在Android编程中,图片处理是常见且重要的任务。本文将探讨一些关键的代码示例,涉及Bitmap、String、Drawable之间的转换以及如何从资源ID获取Drawable。以下是对这些操作的详细解释: 1. **Bitmap转化为字符串**: 这个过程是将Bitmap对象编码为Base64字符串,便于在网络传输或存储中使用。`compress()`方法用于将Bitmap压缩为PNG格式,并设置质量(这里是100%,意味着无损压缩)。然后,`Base64.encodeToString()`将字节数组转换为可读的字符串。 ```java public static String bitmapToString(Bitmap bitmap) { ByteArrayOutputStream bStream = new ByteArrayOutputStream(); bitmap.compress(CompressFormat.PNG, 100, bStream); byte[] bytes = bStream.toByteArray(); return Base64.encodeToString(bytes, Base64.DEFAULT); } ``` 2. **字符串转化为Bitmap**: 这是反向操作,从Base64字符串解码回Bitmap。`Base64.decode()`将字符串还原为字节数组,然后`BitmapFactory.decodeByteArray()`将字节数组解析为Bitmap。 ```java public static Bitmap stringToBitmap(String string) { try { byte[] bitmapArray = Base64.decode(string, Base64.DEFAULT); return BitmapFactory.decodeByteArray(bitmapArray, 0, bitmapArray.length); } catch (Exception e) { e.printStackTrace(); } return null; } ``` 3. **Bitmap转化为Drawable**: Bitmap和Drawable是Android中两种不同的图像表示方式。`BitmapDrawable`类允许我们将Bitmap转换为Drawable对象。为了确保正确的密度,我们可能需要设置Bitmap的密度。 ```java public static Drawable bitmapToDrawable(Bitmap bitmap) { if (bitmap != null) { if (160 != bitmap.getDensity()) { bitmap.setDensity(160); } return new BitmapDrawable(bitmap); } return null; } ``` 4. **Drawable转化为Bitmap**: 当需要从Drawable返回Bitmap时,可以使用`getBitmap()`方法,但需要注意的是,只有当Drawable是BitmapDrawable时,这个方法才有效。 ```java public static Bitmap drawableToBitmap(Drawable drawable) { return drawable instanceof BitmapDrawable ? ((BitmapDrawable) drawable).getBitmap() : null; } ``` 5. **根据资源ID获取Drawable对象**: 此方法通过资源ID加载Bitmap,然后将其转换为Drawable对象。 ```java public static Drawable resourceToDrawable(Context context, int id) { return context != null ? bitmapToDrawable(BitmapFactory.decodeResource(context.getResources(), id)) : null; } ``` 6. **byte数组转换为Drawable对象**: 同样,我们首先将字节数组解码为Bitmap,然后将其转换为Drawable。 ```java public static Drawable byteArrayToDrawable(byte[] bytes) { return bytes != null ? bitmapToDrawable(BitmapFactory.decodeByteArray(bytes, 0, bytes.length)) : null; } 7. **byte数组转换为Bitmap对象**: 如果需要将字节数组直接转换为Bitmap,可以直接调用`BitmapFactory.decodeByteArray()`。 ```java public static Bitmap byteArrayToBitmap(byte[] bytes) { return bytes != null ? BitmapFactory.decodeByteArray(bytes, 0, bytes.length) : null; } ``` 这些代码片段展示了在Android开发中处理图片的基本操作,它们对于显示、保存、传输或操作图片非常有用。理解并熟练运用这些转换方法能够帮助开发者更有效地管理和使用图片资源。
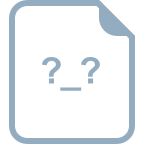
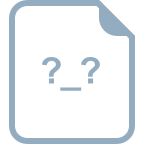
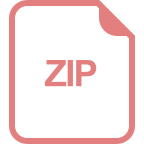
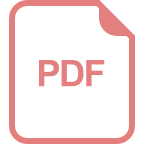
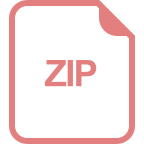
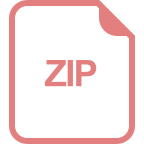
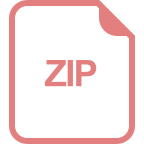
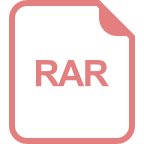
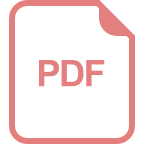
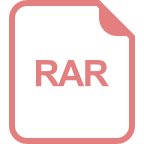
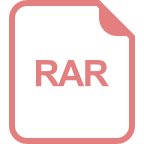
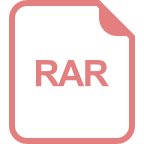
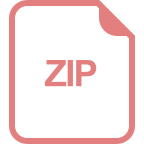
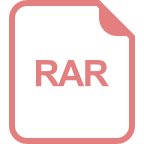
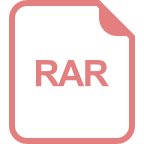
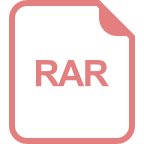
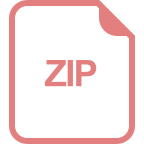
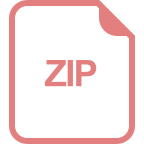
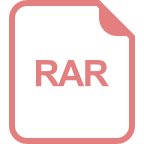
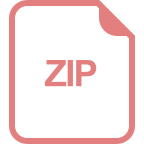
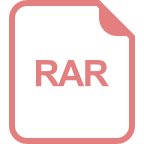
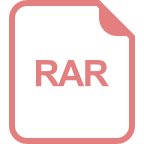
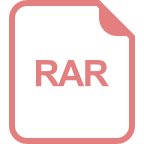
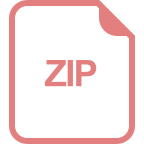
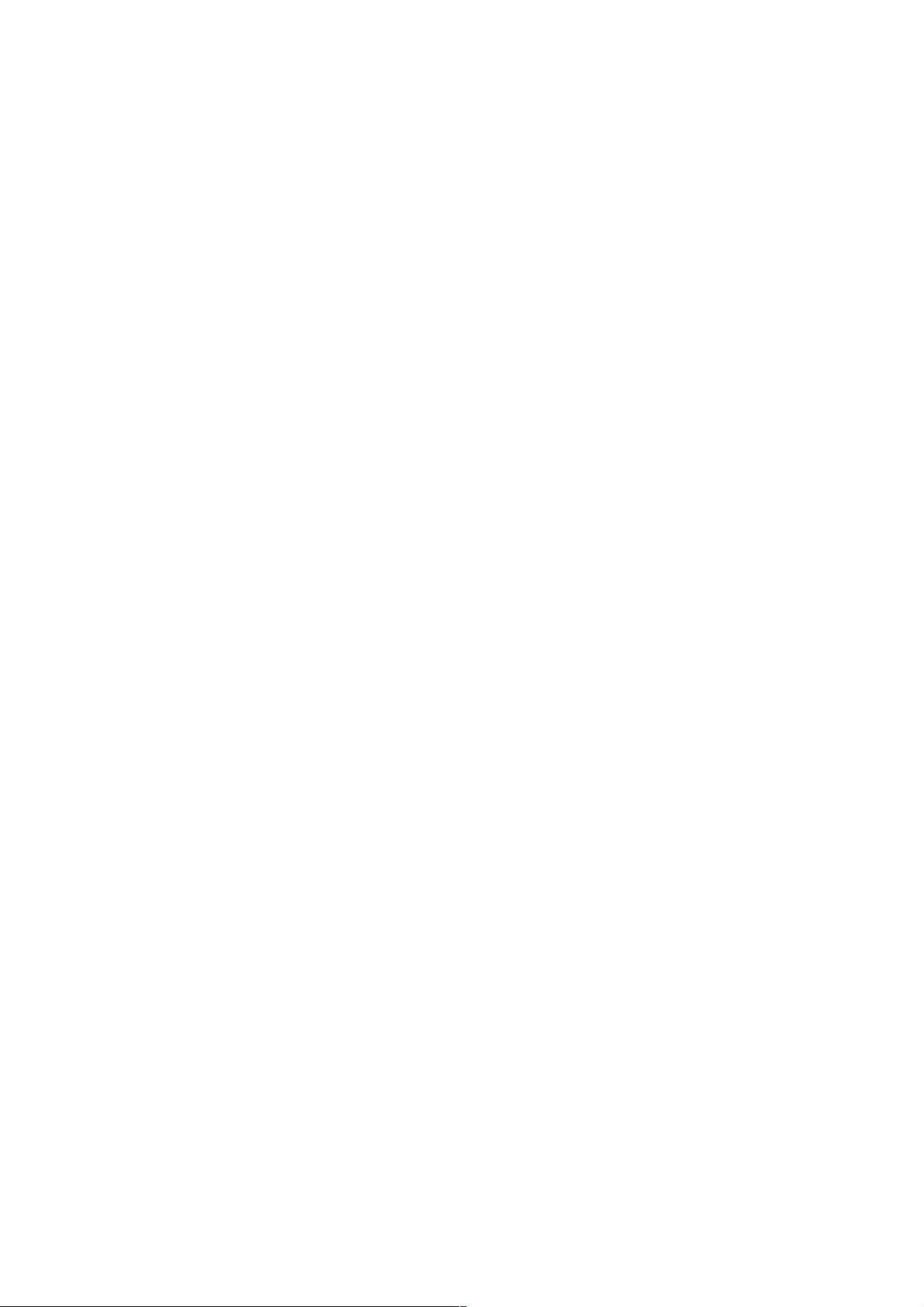
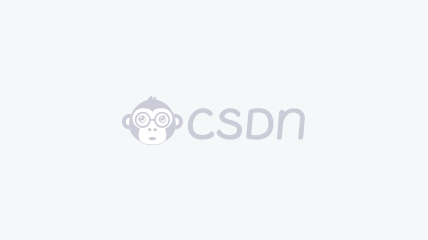

- 粉丝: 1
- 资源: 935
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

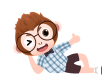
最新资源

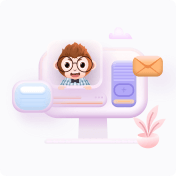
