### 利用ctypes提高Python执行速度 #### 一、ctypes简介与应用场景 `ctypes` 是Python标准库中的一个重要模块,它提供了一系列与C兼容的数据类型,并支持调用外部C语言编写的动态链接库(DLL)或共享库(SO)。通过这种方式,可以将已有的C代码无缝集成到Python程序中,或者编写C语言代码来提升Python程序的性能。 #### 二、ctypes基本用法 在使用`ctypes`之前,首先需要了解几个关键概念: - **数据类型映射**:`ctypes`提供了多种数据类型来映射C语言的数据类型,例如`c_int`对应C中的`int`,`c_char_p`对应C中的`char*`等。 - **函数调用**:可以通过`ctypes.CDLL`加载动态链接库,并调用其中的函数。如果需要传入参数或返回值,还需要指定相应的数据类型。 #### 三、ctypes提高Python执行效率实例 接下来通过两个具体案例,展示如何使用`ctypes`来提高Python的执行速度。 ##### 案例1:素数检测 1. **Python实现**:首先使用纯Python实现素数检测功能。 ```python import math from timeit import timeit def check_prime(x): values = range(2, int(math.sqrt(x)) + 1) for i in values: if x % i == 0: return False return True def get_prime(n): return [x for x in range(2, n) if check_prime(x)] print(timeit(stmt='get_prime(1000000)', setup='from __main__ import get_prime', number=10)) ``` 2. **C语言实现**:编写C语言版本的素数检测函数,并编译为共享库。 ```c #include <stdio.h> #include <math.h> int check_prime(int a) { int c; for (c = 2; c <= sqrt(a); c++) { if (a % c == 0) return 0; } return 1; } ``` 使用命令 `gcc -shared -o prime.so -fPIC prime.c` 编译生成`.so`文件。 3. **整合C语言与Python**:在Python中加载并使用该共享库。 ```python import ctypes import math from timeit import timeit check_prime_in_c = ctypes.CDLL('./prime.so').check_prime def get_prime_in_c(n): return [x for x in range(2, n) if check_prime_in_c(x)] c_time = timeit(stmt='get_prime_in_c(1000000)', setup='from __main__ import get_prime_in_c', number=10) print("C version: {} seconds".format(c_time)) ``` 4. **性能对比**:比较两种方法的时间消耗。 ```plaintext Python version: 43.4539749622 seconds C version: 8.56250786781 seconds ``` 可以明显看出C语言版本的速度远快于纯Python版本。 ##### 案例2:快速排序 1. **C语言实现**:编写快速排序算法,并定义相关结构体用于处理数组范围。 ```c #include <stdio.h> typedef struct _Range { int start, end; } Range; Range new_Range(int s, int e) { Range r; r.start = s; r.end = e; return r; } void swap(int *x, int *y) { int t = *x; *x = *y; *y = t; } void quick_sort(int arr[], const int len) { // 快速排序算法实现... } ``` 2. **Python整合**:加载C语言编写的动态链接库,并调用`quick_sort`函数。 ```python import ctypes mylib = ctypes.CDLL('./mylib.so') # 定义必要的函数参数类型 mylib.quick_sort.argtypes = [ctypes.POINTER(ctypes.c_int), ctypes.c_int] arr = [5, 3, 8, 4, 2] arr_c = (ctypes.c_int * len(arr))(*arr) mylib.quick_sort(arr_c, len(arr)) print(list(arr_c)) # 输出排序后的结果 ``` 通过以上案例可以看出,利用`ctypes`模块将C语言代码集成到Python中,可以在保持Python程序灵活性的同时,显著提升关键部分的执行效率。这对于处理大量数据或执行密集型任务时尤为有用。
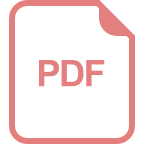
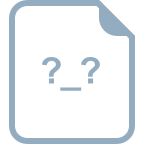
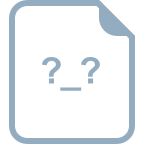
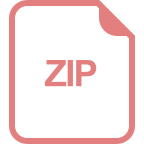
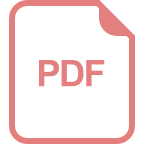
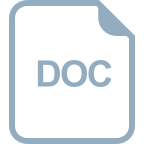
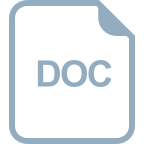
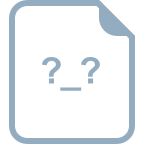
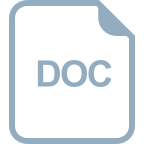
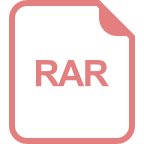
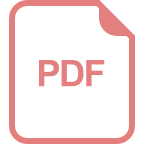
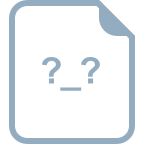
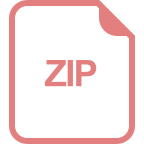
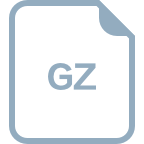
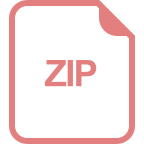
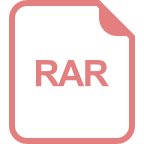
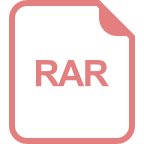
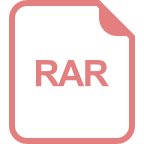
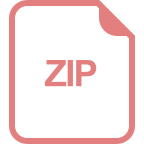
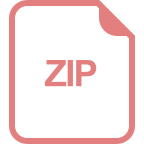
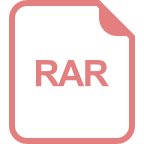
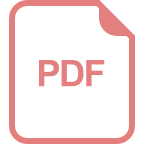
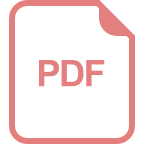
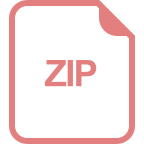
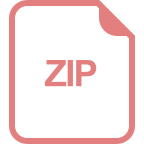
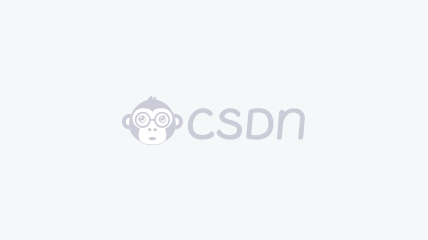

- 粉丝: 1
- 资源: 949
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

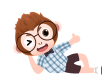
最新资源

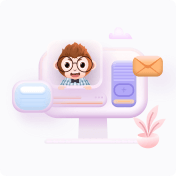
