package com.bootdo.front.delegate;
import com.alibaba.fastjson.JSONArray;
import com.alibaba.fastjson.JSONObject;
import com.bootdo.common.domain.DictDO;
import com.bootdo.common.domain.Tree;
import com.bootdo.common.service.DictService;
import com.bootdo.common.utils.DateTimeUtils;
import com.bootdo.common.utils.DateUtils;
import com.bootdo.common.utils.PageUtils;
import com.bootdo.common.utils.Query;
import com.bootdo.common.utils.R;
import com.bootdo.common.utils.ShiroUtils;
import com.bootdo.common.utils.StringUtils;
import com.bootdo.kinder.entity.CourseVO;
import com.bootdo.kinder.entity.PeriodVO;
import com.bootdo.kinder.entity.SchoolLunchVO;
import com.bootdo.kinder.entity.StudentInfoVO;
import com.bootdo.kinder.entity.TeacherVO;
import com.bootdo.kinder.service.CourseService;
import com.bootdo.kinder.service.PeriodService;
import com.bootdo.kinder.service.SchoolLunchService;
import com.bootdo.kinder.service.StudentInfoService;
import com.bootdo.kinder.service.TeacherService;
import com.bootdo.oa.domain.NotifyDO;
import com.bootdo.oa.service.NotifyRecordService;
import com.bootdo.oa.service.NotifyService;
import com.bootdo.system.domain.MenuDO;
import com.bootdo.system.domain.RoleDO;
import com.bootdo.system.domain.UserDO;
import com.bootdo.system.service.MenuService;
import com.bootdo.system.service.RoleService;
import com.bootdo.system.service.UserService;
import com.bootdo.system.vo.UserVO;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import springfox.documentation.spring.web.json.Json;
import java.util.ArrayList;
import java.util.Date;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
@Component
public class AppReqDelegate {
private Logger logger = LoggerFactory.getLogger(AppReqDelegate.class);
@Autowired
private UserService userService;
@Autowired
MenuService menuService;
@Autowired
private TeacherService teacherService;
@Autowired
private StudentInfoService studentInfoService;
@Autowired
private DictService dictService;
@Autowired
private PeriodService periodService;
@Autowired
private SchoolLunchService schoolLunchService;
@Autowired
private CourseService courseService;
@Autowired
private RoleService roleService;
@Autowired
private NotifyService notifyService;
@Autowired
private NotifyRecordService notifyRecordService;
/**
* 登录系统
* @param username
* @param password
* @return
*/
public String login(JSONObject jsonObj){
logger.info("手机端开始登录...请求参数为:"+jsonObj);
String username = jsonObj.getString("userName");
String password = jsonObj.getString("password");
JSONObject resultJson = new JSONObject();
try{
UserDO userDo = userService.login(username, password);
List<Tree<MenuDO>> menus = menuService.listMenuTree(userDo.getUserId());
JSONArray menuArray = new JSONArray();
resultJson.put("menus",menuArray);
if (menus!=null&&menus.size()>0){
List<Tree<MenuDO>> children = menus.get(0).getChildren();
JSONObject menuJson = null;
for (Tree<MenuDO> tree : children){
menuJson = new JSONObject();
menuJson.put("menuId",tree.getId());
menuJson.put("menuName",tree.getText());
menuArray.add(menuJson);
}
resultJson.put("menus",menuArray);
}
String roleId = "";
String roleName = "";
List<Long> roleIds = userDo.getRoleIds();
if (roleIds!=null&&roleIds.size()>0){
roleId = roleIds.get(0)+"";
RoleDO roleDO = roleService.get(roleIds.get(0));
roleName = roleDO==null ? "" : roleDO.getRoleSign();
}
String loginId = userDo.getUserId()+"";
if ("teacher".equals(roleName)){
TeacherVO teacherVO = teacherService.get(loginId);
if (teacherVO!=null) {
loginId = teacherVO.getTId();
}
}else if ("parent".equals(roleName)){
StudentInfoVO studentInfoVO = studentInfoService.get(loginId);
if (studentInfoVO!=null){
loginId = studentInfoVO.getStuId();
}
}
resultJson.putAll(R.appOk());
resultJson.put("name",userDo.getName());
resultJson.put("roleName",roleName);
resultJson.put("userId",userDo.getUserId()+"");
resultJson.put("loginId",loginId);
resultJson.put("deptName",userDo.getDeptName());
resultJson.put("deptId",userDo.getDeptId());
resultJson.put("roleId",roleId);
}catch (Exception e){
resultJson.putAll(R.appOk("1",e.getMessage()));
}
logger.info("手机端结束登录...请求参数为:"+jsonObj);
return resultJson.toString();
}
/**
* 获取老师列表
* @param jsonObj
* @return
*/
public String getTeacherList(JSONObject jsonObj){
logger.info("开始获取老师列表...请求参数为:"+jsonObj);
Integer pageNo = jsonObj.getInteger("pagNo");
Integer pageSize = jsonObj.getInteger("pageSize");
Integer deptId = jsonObj.getInteger("classId");
JSONObject resultJson = new JSONObject();
JSONObject queryJson = new JSONObject();
queryJson.put("status","1");
queryJson.put("deptId",deptId);
Query query = new Query(queryJson,pageNo,pageSize);
PageUtils page = teacherService.findPage(query);
List<TeacherVO> teacherList = (List<TeacherVO>) page.getRows();
JSONArray teacherArray = new JSONArray();
JSONObject teacherJson = null;
for (TeacherVO teacherVO : teacherList){
teacherJson = teacherToJson(teacherVO);
teacherArray.add(teacherJson);
}
resultJson.putAll(R.appOk());
resultJson.put("teacherList",teacherArray);
resultJson.put("pageNo",pageNo);
resultJson.put("pageSize",pageSize);
resultJson.put("pageCount",page.getPageCount(pageSize));
logger.info("结束获取老师列表...请求参数为:"+jsonObj);
return resultJson.toString();
}
/**
* 获取学生列表
* @param jsonObj
* @return
*/
public String getStudentList(JSONObject jsonObj){
logger.info("开始获取学生列表...请求参数为:"+jsonObj);
Integer pageNo = jsonObj.getInteger("pagNo");
Integer pageSize = jsonObj.getInteger("pageSize");
Integer classId = jsonObj.getInteger("classId");
Object stuId = jsonObj.get("userId");//学生id
String roleName = jsonObj.getString("roleName");//用户标识
JSONObject resultJson = new JSONObject();
JSONObject queryJson = new JSONObject();
queryJson.put("status","1");
queryJson.put("sClasss",classId);
if ("parent".equals(roleName)){
queryJson.put("stuId", stuId);
}
Query query = new Query(queryJson,pageNo,pageSize);
PageUtils studentPage = studentInfoService.findPage(query);
List<StudentInfoVO> studentInfoList = (List<StudentInfoVO>) studentPage.getRows();
JSONArray studentArray = new JSONArray();
JSONObject studentJson = null;
for (StudentInfoVO studentInfoVO : studentInfoList){
studentJson = stuToJson(studentInfoVO);
studentArray.add(studentJson);
}
resultJson.putAll(R.appOk());
resultJson.put("studentList",studentArray);
resultJson.put("pageNo",pageNo);
resultJson.put("pageSize",pageSize);
resu
没有合适的资源?快使用搜索试试~ 我知道了~
java开发oa办公系统源码-kindergartenSystem:幼儿园系统
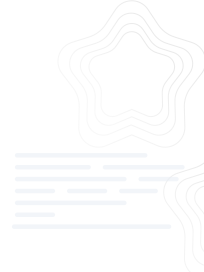
共1330个文件
js:458个
html:246个
java:222个

需积分: 29 4 下载量 128 浏览量
2021-06-05
11:18:06
上传
评论
收藏 12.36MB ZIP 举报
温馨提示
java开发oa办公系统源码 BootDo 面向学习型的开源框架 部分代码优化 主要将代码生成模块进行了优化,让生成的代码直接可以进行基础的CRUD操作 此项目作为模板使用,不产生业务逻辑修改 该项目修改后,使用Tomcat启动 平台简介 BootDo是高效率,低封装,面向学习型,面向微服的开源Java EE开发框架。 BootDo是在SpringBoot基础上搭建的一个Java基础开发平台,MyBatis为数据访问层,ApacheShiro为权限授权层,Ehcahe对常用数据进行缓存。 BootDo主要定位于后台管理系统学习交流,已内置后台管理系统的基础功能和高效的代码生成工具, 包括:系统权限组件、数据权限组件、数据字典组件、核心工具组件、视图操作组件、工作流组件、代码生成等。 前端界面风格采用了结构简单、性能优良、页面美观大气的Twitter Bootstrap页面展示框架。 采用分层设计、双重验证、提交数据安全编码、密码加密、访问验证、数据权限验证。 使用Maven做项目管理,提高项目的易开发性、扩展性。 BootDo目前包括以下四大模块,系统管理(SYS)模块、 内容管理(C
资源详情
资源评论
资源推荐
收起资源包目录

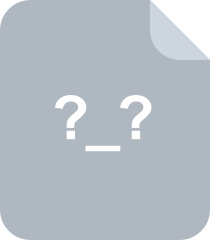
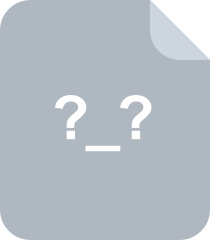
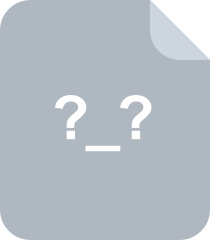
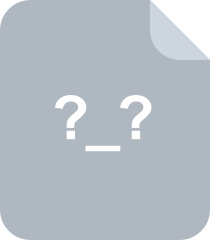
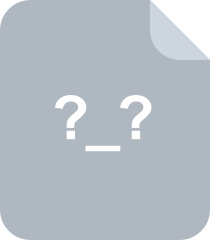
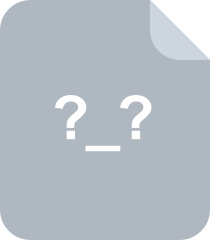
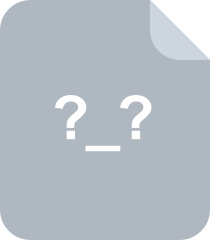
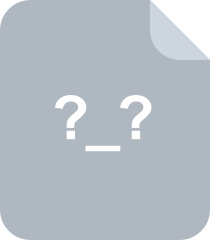
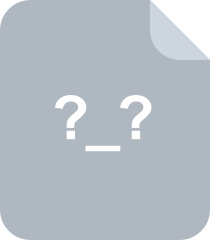
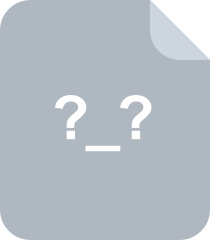
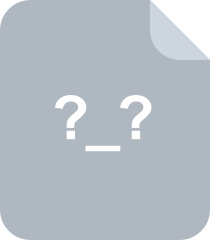
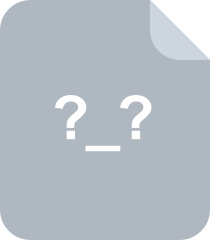
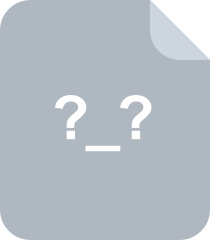
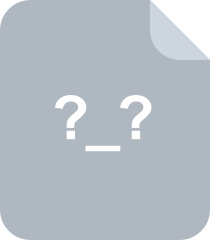
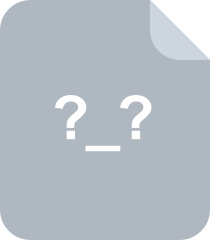
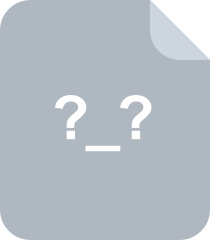
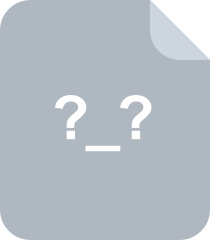
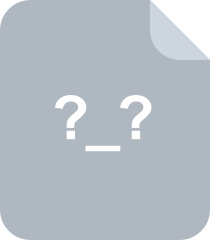
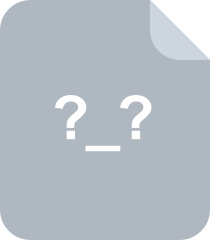
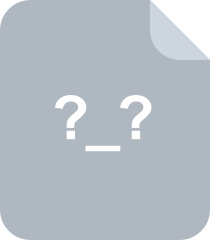
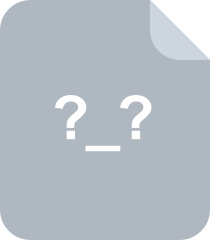
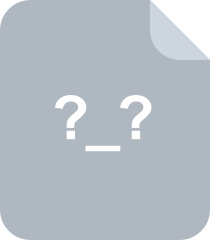
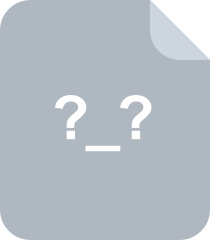
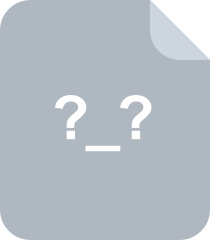
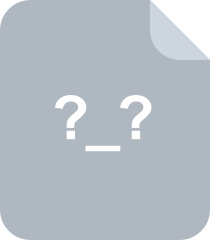
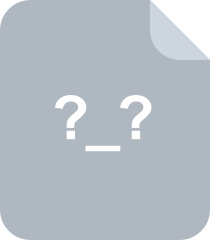
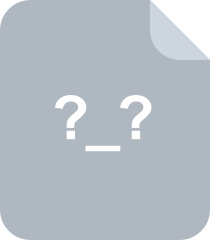
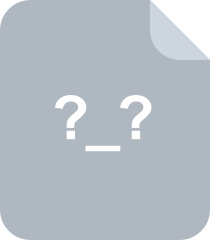
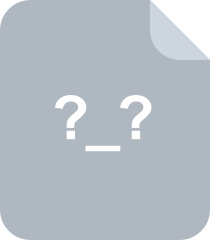
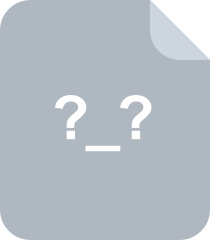
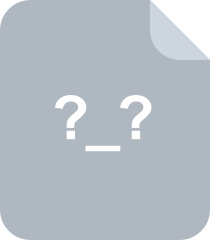
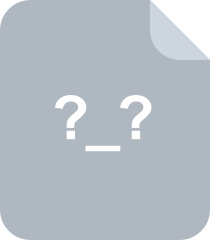
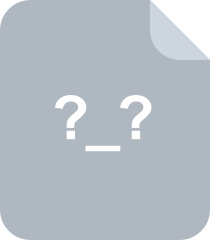
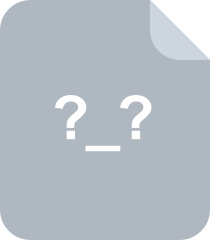
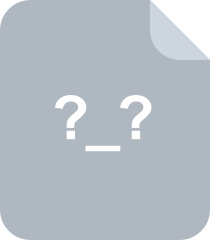
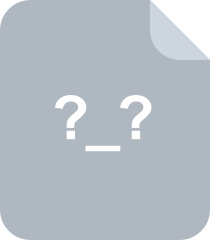
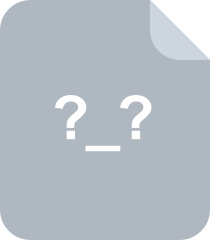
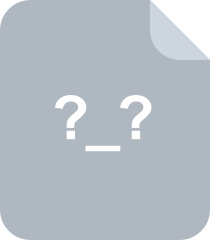
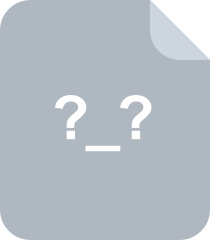
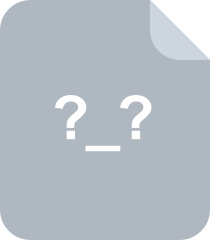
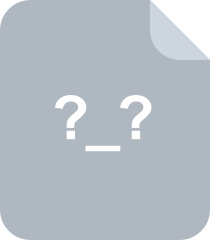
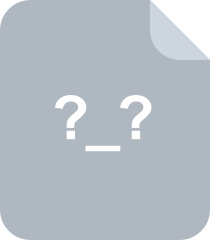
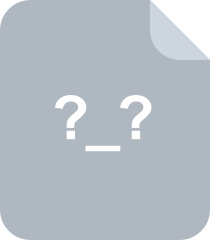
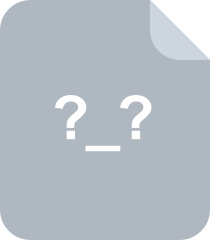
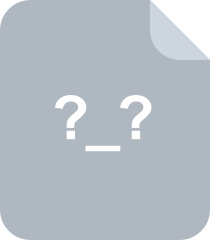
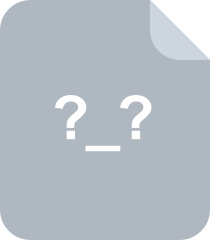
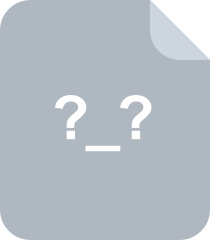
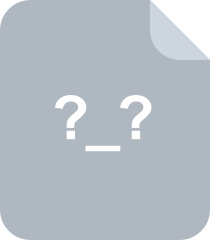
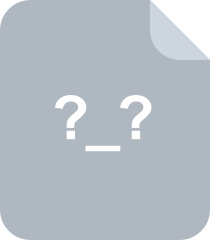
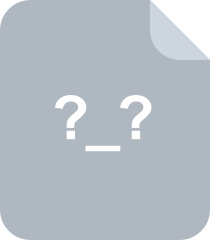
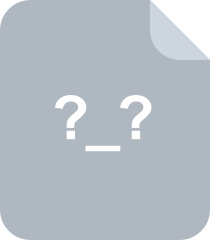
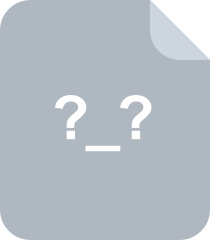
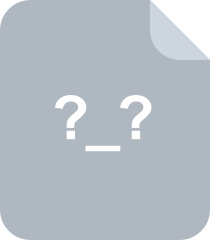
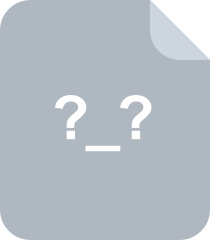
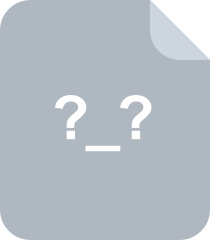
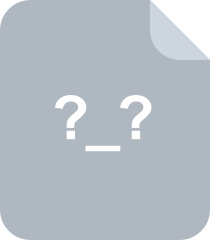
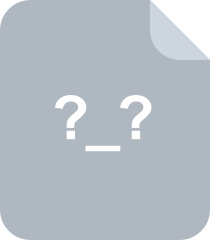
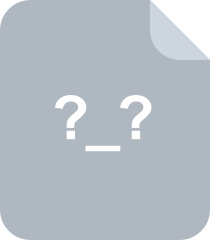
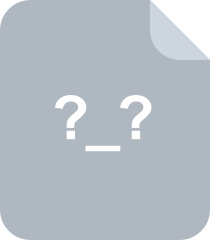
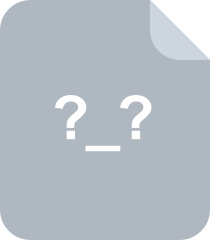
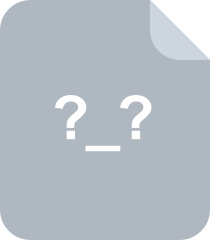
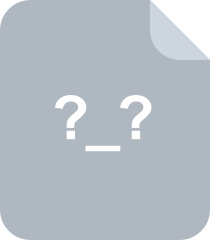
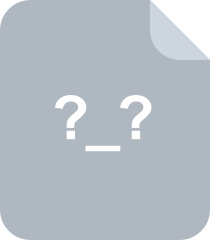
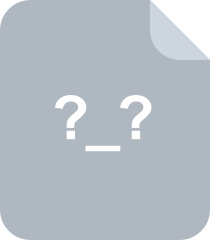
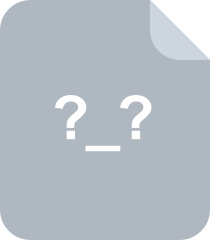
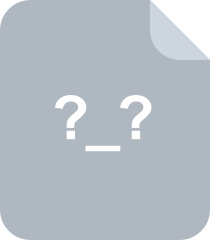
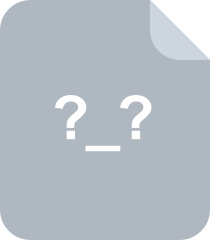
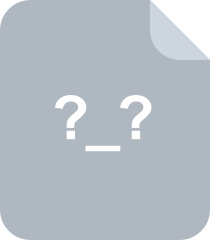
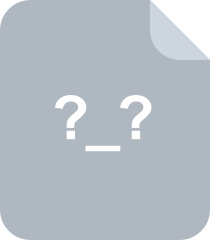
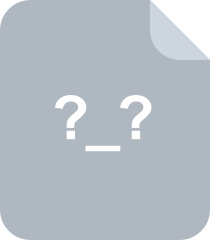
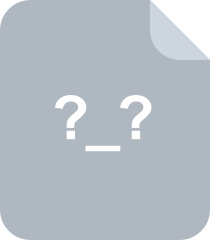
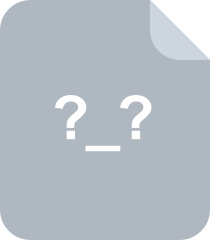
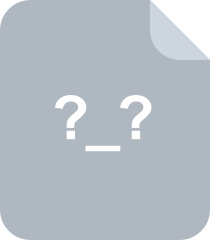
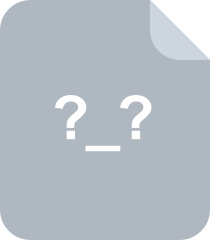
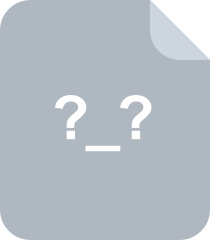
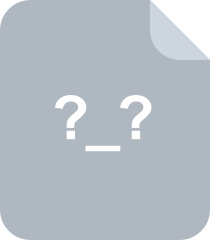
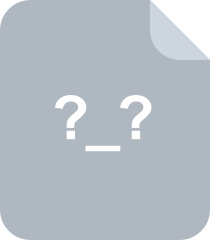
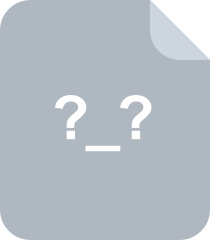
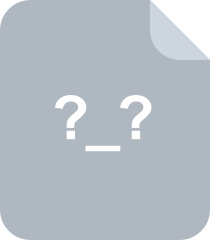
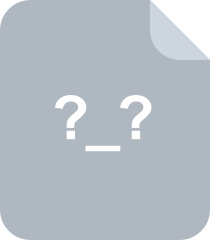
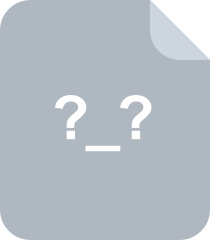
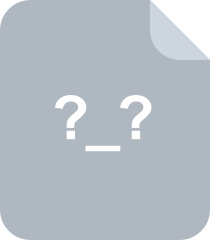
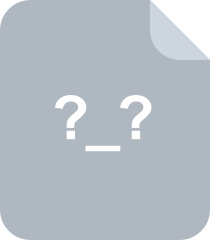
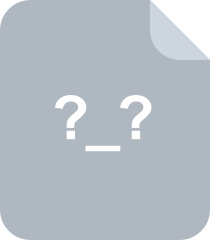
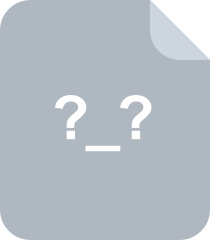
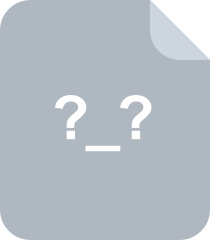
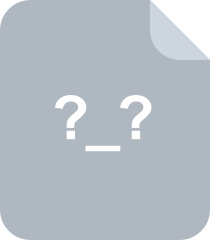
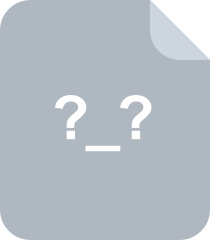
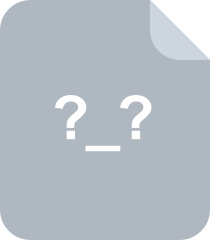
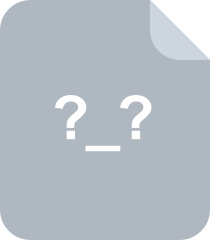
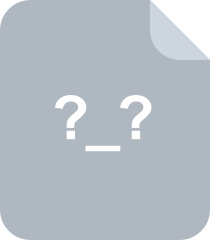
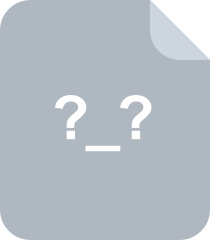
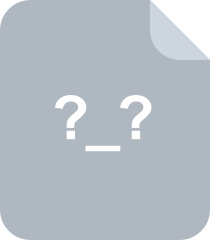
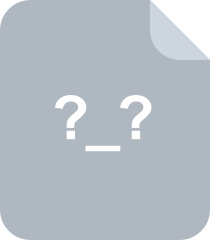
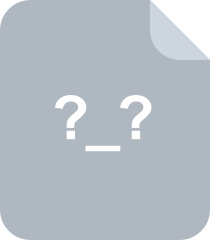
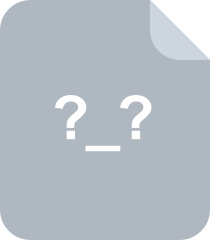
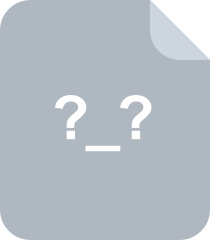
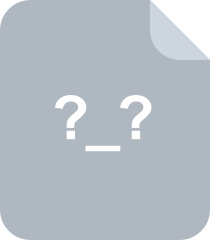
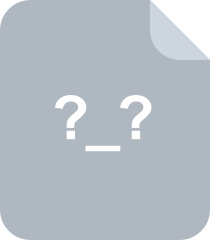
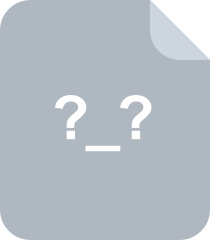
共 1330 条
- 1
- 2
- 3
- 4
- 5
- 6
- 14
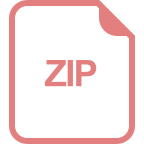
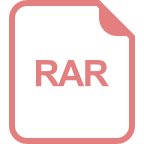
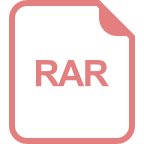
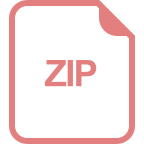
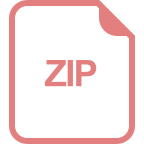
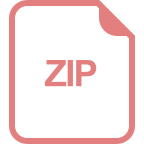
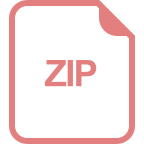
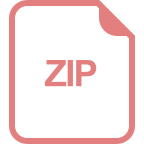
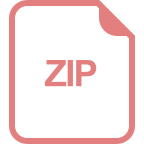
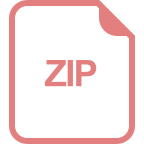
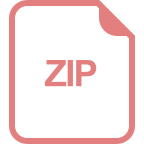
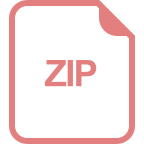
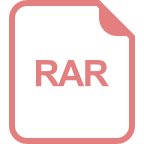
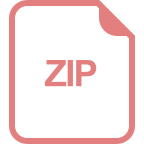
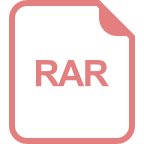

weixin_38620839
- 粉丝: 8
- 资源: 938
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

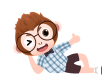
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


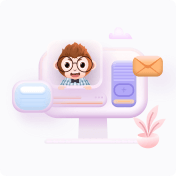
安全验证
文档复制为VIP权益,开通VIP直接复制

评论0