python中的itertools的使用详解
在Python编程语言中,`itertools` 是一个强大的内置模块,提供了各种高效的迭代器函数,用于处理序列数据。本文将详细介绍 `itertools` 模块的常用功能,并通过实例代码来展示它们的用法。 1. 无穷的迭代器 1.1 `count(start, [step])` `count()` 函数生成一个无限的序列,序列的值从 `start` 开始,每次增加 `step`。如果不提供 `step` 参数,默认值为1。 ```python from itertools import count c = count(0, 2) v = next(c) while v < 10: v = next(c) print(v, end=',') ``` 1.2 `cycle()` `cycle()` 会无限重复传入的序列,直至外部中断。 ```python from itertools import cycle c = cycle('ABCD') for i in range(10): print(next(c), end=',') ``` 1.3 `repeat(elem, [n])` `repeat()` 会重复迭代 `elem`,如果提供了 `n` 参数,则重复 `n` 次,否则无限重复。 ```python from itertools import repeat r = repeat(1, 3) for i in range(3): print(next(r), end=',') ``` 2. 迭代器 2.1 `accumulate(p, [func])` `accumulate()` 会对序列 `p` 中的元素进行累加,可以指定累加函数 `func`(默认为 `operator.add`)。 ```python from itertools import accumulate from operator import mul test_list = [i for i in range(1, 11)] for i in accumulate(test_list): print(i, end=',') print() for i in accumulate(test_list, mul): print(i, end=',') ``` 2.2 `chain()` `chain()` 可以合并多个迭代器为一个单一的迭代器。 ```python from itertools import chain ch = chain([1, 2, 3], {4: 4, 5: 5}, {6, 7, 8}, (9,), [10, [11, 12]]) for i in ch: print(i) ``` 2.3 `chain.from_iterable()` 与 `chain()` 不同,`chain.from_iterable()` 接受一个产生迭代对象的迭代器。 ```python from itertools import chain def gen_iterables(): for i in range(10): yield range(i) for i in chain.from_iterable(gen_iterables()): print(i) ``` 2.4 `compress(data, selectors)` `compress()` 会根据 `selectors` 中的元素选择 `data` 中相应的元素。 ```python from itertools import compress print(list(compress(['A', 'B', 'C', 'D'], [0, 1, 1, 1]))) ``` 2.5 `dropwhile(pred, seq)` `dropwhile()` 会在遇到第一个不满足条件 `pred` 的元素时开始迭代。 ```python from itertools import dropwhile l = [1, 7, 6, 3, 8, 2, 10] print(list(dropwhile(lambda x: x < 3, l))) ``` 2.6 `groupby()` `groupby()` 可以根据元素的某个属性对序列进行分组。 ```python from itertools import groupby # 对字符串进行分组 for k, g in groupby('11111234567'): print(k, list(g)) d = {1: 1, 2: 2, 3: 2} # 按照字典value来进行分组 for k, g in groupby(d, lambda x: d.get(x)): print(k, list(g)) ``` 2.7 `islice(seq, start, stop[, step])` `islice()` 用于切片迭代器,类似于列表切片。 ```python from itertools import islice s = 'abcdefg' for i in islice(s, 2, 5): print(i) ``` 2.8 `tee(iterable, n=2)` `tee()` 将一个迭代器复制成多个独立的迭代器,可以同时进行多次迭代。 ```python from itertools import tee it, it2 = tee(range(5)) for i in it: print(i, end=' ') print() for i in it2: print(i, end=' ') ``` 通过这些 `itertools` 函数,开发者可以更高效地处理序列数据,减少循环次数,提高代码的性能和可读性。理解并熟练运用 `itertools` 模块是每个Python程序员必备的技能之一。
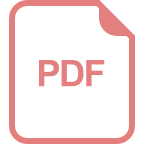
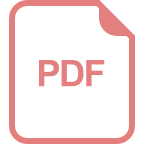
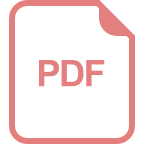
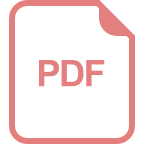
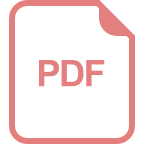
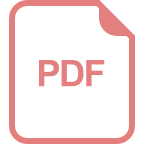
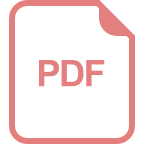
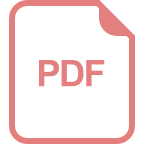
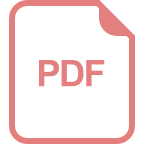
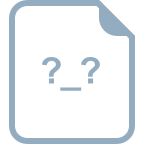
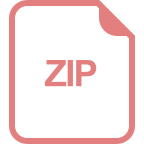
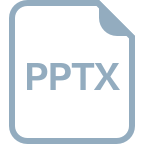
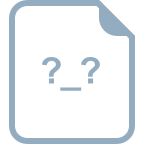
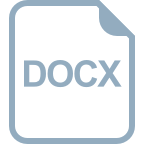
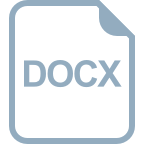
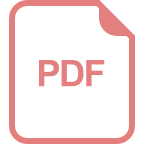
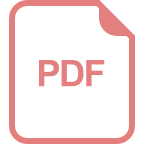
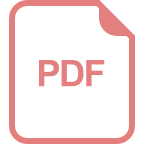
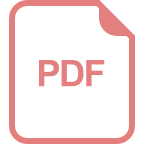
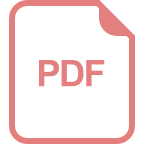
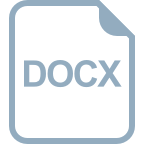
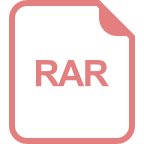
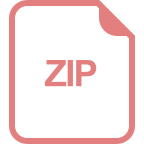
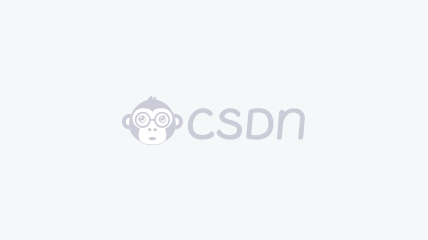

- 粉丝: 6
- 资源: 1017
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

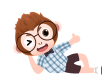
最新资源
- postman1111
- 快递盒打包机sw18可编辑全套技术资料100%好用.zip
- 画流程图程序的资源包,下载后npm执行就可以
- 基于OOD、JAVA和SWING的足球联赛管理系统软件的设计与实现
- 冷却收卷生产线(sw18可编辑+工程图+bom)全套技术资料100%好用.zip
- 雷达主板测试设备sw19可编辑全套技术资料100%好用.zip
- 龙门桁架sw18可编辑全套技术资料100%好用.zip
- 流水线贴标机sw18全套技术资料100%好用.zip
- 链式传输带sw18可编辑全套技术资料100%好用.zip
- CrystalDiskInfo可检测固态硬盘NAND写入量
- 龙门架双机械手sw18可编辑全套技术资料100%好用.zip
- SSD系列X2.zipSSD系列X2.zip
- 闪迪SSD管理工具Dashboard-V2.3.1.0.zip
- 龙门架快递搬运机器人sw18全套技术资料100%好用.zip
- 闪迪SSD工具Dashboard-v3.2.2.9.zip
- 计算机网络实验报告:使用Wireshark嗅探来自两个HTTP网站的数据包

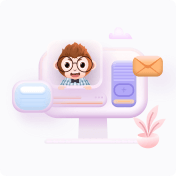
