Spring AOP(面向切面编程)是Spring框架的重要组成部分,它提供了在不修改源代码的情况下,对现有代码进行扩展和增强的功能。AOP的主要目的是解耦关注点,将横切关注点(如日志、事务管理、安全检查等)从核心业务逻辑中分离出来,使得代码更加模块化和易于维护。 在本文中,我们将通过一个简单的入门Demo来了解如何在Spring中使用AOP。我们需要创建一个Maven项目,并添加相关的依赖。在`pom.xml`文件中,我们需要引入Spring的核心库、Spring的上下文库、Spring的AOP库以及AspectJ的 weaving 库,这些依赖如下: ```xml <dependencies> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-core</artifactId> <version>4.0.5.RELEASE</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-beans</artifactId> <version>4.0.5.RELEASE</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>4.0.5.RELEASE</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-aop</artifactId> <version>4.0.5.RELEASE</version> </dependency> <dependency> <groupId>org.aspectj</groupId> <artifactId>aspectjweaver</artifactId> <version>1.8.1</version> </dependency> </dependencies> ``` 接下来,我们编写业务代码。这里创建了一个名为`Car`的类,其中有一个`go()`方法,表示汽车行驶的动作。 ```java package com.wowo.spring_aop_demo1; public class Car { public void go() { System.out.println("go go go!"); } } ``` 然后,我们编写切面类`CarLogger`,用于记录日志。切面类通常包含了通知(advice),在这里我们定义了两个方法:`beforeRun()`作为前置通知,`afterRun()`作为后置通知。 ```java package com.wowo.spring_aop_demo1; public class CarLogger { public void beforeRun() { System.out.println("car is going to run"); } public void afterRun() { System.out.println("car has run successfully"); } } ``` 在Spring配置文件中,我们需要定义`Car`和`CarLogger`这两个bean,并声明切面。切面通过`@Aspect`注解标识,通知则通过`@Before`、`@After`等注解指定。 ```xml <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:aop="http://www.springframework.org/schema/aop" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop.xsd"> <bean id="car" class="com.wowo.spring_aop_demo1.Car"/> <bean id="carLogger" class="com.wowo.spring_aop_demo1.CarLogger"/> <!-- 声明切面 --> <aop:config> <aop:aspect ref="carLogger"> <!-- 前置通知 --> <aop:before method="beforeRun" pointcut="execution(* com.wowo.spring_aop_demo1.Car.go(..))"/> <!-- 后置通知 --> <aop:after method="afterRun" pointcut="execution(* com.wowo.spring_aop_demo1.Car.go(..))"/> </aop:aspect> </aop:config> </beans> ``` 在这个配置中,`pointcut`定义了匹配方法执行的切入点表达式,`execution(* com.wowo.spring_aop_demo1.Car.go(..))`表示匹配`Car`类中的`go()`方法。 现在,当我们在Spring IoC容器中通过`car` bean调用`go()`方法时,会先执行`CarLogger`的`beforeRun()`方法,再执行`go()`方法,最后执行`afterRun()`方法。这就是Spring AOP的基本用法,通过这种方式,我们可以将日志记录这样的横切关注点从`Car`类中分离出来,降低了代码的耦合度。 总结来说,Spring AOP提供了一种优雅的方式来处理系统中的横切关注点,通过定义切面和通知,我们可以实现代码的解耦和模块化。在实际开发中,AOP广泛应用于日志记录、事务管理、性能统计、安全控制等多个场景,极大地提高了代码的可读性和可维护性。通过本文的入门Demo,希望你能对Spring AOP有更深入的理解,并能在实践中灵活运用。
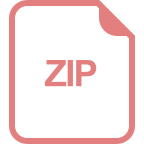
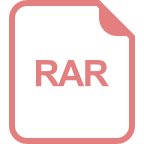
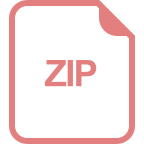
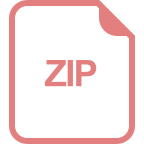
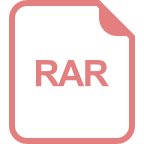
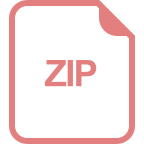
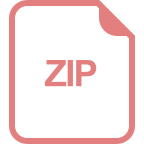
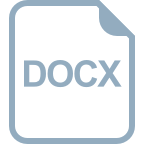
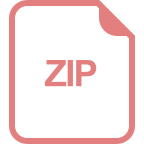
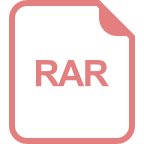
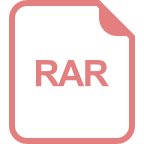
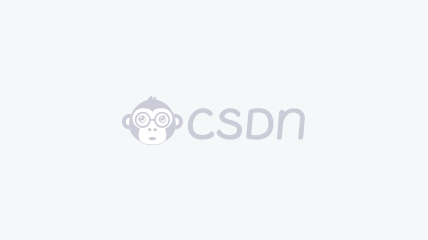

- 粉丝: 8
- 资源: 907
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

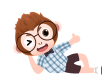
最新资源
- qaxbrowser-1.1.32574.52.exe (奇安信浏览器windows安装包)
- C#编写modbus tcp客户端读取modbus tcp服务器数据
- 某房地产瑞六补环境部分代码
- 基于Matlab实现无刷直流电机仿真(模型+说明文档).rar
- AllSort(直接插入排序,希尔排序,选择排序,堆排序,冒泡排序,快速排序,归并排序)
- 模拟qsort,改造冒泡排序使其能排序任意数据类型,即日常练习
- carsim+simulink联合仿真实现变道 包含路径规划算法+mpc轨迹跟踪算法 可选simulink版本和c++版本算法 可以适用于弯道道路,弯道车道保持,弯道变道 carsim内规划轨迹可视化
- 数组经典习题之顺序排序和二分查找和冒泡排序
- 永磁同步电机神经网络自抗扰控制,附带编程涉及到的公式文档,方便理解,模型顺利运行,效果好,位置电流双闭环采用二阶自抗扰控制,永磁同步电机三闭环控制,神经网络控制,自抗扰中状态扩张观测器与神经网络结合
- 基于 Oops Framework 提供的游戏项目开发模板,项目中提供了最新版本 Cocos Creator 3.x 插件与游戏资源初始化通用逻辑

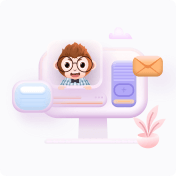
