iOS开发UICollectionView实现拖拽效果
iOS开发UICollectionView实现拖拽效果 iOS开发中,UICollectionView是非常常用的控件之一,用于实现列表、网格等视图。今天,我们将讨论如何在UICollectionView中实现拖拽效果。 一、iOS9之前实现拖拽效果 在iOS9之前,实现拖拽效果需要我们自己写方法来实现。这其中最重要的步骤是添加长按手势,捕捉用户的长按事件,并在手势方法中实现拖拽效果。 我们需要添加长按手势到UICollectionView中: ```objective-c UILongPressGestureRecognizer *longPress = [[UILongPressGestureRecognizer alloc] initWithTarget:self action:@selector(handlelongGesture:)]; [collectionView addGestureRecognizer:longPress]; ``` 然后,在handlelongGesture:方法中,我们需要获取当前选中的cell的IndexPath,并将其截图保存起来,用于后续的视图交换: ```objective-c - (void)handlelongGesture:(UILongPressGestureRecognizer *)gesture { NSIndexPath *indexPath = [self.collectionView indexPathForItemAtPoint:[gesture locationInView:self.collectionView]]; // 获取当前选中的cell的截图 UIView *snapshotView = [self createSnapshotView:indexPath]; // 保存截图 self.snapshotView = snapshotView; // 保存当前选中的IndexPath self.oldIndexPath = indexPath; } ``` 接着,我们需要在UICollectionView的代理方法中实现拖拽效果。在collectionView:moveItemAtIndexPath:toIndexPath:方法中,我们需要交换数据源,并更新UICollectionView的显示: ```objective-c - (void)collectionView:(UICollectionView *)collectionView moveItemAtIndexPath:(NSIndexPath *)sourceIndexPath toIndexPath:(NSIndexPath *)destinationIndexPath { // 交换数据源 [self.dataArr exchangeObjectAtIndex:sourceIndexPath.item withObjectAtIndex:destinationIndexPath.item]; // 更新UICollectionView的显示 [collectionView moveItemAtIndexPath:sourceIndexPath toIndexPath:destinationIndexPath]; } ``` 二、iOS9之后实现拖拽效果 在iOS9之后,苹果提供了自带的API来实现拖拽效果,我们可以使用UICollectionView的built-in方法来实现拖拽效果。 我们需要添加长按手势到UICollectionView中: ```objective-c UILongPressGestureRecognizer *longPress = [[UILongPressGestureRecognizer alloc] initWithTarget:self action:@selector(handlelongGesture:)]; [collectionView addGestureRecognizer:longPress]; ``` 然后,在handlelongGesture:方法中,我们可以直接调用iOS9的API来实现拖拽效果: ```objective-c - (void)handlelongGesture:(UILongPressGestureRecognizer *)gesture { [collectionView beginInteractiveMovementForItemAtIndexPath:[self.collectionView indexPathForItemAtPoint:[gesture locationInView:self.collectionView]]]; } ``` 三、完整实现代码 下面是完整的实现代码: ```objective-c #import <UIKit/UIKit.h> @interface ViewController () <UICollectionViewDelegate, UICollectionViewDataSource, UICollectionViewDelegateFlowLayout> @property (nonatomic, strong) NSMutableArray *dataArr; @property (nonatomic, strong) UICollectionView *collectionView; @property (nonatomic, strong) NSIndexPath *oldIndexPath; @property (nonatomic, strong) UIView *snapshotView; @property (nonatomic, strong) NSIndexPath *moveIndexPath; @end @implementation ViewController - (void)viewDidLoad { [super viewDidLoad]; // 初始化UICollectionView UICollectionViewFlowLayout *flowLayout = [[UICollectionViewFlowLayout alloc] init]; flowLayout.itemSize = CGSizeMake((SCREEN_WIDTH-40.0)/3, (SCREEN_WIDTH-40.0)/3); UICollectionView *collectionView = [[UICollectionView alloc] initWithFrame:CGRectMake(0, 50.0, SCREEN_WIDTH, (SCREEN_WIDTH-40.0)/3+20.0) collectionViewLayout:flowLayout]; collectionView.dataSource = self; collectionView.delegate = self; collectionView.backgroundColor = [UIColor whiteColor]; [collectionView registerClass:[UICollectionViewCell class] forCellWithReuseIdentifier:@"uicollectionviewcell"]; [self.view addSubview:self.collectionView = collectionView]; // 添加长按手势 UILongPressGestureRecognizer *longPress = [[UILongPressGestureRecognizer alloc] initWithTarget:self action:@selector(handlelongGesture:)]; [collectionView addGestureRecognizer:longPress]; // 初始化数据源 self.dataArr = [[NSMutableArray alloc] init]; for (NSInteger index = 0; index < 50; index ++) { CGFloat hue = (arc4random()%256/256.0); //0.0 到 1.0 CGFloat saturation = (arc4random()%128/256.0)+0.5; //0.5 到 1.0 CGFloat brightness = (arc4random()%128/256.0)+0.5; //0.5 到 1.0 UIColor *color = [UIColor colorWithHue:hue saturation:saturation brightness:brightness alpha:0.8]; [self.dataArr addObject:color]; } } - (void)handlelongGesture:(UILongPressGestureRecognizer *)gesture { NSIndexPath *indexPath = [self.collectionView indexPathForItemAtPoint:[gesture locationInView:self.collectionView]]; UIView *snapshotView = [self createSnapshotView:indexPath]; self.snapshotView = snapshotView; self.oldIndexPath = indexPath; } - (UIView *)createSnapshotView:(NSIndexPath *)indexPath { UICollectionViewCell *cell = [self.collectionView cellForItemAtIndexPath:indexPath]; UIView *snapshotView = [cell snapshotViewAfterScreenUpdates:YES]; return snapshotView; } - (void)collectionView:(UICollectionView *)collectionView moveItemAtIndexPath:(NSIndexPath *)sourceIndexPath toIndexPath:(NSIndexPath *)destinationIndexPath { [self.dataArr exchangeObjectAtIndex:sourceIndexPath.item withObjectAtIndex:destinationIndexPath.item]; [collectionView moveItemAtIndexPath:sourceIndexPath toIndexPath:destinationIndexPath]; } @end ``` 实现UICollectionView的拖拽效果需要添加长按手势,捕捉用户的长按事件,并在手势方法中实现拖拽效果。在iOS9之前,我们需要自己写方法来实现拖拽效果,而在iOS9之后,我们可以使用苹果提供的API来实现拖拽效果。
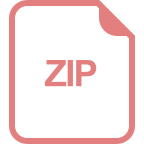
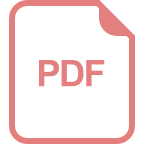
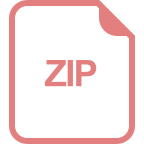
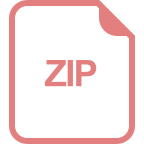
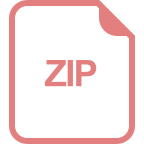
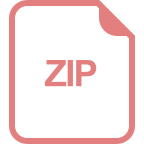
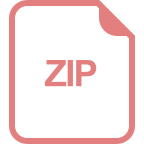
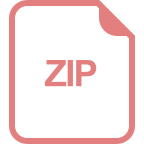
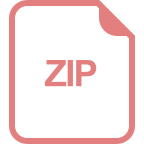
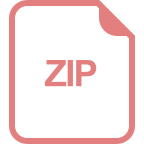
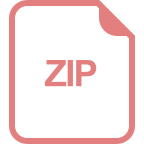
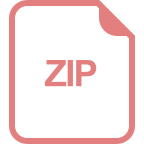
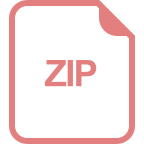
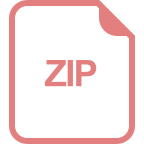
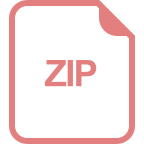
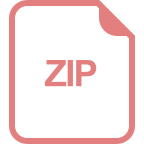
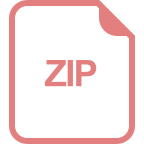
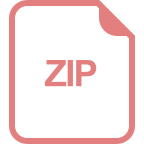
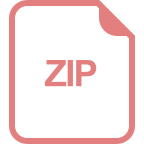
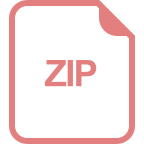
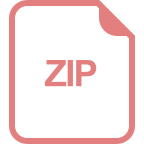
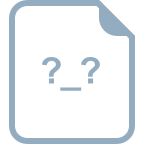
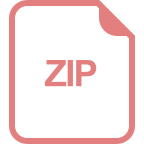
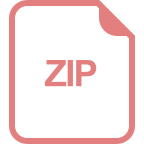
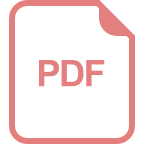
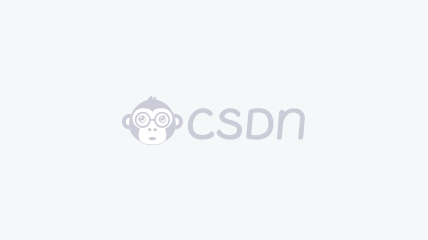

- 粉丝: 2
- 资源: 880
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

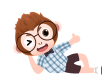
最新资源

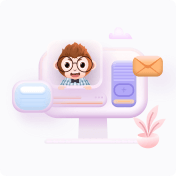
