package person.wangchen11.qrcode;
/**
* java 二维码生成工具
*
* @author wangchen11,望尘11
* q1012371864
*/
import java.util.LinkedList;
import person.wangchen11.rscode.RS;
public class QRCode {
private int mSize;//尺寸
private boolean []mData;
private boolean []mIsData;//用于标示是否为数据区
public final static boolean BLACK_BLOCK=true;
public final static boolean WHITE_BLOCK=!BLACK_BLOCK;
public final static boolean IS_NOT_DATA=true;
public final static boolean IS_DATA=!IS_NOT_DATA;
public final static byte MODE_ECI=0x7;//0b0111
public final static byte MODE_NUMERIC=0x1;//0b0001
public final static byte MODE_ALPHANUMERIC=0x2;//0b0010
public final static byte MODE_8BIT_BYTE=0x4;//0100
public final static byte MODE_KANJI=0x8;//01000
public final static byte MODE_STRUCTURED_APPEND=0x3;//0b0011
public final static byte MODE_FNC1=0x5;//0b0101(first postion)
public final static byte MODE_FNC2=0x9;//0b1001(second postion)
public final static byte MODE_TERMINATOR=0x0;//0b0000(end of message)
public final static byte ERROR_CORRECTION_LEVEL_L= 0;
public final static byte ERROR_CORRECTION_LEVEL_M= 1;
public final static byte ERROR_CORRECTION_LEVEL_Q= 2;
public final static byte ERROR_CORRECTION_LEVEL_H= 3;
private final static boolean PositionDetectionMap[][] =new boolean[][]{
{BLACK_BLOCK,BLACK_BLOCK,BLACK_BLOCK,BLACK_BLOCK,BLACK_BLOCK,BLACK_BLOCK,BLACK_BLOCK},
{BLACK_BLOCK,WHITE_BLOCK,WHITE_BLOCK,WHITE_BLOCK,WHITE_BLOCK,WHITE_BLOCK,BLACK_BLOCK},
{BLACK_BLOCK,WHITE_BLOCK,BLACK_BLOCK,BLACK_BLOCK,BLACK_BLOCK,WHITE_BLOCK,BLACK_BLOCK},
{BLACK_BLOCK,WHITE_BLOCK,BLACK_BLOCK,BLACK_BLOCK,BLACK_BLOCK,WHITE_BLOCK,BLACK_BLOCK},
{BLACK_BLOCK,WHITE_BLOCK,BLACK_BLOCK,BLACK_BLOCK,BLACK_BLOCK,WHITE_BLOCK,BLACK_BLOCK},
{BLACK_BLOCK,WHITE_BLOCK,WHITE_BLOCK,WHITE_BLOCK,WHITE_BLOCK,WHITE_BLOCK,BLACK_BLOCK},
{BLACK_BLOCK,BLACK_BLOCK,BLACK_BLOCK,BLACK_BLOCK,BLACK_BLOCK,BLACK_BLOCK,BLACK_BLOCK},
};
private final static boolean AlignmentPatternMap[][] =new boolean[][]{
{BLACK_BLOCK,BLACK_BLOCK,BLACK_BLOCK,BLACK_BLOCK,BLACK_BLOCK},
{BLACK_BLOCK,WHITE_BLOCK,WHITE_BLOCK,WHITE_BLOCK,BLACK_BLOCK},
{BLACK_BLOCK,WHITE_BLOCK,BLACK_BLOCK,WHITE_BLOCK,BLACK_BLOCK},
{BLACK_BLOCK,WHITE_BLOCK,WHITE_BLOCK,WHITE_BLOCK,BLACK_BLOCK},
{BLACK_BLOCK,BLACK_BLOCK,BLACK_BLOCK,BLACK_BLOCK,BLACK_BLOCK},
};
public int getVersion()
{
return (mSize-21)/4+1;
}
public int getSize()
{
return mSize;
}
public boolean isBlack(int x,int y)
{
return mData[x+y*mSize]==BLACK_BLOCK;
}
/**
*自动选用版本编码字符串
*目前 仅实现了字节流模式的编码
*
*@data 待编码数据流
*@dataLength 待编码数据流长度
*@errCodeLevel 纠错等级
*@maskPattern [0,7]选用什么掩码
*@mode 编码模式,暂未使用 ,请填 0
*/
public static QRCode autoEncodeString(byte []data,int dataLength,int errCodeLevel,int maskPattern,int mode)
{
int i;
QRCode code=null;
//[1,40]版本号中选择最小且能用的
for(i=1;i<=40;i++)
{
code=encodeString(data,dataLength,i,errCodeLevel,maskPattern,mode);
if(code!=null)
return code;
}
return null;
}
/**
*编码字符串
*目前 仅实现了字节流模式的编码
*
*@data 待编码数据流
*@dataLength 待编码数据流长度
*@version 版本号[1,40] 版本号越大生成的图片规格也越大
*@errCodeLevel 纠错等级
*@maskPattern [0,7]选用什么掩码
*@mode 编码模式,暂未使用 ,请填 0
*/
public static QRCode encodeString(byte []data,int dataLength,int version,int errCodeLevel,int maskPattern,int mode)
{
byte []encodedData=null;
QRCode code=null;
if(dataLength<=0)
return null;
encodedData=encodeData(data, version, errCodeLevel,mode);
if(encodedData==null)
return null;
code=createEmptyQRCode(version,errCodeLevel,maskPattern);
code.drawData(encodedData,encodedData.length);
code.maskData(maskPattern);
return code;
}
public static QRCode createEmptyQRCode(int version,int errorLevel,int maskPattern)
{
QRCode code=null;
code=new QRCode();
code.mSize=getSizeByVersion(version);
code.mData=new boolean[(code.mSize*code.mSize)];
code.mIsData=new boolean[(code.mSize*code.mSize)];
RS.memset(code.mData,WHITE_BLOCK,code.mSize*code.mSize);
RS.memset(code.mIsData,IS_DATA,code.mSize*code.mSize);
//System.out.println("new :");
//code.printQRCode();
code.drawPositionDetections();
code.drawTimingPatterns();
code.drawDarkModule();
code.drawUnusedSpace();
//System.out.println("drawUnusedSpace :");
//code.printQRCode();
code.drawAlignmentPatterns();
code.drawFormatInformation(errorLevel,maskPattern);
code.drawVersionInfomation(version);
//System.out.println("drawVersionInfomation :");
//code.printQRCode();
return code;
}
/**
*画所有的定位发现框
*/
void drawPositionDetections()
{
drawPositionDetection(0,0);
drawPositionDetection(this.mSize-7,0);
drawPositionDetection(0,this.mSize-7);
}
/**
*画定位发现框
*/
void drawPositionDetection(int xPostion,int yPostion)
{
int i;
int j;
int pos;
for(i=0;i<7;i++)
{
for(j=0;j<7;j++)
{
pos= xPostion+j+(yPostion+i)*this.mSize;
this.mData[pos] = PositionDetectionMap[i][j];
this.mIsData[pos] = IS_NOT_DATA;
}
}
}
void drawAlignmentPattern(int xPostion,int yPostion)
{
int i;
int j;
int pos;
if( (xPostion<=8&&yPostion<=8)||(xPostion<=8&&yPostion+4>=mSize-9)||(xPostion+4>=mSize-9&&yPostion<=8) )
{
return ;
}
for(i=0;i<5;i++)
{
for(j=0;j<5;j++)
{
pos=(xPostion+j)+(yPostion+i)*this.mSize;
this.mData[pos]=AlignmentPatternMap[i][j];
this.mIsData[pos]=IS_NOT_DATA;
}
}
}
int alignmentPattern[][] =new int[][] {
{ 0, 0},
{ 0, 0}, {18, 0}, {22, 0}, {26, 0}, {30, 0}, // 1- 5
{34, 0}, {22, 38}, {24, 42}, {26, 46}, {28, 50}, // 6-10
{30, 54}, {32, 58}, {34, 62}, {26, 46}, {26, 48}, //11-15
{26, 50}, {30, 54}, {30, 56}, {30, 58}, {34, 62}, //16-20
{28, 50}, {26, 50}, {30, 54}, {28, 54}, {32, 58}, //21-25
{30, 58}, {34, 62}, {26, 50}, {30, 54}, {26, 52}, //26-30
{30, 56}, {34, 60}, {30, 58}, {34, 62}, {30, 54}, //31-35
{24, 50}, {28, 54}, {32, 58}, {26, 54}, {30, 58}, //35-40
};
/**
*画矫正框
*/
void drawAlignmentPatterns()
{
int i,j;
int version=(this.mSize-21)/4+1;
if(alignmentPattern[ version ][0]<=0)
return ;
for(i=6;i<this.mSize-3;)
{
for(j=6;j<this.mSize-3;)
{
drawAlignmentPattern(j-2,i-2);
if(j>=alignmentPattern[ version ][0])
{
if(alignmentPattern[ version ][1]<=0)
break;
j+=(alignmentPattern[ version ][1]-alignmentPattern[ version ][0]);
}
else
j+=(alignmentPattern[ version ][0]-6);
}
if(i>=alignmentPattern[ version ][0])
{
if(alignmentPattern[ version ][1]<=0)
break;
i+=(alignmentPattern[ version ][1]-alignmentPattern[ version ][0]);
}
else
i+=(alignmentPattern[ version ][0]-6);
}
}
/**
*画校校正线
*/
void drawTimingPatterns()
{
int i;
int pos;
//y=6
for(i=7;i<this.mSize-7;i++)
{
pos=(6)*this.mSize+i;
if(i%2==1)
{
this.mData[pos]=WHITE_BLOCK;
}
else
{
this.mData[pos]=BLACK_BLOCK;
}
this.mIsData[pos] = IS_NOT_DATA;
}
//x=6
for(i=7;i<this.mSize-7;i++)
{
pos=i*this.mSize+6;
if(i%2==1)
{
this.mData[pos]=WHITE_BLOCK;
}
else
{
this.mData[pos]=BLACK_BLOCK;
}
this.mIsData[pos] = IS_NOT_DATA;
}
}
void drawDarkModule()
{
int pos;
pos=(this.mSize-8)*this.mSize+8;
this.mData[pos]=BLACK_BLOCK;
this.mIsData[pos] = IS_NOT_DATA;
}
/**
*画格式信息
*@errorLevel 0,1,2,3 2bit &0b 11 <<13
*@maskPattern 8个mask可供选择 3bit &0b 111 <<10
*@bch 校验码 10bit &0b 11 1111

w1012371864
- 粉丝: 3
- 资源: 5
最新资源
- 数据库大作业01234.zip
- 飞机故障诊断技术学期考查作业模板:编写规范及内容指引
- 纯电动汽车两档ATM变速箱simulink模型,模型实现了两档AMT挡策略和挡过程仿真,内含详细文档和注释模型,可运行
- 基于LM393比较器与LM321运放电流采样及硬件过流检测电路
- 4-IEEE trans顶刊复现,水下机器人AUV的路径规划和基于模型预测控制MPC的跟踪框架 参考文献和建模过程请参考图片中的文章,本代码包括路径规划和MPC路径跟踪两个模块,两个模块均采用优化求
- 数据挖掘管道搭建示例 基于大航杯“智造扬中”电力AI大赛.zip
- MATLAB直线倒立摆一阶倒立摆LQR控制仿真,小车倒立摆起摆和平衡控制,附带参考文献 三种控制方法对比 pd控制、lqr控制、mpc模型预测控制
- anaconda配置pytorch环境.md
- 数据结构与算法基础(青岛大学-王卓).zip
- 无穷大功率电源供电系统三相短路Matlab Simulink仿真 1.仿真在0.02s变压器低压母线发生三相短路故障,仿真其短路电流周期分量幅值和冲击电流的大小 2.仿真的具体参数见下图,按照仿真数据
- COMSOL 光学 手性 BIC 仿真 光子晶体板中连续域束缚态 BIC 赋予的手性 包含正入射斜入射琼斯矩阵透射谱,模式耦合各种透射谱分量,动量空间偏振图 下图是仿真文件截图,所见即所得
- 日常总结java + 大数据.zip
- 暨南大学计算机系数据库课程设计.zip
- 本系统是我的毕业设计项目,题目为“基于用户画像的电影推荐系统的设计与实现” 主要是以Django作为基础框架,采用MTV模式,数据库使用MongoDB、MySQL和Redis,以从豆瓣平台爬取.zip
- 本项目使用C++实现基于跳表实现的轻量级键值型存储引擎,其主要功能有插入数据、查询数据、删除数据、数据展示、数据库大小、数据库清空、数据落盘以及文件加载数据 .zip
- 条形码的那些事儿:为什么 12345242 变成了 12345243?
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


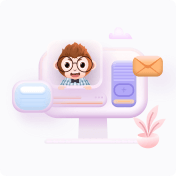