在C语言编程中,任务三1涉及到两个主要的函数实现:`power`和`equation`。`power`函数用于执行幂运算,而`equation`函数则用于解决一元二次方程。让我们深入探讨这两个函数的设计和实现。 `power`函数接收两个参数,即底数和指数。它需要计算底数被指数次幂的结果。在C语言中,可以使用循环或内置的`pow`函数来实现这个功能。如果选择使用循环,一个简单的实现方法是: ```c int power(int base, int exponent) { int result = 1; for (int i = 0; i < exponent; i++) { result *= base; } return result; } ``` 如果允许使用数学库,可以改用`pow`函数,但记得包含`<math.h>`头文件并处理可能的错误情况: ```c #include <math.h> double power(double base, int exponent) { double result = pow(base, exponent); if (isnan(result)) { // 检查是否为非数字 printf("Error: Invalid input for power operation.\n"); exit(1); } return result; } ``` 接下来是`equation`函数,它用于求解一元二次方程`ax^2 + bx + c = 0`。根据二次公式`x = [-b ± sqrt(b^2 - 4ac)] / (2a)`,我们可以编写如下代码: ```c #include <math.h> char equation(int a, int b, int c, double result[2]) { double discriminant = b * b - 4 * a * c; if (discriminant < 0) { // 无实数解 return 0; } else if (discriminant == 0) { // 一个实数解 result[0] = -b / (2 * a); return 1; } else { // 两个实数解 result[0] = (-b + sqrt(discriminant)) / (2 * a); result[1] = (-b - sqrt(discriminant)) / (2 * a); return 1; } } ``` 在这个函数中,我们首先计算判别式`b^2 - 4ac`,然后根据判别式的值来确定方程的解的数量。如果有解,我们将解存储在传入的`result`数组中,并返回1表示有解;否则,返回0表示无解。 在`main`函数中,你可以调用这两个函数来测试它们的功能,例如: ```c int main() { double result[2]; // 测试power函数 printf("2 to the power of 3: %d\n", power(2, 3)); // 测试equation函数 char a_result = equation(5, 2, 1, result); if (a_result) { printf("The equation 5x^2 + 2x + 1 = 0 has no solution.\n"); } else { printf("The equation 5x^2 + 2x + 1 = 0 has solutions: x1 = %.2f, x2 = %.2f\n", result[0], result[1]); } a_result = equation(5, 2, -1, result); if (a_result) { printf("The equation 5x^2 + 2x - 1 = 0 has solutions: x1 = %.2f, x2 = %.2f\n", result[0], result[1]); } else { printf("The equation 5x^2 + 2x - 1 = 0 has no solution.\n"); } return 0; } ``` 这将输出`power`和`equation`函数的结果。你可以进一步扩展`main`函数,增加更多的测试用例或实现其他与数学运算相关的辅助函数,以提高程序的实用性。
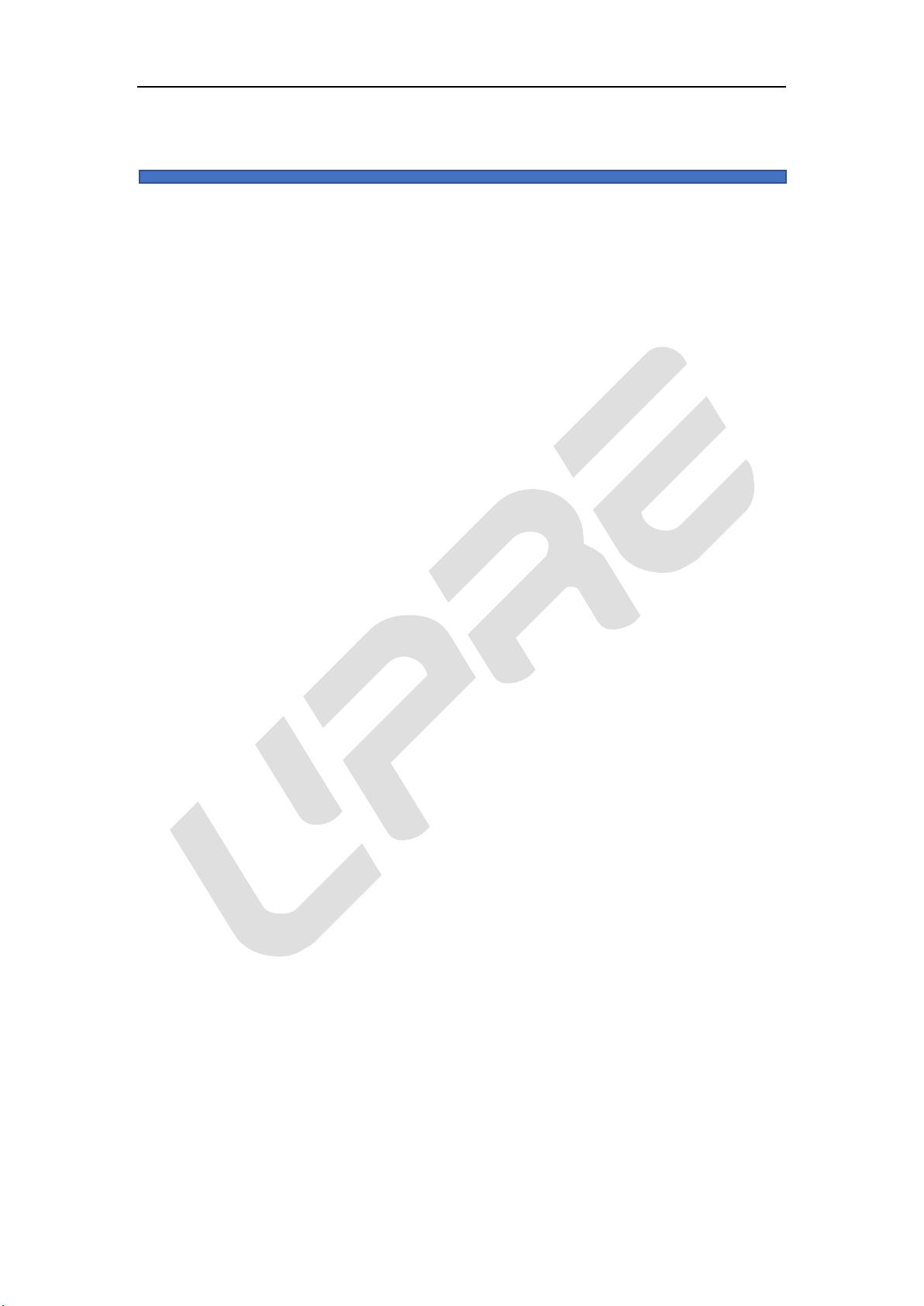
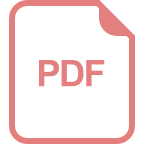
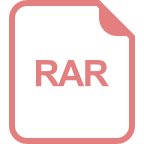
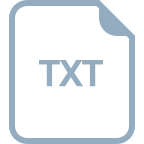
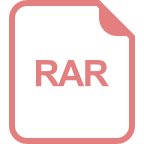
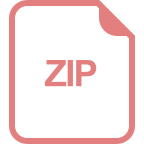
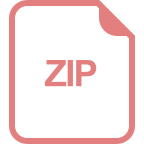
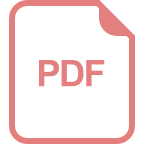
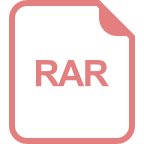
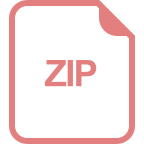
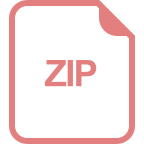
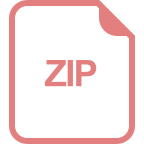
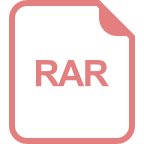
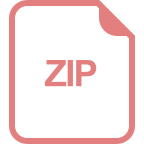
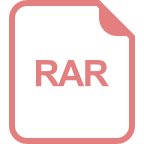
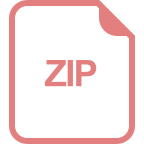
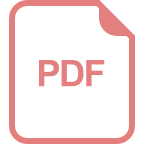
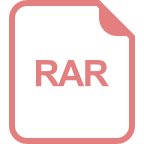
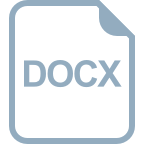
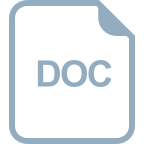
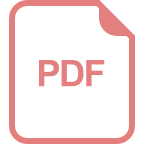
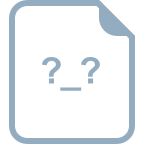
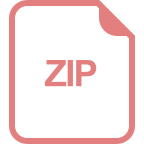
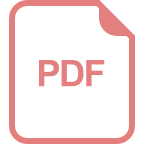
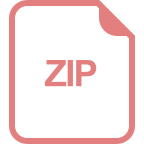
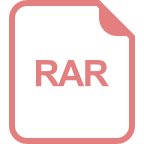
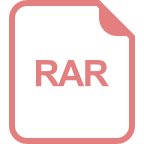
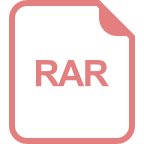

- 粉丝: 30
- 资源: 343
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

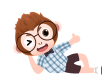

评论0