=====================================================================
Found a 121 line (629 tokens) duplication in the following files:
Starting at line 265 of /usr/local/java/src/java/nio/DirectCharBufferU.java
Starting at line 265 of /usr/local/java/src/java/nio/DirectCharBufferS.java
DirectCharBufferS sb = (DirectCharBufferS)src;
int spos = sb.position();
int slim = sb.limit();
assert (spos <= slim);
int srem = (spos <= slim ? slim - spos : 0);
int pos = position();
int lim = limit();
assert (pos <= lim);
int rem = (pos <= lim ? lim - pos : 0);
if (srem > rem)
throw new BufferOverflowException();
unsafe.copyMemory(sb.ix(spos), ix(pos), srem << 1);
sb.position(spos + srem);
position(pos + srem);
} else if (!src.isDirect()) {
int spos = src.position();
int slim = src.limit();
assert (spos <= slim);
int srem = (spos <= slim ? slim - spos : 0);
put(src.hb, src.offset + spos, srem);
src.position(spos + srem);
} else {
super.put(src);
}
return this;
}
public CharBuffer put(char[] src, int offset, int length) {
if ((length << 1) > Bits.JNI_COPY_FROM_ARRAY_THRESHOLD) {
checkBounds(offset, length, src.length);
int pos = position();
int lim = limit();
assert (pos <= lim);
int rem = (pos <= lim ? lim - pos : 0);
if (length > rem)
throw new BufferOverflowException();
if (order() != ByteOrder.nativeOrder())
Bits.copyFromCharArray(src, offset << 1,
ix(pos), length << 1);
else
Bits.copyFromByteArray(src, offset << 1,
ix(pos), length << 1);
position(pos + length);
} else {
super.put(src, offset, length);
}
return this;
}
public CharBuffer compact() {
int pos = position();
int lim = limit();
assert (pos <= lim);
int rem = (pos <= lim ? lim - pos : 0);
unsafe.copyMemory(ix(pos), ix(0), rem << 1);
position(rem);
limit(capacity());
return this;
}
public boolean isDirect() {
return true;
}
public boolean isReadOnly() {
return false;
}
public String toString(int start, int end) {
if ((end > limit()) || (start > end))
throw new IndexOutOfBoundsException();
try {
int len = end - start;
char[] ca = new char[len];
CharBuffer cb = CharBuffer.wrap(ca);
CharBuffer db = this.duplicate();
db.position(start);
db.limit(end);
cb.put(db);
return new String(ca);
} catch (StringIndexOutOfBoundsException x) {
throw new IndexOutOfBoundsException();
}
}
// --- Methods to support CharSequence ---
public CharSequence subSequence(int start, int end) {
int len = length();
int pos = position();
assert (pos <= len);
pos = (pos <= len ? pos : len);
if ((start < 0) || (end > len) || (start > end))
throw new IndexOutOfBoundsException();
int sublen = end - start;
int off = (pos + start) << 1;
return new DirectCharBufferS(this, -1, 0, sublen, sublen, off);
=====================================================================
Found a 294 line (531 tokens) duplication in the following files:
Starting at line 486 of /usr/local/java/src/java/lang/StrictMath.java
Starting at line 575 of /usr/local/java/src/java/lang/Math.java
public static int round(float a) {
return (int)floor(a + 0.5f);
}
/**
* Returns the closest <code>long</code> to the argument. The result
* is rounded to an integer by adding 1/2, taking the floor of the
* result, and casting the result to type <code>long</code>. In other
* words, the result is equal to the value of the expression:
* <p><pre>(long)Math.floor(a + 0.5d)</pre>
* <p>
* Special cases:
* <ul><li>If the argument is NaN, the result is 0.
* <li>If the argument is negative infinity or any value less than or
* equal to the value of <code>Long.MIN_VALUE</code>, the result is
* equal to the value of <code>Long.MIN_VALUE</code>.
* <li>If the argument is positive infinity or any value greater than or
* equal to the value of <code>Long.MAX_VALUE</code>, the result is
* equal to the value of <code>Long.MAX_VALUE</code>.</ul>
*
* @param a a floating-point value to be rounded to a
* <code>long</code>.
* @return the value of the argument rounded to the nearest
* <code>long</code> value.
* @see java.lang.Long#MAX_VALUE
* @see java.lang.Long#MIN_VALUE
*/
public static long round(double a) {
return (long)floor(a + 0.5d);
}
private static Random randomNumberGenerator;
private static synchronized void initRNG() {
if (randomNumberGenerator == null)
randomNumberGenerator = new Random();
}
/**
* Returns a <code>double</code> value with a positive sign, greater
* than or equal to <code>0.0</code> and less than <code>1.0</code>.
* Returned values are chosen pseudorandomly with (approximately)
* uniform distribution from that range.
* <p>
* When this method is first called, it creates a single new
* pseudorandom-number generator, exactly as if by the expression
* <blockquote><pre>new java.util.Random</pre></blockquote>
* This new pseudorandom-number generator is used thereafter for all
* calls to this method and is used nowhere else.
* <p>
* This method is properly synchronized to allow correct use by more
* than one thread. However, if many threads need to generate
* pseudorandom numbers at a great rate, it may reduce contention for
* each thread to have its own pseudorandom-number generator.
*
* @return a pseudorandom <code>double</code> greater than or equal
* to <code>0.0</code> and less than <code>1.0</code>.
* @see java.util.Random#nextDouble()
*/
public static double random() {
if (randomNumberGenerator == null) initRNG();
return randomNumberGenerator.nextDouble();
}
/**
* Returns the absolute value of an <code>int</code> value.
* If the argument is not negative, the argument is returned.
* If the argument is negative, the negation of the argument is returned.
* <p>
* Note that if the argument is equal to the value of
* <code>Integer.MIN_VALUE</code>, the most negative representable
* <code>int</code> value, the result is that same value, which is
* negative.
*
* @param a the argument whose absolute value is to be determined
* @return the absolute value of the argument.
* @see java.lang.Integer#MIN_VALUE
*/
public static int abs(int a) {
return (a < 0) ? -a : a;
}
/**
* Returns the absolute value of a <code>long</code> value.
* If the argument is not negative, the argument is returned.
* If the argument is negative, the negation of the argument is returned.
* <p>
* Note that if the argument is equal to the value of
* <code>Long.MIN_VALUE</code>, the most negative representable
* <code>long</code> value, the result is that same value, which is
* negative.
*
* @param a the argument whose absolute value is to be determined
* @return the absolute value of the argument.
* @see java.lang.Long#MIN_VALUE
*/
public static long abs(long a) {
return (a < 0) ? -a : a;
}
/**
* Returns the absolute value of a <code>float</code> value.
* If the argument is not negative, the argument is returned.
* If the argument is negative, the negation of the argument is returned.
* Special cases:
* <ul><li>If the argument is positive zero or negative zero, the
* result is positive zero.
* <li>If the argument is infinite, the result is positive infinity.
* <li>If the argument is NaN, the result is NaN.</ul>
* In other words, the result is the same as the value of the expression:
没有合适的资源?快使用搜索试试~ 我知道了~
pmdJava程序代码检查工具
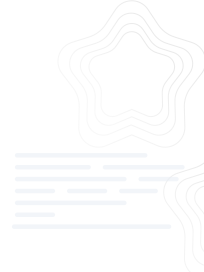
共2482个文件
html:2394个
gif:22个
jar:12个


温馨提示
PMD是一款采用BSD协议发布的Java程序代码检查工具。该工具可以做到检查Java代码中是否含有未使用的变量、是否含有空的抓取块、是否含有不必要的对象等。该软件功能强大,扫描效率高,是Java程序员debug的好帮手。 它可以为您检查Java代码中存在的如下问题: 1、隐藏的bug,例如空的try catch,switch 2、未调用的代码,例如没有使用的局部变量、参数和私有方法 3、未优化的代码,例如String的不正确使用 4、过于复杂的表达式,没有必要的表达式循环,判断 5、重复代码 PMD支持的编辑器包括: JDeveloper、Eclipse、JEdit、JBuilder、BlueJ、CodeGuide、NetBeans/Sun Java Studio Enterprise/Creator、IntelliJ IDEA、TextPad、Maven、Ant,、Gel、JCreator和Emacs。 此次版本的主要变化: 1、修复了已有规则的一些bug 2、修改了 CPD 算法 3、JSP/JSF 解析器支持 Unicode 4、可处理 标签; 5、AST HtmlScript 节点包含内容,支持 Ecmascript 等等
资源推荐
资源详情
资源评论
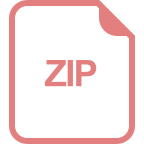
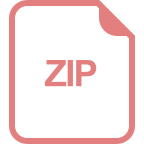
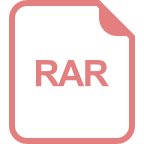
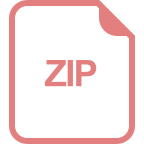
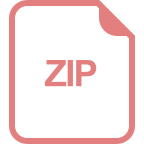
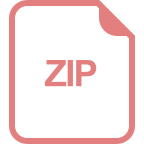
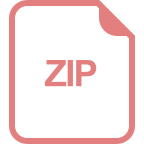
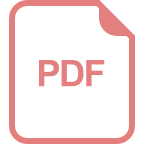
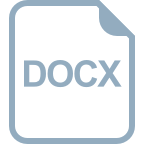
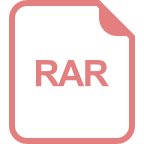
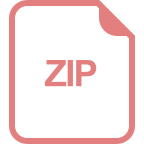
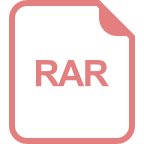
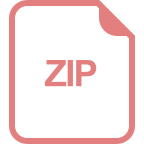
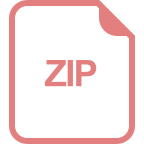
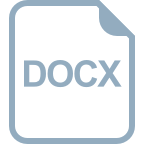
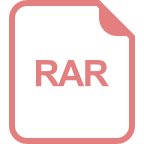
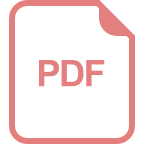
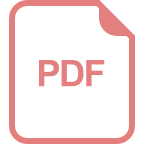
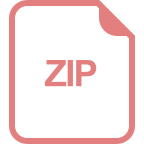
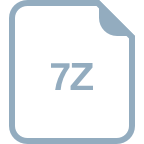
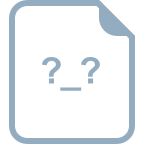
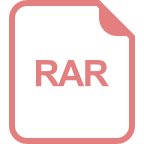
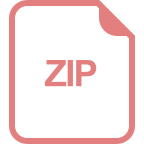
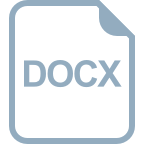
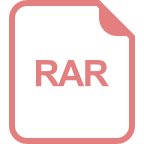
收起资源包目录

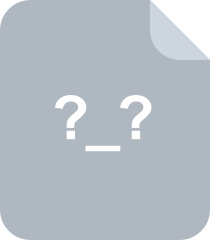
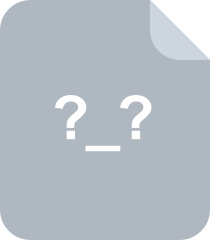
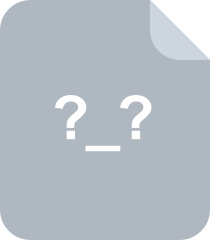
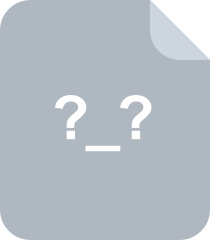
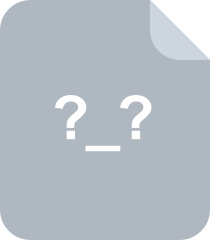
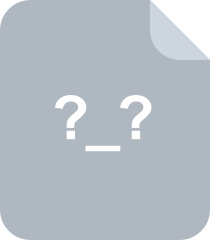
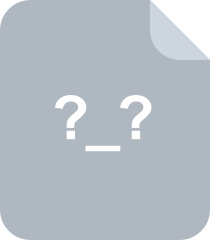
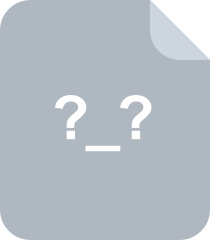
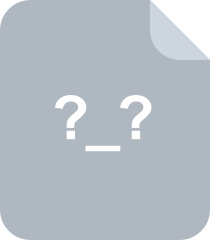
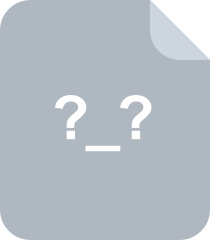
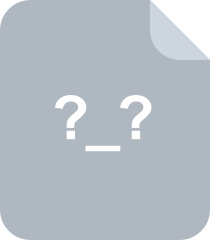
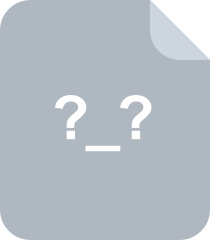
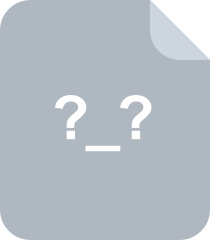
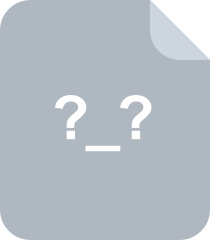
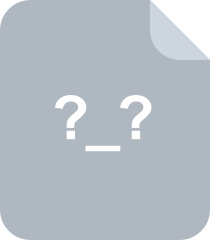
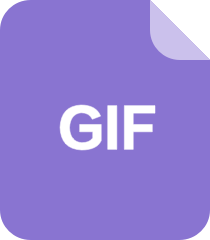
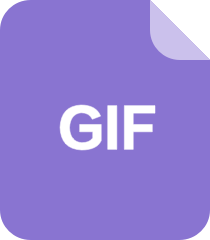
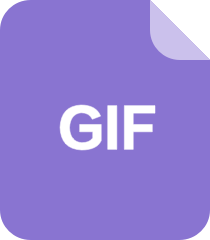
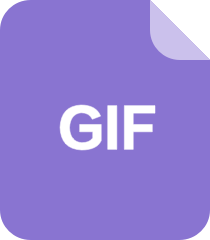
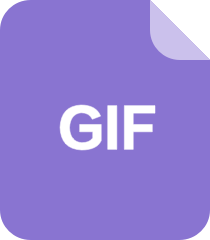
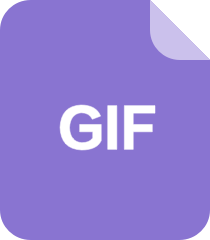
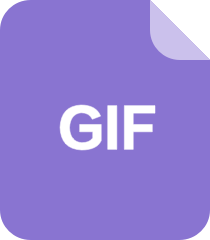
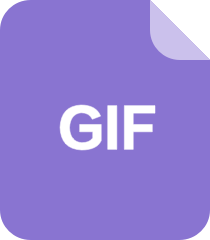
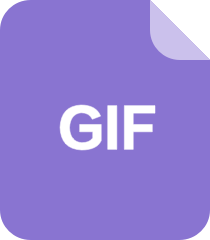
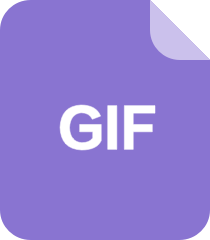
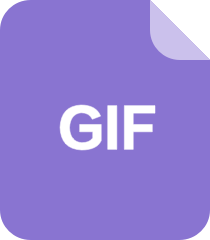
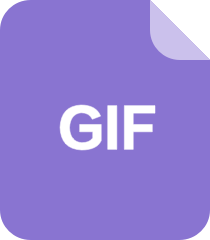
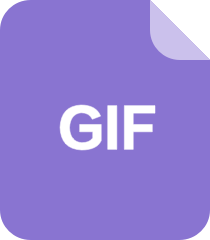
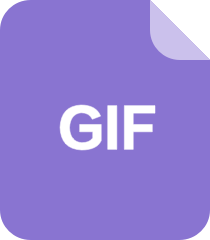
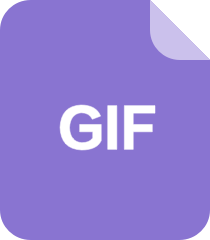
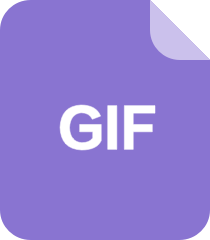
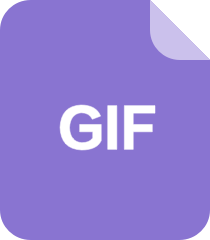
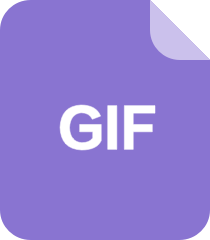
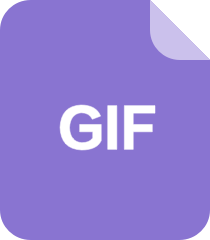
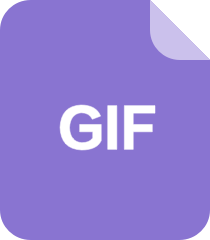
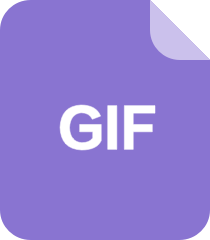
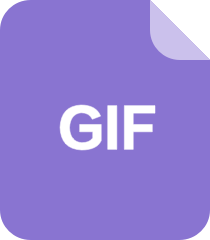
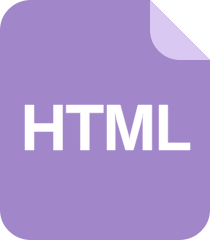
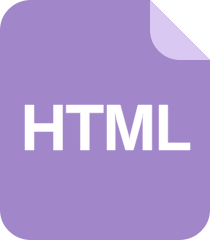
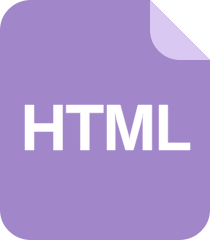
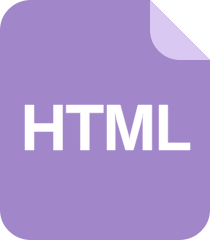
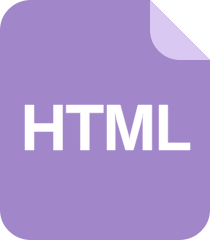
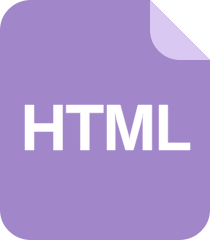
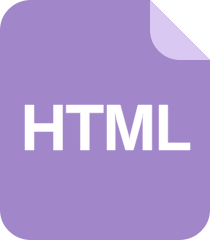
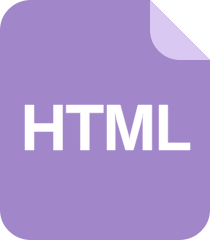
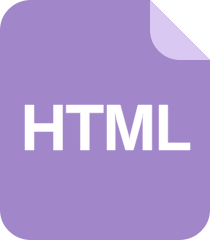
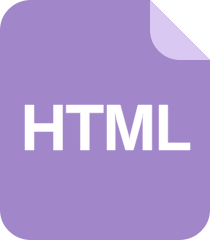
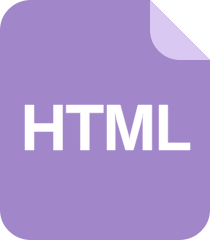
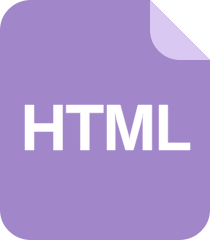
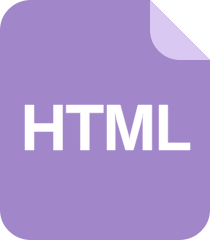
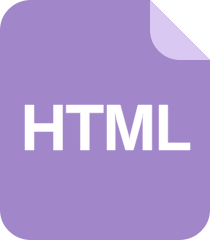
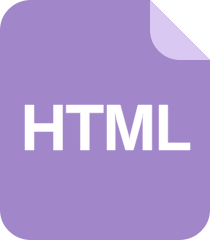
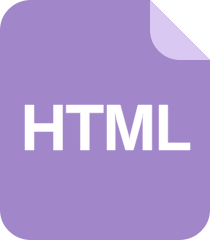
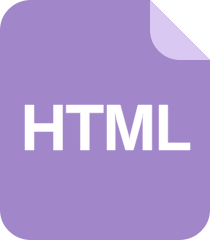
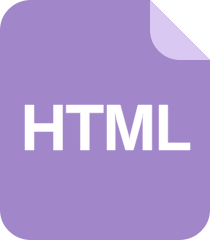
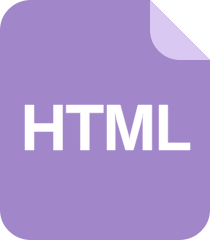
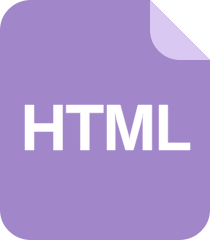
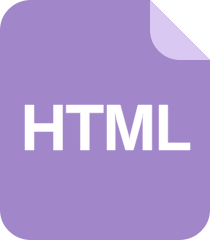
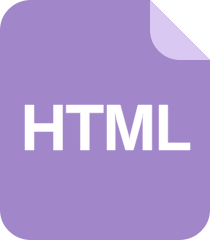
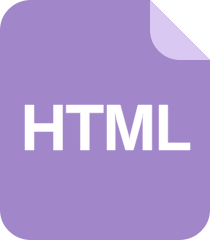
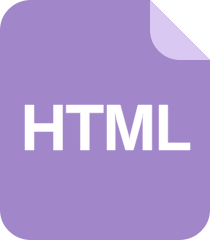
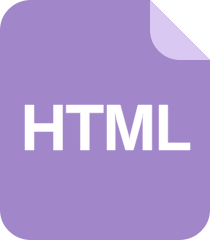
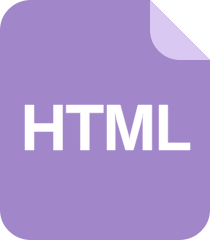
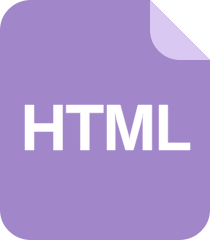
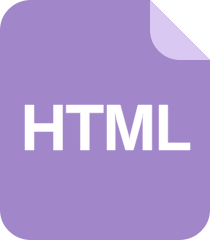
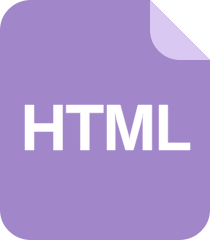
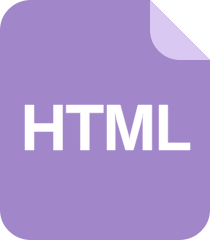
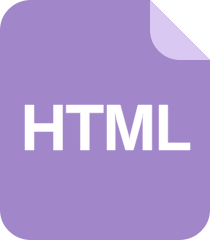
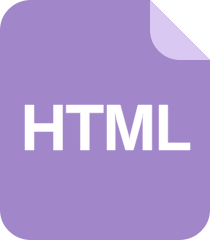
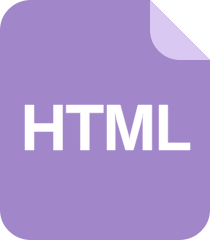
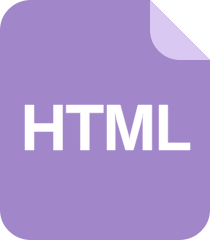
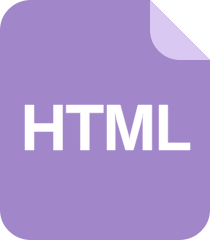
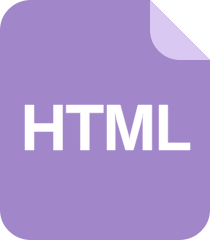
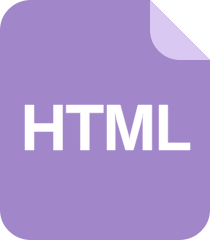
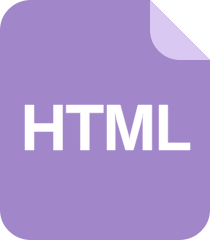
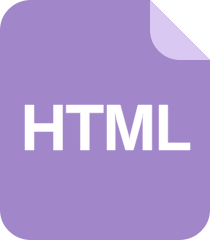
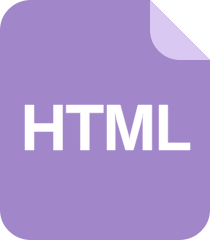
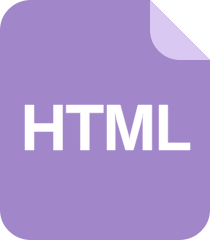
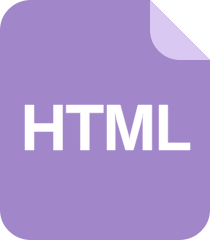
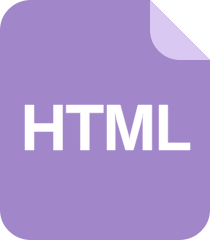
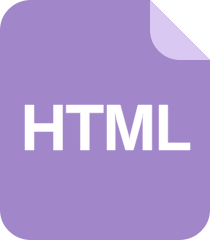
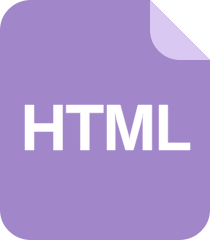
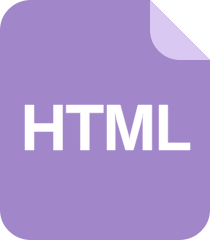
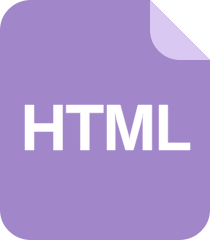
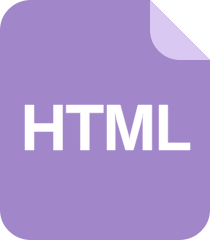
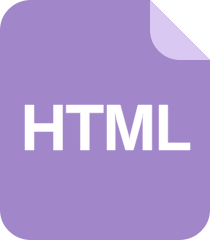
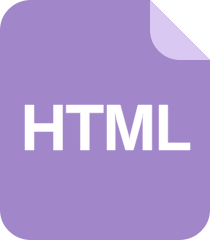
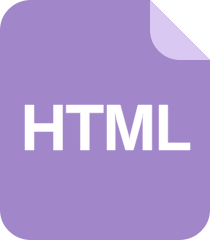
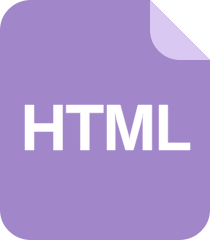
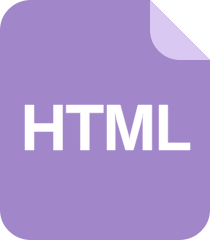
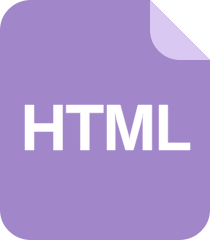
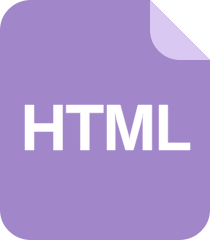
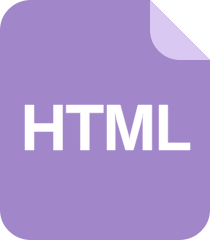
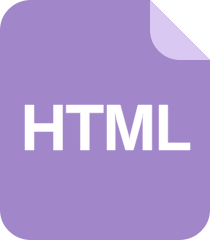
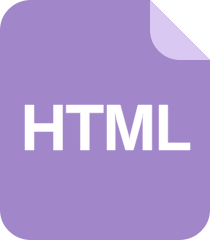
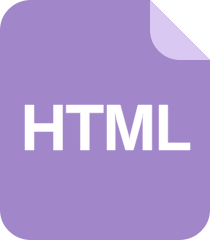
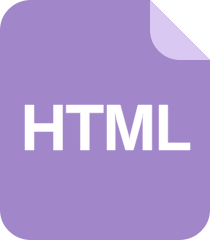
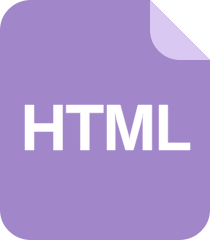
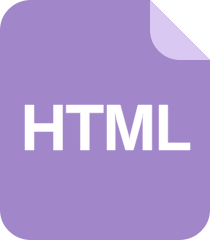
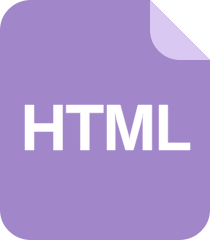
共 2482 条
- 1
- 2
- 3
- 4
- 5
- 6
- 25

wang31xin
- 粉丝: 1
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

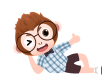
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


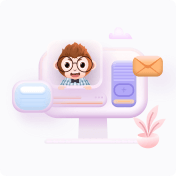
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
前往页