,但是每次都要封装函数,通用性不强。 方法三:通过泛型类实现 使用泛型类可以实现IEnumerator接口,从而解决foreach遍历删除集合中元素的问题。 ```csharp public class RemoveClass<T> { RemoveClassCollection<T> collection = new RemoveClassCollection<T>(); public IEnumerator GetEnumerator() { return collection; } } public class RemoveClassCollection<T> : IEnumerable<T> { private List<T> list = new List<T>(); public void Add(T item) { list.Add(item); } public IEnumerator<T> GetEnumerator() { return list.GetEnumerator(); } IEnumerator IEnumerable.GetEnumerator() { return GetEnumerator(); } } static void Main(string[] args) { RemoveClass<Group> tempList = new RemoveClass<Group>(); tempList.Add(new Group() { id = 1, name="Group1" }) ; tempList.Add(new Group() { id = 2, name = "Group2" }); tempList.Add(new Group() { id = 2, name = "Group2" }); tempList.Add(new Group() { id = 3, name = "Group3" }); foreach (Group item in tempList) { if (item.id==2) { tempList.Remove(item); } } foreach (Group item in tempList) { Console.Write(item.id+","); } } ``` 控制台输出结果:1,3。 删除集合中元素时,使用for循环和倒序遍历、递归、泛型类实现IEnumerator接口等方法都可以解决foreach遍历删除集合中元素的问题。
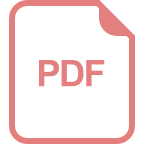
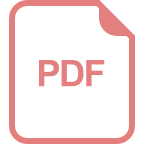
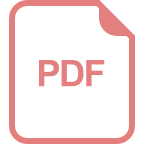
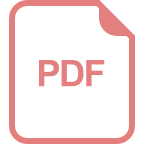
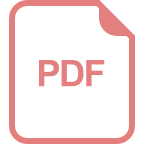
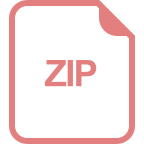
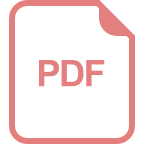
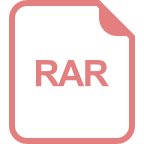
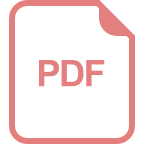
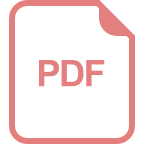
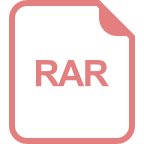
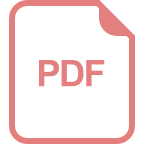
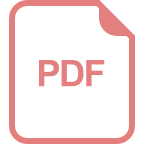
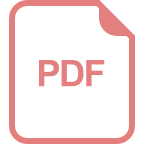
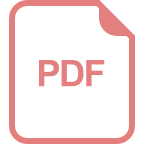
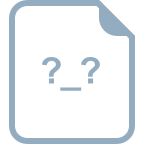
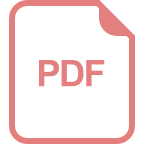
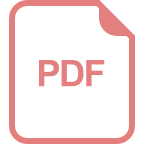
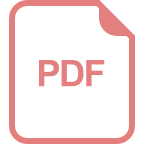
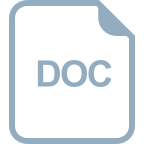
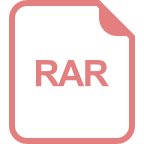
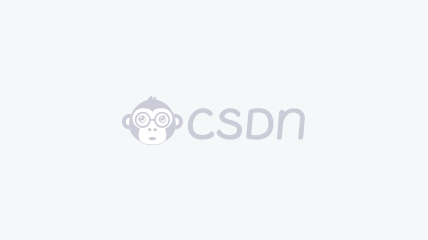
- 吉利吉利2023-06-14或网页,但您没有提供任何具体的问题或需求。请提供更多信息以便我能帮助您解决问题或满足需求。

- 粉丝: 1
- 资源: 957
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

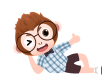
最新资源

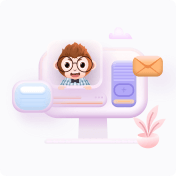
