#include "editwidget.h"
#include <QFile>
#include <QMessageBox>
#include <QTextStream>
#include <QVBoxLayout>
#include <QColorDialog>
#include <QDebug>
#include <QTextCursor>
#include <QTextBlockFormat>
#include <QTextCharFormat>
#include <QTextObject>
#include <QRegularExpression>
#include <QLineEdit>
#include <QPushButton>
#include <QVBoxLayout>
editWidget::editWidget(QWidget *parent) : QWidget(parent)
{
ref_num = 0;
refTable.append(ref_num);
//创建工具栏
toolBar = new QToolBar(this);
toolBar->setIconSize(QSize(25, 25));
//创建编辑窗口
textedit = new QTextEdit(this);
//保存按钮
saveAction = new QAction(QIcon(":/ico/ico/save.ico"), "保存", this);
saveAction->setShortcut(QKeySequence::Save);
toolBar->addAction(saveAction);
connect(saveAction, SIGNAL(triggered()), this, SLOT(slotSave()));
//字体
fontBox = new QFontComboBox();
fontBox->setFontFilters( QFontComboBox::ScalableFonts );
toolBar->addWidget( fontBox );
//字号
sizeBox = new QComboBox();
toolBar->addWidget( sizeBox );
QFontDatabase db;
foreach( int nSize, db.standardSizes() )
sizeBox->addItem( QString::number( nSize ) );
toolBar->addSeparator();
//加粗、斜体、下划线、颜色
boldBtn = new QToolButton();
boldBtn->setIcon( QIcon( ":/ico/ico/format_text_bold.ico" ) );
boldBtn->setCheckable( true );
toolBar->addWidget( boldBtn );
//斜体
italicBtn = new QToolButton();
italicBtn->setIcon( QIcon( ":/ico/ico/format_text_italic.ico" ) );
italicBtn->setCheckable( true );
toolBar->addWidget( italicBtn );
//下划线
underlineBtn = new QToolButton();
underlineBtn->setIcon( QIcon( ":/ico/ico/format_text_underline.ico" ) );
underlineBtn->setCheckable( true );
toolBar->addWidget( underlineBtn );
toolBar->addSeparator();
//查找按钮
findAction = new QAction(QIcon(":/ico/ico/lookup.png"), "查找", this);
findAction->setShortcut(QKeySequence::Find);
toolBar->addAction(findAction);
connect(findAction, SIGNAL(triggered()), this, SLOT(showFindText()));
findDlg = new QDialog(this);
findDlg->setWindowTitle("查找");
findLineEdit = new QLineEdit(findDlg);
QPushButton *btn = new QPushButton("查找下一个", findDlg);
QVBoxLayout * layout_finddlg = new QVBoxLayout(findDlg);
layout_finddlg->addWidget(findLineEdit);
layout_finddlg->addWidget(btn);
connect(btn, &QPushButton::clicked, this, &editWidget::slotFind);
//颜色
colorBtn = new QToolButton();
colorBtn->setIcon( QIcon( ":/ico/ico/format_color.ico" ) );
toolBar->addWidget( colorBtn );
//连接信号与槽函数
connect( fontBox, SIGNAL( activated( QString ) ), this, SLOT( slotFont( QString ) ) );
connect( sizeBox, SIGNAL( activated( QString ) ), this, SLOT( slotSize( QString ) ) );
connect( boldBtn, SIGNAL( clicked() ), this, SLOT( slotBold() ) );
connect( italicBtn, SIGNAL( clicked() ), this, SLOT( slotItalic() ) );
connect( underlineBtn, SIGNAL( clicked() ), this, SLOT( slotUnder() ) );
connect( colorBtn, SIGNAL( clicked() ), this, SLOT( slotColor() ) );
connect( textedit, SIGNAL( currentCharFormatChanged( const QTextCharFormat & ) ), this, SLOT( slotNowFormatChanged( const QTextCharFormat& ) ) );
//上一个引用
prevRefAction = new QAction(QIcon(":/ico/ico/prevref.png"), "上一个引用", this);
toolBar->addAction(prevRefAction);
connect(prevRefAction, SIGNAL(triggered()), this, SLOT(slotPrevRef()));
//下一个引用
nextRefAction = new QAction(QIcon(":/ico/ico/nextref.png"), "上一个引用", this);
toolBar->addAction(nextRefAction);
connect(nextRefAction, SIGNAL(triggered()), this, SLOT(slotNextRef()));
//跳转到参考文献
skipRefAction = new QAction(QIcon(":/ico/ico/skipRef.png"), "跳转到文献", this);
toolBar->addAction(skipRefAction);
connect(skipRefAction, SIGNAL(triggered()), this, SLOT(slotSkipToRef()));
//删除参考文献
deleteRefAction = new QAction(QIcon(":/ico/ico/deletered.ico"), "删除文献", this);
toolBar->addAction(deleteRefAction);
connect(deleteRefAction, SIGNAL(triggered()), this, SLOT(deleteRef()));
//设置布局 工具栏在上 编辑器在下
QVBoxLayout * layout = new QVBoxLayout();
layout->addWidget(toolBar);
layout->addWidget(textedit);
this->setLayout(layout);
}
editWidget::~editWidget()
{
}
//点击保存按钮后的动作
void editWidget::slotSave()
{
QFile file(notepath);
if (!file.open(QIODevice::WriteOnly))
{
return;
}
QTextStream out(&file);
out << textedit->toHtml();
textedit->document()->setModified(false);
file.close();
//保存文献信息表
saveRefTable();
}
void editWidget::slotLoadText(QString str)
{
if (!firstload)
slotSave();
notepath = str;
QFile openFile(str);
if (!openFile.open(QIODevice::ReadOnly))
return;
QTextStream in(&openFile);
textedit->setText(in.readAll());
openFile.close();
loadRefTable();
firstload = false;
}
void editWidget::slotFind()
{
QString str = findLineEdit->text();
if (!textedit->find(str, QTextDocument::FindBackward))
{
QMessageBox::warning(this, "查找", "找不到 "+ str);
}
}
void editWidget::showFindText()
{
findDlg->show();
}
//设置字体
void editWidget::slotFont(QString f)
{
QTextCharFormat fmt;
fmt.setFontFamily(f);
mergeFormat(fmt);
}
//设置字号
void editWidget::slotSize(QString num)
{
QTextCharFormat fmt;
fmt.setFontPointSize(num.toFloat());
mergeFormat(fmt);
}
//加粗
void editWidget::slotBold()
{
QTextCharFormat fmt;
fmt.setFontWeight(boldBtn->isChecked() ? QFont::Bold : QFont::Normal);
mergeFormat(fmt);
}
//斜体
void editWidget::slotItalic()
{
QTextCharFormat fmt;
fmt.setFontItalic(italicBtn->isChecked());
mergeFormat(fmt);
}
//下划线
void editWidget::slotUnder()
{
QTextCharFormat fmt;
fmt.setFontUnderline(underlineBtn->isChecked());
mergeFormat(fmt);
}
//设置颜色
void editWidget::slotColor()
{
QColor color = QColorDialog::getColor(Qt::red, this);
if (color.isValid())
{
QTextCharFormat fmt;
fmt.setForeground(color);
mergeFormat(fmt);
}
}
//当光标所在处的字符格式发生变化时调用,函数根据新的字符格式把工具栏上的各个格式控件的显示更新
void editWidget::slotNowFormatChanged(const QTextCharFormat & fmt)
{
fontBox->setCurrentIndex(fontBox->findText(fmt.fontFamily()));
sizeBox->setCurrentIndex(sizeBox->findText(QString::number(fmt.fontPointSize())));
boldBtn->setChecked(fmt.font().bold());
italicBtn->setChecked(fmt.fontItalic());
underlineBtn->setChecked(fmt.fontUnderline());
}
//设置光标的选区,使格式作用于选区内的字符,若没有选区则作用于光标所在处的字符
void editWidget::mergeFormat(QTextCharFormat fmt)
{
QTextCursor cursor = textedit->textCursor();
if (!cursor.hasSelection())
cursor.select(QTextCursor::WordUnderCursor);
cursor.mergeCharFormat(fmt);
textedit->mergeCurrentCharFormat(fmt);
}
/* 插入参考文献 str是引用时的文字格式 ref_id是引用文献对应的唯一编号
该函数在文本中查找光标处上下文引用,生成正确的编号 并更新后面的编号
该函数还要修改参考文献后面的引用
*/
void editWidget::slotInsertReference(QString str, int ref_id)
{
//本笔记引用数加1
++ref_num;
int thisnum;
//qDebug() << str;
//获取编辑区文档和光标 以供后面修改
QTextDocument * doc = textedit->document();
QTextCursor cursor(textedit->textCursor());
//设置格式为上标
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
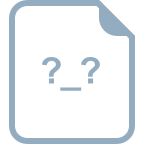
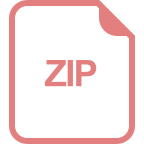
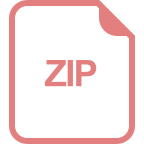
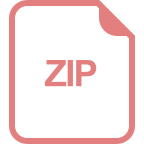
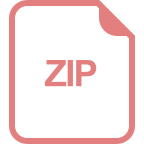
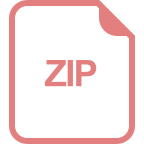
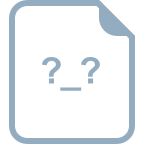
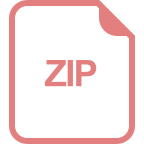
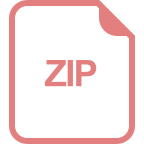
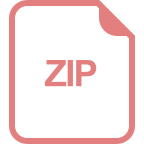
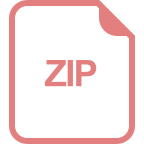
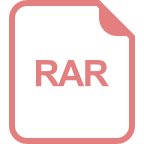
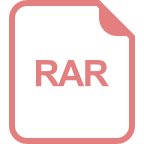
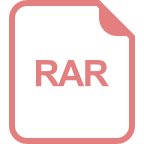
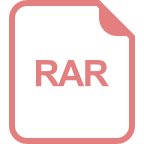
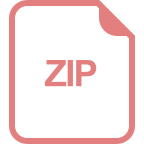
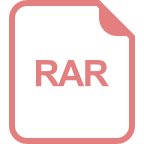
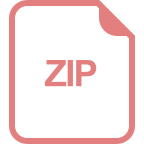
收起资源包目录


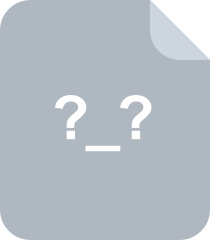
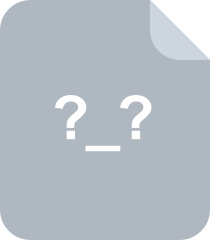
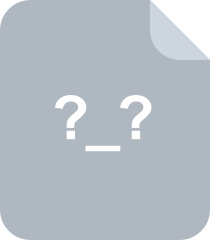
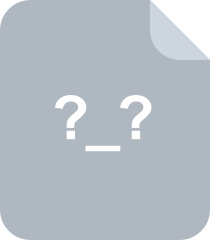
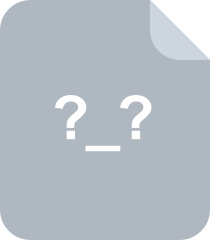
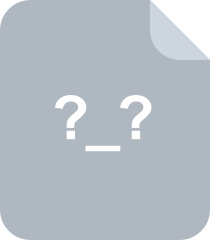
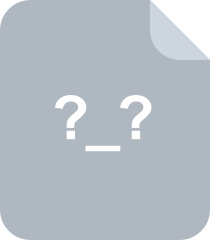
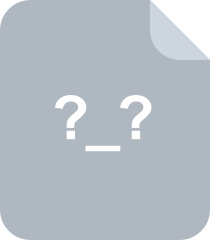
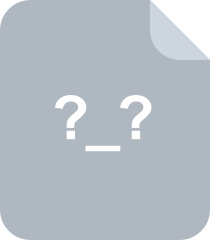
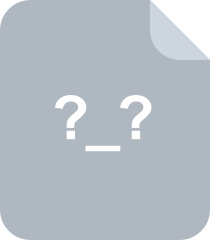
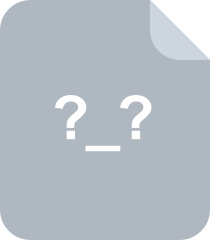
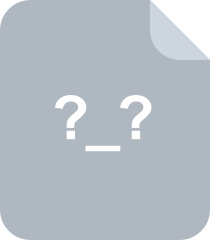
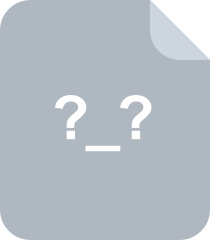
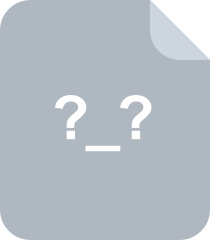
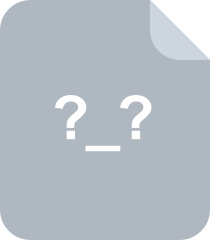
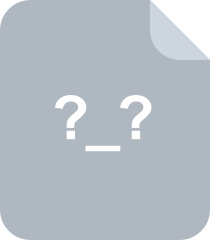
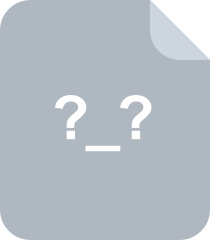
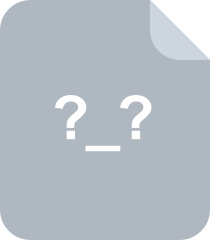
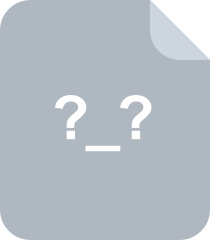

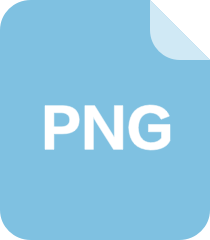
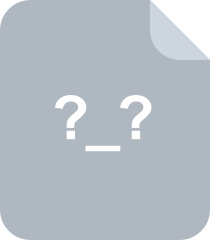
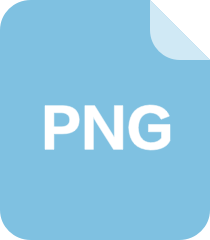
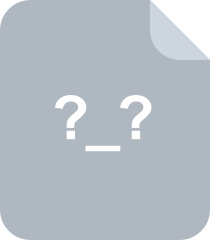
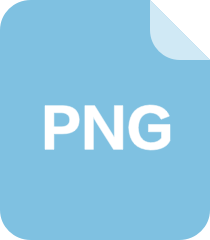
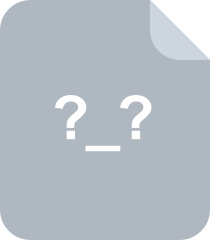
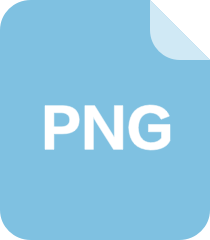
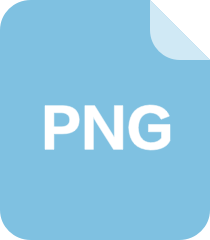
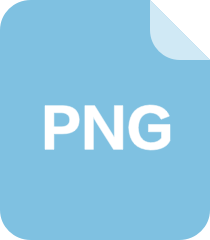
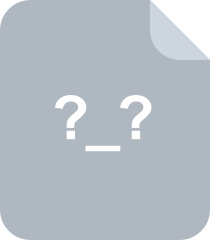
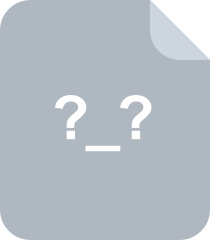
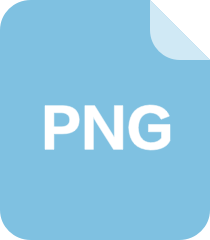
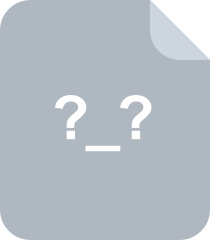
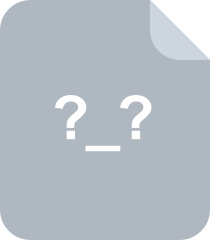
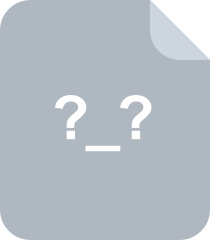
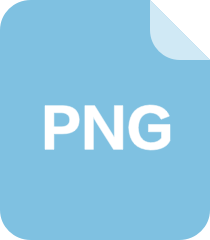
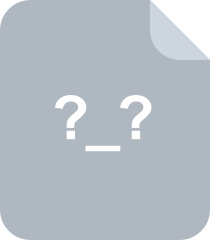
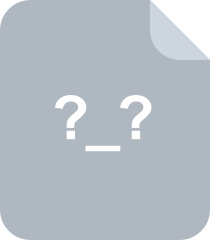
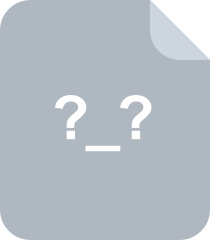
共 38 条
- 1
资源评论
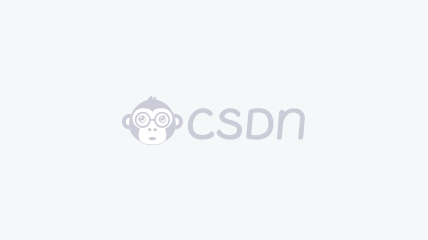

马coder
- 粉丝: 1206
- 资源: 6602
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

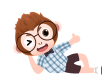
最新资源
- densenet模型-python语言pytorch框架的图像分类宠物行为分类识别-不含数据集图片-含逐行注释和说明文档.zip
- alexnet模型-基于深度学习识别水果种类-不含数据集图片-含逐行注释和说明文档.zip
- earsertrweeqewrr
- alexnet模型-基于深度学习对草莓品级果识别-不含数据集图片-含逐行注释和说明文档.zip
- 微信小程序五子棋游戏,有悔棋,清屏等功能
- alexnet模型-python语言pytorch框架训练识别餐桌美食-不含数据集图片-含逐行注释和说明文档.zip
- proteus下载安装 具体步骤
- proteus下载安装 具体步骤
- proteus下载安装 具体步骤
- proteus下载安装 具体步骤
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


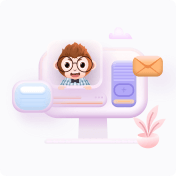
安全验证
文档复制为VIP权益,开通VIP直接复制
