import java.awt.Color;
import java.util.ArrayList;
public class Manager {
private int pageSize=4;
private int minBlock=4;
private Color c[]=new Color[6];
private int method;
private PCB pcb[];
private Memory m=new Memory(8,32);
CompanySystem cs=new CompanySystem(minBlock,256);
PageSystem ps=new PageSystem(pageSize,256/pageSize);
SegmentSystem ss=new SegmentSystem(256);
public Manager(int method) {
super();
this.method = method;
c[0]=new Color(255,100,100);
c[1]=new Color(100,255,100);
c[2]=new Color(100,100,255);
c[3]=new Color(255,255,100);
c[4]=new Color(255,100,255);
c[5]=new Color(100,255,255);
pcb=new PCB[6];
int[] block=new int[256];
switch(method){
case 0:break;
case 1:break;
case 2:
case 3:
double i=0;
for(int j=0;j<block.length;j++){
block[j]=(int)i;
i+=1.0/pageSize;
}
}
m.setMb(block);
}
public int getFreePCB(){
for(int i=0;i<pcb.length;i++)
if(pcb[i]==null)
return i;
return -1;
}
public boolean cancel(int pid){
m.setPointer(-1);
if(pid<0||pid>5) return false;
switch(method){
case 0:
if(pcb[pid]!=null){
cs.free(pcb[pid].getStartAddress());
clearSegment(pcb[pid]);
pcb[pid]=null;
return true;
}
else
return false;
case 1:
if(pcb[pid]!=null){
clearSegment(pcb[pid]);
pcb[pid]=null;
return true;
}
else
return false;
case 2:
case 3:
if(pcb[pid]!=null){
clearPage(pcb[pid]);
pcb[pid]=null;
return true;
}
else
return false;
}
return false;
}
public boolean submit(PCB p){
m.setPointer(-1);
int ci=getFreePCB();
if(ci==-1)
return false;
else
p.setId(ci);
int a;
int totalSize=0;
ArrayList<int[]> pages;
switch(method){
case 0:
for(Integer i:p.getSegmentList())
totalSize+=i;
a=cs.allocate(totalSize);
System.out.println(totalSize);
if(a==-1)
return false;
else{
p.setStartAddress(a);
int k=a;
for(Integer i:p.getSegmentList()){
p.getSegmentTable().add(k);
k+=i;
}
writeSegment(p);
pcb[ci]=p;
return true;
}
case 1:
for(Integer i:p.getSegmentList()){
a=ss.allocate(i);
System.out.println(a);
if(a==-1){
clearSegment(p);
return false;
}
p.getSegmentTable().add(a);
}
writeSegment(p);
pcb[ci]=p;
return true;
case 2:
for(Integer i:p.getSegmentList())
totalSize+=i;
pages=ps.allocate(totalSize);
if(pages==null){
clearPage(p);
return false;
}
p.setStartAddress(pages.get(0)[0]*pageSize);
for(int[] pr:pages)
for(int k=pr[0];k<=pr[1];k++)
p.getPageTable().add(k);
writePage(p);
pcb[ci]=p;
return true;
case 3:
int pi=0;
for(Integer i:p.getSegmentList()){
pages=ps.allocate(i);
if(pages==null){
clearPage(p);
return false;
}
p.getSegmentTable().add(pi);
for(int[] pr:pages)
for(int k=pr[0];k<=pr[1];k++,pi++)
p.getPageTable().add(k);
}
writeSegmentPage(p);
pcb[ci]=p;
return true;
}
return false;
}
private void writePage(PCB p){
int totalSize=0;
for(Integer i:p.getSegmentList())
totalSize+=i;
int si=0,pi=0,sk=0;
int sil=p.getSegmentList().get(si);
for(int pk:p.getPageTable()){
for(int a=0;a<pageSize;a++,pi++){
if(pi==totalSize) return;
m.write(a+pk*pageSize,"p"+p.getId()+".s"+si+"."+sk++, c[p.getId()]);
if(sk==sil&&si<p.getSegmentList().size()-1) {sk=0;sil=p.getSegmentList().get(++si);}
}
}
}
private void clearPage(PCB p){
for(int pk:p.getPageTable()){
ps.free(pk, pk);
for(int a=0;a<pageSize;a++)
m.clear(a+pk*pageSize);
}
}
private void writeSegmentPage(PCB p){
int si=0;
for(int pti:p.getSegmentTable()){
int sk=0;
System.out.println(p.getPageTable().size());
int pageNum=p.getSegmentList().get(si)/pageSize;
if(p.getSegmentList().get(si)%pageSize!=0)
pageNum++;
System.out.println(pageNum);
for(int pi=pti;pi<pti+pageNum;pi++){
int pk=p.getPageTable().get(pi)*pageSize;
System.out.println(pk);
for(int a=0;a<pageSize&&sk<p.getSegmentList().get(si);a++)
m.write(a+pk,"p"+p.getId()+".s"+si+"."+sk++, c[p.getId()]);
}
si++;
}
}
private void clearSegment(PCB p){
Integer si=0,i;
for(Integer k:p.getSegmentTable()){
i=p.getSegmentList().get(si);
ss.free(k, k+i-1);
for(int j=k;j<k+i;j++)
m.clear(j);
si++;
}
}
private void writeSegment(PCB p){
int si=0,k;
for(Integer i:p.getSegmentList()){
k=p.getSegmentTable().get(si);
for(int j=0;j<i;j++)
m.write(k++,"p"+p.getId()+".s"+si+"."+j, c[p.getId()]);
si++;
}
}
public boolean visit(int pid,int shift){//cs,ps
if(pid<0||pid>5||pcb[pid]==null)
return false;
int totalSize=0;
for(Integer i:pcb[pid].getSegmentList())
totalSize+=i;
if(shift<0||shift>=totalSize)
return false;
if(method==0){
System.out.println("hhh");
m.setPointer(pcb[pid].getStartAddress()+shift);
return true;
}
if(method==2){
for(Integer i:pcb[pid].getSegmentList())
totalSize+=i;
if(shift<0||shift>=totalSize) return false;
int pti=0;
int pn=(shift+1)/pageSize;
int psf=shift%pageSize;
if((shift+1)%pageSize!=0) pn++;
int a=pcb[pid].getPageTable().get(pti+pn-1)*pageSize+psf;
m.setPointer(a);
return true;
}
return false;
}
public boolean visit(int pid,int sid,int shift){//ss,sps
if(pid<0||pid>5||pcb[pid]==null||sid>=pcb[pid].getSegmentList().size())
return false;
int sil=pcb[pid].getSegmentList().get(sid);
if(shift<0||shift>=sil) return false;
if(method==1){
m.setPointer(pcb[pid].getSegmentTable().get(sid)+shift);
return true;
}
if(method==3){
if(shift<0||shift>=pcb[pid].getSegmentList().get(sid)) return false;
int pti=pcb[pid].getSegmentTable().get(sid);
int pn=(shift+1)/pageSize;
int psf=shift%pageSize;
if((shift+1)%pageSize!=0) pn++;
int a=pcb[pid].getPageTable().get(pti+pn-1)*pageSize+psf;
m.setPointer(a);
return true;
}
return false;
}
public Memory getM() {
return m;
}
public int getMethod() {
return method;
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
操作系统课程设计---存储管理功能
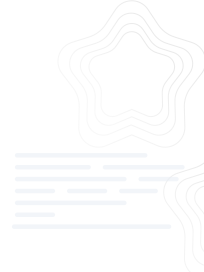
共27个文件
class:15个
java:10个
classpath:1个


温馨提示
通过编程模拟实现操作系统存储管理的基本功能,包括分区存储管理、分页存储管理,段式存储管理和段页式存储管理等机制的基本思想。对于每种管理方式,要求显示出存储空间的分配和回收的执行情况;地址空间的重定位的变换情况;动态展示相应数据结构的全部内容。
资源推荐
资源详情
资源评论
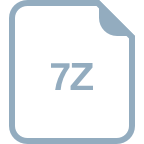
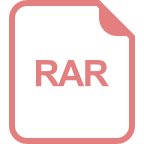
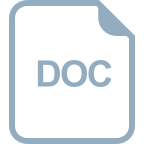
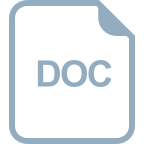
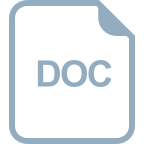
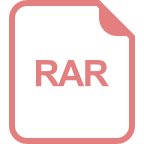
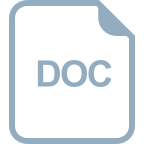
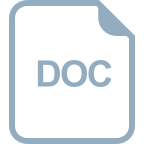
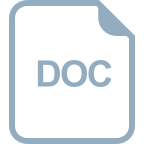
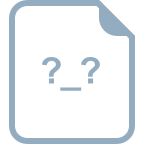
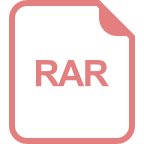
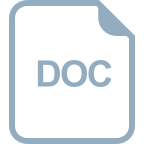
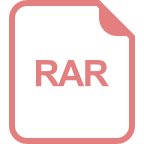
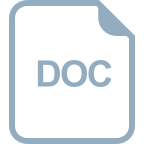
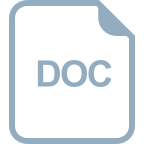
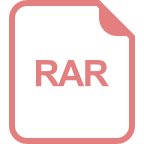
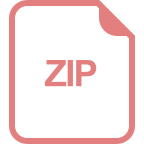
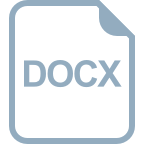
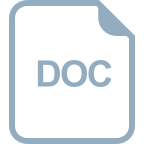
收起资源包目录



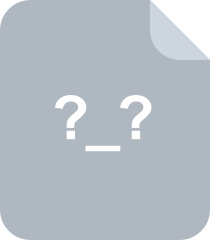
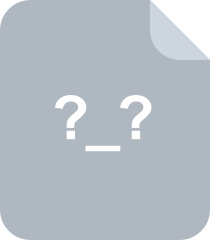
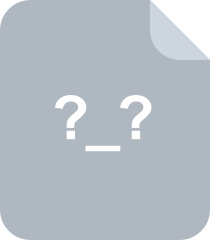
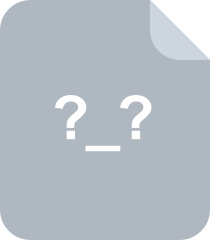
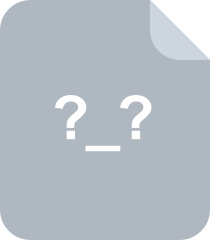
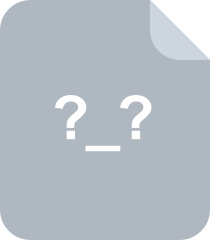
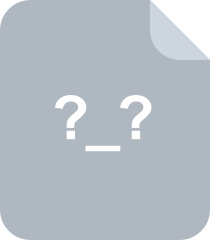
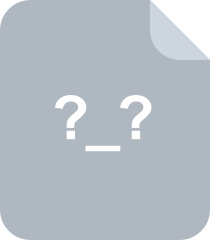
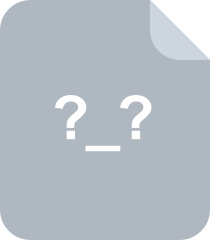
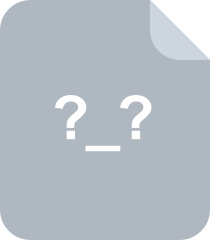
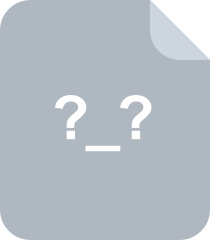
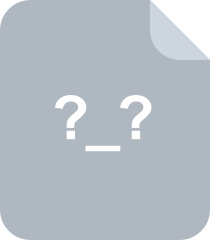
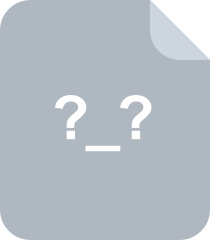
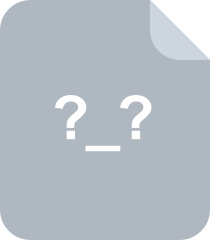
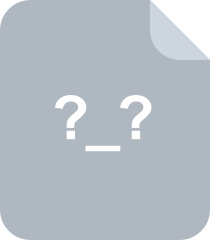

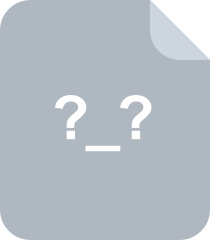
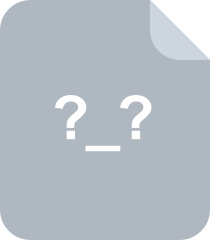
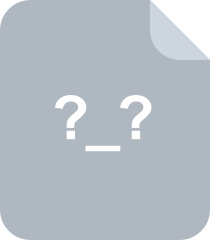
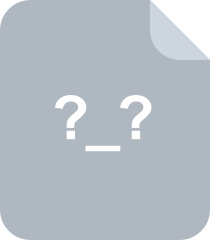
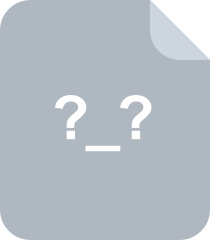
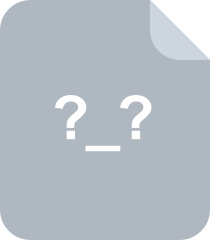
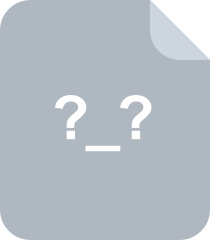
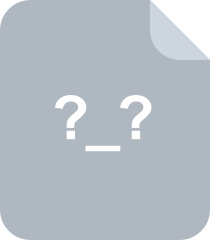
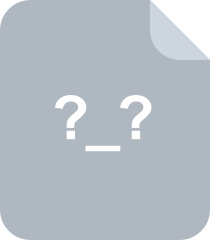
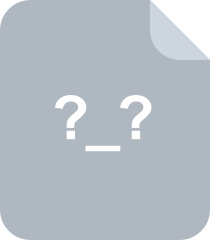
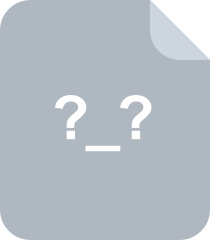
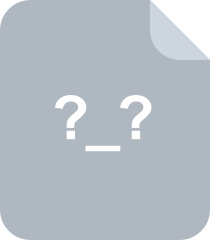
共 27 条
- 1
资源评论
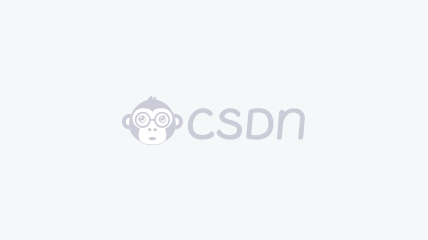
- u0102573562014-03-21功能有点简单 不过很详细了
- talentmxz2011-10-29存储管理功能的实现!
- q4550346652012-09-27内存的分配与回收,值得借鉴~~~
- 梧桐飘飞2011-12-13貌似界面显示的只有内存的分配与回收,不过代码很清楚
- a3856097222013-06-01貌似界面显示的只有内存的分配与回收,还可以 能用

wddpwzzwanggz
- 粉丝: 0
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

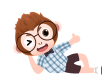
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


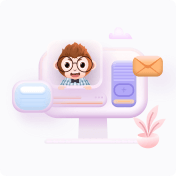
安全验证
文档复制为VIP权益,开通VIP直接复制
