### C# 中 List 的序列化详解 #### 一、引言 序列化是将对象的状态信息转换为可以存储或传输的形式的过程。在 .NET 框架中,序列化技术被广泛应用于各种场景,例如持久化对象状态到磁盘、通过网络传输对象等。对于集合类型,尤其是 `List<T>` 这样的泛型集合,序列化同样非常重要。本文将详细介绍如何在 C# 中序列化 `List<T>` 类型,并讨论一些进阶用法。 #### 二、基础知识回顾 在深入了解序列化之前,我们先简要回顾一些基本概念。 ##### 2.1 Collection 数据类型 在 C# 中,`Collection` 主要指的是用于存储多个元素的数据结构,常见的包括但不限于 `Array`、`ArrayList`、`List<T>` 和 `Dictionary<K,V>` 等。 ##### 2.2 XML 序列化 XML (Extensible Markup Language) 是一种常用的标记语言,用于结构化地表示数据。在 .NET 中,可以通过 `System.Xml.Serialization` 命名空间提供的工具实现对象到 XML 文档的转换。 ##### 2.3 IEnumerable 接口 `IEnumerable` 是一个定义了迭代器模式的基础接口,使得类型可以支持简单的枚举。许多集合类型,包括 `List<T>`,都实现了该接口。 #### 三、序列化 `List<T>` 接下来我们将详细探讨如何序列化一个 `List<T>` 类型的集合。 ##### 3.1 基础示例 下面的代码演示了如何序列化一个 `List<Item>`,其中 `Item` 是一个自定义的类。 ```csharp using System; using System.Collections.Generic; using System.IO; using System.Xml.Serialization; namespace SerializeCollection { class Program { static void Main(string[] args) { Program test = new Program(); test.SerializeDocument("e:\\books.xml"); } public void SerializeDocument(string filename) { XmlSerializer serializers = new XmlSerializer(typeof(MyRootClass)); TextWriter myWriter = new StreamWriter(filename); MyRootClass myRootClass = new MyRootClass(); // 创建 Item 实例并添加到 List 中 Item item1 = new Item { ItemName = "Widget1", ItemCode = "w1", ItemPrice = 231, ItemQuantity = 3 }; Item item2 = new Item { ItemName = "Widget2", ItemCode = "w2", ItemPrice = 800, ItemQuantity = 2 }; myRootClass.Items.Add(item1); myRootClass.Items.Add(item2); // 序列化并写入磁盘 serializers.Serialize(myWriter, myRootClass); myWriter.Close(); } } [Serializable] public class MyRootClass { public MyRootClass() { items = new List<Item>(); } private List<Item> items; public List<Item> Items { get { return items; } set { items = value; } } } public class Item { [XmlElement(ElementName = "OrderItem")] public string ItemName { get; set; } public string ItemCode { get; set; } public decimal ItemPrice { get; set; } public int ItemQuantity { get; set; } } } ``` ##### 3.2 生成的 XML 结构 执行上述代码后,会在指定路径下生成名为 `books.xml` 的文件,内容类似于以下结构: ```xml <?xml version="1.0" encoding="utf-8"?> <MyRootClass xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema"> <Items> <OrderItem> <ItemName>Widget1</ItemName> <ItemCode>w1</ItemCode> <ItemPrice>231</ItemPrice> <ItemQuantity>3</ItemQuantity> </OrderItem> <OrderItem> <ItemName>Widget2</ItemName> <ItemCode>w2</ItemCode> <ItemPrice>800</ItemPrice> <ItemQuantity>2</ItemQuantity> </OrderItem> </Items> </MyRootClass> ``` #### 四、进阶技巧 在实际应用中,有时我们需要对序列化过程有更精细的控制,例如定制 XML 结构、添加命名空间等。 ##### 4.1 定制 XML 结构 通过使用 `XmlAttribute`、`XmlElement` 等属性,可以更精确地控制序列化结果。例如,在 `Item` 类中使用 `XmlElement` 指定元素名称: ```csharp public class Item { [XmlElement(ElementName = "ProductName")] public string ItemName { get; set; } [XmlElement(ElementName = "ProductCode")] public string ItemCode { get; set; } [XmlElement(ElementName = "Price")] public decimal ItemPrice { get; set; } [XmlElement(ElementName = "Quantity")] public int ItemQuantity { get; set; } } ``` ##### 4.2 添加命名空间 为了使 XML 更易于理解且符合标准,可以在序列化过程中添加 XML 命名空间: ```csharp XmlSerializer serializers = new XmlSerializer(typeof(MyRootClass)); TextWriter myWriter = new StreamWriter(filename); XmlSerializerNamespaces ns = new XmlSerializerNamespaces(); ns.Add("", ""); // 添加默认命名空间 serializers.Serialize(myWriter, myRootClass, ns); ``` #### 五、总结 通过本文的学习,我们了解了在 C# 中如何序列化 `List<T>` 类型,以及如何通过属性定制 XML 结构。序列化技术在现代软件开发中至关重要,尤其是在涉及数据持久化和网络通信时。掌握这些基本技能将有助于开发者更好地应对实际项目中的挑战。
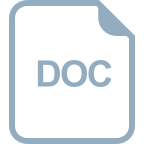
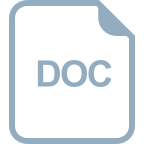
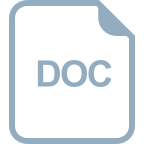
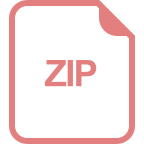
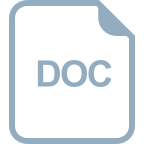
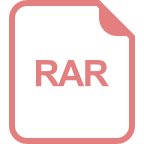
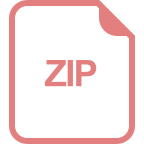
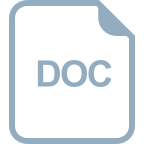
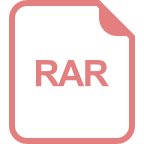
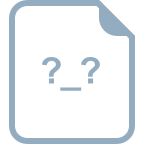
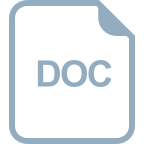
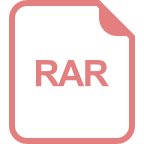
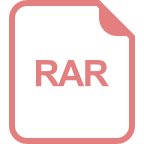
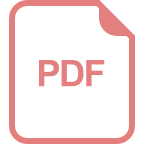
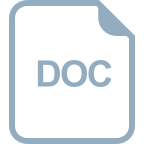
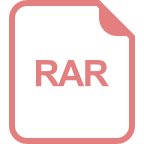
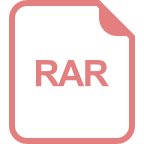
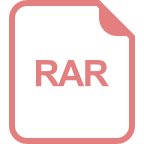
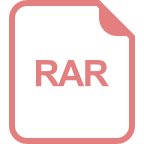
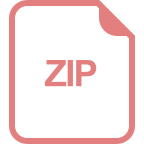
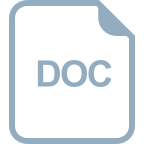
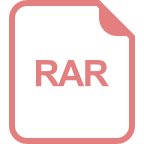
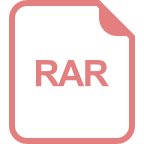
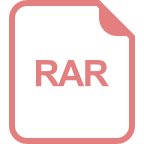
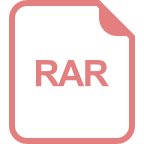
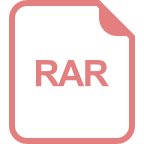
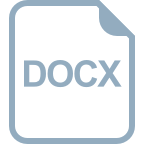
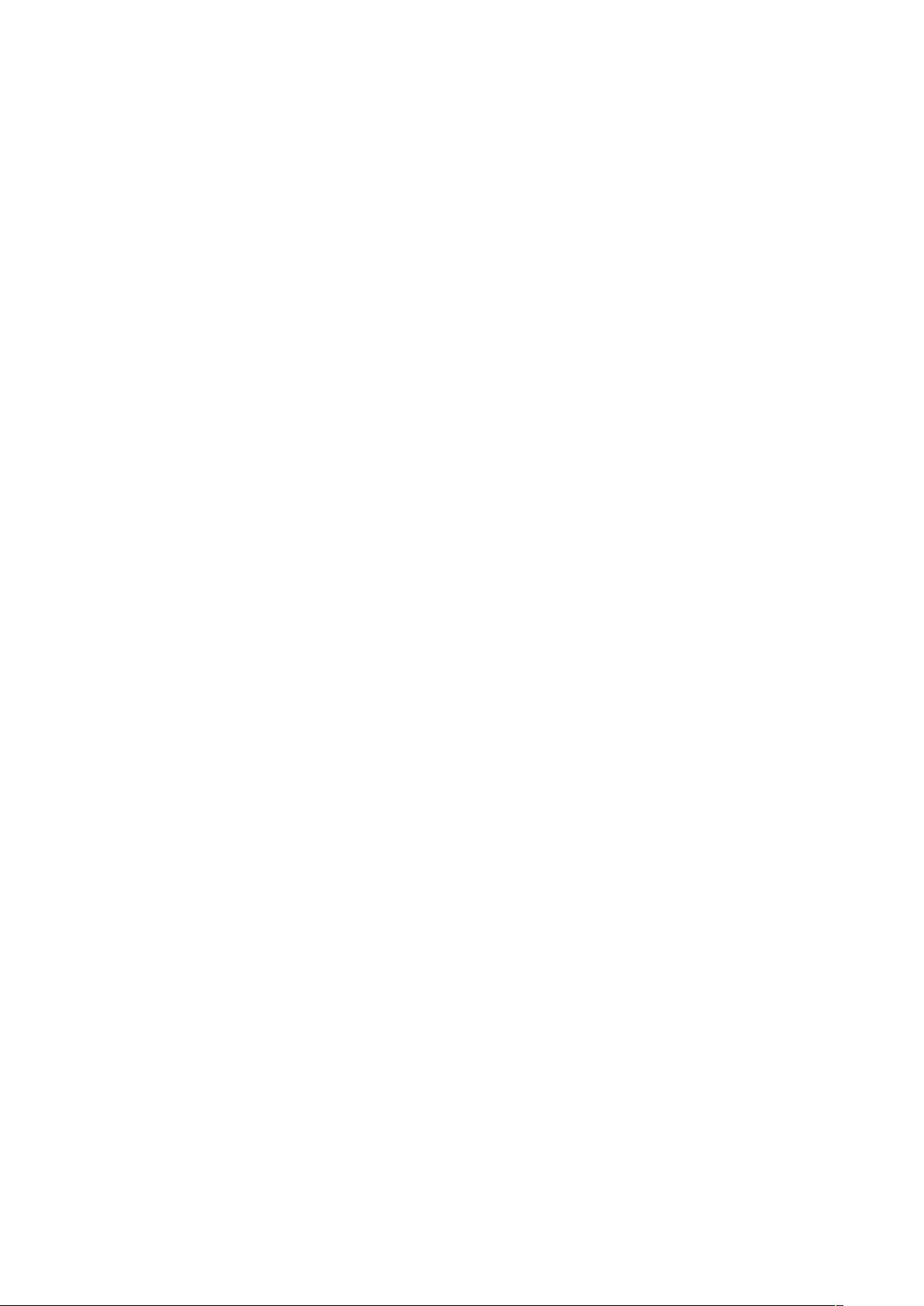
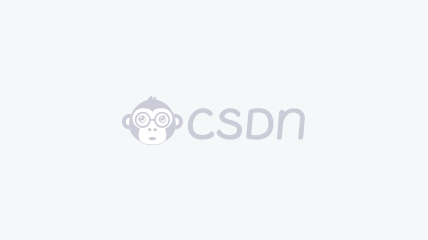

- 粉丝: 8
- 资源: 29
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

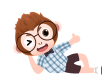
最新资源

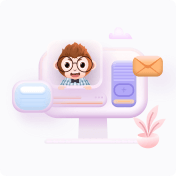
