决策树剪枝算法是机器学习中用于优化决策树模型的一种技术,目的是防止过拟合,提高模型泛化能力。在Python中实现决策树剪枝,通常会涉及到几个关键概念和算法,包括ID3、C4.5、CART等。 ID3算法是决策树构建的基础之一,它基于信息增益来选择最优属性进行节点划分。信息增益是衡量一个属性能带来多少信息减少,即减少了不确定性。ID3算法选择信息增益最高的属性作为分割依据,但容易偏向于选择取值较多的属性,因此存在过拟合风险。 C4.5是ID3的改进版本,通过信息增益率来解决ID3的偏向问题,信息增益率考虑了属性值的数量,使得选择更公平。C4.5还能处理连续型数据,通过设置阈值进行切分。 CART(Classification and Regression Trees)算法则用于构建分类和回归决策树,它使用基尼指数(Gini Index)作为划分标准。基尼指数衡量的是数据集的纯度,数值越小,纯度越高。对于分类任务,CART会选择划分后子集基尼指数之和最小的属性作为划分依据。 在Python中实现决策树剪枝,通常可以使用scikit-learn库,它提供了`DecisionTreeClassifier`和`DecisionTreeRegressor`类,支持预剪枝和后剪枝。预剪枝是在树生长过程中设定停止条件,如最大深度、最小样本数等,避免树过度复杂。后剪枝则是在树完全生长后,从叶子节点开始逐步回溯,通过比较剪枝前后的泛化误差来决定是否保留子树。 具体实现上,可以编写函数来计算熵、基尼指数,以及进行数据集的划分。例如,`calcShannonEnt`函数用于计算数据集的香农熵,`splitDataSet`函数用于根据特定特征值划分数据集。在训练决策树时,可以通过递归方式不断选择最佳分割属性,直到满足剪枝条件为止。 总结来说,决策树剪枝算法的Python实现主要包括以下几个步骤: 1. 定义计算熵和基尼指数的函数。 2. 实现数据集的划分功能,针对离散和连续特征。 3. 使用递归或循环构建决策树,每次选择最优分割属性。 4. 应用剪枝策略,如预剪枝或后剪枝,通过比较不同树结构的性能来确定最终模型。 在实际应用中,可以结合交叉验证、网格搜索等技术调整决策树的参数,找到最佳的剪枝策略和模型复杂度,以获得更好的泛化性能。
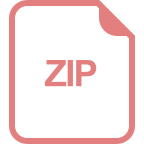
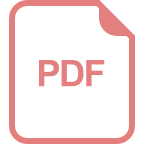
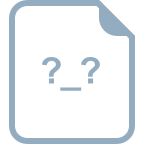
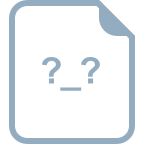
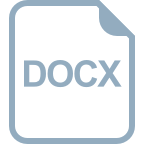
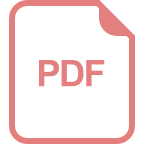
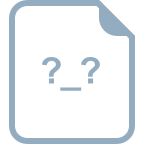
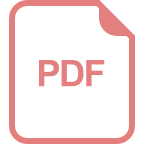
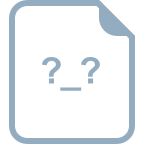
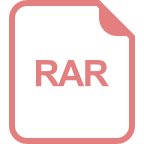
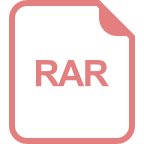
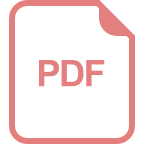
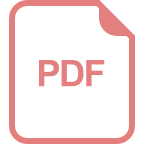
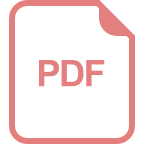
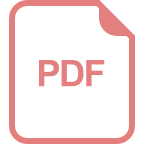
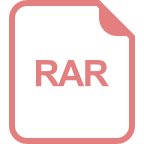
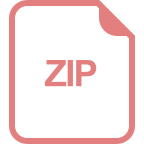
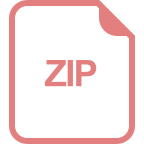
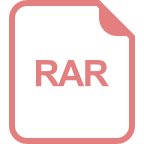
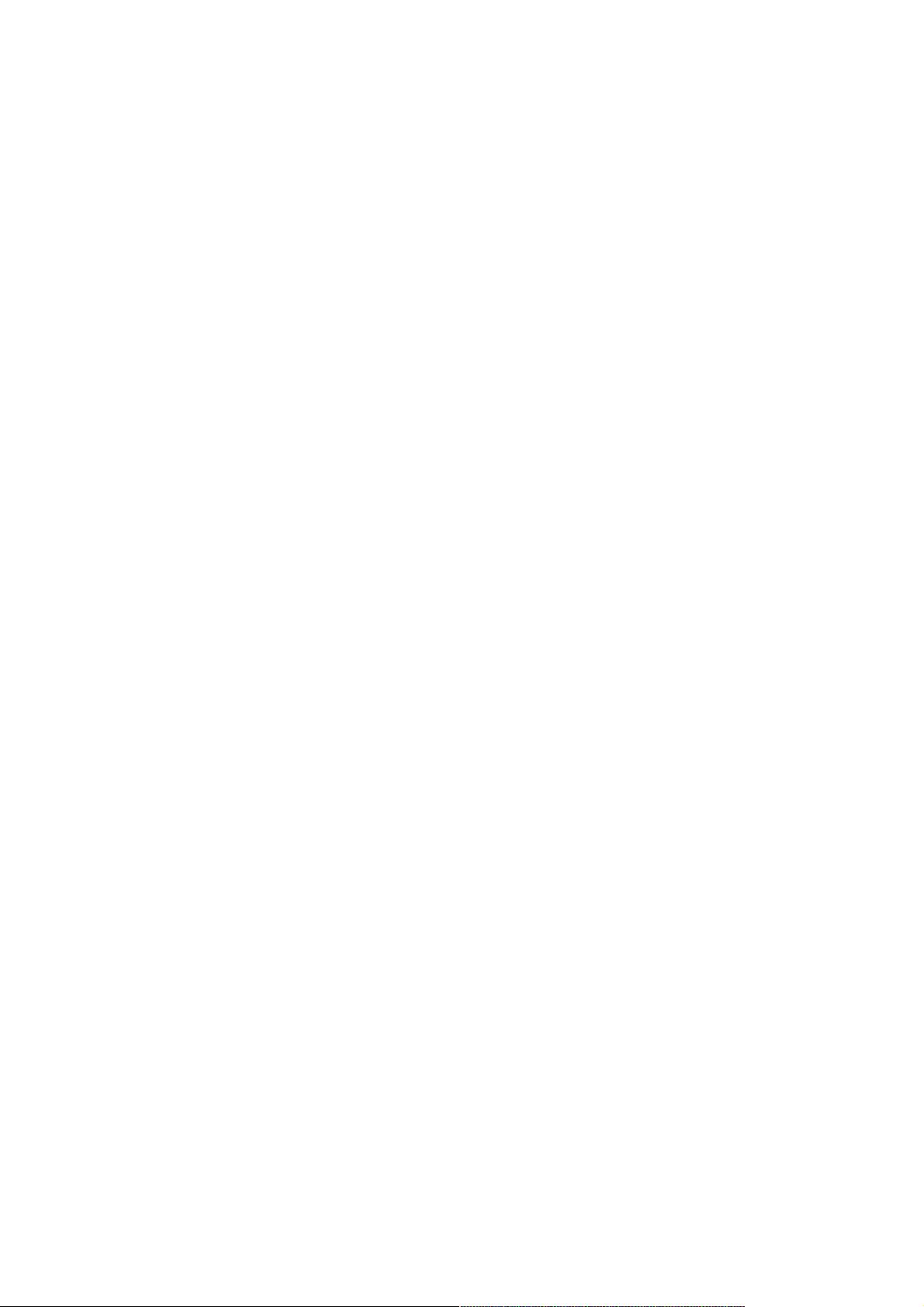
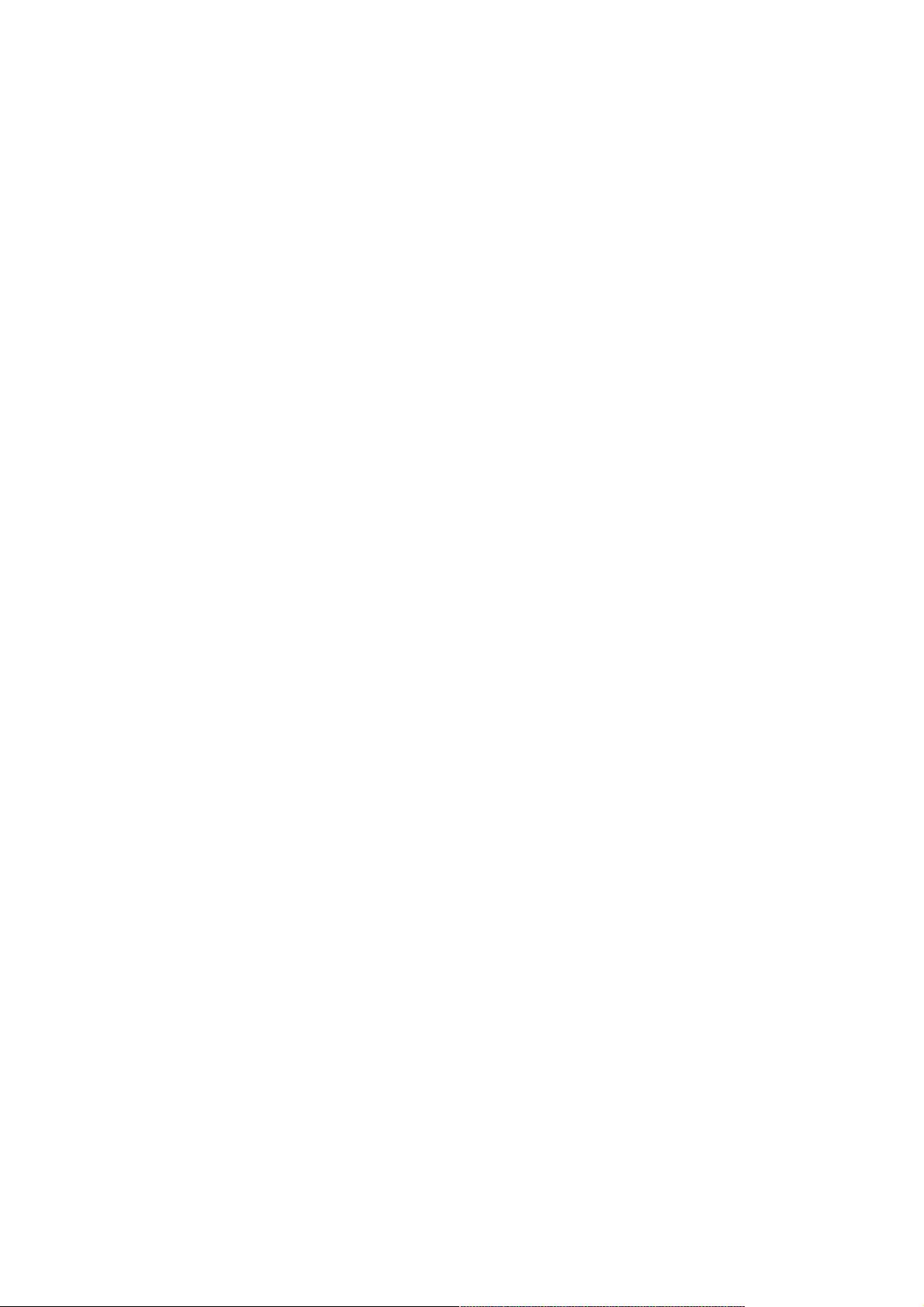
剩余6页未读,继续阅读
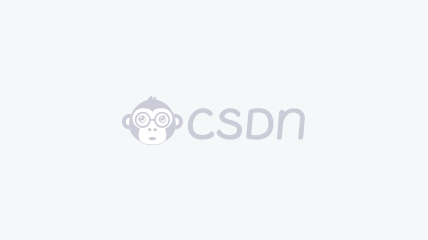
- zh2223332023-07-25这篇文章解决了我对决策树剪枝算法的疑惑,是一篇值得推荐的优秀文件。
- 杏花朵朵2023-07-25这篇文件详细介绍了决策树剪枝算法的python实现方法,让人受益匪浅。
- 柏傅美2023-07-25作者对算法原理进行了深入讲解,实例代码清晰易懂,能够帮助读者快速上手。
- XiZi2023-07-25文章不仅介绍了算法的基本思想,还给出了具体的实现步骤,方便读者实际应用。
- 优游的鱼2023-07-25文章简洁明了,语言质朴,容易理解,适合初学者阅读。

- 粉丝: 4
- 资源: 922
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

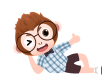
最新资源
- (源码)基于ESP32和AWS IoT Core的室内温湿度监测系统.zip
- (源码)基于Arduino的I2C协议交通灯模拟系统.zip
- coco.names 文件
- (源码)基于Spring Boot和Vue的房屋租赁管理系统.zip
- (源码)基于Android的饭店点菜系统.zip
- (源码)基于Android平台的权限管理系统.zip
- (源码)基于CC++和wxWidgets框架的LEGO模型火车控制系统.zip
- (源码)基于C语言的操作系统实验项目.zip
- (源码)基于C++的分布式设备配置文件管理系统.zip
- (源码)基于ESP8266和Arduino的HomeMatic水表读数系统.zip

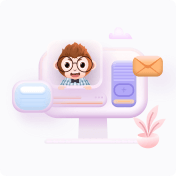
