package com.zook;
import java.io.IOException;
import java.io.InputStream;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import android.app.Activity;
import android.app.ProgressDialog;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.os.Bundle;
import android.os.Handler;
import android.os.Message;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.FrameLayout;
import android.widget.ImageView;
import android.widget.ProgressBar;
public class main extends Activity implements OnClickListener{
private static final String params="http://upload.wikimedia.org/wikipedia/commons/thumb/e/ea/Hukou_Waterfall.jpg/800px-Hukou_Waterfall.jpg";
private Button btnFirst,btnSecond,btnThree;
private ProgressBar progress;
private FrameLayout frameLayout;
private Bitmap bitmap=null;
ProgressDialog dialog=null;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
btnFirst=(Button)this.findViewById(R.id.btnFirst);
btnSecond=(Button)this.findViewById(R.id.btnSecond);
btnThree=(Button)this.findViewById(R.id.btnThree);
progress=(ProgressBar)this.findViewById(R.id.progress);
progress.setVisibility(View.GONE);
frameLayout=(FrameLayout)this.findViewById(R.id.frameLayout);
btnFirst.setOnClickListener(this);
btnSecond.setOnClickListener(this);
btnThree.setOnClickListener(this);
}
//前台ui线程在显示ProgressDialog,
//后台线程在下载数据,数据下载完毕,关闭进度框
@Override
public void onClick(View view) {
switch(view.getId()){
case R.id.btnFirst:
System.out.println("open");
dialog = ProgressDialog.show(this, "",
"下载数据,请稍等 …", true, true);
//启动一个后台线程
handler.post(new Runnable(){
@Override
public void run() {
//这里下载数据
try{
URL url = new URL(params);
HttpURLConnection conn = (HttpURLConnection)url.openConnection();
conn.setDoInput(true);
conn.connect();
InputStream inputStream=conn.getInputStream();
bitmap = BitmapFactory.decodeStream(inputStream);
Message msg=new Message();
msg.what=1;
System.out.println("11111111111111");
handler.sendMessage(msg);
} catch (MalformedURLException e1) {
e1.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
});
break;
case R.id.btnSecond:
MyASyncTask yncTask=new MyASyncTask(this,frameLayout);
yncTask.execute(params);
break;
case R.id.btnThree:
System.out.println("start");
progress.setVisibility(View.VISIBLE);
final Handler newhandler=new Handler();
ExecutorService executorService=Executors.newFixedThreadPool(1);
// executorService.execute(command)
executorService.execute(new Runnable(){
@Override
public void run() {
try {
URL newurl = new URL(params);
HttpURLConnection conn = (HttpURLConnection)newurl.openConnection();
conn.setDoInput(true);
conn.connect();
InputStream inputStream=conn.getInputStream();
bitmap = BitmapFactory.decodeStream(inputStream);
newhandler.post(new Runnable(){
@Override
public void run() {
ImageView view=(ImageView)frameLayout.findViewById(R.id.image);
view.setImageBitmap(bitmap);
}
});
} catch (MalformedURLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
});
progress.setVisibility(View.GONE);
break;
}
}
/**这里重写handleMessage方法,接受到子线程数据后更新UI**/
private Handler handler=new Handler(){
@Override
public void handleMessage(Message msg){
switch(msg.what){
case 1:
//关闭
ImageView view=(ImageView)frameLayout.findViewById(R.id.image);
view.setImageBitmap(bitmap);
System.out.println("close");
dialog.dismiss();
break;
}
}
};
}

warzook
- 粉丝: 0
- 资源: 4
最新资源
- (172760630)数据结构课程设计文档1
- (30485858)SSM(Spring+springmvc+mybatis)项目实例.zip
- Java Web实现电子购物系统
- 计算机网络四次实验报告参考
- (176419244)订餐系统-小程序.zip
- (176636410)微信外卖小程序源码模板
- (14173842)条形码例子
- (171674830)PYQT5+openCV项目实战:微循环仪图片、视频记录和人工对比软件源码
- (177666394)基于Qt开发的OpenCV数字图像处理工具箱.zip
- 新建 文本文档.docx
- (170644008)Eclipse+MySql+JavaSwing选课成绩管理系统
- (175526236)【动漫网页设计】源码免费分享,让你的网站更有趣!
- (177269606)使用Taro开发鸿蒙原生应用.zip
- 2_信息工程学院全国大学生职业规划报名模板(1).zip
- 计算机二级C语言考试大纲的C语言程序设计习题代码
- (177121232)windows电脑下载OpenHarmony鸿蒙命令行工具hdc-std
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


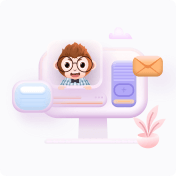