//*****************************************************************************
// Copyright (C) 2014 Texas Instruments Incorporated
//
// All rights reserved. Property of Texas Instruments Incorporated.
// Restricted rights to use, duplicate or disclose this code are
// granted through contract.
// The program may not be used without the written permission of
// Texas Instruments Incorporated or against the terms and conditions
// stipulated in the agreement under which this program has been supplied,
// and under no circumstances can it be used with non-TI connectivity device.
//
//*****************************************************************************
//*****************************************************************************
//
// Application Name - MQTT Client
// Application Overview - This application acts as a MQTT client and connects
// to the IBM MQTT broker, simultaneously we can
// connect a web client from a web browser. Both
// clients can inter-communicate using appropriate
// topic names.
//
// Application Details -
// http://processors.wiki.ti.com/index.php/CC32xx_MQTT_Client
// or
// docs\examples\CC32xx_MQTT_Client.pdf
//
//*****************************************************************************
//*****************************************************************************
//
//! \addtogroup mqtt_client
//! @{
//
//*****************************************************************************
// Standard includes标准包括
#include <stdlib.h>
// simplelink includes
#include "simplelink.h"
// driverlib includes
#include "hw_types.h"
#include "hw_ints.h"
#include "hw_memmap.h"
#include "interrupt.h"
#include "rom_map.h"
#include "prcm.h"
#include "uart.h"
#include "timer.h"
// common interface includes常见的接口包括
#include "network_if.h"
#ifndef NOTERM
#include "uart_if.h"
#endif
#include "button_if.h"
#include "gpio_if.h"
#include "timer_if.h"
#include "common.h"
// Standard includes1
#include <string.h>
#include <stdint.h>
#include <stdbool.h>
// Driverlib includes
#include "utils.h"
#include "hw_common_reg.h"
#include "hw_adc.h"
#include "hw_ints.h"
#include "hw_gprcm.h"
#include "rom.h"
#include "pin.h"
#include "gpio.h"
#include "adc.h"
#include "temp.h"
#include "ph.h"
#include "utils.h"
#include "sl_mqtt_client.h"
// application specific includes 特定应用程序包括
#include "pinmux.h"
#define APPLICATION_VERSION "1.1.1"
/*Operate Lib in MQTT 3.1 mode. 在MQTT 3.1模式下操作Lib.*/
#define MQTT_3_1_1 false /*MQTT 3.1.1 */
#define MQTT_3_1 true /*MQTT 3.1*/
#define WILL_TOPIC "Client"
#define WILL_MSG "Client Stopped"
#define WILL_QOS QOS2
#define WILL_RETAIN false
/*Defining Broker IP address and port Number. 定义代理IP地址和端口号*/
#define SERVER_ADDRESS "47.93.253.168"
#define PORT_NUMBER 1883
#define MAX_BROKER_CONN 1
#define SERVER_MODE MQTT_3_1
/*Specifying Receive time out for the Receive task. 指定接收任务的接收时间*/
#define RCV_TIMEOUT 30
/*Background receive task priority 背景接收任务优先级*/
#define TASK_PRIORITY 3
/* Keep Alive Timer value 维持计时器值*/
#define KEEP_ALIVE_TIMER 25
/*Clean session flag 清除会话标志*/
#define CLEAN_SESSION true
/*Retain Flag. Used in publish message 保留标志.用于发布消息. */
#define RETAIN 1
/*Defining Publish Topic 定义发布主题*/
#define PUB_TOPIC "/Data/send"
/*Defining Number of topics 定义主题数量*/
#define TOPIC_COUNT 1
/*Defining Subscription Topic Values 定义订阅主题值*/
#define TOPIC1 "/Data/receive"
/*定义控制数据*/
#define DateHeatingOn "\"heating\":\"1\""
#define DateHeatingOff "\"heating\":\"0\""
/*Defining QOS levels 定义QOS级别*/
#define QOS0 0
#define QOS1 1
#define QOS2 2
/*Spawn task priority and OSI Stack Size 生成任务优先级和OSI堆栈大小*/
#define OSI_STACK_SIZE 2048
#define UART_PRINT Report
typedef struct connection_config{
SlMqttClientCtxCfg_t broker_config;
void *clt_ctx;
unsigned char *client_id;
unsigned char *usr_name;
unsigned char *usr_pwd;
bool is_clean;
unsigned int keep_alive_time;
SlMqttClientCbs_t CallBAcks;
int num_topics;
char *topic[TOPIC_COUNT];
unsigned char qos[TOPIC_COUNT];
SlMqttWill_t will_params;
bool is_connected;
}connect_config;
typedef enum
{
PUSH_BUTTON_SW2_PRESSED,
PUSH_BUTTON_SW3_PRESSED,
BROKER_DISCONNECTION
}events;
typedef struct
{
void * hndl;
events event;
}event_msg;
//*****************************************************************************
// LOCAL FUNCTION PROTOTYPES 本地函数原型
//*****************************************************************************
static void
Mqtt_Recv(void *app_hndl, const char *topstr, long top_len, const void *payload,
long pay_len, bool dup,unsigned char qos, bool retain);
static void sl_MqttEvt(void *app_hndl,long evt, const void *buf,
unsigned long len);
static void sl_MqttDisconnect(void *app_hndl);
void pushButtonInterruptHandler2();
void pushButtonInterruptHandler3();
void TimerPeriodicIntHandler(void);
void LedTimerConfigNStart();
void LedTimerDeinitStop();
void BoardInit(void);
static void DisplayBanner(char * AppName);
void MqttClient(void *pvParameters);
//*****************************************************************************
// GLOBAL VARIABLES -- Start 全局变量——开始
//*****************************************************************************
#ifdef USE_FREERTOS
#if defined(ewarm)
extern uVectorEntry __vector_table;
#endif
#if defined(ccs)
extern void (* const g_pfnVectors[])(void);
#endif
#endif
unsigned short g_usTimerInts;
/* AP Security Parameters AP安全参数*/
SlSecParams_t SecurityParams = {0};
/*Message Queue 消息队列*/
OsiMsgQ_t g_PBQueue;
/* connection configuration 连接配置 */
connect_config usr_connect_config[] =
{
{
{
{
SL_MQTT_NETCONN_URL,
SERVER_ADDRESS,
PORT_NUMBER,
0,
0,
0,
NULL
},
SERVER_MODE,
true,
},
NULL,
"DataUser1",
//NULL,
//NULL,
"aoonsqmp",
"8cDKdRxcTDXu",
true,
KEEP_ALIVE_TIMER,
{Mqtt_Recv, sl_MqttEvt, sl_MqttDisconnect},
TOPIC_COUNT,
{TOPIC1},
{QOS2},
{WILL_TOPIC,WILL_MSG,WILL_QOS,WILL_RETAIN},
false
}
};
/* library configuration 库配置*/
SlMqttClientLibCfg_t Mqtt_Client={
1882,
TASK_PRIORITY,
30,
true,
(long(*)(const char *, ...))UART_PRINT
};
/*Publishing topics and messages 发布主题和消息*/
const char *pub_topic_sw3 = PUB_TOPIC;
char *data_sw3={"{\"temp\":\"00.00\"}"};
//char *data_sw3={"{\"temp\":\"00.00\",\"pH\":\"00.00\",\"LiquidLevel\":\"0\"}"};
void *app_hndl = (void*)usr_connect_config;
//*****************************************************************************
// GLOBAL VARIABLES -- End 全局变量——结束
//*****************************************************************************
//****************************************************************************
//! Defines Mqtt_Pub_Message_Receive event handler. 定义Mqtt_Pub_Message_Receive事件处理程序
//! Client App needs to register this event handler with sl_ExtLib_mqtt_Init 客户端应用程序需要用slextlibmqttinit注册这个事件处理程序
//! API. Background receive task invokes this handler whenever MQTT Client API.后台接收任务在MQTT客户端调用此处理程序
//! receives a Publish Message from the broker. 从代理处接收发布消息。
//!
//!\param[out] topstr => pointer to topic of the messag
没有合适的资源?快使用搜索试试~ 我知道了~
环境参数采集,MQTT协议实现数据传输.zip
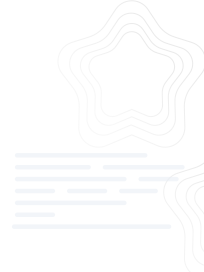
共57个文件
pp:11个
obj:11个
launch:5个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 86 浏览量
2024-01-02
14:52:35
上传
评论
收藏 915KB ZIP 举报
温馨提示
嵌入式相关的项目源码、数据集等资源
资源推荐
资源详情
资源评论
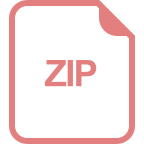
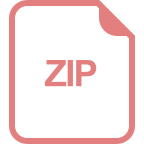
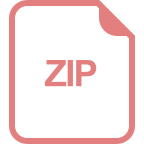
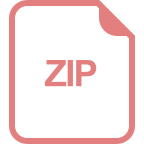
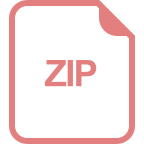
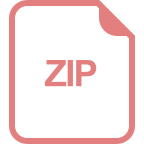
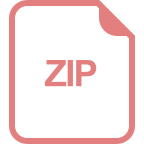
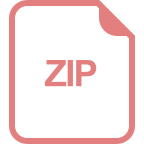
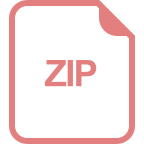
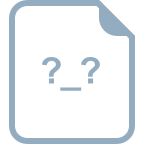
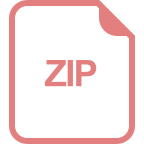
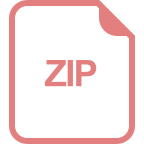
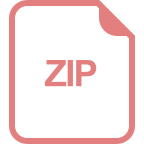
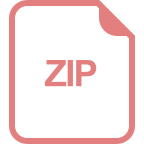
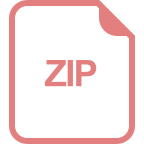
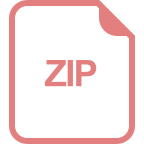
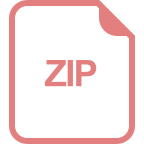
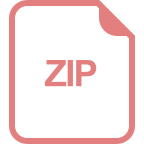
收起资源包目录


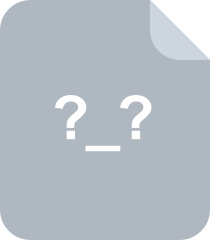

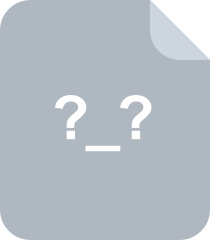
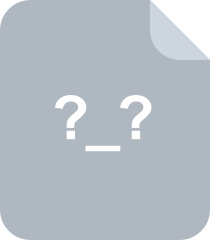
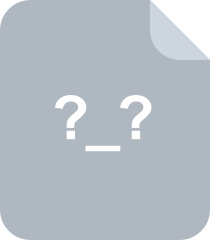
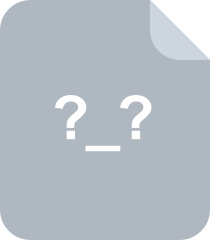
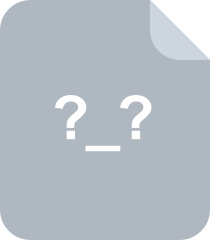
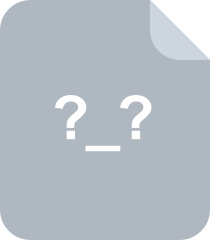
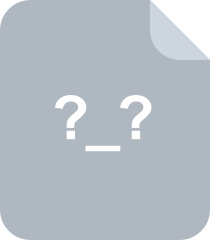
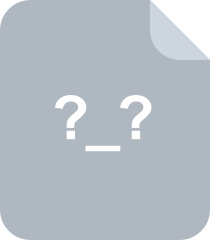
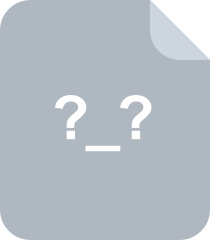
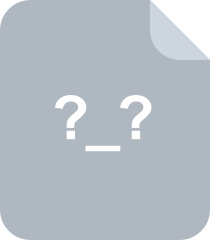
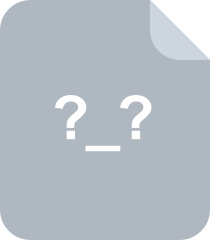
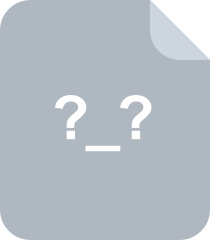
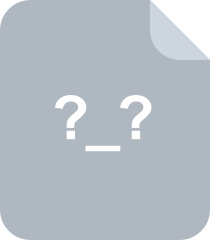
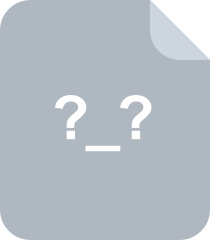
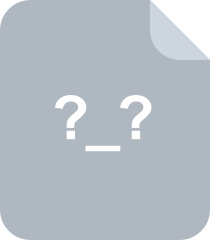
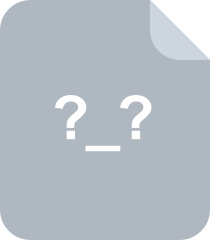
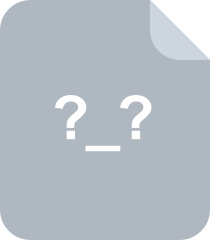
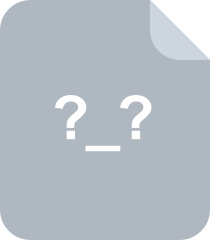
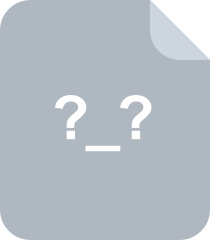
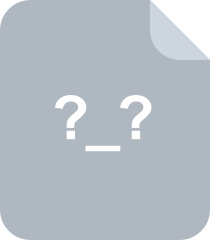
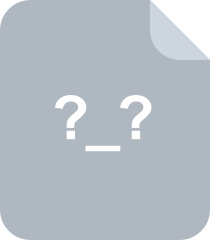
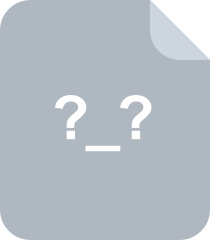
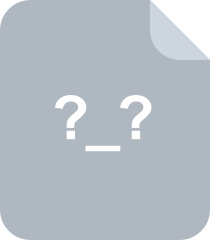
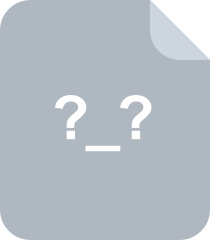
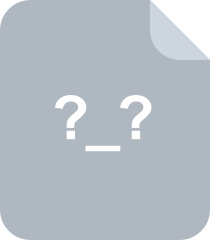
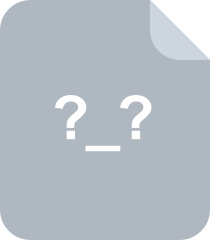
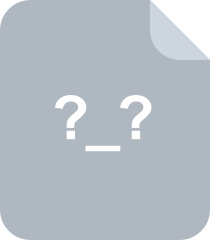
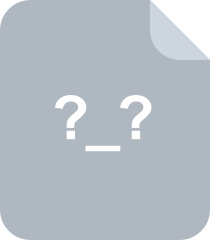
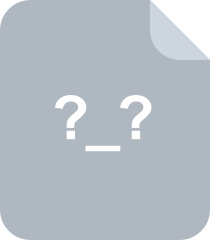
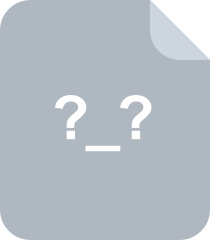
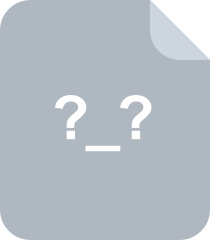
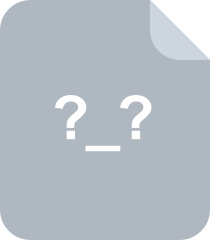
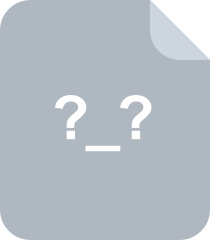
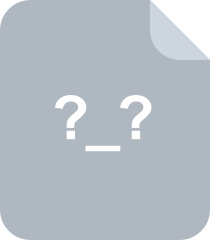
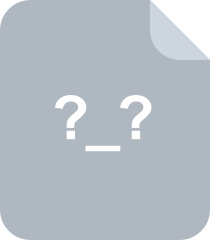
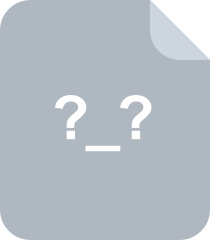

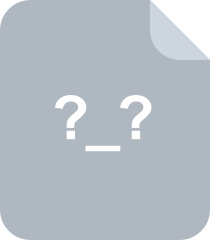
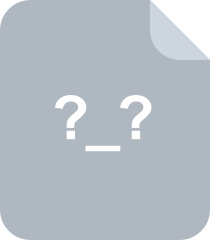
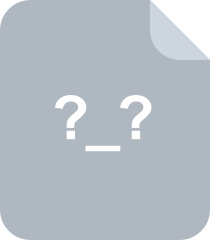
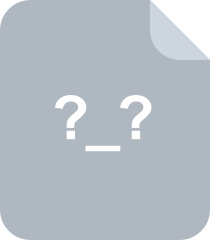
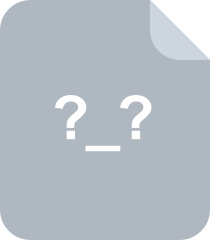

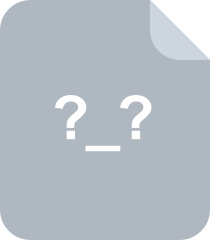
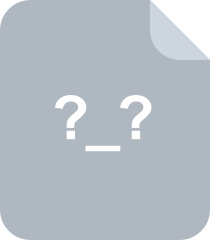
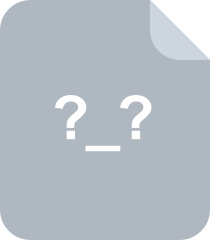
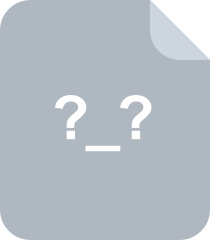

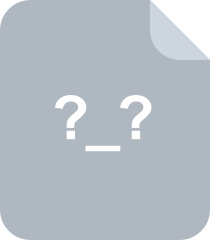
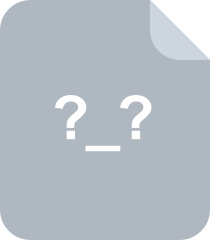
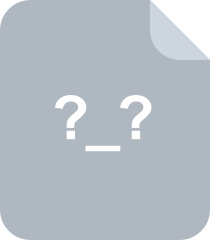
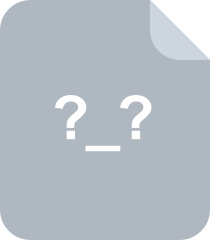
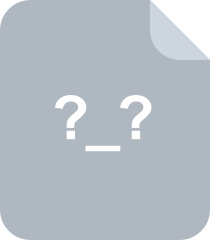
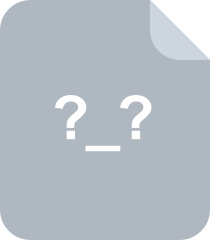
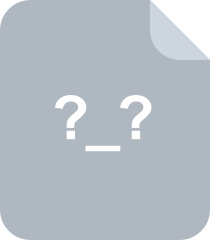
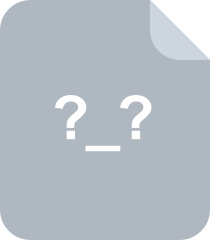
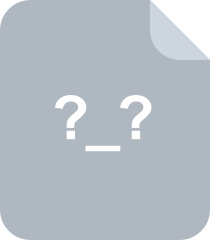
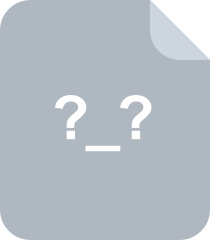
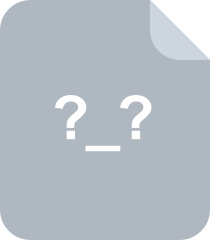
共 57 条
- 1
资源评论
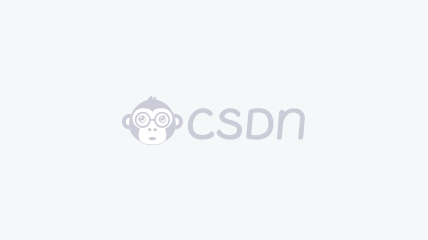

马coder
- 粉丝: 1200
- 资源: 6602
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

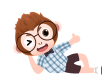
最新资源
- foldcraftlauncher_262944.apk
- 珍藏多年的基于matlab实现潮流计算程序源代码集合,包含多个潮流计算程序.rar
- 使用FPGA实现串-并型乘法器
- 基于matlab实现针对基于双曲线定位的DV-Hop算法中误差误差出一种基于加权双曲线定位的DV-Hop改进算法.rar
- 基于matlab实现由遗传算法开发的整数规划,车辆调度问题.rar
- 电视家7.0(对电视配置要求高).apk
- 免费计算机毕业设计-基于JavaEE的医院病历管理系统设计与实现(包含论文+源码)
- 手机端 我的世界融合植物大战僵尸版.apk
- 植物大战僵尸 · 戴夫的老年生活 手机版.apk
- Runcraft · 我的世界跑酷游戏 手机端.apk
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


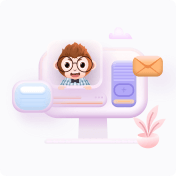
安全验证
文档复制为VIP权益,开通VIP直接复制
