在Android开发中,允许用户从图库选择图片是一项常见的功能,这对于创建具有用户交互性的应用至关重要。下面将详细讲解如何实现这一功能。 我们需要在布局文件中添加一个`ImageView`用于展示用户选择的图片,以及一个`Button`供用户触发选择图片的操作。以下是一个简单的布局示例: ```xml <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="fill_parent" android:layout_height="fill_parent"> <ImageView android:id="@+id/imgView" android:layout_width="fill_parent" android:layout_weight="1" android:layout_height="wrap_content" /> <Button android:layout_height="wrap_content" android:text="Load Picture" android:layout_width="wrap_content" android:id="@+id/buttonLoadPicture" android:layout_weight="0" android:layout_gravity="center" /> </LinearLayout> ``` 接下来,我们需要在`Button`的点击事件中启动一个`Intent`来打开图库。这个`Intent`使用`ACTION_PICK`动作,并指定`MediaStore.Images.Media.EXTERNAL_CONTENT_URI`作为数据源,以便让用户选择存储在设备上的图片。代码如下: ```java Intent i = new Intent(Intent.ACTION_PICK, android.provider.MediaStore.Images.Media.EXTERNAL_CONTENT_URI); startActivityForResult(i, RESULT_LOAD_IMAGE); ``` 这里的`RESULT_LOAD_IMAGE`是一个自定义常量,通常定义为一个整数值,用于在`onActivityResult()`方法中区分不同的请求结果。 当用户从图库中选择图片并返回后,系统会调用`onActivityResult()`方法。我们需要在这里处理返回的数据,将所选图片显示在`ImageView`上。首先检查请求码、结果码和数据是否有效,然后通过`getData()`获取到用户选择的图片的`Uri`。接着,执行SQL查询以获取图片的本地路径,最后使用`BitmapFactory.decodeFile()`将图片加载为`Bitmap`对象,并设置到`ImageView`上。完整的`onActivityResult()`方法如下: ```java @Override protected void onActivityResult(int requestCode, int resultCode, Intent data) { super.onActivityResult(requestCode, resultCode, data); if (requestCode == RESULT_LOAD_IMAGE && resultCode == RESULT_OK && null != data) { Uri selectedImage = data.getData(); String[] filePathColumn = { MediaStore.Images.Media.DATA }; Cursor cursor = getContentResolver().query(selectedImage, filePathColumn, null, null, null); cursor.moveToFirst(); int columnIndex = cursor.getColumnIndex(filePathColumn[0]); String picturePath = cursor.getString(columnIndex); cursor.close(); ImageView imageView = (ImageView) findViewById(R.id.imgView); imageView.setImageBitmap(BitmapFactory.decodeFile(picturePath)); } } ``` 至此,我们就完成了从图库中选取图片并显示在`ImageView`上的基本操作。需要注意的是,为了优化性能,可能需要考虑使用`Glide`或`Picasso`等库来加载图片,而不是直接使用`BitmapFactory.decodeFile()`,因为后者可能会导致内存溢出。此外,对于较大的图片,还应进行适当的缩放处理,以防止内存消耗过大。 Android应用程序中从图库选择图片涉及的关键知识点包括:`Intent`的使用,尤其是`ACTION_PICK`动作;`onActivityResult()`方法的处理;以及如何从`Uri`获取图片路径并加载到`ImageView`。理解并掌握这些知识点是构建用户友好型应用的基础。
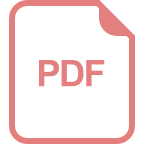
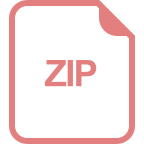
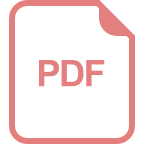
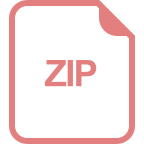
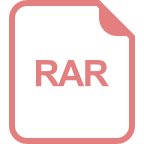
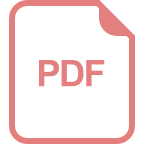
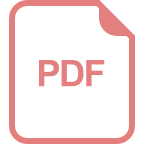
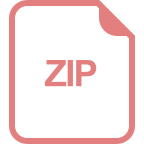
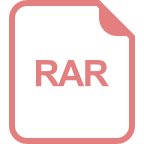
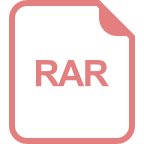
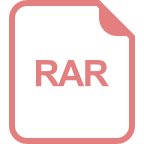
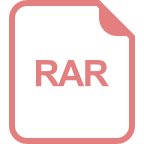
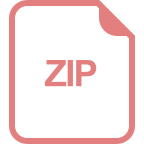
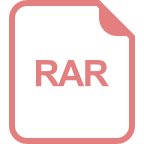
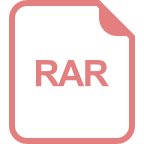
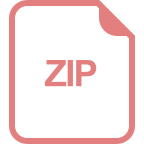
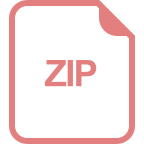
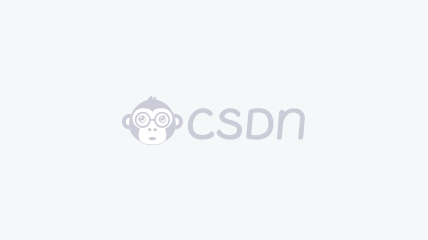

- 粉丝: 7
- 资源: 896
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

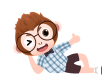
最新资源
- 人和箱子检测2-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- 清华大学2022年秋季学期 高等数值分析课程报告
- GEE错误集-Cannot add an object of type <Element> to the map. Might be fixable with an explicit .pdf
- 清华大学2022年秋季学期 高等数值分析课程报告
- 矩阵与线程的对应关系图
- 人体人员检测46-YOLO(v5至v9)、COCO、Darknet、TFRecord数据集合集.rar
- GEMM优化代码实现1
- java实现的堆排序 含代码说明和示例.docx
- 资料阅读器(先下载解压) 5.0.zip
- 人、垃圾、非垃圾检测18-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar

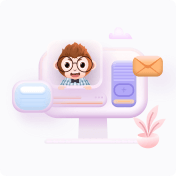
