### Python基础总结 #### 一、基础知识概览 在Python编程语言的学习过程中,掌握基础知识是非常重要的一步。本文档旨在提供一份全面的Python基础知识总结,帮助初学者更好地理解Python的核心概念和编程技巧。 #### 二、用户输入与字典操作 **用户输入**:在Python中,`input()`函数用于获取用户的键盘输入。例如: ```python name = input("请输入您的名字: ") ``` **字典操作**:字典是一种存储键值对的数据结构。向字典添加元素时,使用以下语法: ```python my_dict = {} my_dict['key'] = 'value' ``` 遍历字典时,可以使用`items()`方法来同时获取键和值: ```python for key, value in my_dict.items(): print(key, value) ``` #### 三、条件判断与循环 **条件判断**:在Python中,使用`if`语句来进行条件判断。注意`==`用于比较,而`=`用于赋值: ```python x = 5 if x == 5: print("x is 5") ``` **循环**:使用`for`循环遍历集合(如列表或字典): ```python for item in [1, 2, 3]: print(item) ``` #### 四、函数 **函数参数**:函数可以定义默认参数值。如果在调用函数时不传递该参数,将使用默认值。例如: ```python def greet(name='World'): print(f"Hello, {name}!") greet() # 输出: Hello, World! greet('Alice') # 输出: Hello, Alice! ``` **函数返回值**:`return`语句用于从函数返回值。如果没有显式返回值,默认返回`None`: ```python def add(a, b): return a + b result = add(3, 4) print(result) # 输出: 7 ``` **传递列表给函数**:可以通过将列表作为参数传递给函数来修改列表内容: ```python def modify_list(lst): lst.append('new item') my_list = ['item1', 'item2'] modify_list(my_list) print(my_list) # 输出: ['item1', 'item2', 'new item'] ``` **传递列表副本**:为了防止函数修改原始列表,可以传递列表的副本: ```python def modify_list_copy(lst): lst.append('new item') original_list = ['item1', 'item2'] modify_list_copy(original_list[:]) # 使用切片 [:] 创建副本 print(original_list) # 输出: ['item1', 'item2'] ``` **传递任意数量的参数**:使用星号(*)前缀定义可以接受任意数量参数的函数: ```python def print_items(*args): for arg in args: print(arg) print_items('apple', 'banana', 'cherry') ``` #### 五、面向对象编程 **类与对象**:Python支持面向对象编程。类是创建对象的模板,对象则是类的实例: ```python class Car: def __init__(self, make, model, year): self.make = make self.model = model self.year = year self.odometer_reading = 0 def get_descriptive_name(self): long_name = f"{self.year} {self.make} {self.model}" return long_name.title() my_new_car = Car('audi', 'a4', 2019) print(my_new_car.get_descriptive_name()) ``` **修改属性值**:可以通过多种方式修改类的属性值: 1. **直接修改属性值**: ```python my_new_car.odometer_reading = 23 ``` 2. **通过方法修改属性值**: ```python class Car: def update_odometer(self, mileage): self.odometer_reading = mileage my_new_car.update_odometer(23) ``` 3. **通过方法递增属性值**: ```python class Car: def increment_odometer(self, miles): self.odometer_reading += miles my_new_car.increment_odometer(100) ``` **继承**:子类继承父类的属性和方法: ```python class ElectricCar(Car): def __init__(self, make, model, year): super().__init__(make, model, year) self.battery_size = 75 my_tesla = ElectricCar('tesla', 'model s', 2019) print(my_tesla.get_descriptive_name()) ``` #### 六、总结 通过上述内容的学习,我们可以看到Python作为一种高级编程语言,其语法简洁且功能强大。无论是基本的数据类型操作还是复杂的面向对象编程,Python都提供了丰富的内置函数和强大的语法支持。掌握这些基础知识对于进一步深入学习Python非常关键。希望本总结能够帮助大家建立起坚实的Python编程基础。
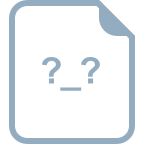
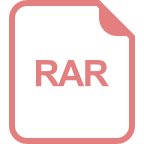
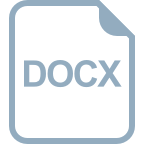
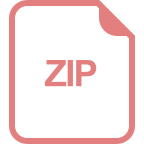
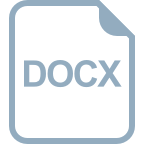
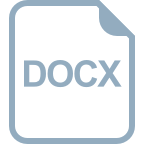
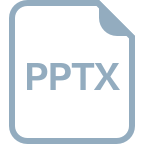
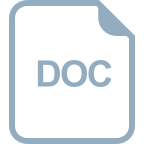
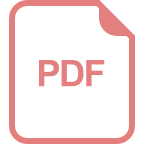
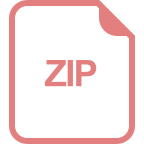
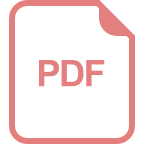
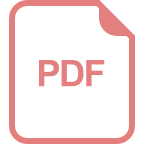
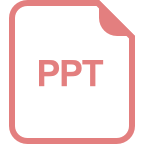
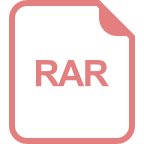
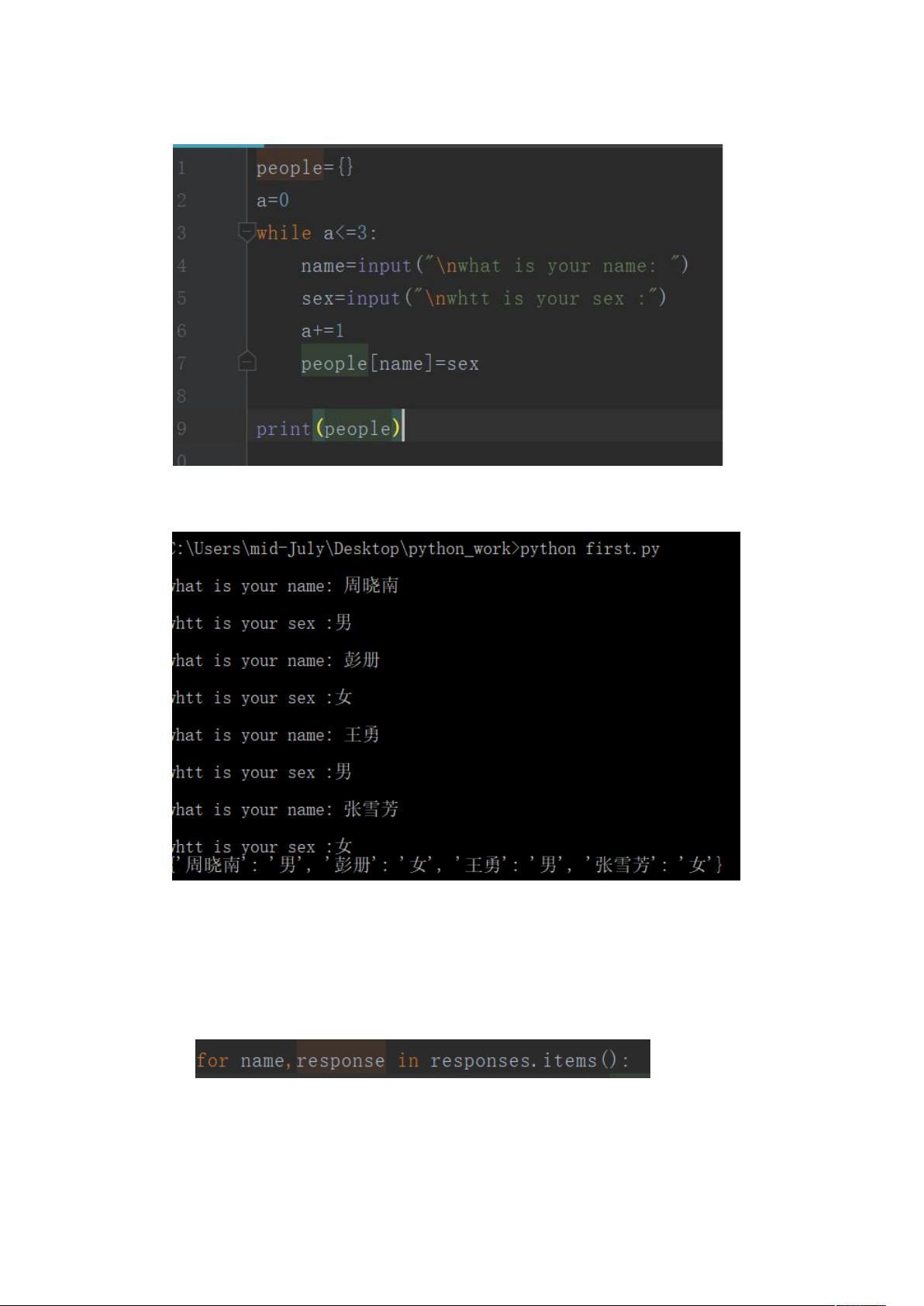
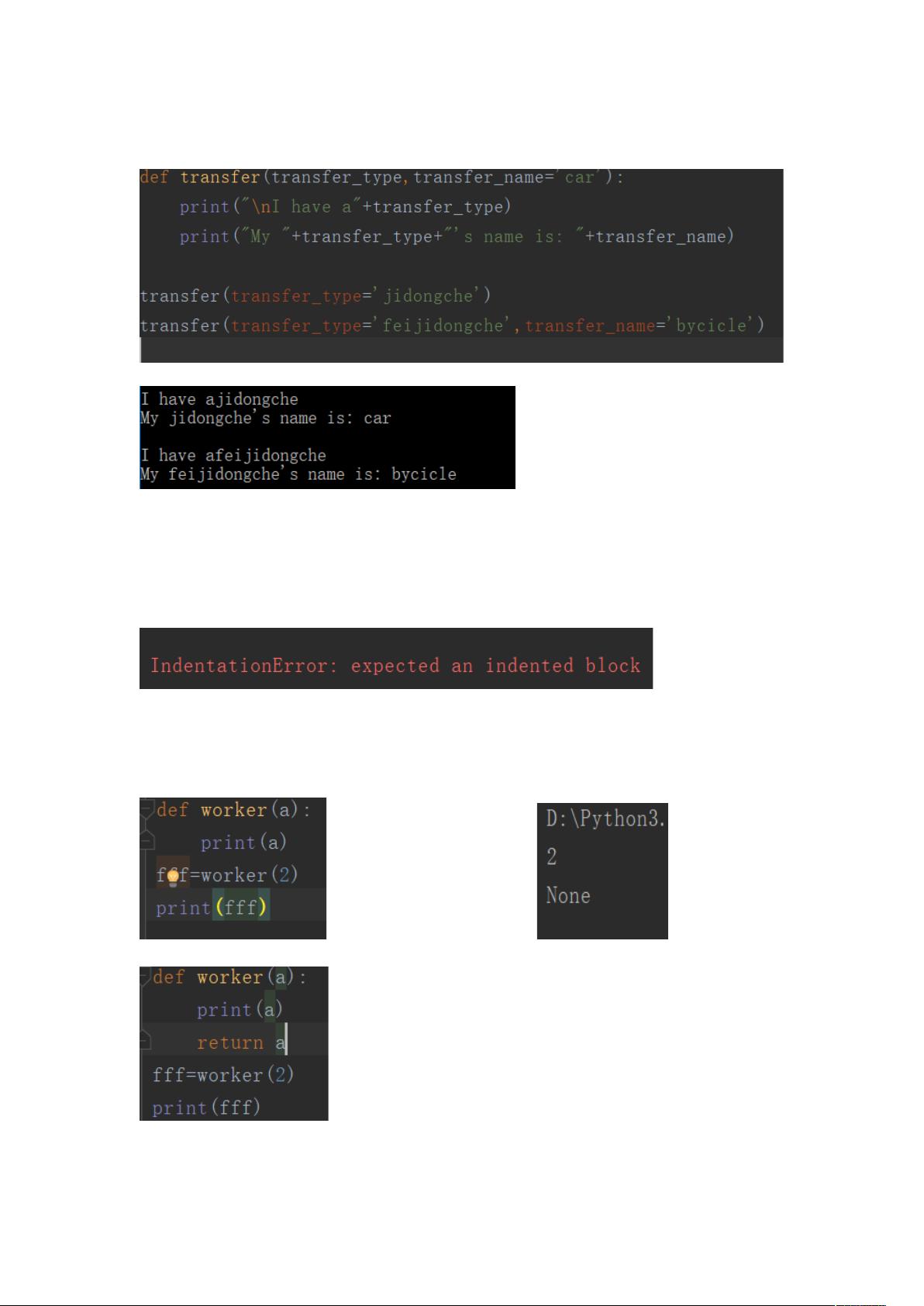
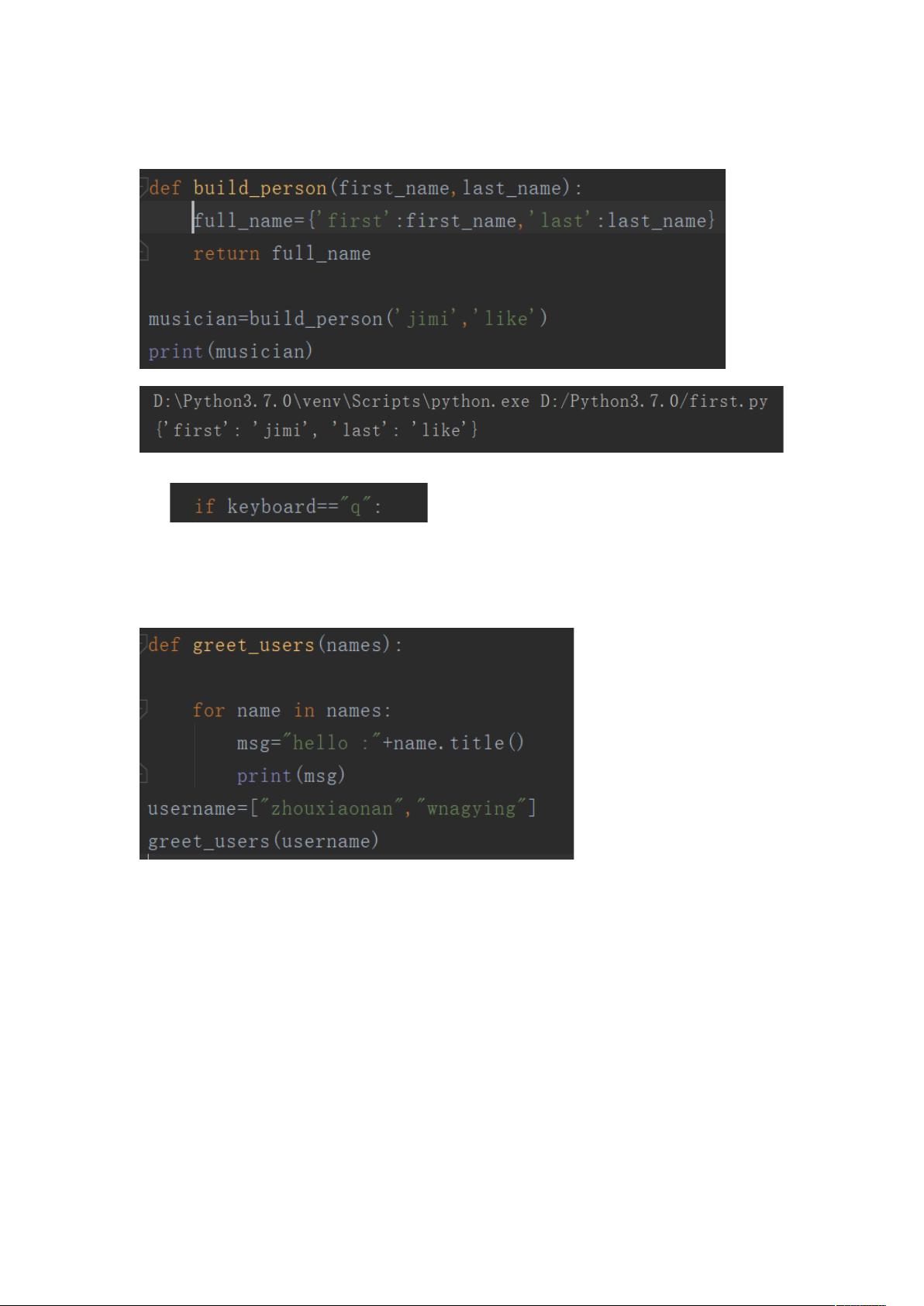
剩余17页未读,继续阅读
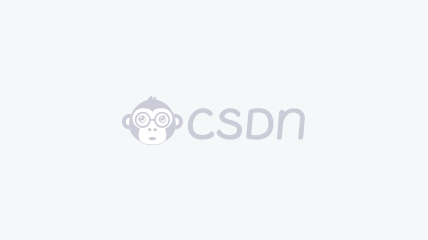

- 粉丝: 0
- 资源: 1
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

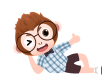
