在Android平台上,实现悬浮窗功能是一项常见的需求,尤其在视频通话和系统辅助工具等场景中。悬浮窗可以在用户使用其他应用时依然保持可见,提供便捷的交互方式。本篇文章将详细讲解如何在Android中实现这样的功能,以Kotlin语言为例进行说明。 要创建一个悬浮窗,你需要在AndroidManifest.xml文件中声明`SYSTEM_ALERT_WINDOW`权限: ```xml <uses-permission android:name="android.permission.SYSTEM_ALERT_WINDOW" /> ``` 这个权限允许应用在其他应用之上绘制窗口,是实现悬浮窗必不可少的。自Android 6.0(API Level 23)起,用户需手动授予这一特殊权限,因此你需要在运行时检查并请求该权限。以下是如何判断并请求悬浮窗权限的代码: ```kotlin if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.M) { if (!Settings.canDrawOverlays(this)) { // 显示提示,引导用户到权限设置页面 Toast.makeText(this, "当前无权限,请授权", Toast.LENGTH_SHORT).show() val intent = Intent(Settings.ACTION_MANAGE_OVERLAY_PERMISSION, Uri.parse("package:${packageName}")) startActivityForResult(intent, 0) } else { // 已有权限,执行悬浮窗展示逻辑 } } ``` 接下来,创建一个悬浮窗服务类,如`FloatWindowServices`,继承自`Service`。在这个服务中,你需要创建一个悬浮窗视图,并将其添加到屏幕顶部: ```kotlin class FloatWindowServices : Service() { private lateinit var windowManager: WindowManager private lateinit var windowParams: WindowManager.LayoutParams override fun onCreate() { super.onCreate() windowManager = getSystemService(WINDOW_SERVICE) as WindowManager initLayoutParams() createFloatView() } private fun initLayoutParams() { windowParams = WindowManager.LayoutParams( ViewGroup.LayoutParams.WRAP_CONTENT, ViewGroup.LayoutParams.WRAP_CONTENT, if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) { WindowManager.LayoutParams.TYPE_APPLICATION_OVERLAY } else { WindowManager.LayoutParams.TYPE_PHONE }, WindowManager.LayoutParams.FLAG_NOT_FOCUSABLE or WindowManager.LayoutParams.FLAG_LAYOUT_IN_SCREEN or WindowManager.LayoutParams.FLAG_LAYOUT_NO_LIMITS, PixelFormat.TRANSLUCENT ) // 设置悬浮窗位置,例如在屏幕右上角 windowParams.x = 0 windowParams.y = 0 } private fun createFloatView() { val floatView = LayoutInflater.from(this).inflate(R.layout.float_view, null) windowManager.addView(floatView, windowParams) // 添加触摸事件监听,以便处理悬浮窗的拖动和点击 floatView.setOnTouchListener { v, event -> when (event.action) { MotionEvent.ACTION_DOWN -> { val (dx, dy) = getDelta(v, event) windowParams.x += dx windowParams.y += dy windowManager.updateViewLayout(v, windowParams) true } MotionEvent.ACTION_UP -> { // 处理点击事件 } else -> false } } } private fun getDelta(view: View, event: MotionEvent): Pair<Int, Int> { val xDiff = event.x.toInt() - (view.right - view.left) / 2 val yDiff = event.y.toInt() - (view.bottom - view.top) / 2 return Pair(xDiff, yDiff) } // 其他Service生命周期方法... } ``` 为了使悬浮窗与特定的Activity(例如视频通话页面)相关联,你可以使用`bindService()`方法绑定Service。这样,当Activity进入后台时,悬浮窗显示;当Activity返回前台时,悬浮窗隐藏。在Activity中添加以下代码: ```kotlin private var binder: FloatWindowBinder? = null override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) bindService(Intent(this, FloatWindowServices::class.java), serviceConnection, Context.BIND_AUTO_CREATE) } override fun onDestroy() { unbindService(serviceConnection) super.onDestroy() } val serviceConnection = object : ServiceConnection { override fun onServiceConnected(name: ComponentName?, binder: IBinder?) { this@YourActivity.binder = binder as FloatWindowBinder // 当服务连接成功时,可以通过binder控制悬浮窗 } override fun onServiceDisconnected(name: ComponentName?) { binder = null } } ``` `FloatWindowBinder`是Service的Binder对象,用于在Activity和Service之间通信,控制悬浮窗的显示和隐藏。在Service中定义这个类: ```kotlin inner class FloatWindowBinder : Binder() { fun showFloatWindow() { // 在此处展示悬浮窗 } fun hideFloatWindow() { // 在此处隐藏悬浮窗 } } ``` 为了让悬浮窗在用户切换应用或按下Home键时仍然显示,你需要在Service中创建一个后台线程,持续检测Activity的状态。当通话页面Activity不可见时,显示悬浮窗;当Activity重新回到前台时,隐藏悬浮窗。 以上步骤详细介绍了如何在Android中实现一个简单的悬浮窗功能。实际开发中,你可能还需要处理更多细节,例如悬浮窗的拖动、点击事件、动画效果以及不同设备的兼容性问题等。确保在设计和实现过程中充分考虑用户体验和系统的稳定性。

- 粉丝: 7
- 资源: 915
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

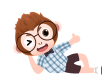
最新资源

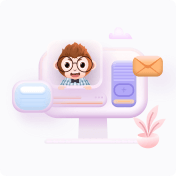
