在Spring Boot项目中集成邮件服务是为了实现自动化发送电子邮件的功能,这在许多业务场景中都非常有用,例如通知用户、发送验证码等。下面将详细介绍如何在Spring Boot中加入对邮件的支持。 为了使用Spring Boot的邮件服务,我们需要在项目的`pom.xml`或`build.gradle`文件中添加相关的依赖。对于Maven项目,你需要引入`spring-boot-starter-mail`模块: ```xml <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-mail</artifactId> </dependency> ``` 添加依赖后,接下来是配置邮件服务。在Spring Boot中,我们通常使用`application.properties`或`application.yml`文件来配置。这里以`application.properties`为例,你需要设置以下关键参数: ```properties # 设置邮箱主机 spring.mail.host=smtp.qq.com # 设置用户名(通常是你的邮箱地址) spring.mail.username=your_email@example.com # 设置密码(如果是QQ邮箱,应使用授权码) spring.mail.password=your_authorization_code # 端口,QQ邮箱通常使用465或587 spring.mail.port=465 # 是否需要认证 spring.mail.properties.mail.smtp.auth=true # 开启STARTTLS加密 spring.mail.properties.mail.smtp.starttls.enable=true spring.mail.properties.mail.smtp.starttls.required=true # 对于SSL连接,可以设置此属性 spring.mail.properties.mail.smtp.socketFactory.class=javax.net.ssl.SSLSocketFactory ``` 配置完成后,我们需要创建一个配置类`MailConfig.java`来实例化`JavaMailSender`并定义发送邮件的方法: ```java @Configuration public class MailConfig { @Resource private JavaMailSenderImpl mailSender; @Value("${spring.mail.username}") private String username; // 发送纯文本邮件 public void sendTextMail(String toEmail, String title, String content) { SimpleMailMessage msg = new SimpleMailMessage(); msg.setFrom(username); msg.setTo(toEmail); msg.setSubject(title); msg.setText(content); mailSender.send(msg); } // 发送HTML邮件 public void sendHtmlMail(String toEmail, String title, String htmlContent) throws MessagingException { MimeMessage message = mailSender.createMimeMessage(); MimeMessageHelper helper = new MimeMessageHelper(message, true); helper.setFrom(username); helper.setTo(toEmail); helper.setSubject(title); helper.setText(htmlContent, true); mailSender.send(message); } // 添加附件 public void sendMailWithAttachment(String toEmail, String title, String content, List<File> attachments) throws MessagingException { MimeMessage message = mailSender.createMimeMessage(); MimeMessageHelper helper = new MimeMessageHelper(message, true); helper.setFrom(username); helper.setTo(toEmail); helper.setSubject(title); helper.setText(content, true); for (File attachment : attachments) { FileSystemResource file = new FileSystemResource(attachment); helper.addAttachment(attachment.getName(), file); } mailSender.send(message); } } ``` 在这个配置类中,我们定义了三个方法:`sendTextMail`用于发送纯文本邮件,`sendHtmlMail`用于发送HTML格式的邮件,而`sendMailWithAttachment`则允许你添加附件。这些方法可以根据实际需求进行调用,例如在业务逻辑中触发邮件发送。 至此,你已经成功地在Spring Boot项目中集成了邮件服务。现在你可以编写代码来调用这些方法,根据需要发送各种类型的邮件。需要注意的是,如果你使用的是QQ邮箱,记得先在QQ邮箱的设置中开启SMTP服务,并获取授权码作为密码。 Spring Boot通过其强大的自动配置功能,使得集成邮件服务变得简单易行。只需几步简单的配置和代码编写,就能实现高效稳定的邮件发送功能。
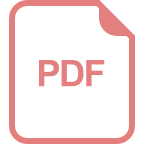
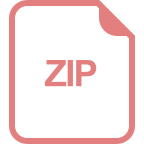
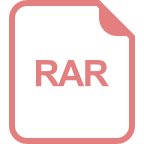
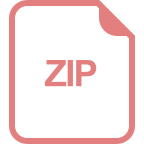
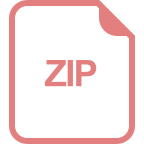
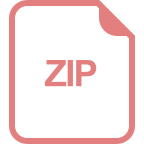
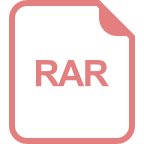
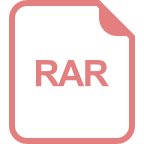
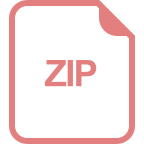
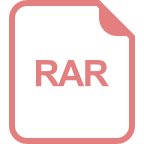
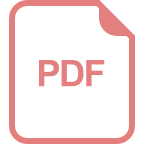
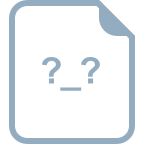
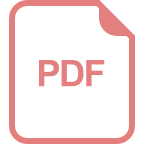
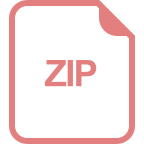
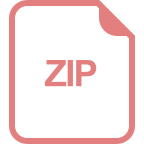
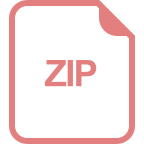
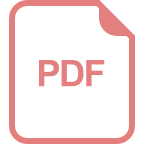
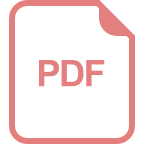
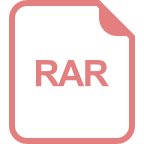
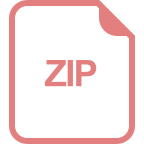
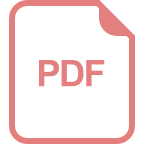
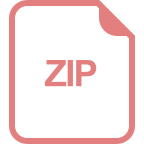
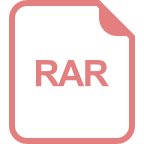
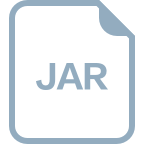
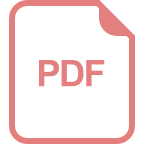
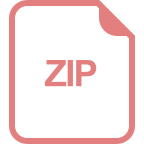
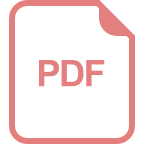
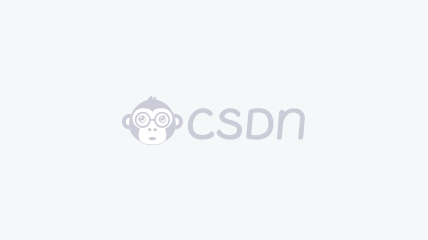

- 粉丝: 4
- 资源: 922
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

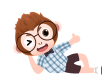
最新资源

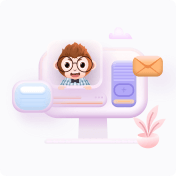
