# Faker
[](https://codecov.io/gh/fzaninotto/Faker)
Faker is a PHP library that generates fake data for you. Whether you need to bootstrap your database, create good-looking XML documents, fill-in your persistence to stress test it, or anonymize data taken from a production service, Faker is for you.
Faker is heavily inspired by Perl's [Data::Faker](http://search.cpan.org/~jasonk/Data-Faker-0.07/), and by ruby's [Faker](https://rubygems.org/gems/faker).
Faker requires PHP >= 5.3.3.
[](https://packagist.org/packages/fzaninotto/faker) [](https://travis-ci.org/fzaninotto/Faker) [](https://insight.sensiolabs.com/projects/eceb78a9-38d4-4ad5-8b6b-b52f323e3549)
# Table of Contents
- [Installation](#installation)
- [Basic Usage](#basic-usage)
- [Formatters](#formatters)
- [Base](#fakerproviderbase)
- [Lorem Ipsum Text](#fakerproviderlorem)
- [Person](#fakerprovideren_usperson)
- [Address](#fakerprovideren_usaddress)
- [Phone Number](#fakerprovideren_usphonenumber)
- [Company](#fakerprovideren_uscompany)
- [Real Text](#fakerprovideren_ustext)
- [Date and Time](#fakerproviderdatetime)
- [Internet](#fakerproviderinternet)
- [User Agent](#fakerprovideruseragent)
- [Payment](#fakerproviderpayment)
- [Color](#fakerprovidercolor)
- [File](#fakerproviderfile)
- [Image](#fakerproviderimage)
- [Uuid](#fakerprovideruuid)
- [Barcode](#fakerproviderbarcode)
- [Miscellaneous](#fakerprovidermiscellaneous)
- [Biased](#fakerproviderbiased)
- [Html Lorem](#fakerproviderhtmllorem)
- [Modifiers](#modifiers)
- [Localization](#localization)
- [Populating Entities Using an ORM or an ODM](#populating-entities-using-an-orm-or-an-odm)
- [Seeding the Generator](#seeding-the-generator)
- [Faker Internals: Understanding Providers](#faker-internals-understanding-providers)
- [Real Life Usage](#real-life-usage)
- [Language specific formatters](#language-specific-formatters)
- [Third-Party Libraries Extending/Based On Faker](#third-party-libraries-extendingbased-on-faker)
- [License](#license)
## Installation
```sh
composer require fzaninotto/faker
```
## Basic Usage
### Autoloading
Faker supports both `PSR-0` as `PSR-4` autoloaders.
```php
<?php
# When installed via composer
require_once 'vendor/autoload.php';
```
You can also load `Fakers` shipped `PSR-0` autoloader
```php
<?php
# Load Fakers own autoloader
require_once '/path/to/Faker/src/autoload.php';
```
*alternatively, you can use any another PSR-4 compliant autoloader*
### Create fake data
Use `Faker\Factory::create()` to create and initialize a faker generator, which can generate data by accessing properties named after the type of data you want.
```php
<?php
// use the factory to create a Faker\Generator instance
$faker = Faker\Factory::create();
// generate data by accessing properties
echo $faker->name;
// 'Lucy Cechtelar';
echo $faker->address;
// "426 Jordy Lodge
// Cartwrightshire, SC 88120-6700"
echo $faker->text;
// Dolores sit sint laboriosam dolorem culpa et autem. Beatae nam sunt fugit
// et sit et mollitia sed.
// Fuga deserunt tempora facere magni omnis. Omnis quia temporibus laudantium
// sit minima sint.
```
Even if this example shows a property access, each call to `$faker->name` yields a different (random) result. This is because Faker uses `__get()` magic, and forwards `Faker\Generator->$property` calls to `Faker\Generator->format($property)`.
```php
<?php
for ($i = 0; $i < 10; $i++) {
echo $faker->name, "\n";
}
// Adaline Reichel
// Dr. Santa Prosacco DVM
// Noemy Vandervort V
// Lexi O'Conner
// Gracie Weber
// Roscoe Johns
// Emmett Lebsack
// Keegan Thiel
// Wellington Koelpin II
// Ms. Karley Kiehn V
```
**Tip**: For a quick generation of fake data, you can also use Faker as a command line tool thanks to [faker-cli](https://github.com/bit3/faker-cli).
## Formatters
Each of the generator properties (like `name`, `address`, and `lorem`) are called "formatters". A faker generator has many of them, packaged in "providers". Here is a list of the bundled formatters in the default locale.
### `Faker\Provider\Base`
randomDigit // 7
randomDigitNot(5) // 0, 1, 2, 3, 4, 6, 7, 8, or 9
randomDigitNotNull // 5
randomNumber($nbDigits = NULL, $strict = false) // 79907610
randomFloat($nbMaxDecimals = NULL, $min = 0, $max = NULL) // 48.8932
numberBetween($min = 1000, $max = 9000) // 8567
randomLetter // 'b'
// returns randomly ordered subsequence of a provided array
randomElements($array = array ('a','b','c'), $count = 1) // array('c')
randomElement($array = array ('a','b','c')) // 'b'
shuffle('hello, world') // 'rlo,h eoldlw'
shuffle(array(1, 2, 3)) // array(2, 1, 3)
numerify('Hello ###') // 'Hello 609'
lexify('Hello ???') // 'Hello wgt'
bothify('Hello ##??') // 'Hello 42jz'
asciify('Hello ***') // 'Hello R6+'
regexify('[A-Z0-9._%+-]+@[A-Z0-9.-]+\.[A-Z]{2,4}'); // sm0@y8k96a.ej
### `Faker\Provider\Lorem`
word // 'aut'
words($nb = 3, $asText = false) // array('porro', 'sed', 'magni')
sentence($nbWords = 6, $variableNbWords = true) // 'Sit vitae voluptas sint non voluptates.'
sentences($nb = 3, $asText = false) // array('Optio quos qui illo error.', 'Laborum vero a officia id corporis.', 'Saepe provident esse hic eligendi.')
paragraph($nbSentences = 3, $variableNbSentences = true) // 'Ut ab voluptas sed a nam. Sint autem inventore aut officia aut aut blanditiis. Ducimus eos odit amet et est ut eum.'
paragraphs($nb = 3, $asText = false) // array('Quidem ut sunt et quidem est accusamus aut. Fuga est placeat rerum ut. Enim ex eveniet facere sunt.', 'Aut nam et eum architecto fugit repellendus illo. Qui ex esse veritatis.', 'Possimus omnis aut incidunt sunt. Asperiores incidunt iure sequi cum culpa rem. Rerum exercitationem est rem.')
text($maxNbChars = 200) // 'Fuga totam reiciendis qui architecto fugiat nemo. Consequatur recusandae qui cupiditate eos quod.'
### `Faker\Provider\en_US\Person`
title($gender = null|'male'|'female') // 'Ms.'
titleMale // 'Mr.'
titleFemale // 'Ms.'
suffix // 'Jr.'
name($gender = null|'male'|'female') // 'Dr. Zane Stroman'
firstName($gender = null|'male'|'female') // 'Maynard'
firstNameMale // 'Maynard'
firstNameFemale // 'Rachel'
lastName // 'Zulauf'
### `Faker\Provider\en_US\Address`
cityPrefix // 'Lake'
secondaryAddress // 'Suite 961'
state // 'NewMexico'
stateAbbr // 'OH'
citySuffix // 'borough'
streetSuffix // 'Keys'
buildingNumber // '484'
city // 'West Judge'
streetName // 'Keegan Trail'
streetAddress // '439 Karley Loaf Suite 897'
postcode // '17916'
address // '8888 Cummings Vista Apt. 101, Susanbury, NY 95473'
country // 'Falkland Islands (Malvinas)'
latitude($min = -90, $max = 90) // 77.147489
longitude($min = -180, $max = 180) // 86.211205
### `Faker\Provider\en_US\PhoneNumber`
phoneNumber // '201-886-026
没有合适的资源?快使用搜索试试~ 我知道了~
PHP短网址生成系统短链接生成系统URL缩短器系统源码.zip
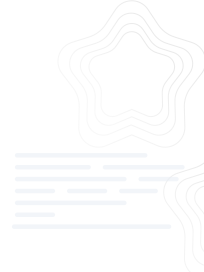
共2000个文件
php:1161个
md:229个
svg:173个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 108 浏览量
2024-07-15
17:28:36
上传
评论
收藏 51.19MB ZIP 举报
温馨提示
全新PHP短网址系统URL缩短器平台,它使您可以轻松地缩短链接, 根据受众群体的位置或平台来定位受众,并为缩短的链接提供分析见解。 系统使用了Laravel框架编写,前后台双语言使用,可以设置多域名,还可以开设套餐等诸多功能,值得使用。 注意的是系统是和谐版,并无其他限制!
资源推荐
资源详情
资源评论
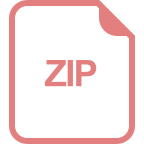
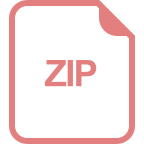
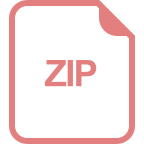
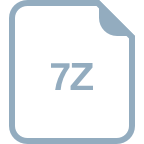
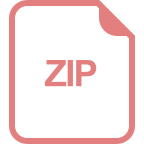
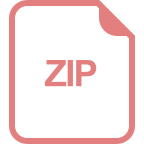
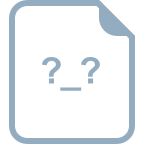
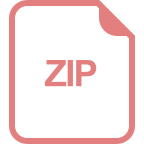
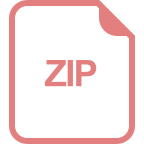
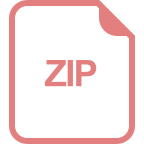
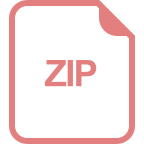
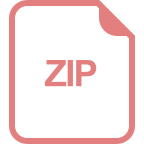
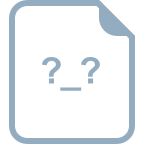
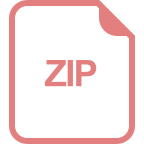
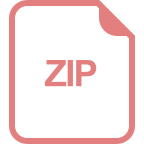
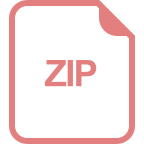
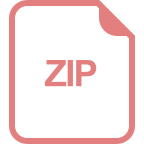
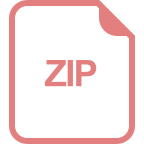
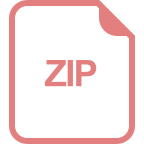
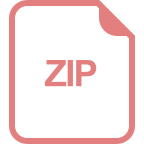
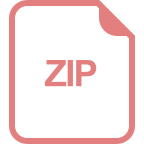
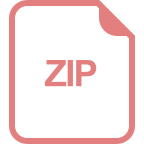
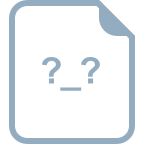
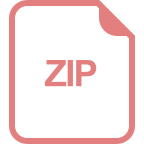
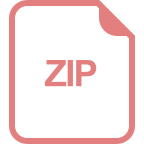
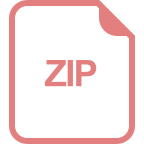
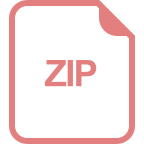
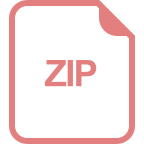
收起资源包目录

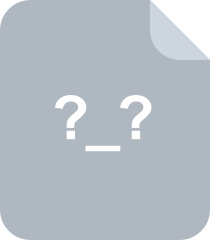
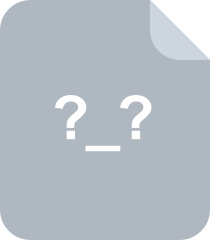
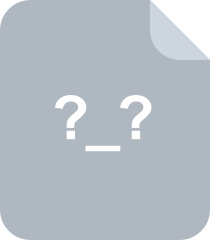
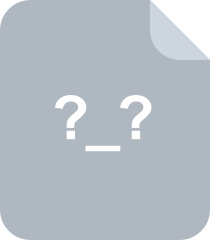
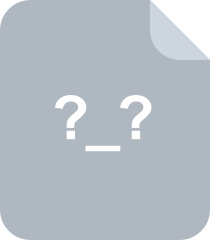
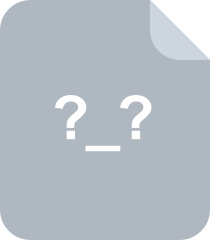
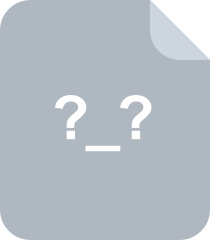
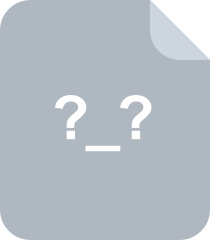
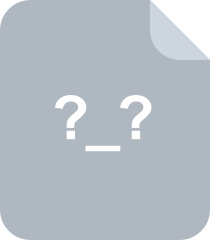
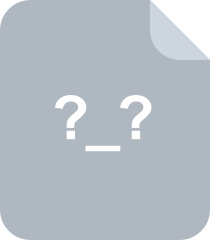
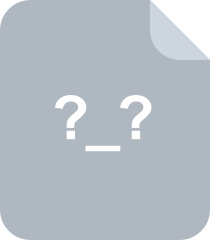
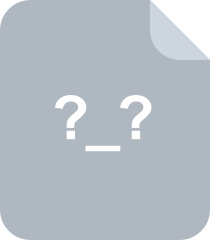
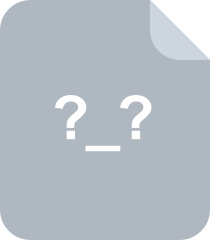
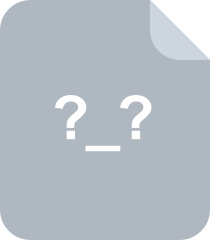
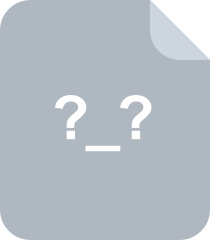
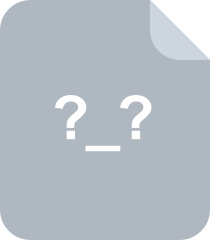
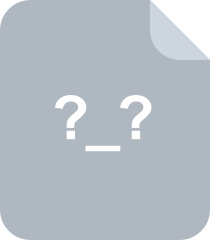
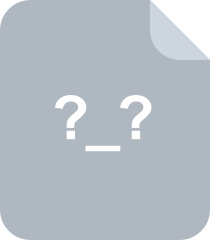
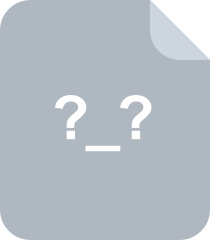
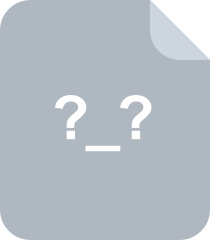
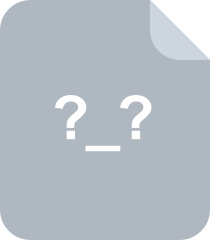
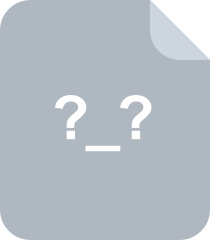
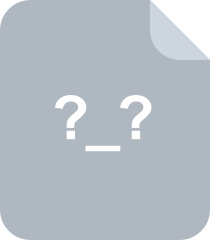
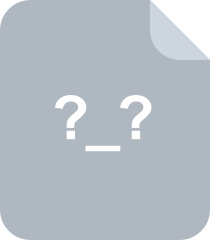
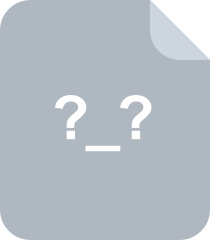
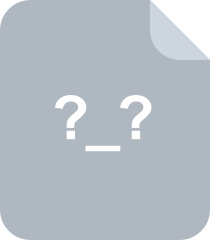
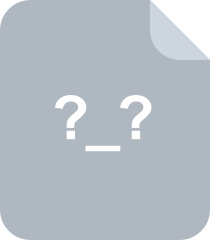
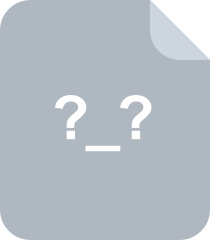
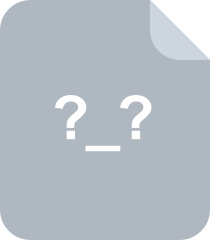
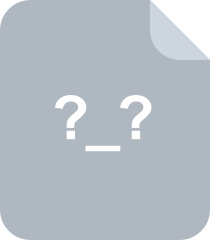
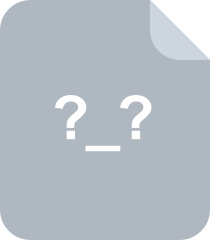
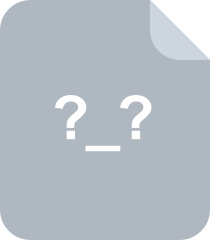
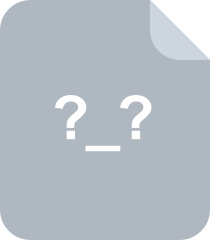
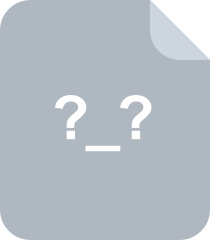
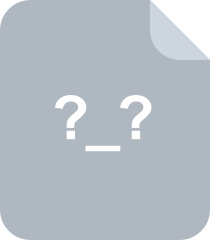
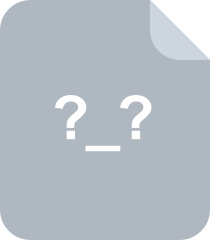
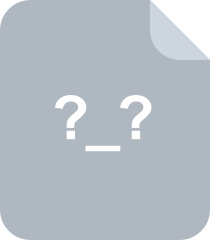
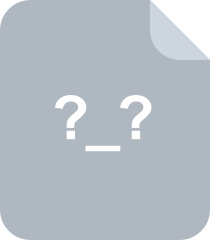
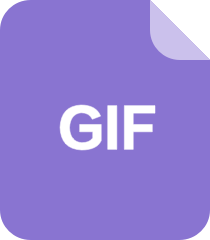
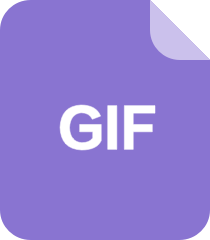
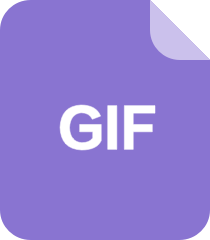
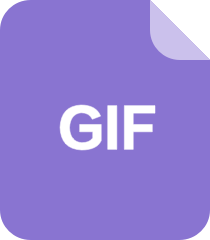
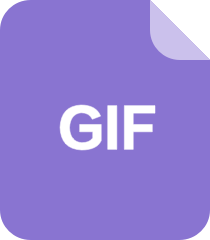
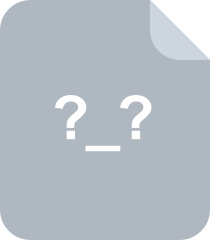
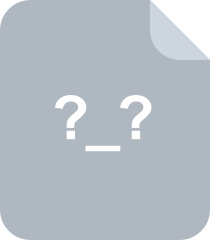
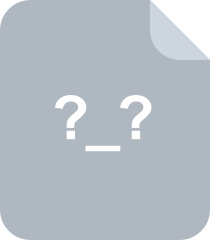
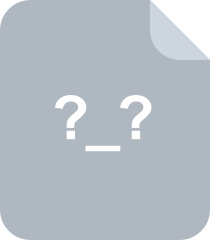
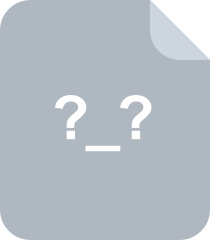
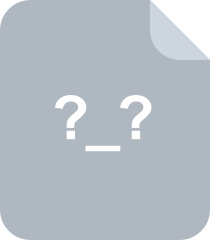
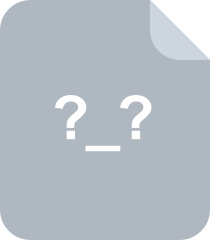
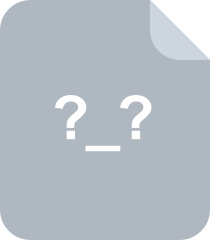
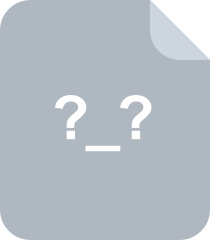
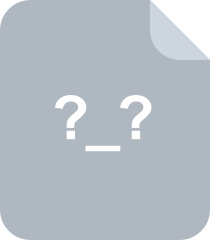
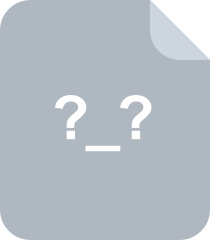
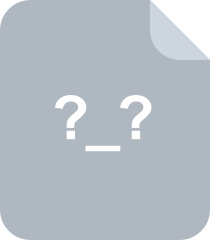
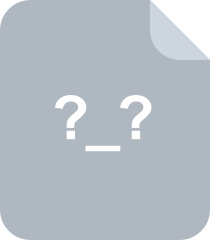
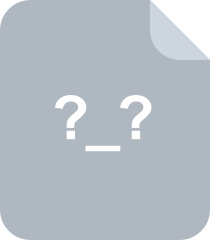
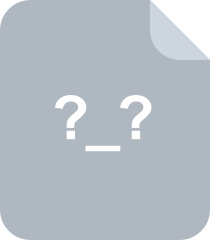
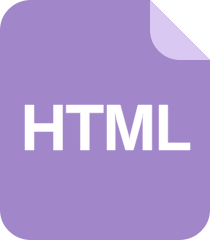
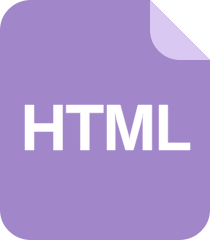
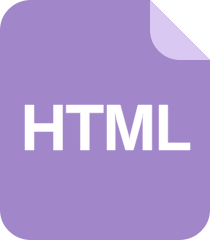
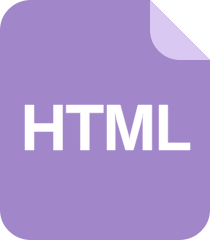
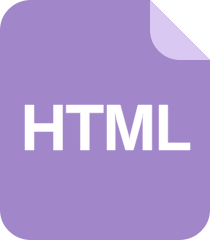
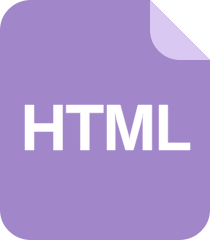
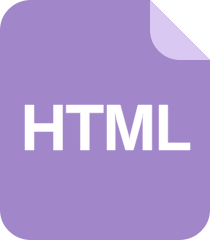
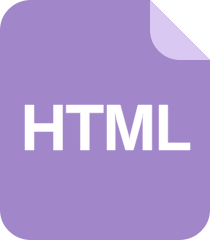
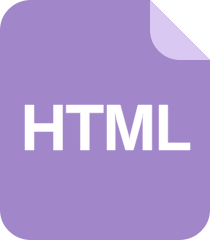
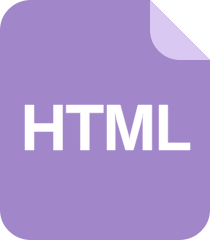
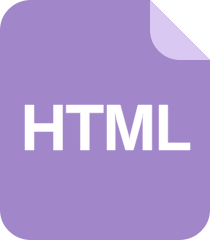
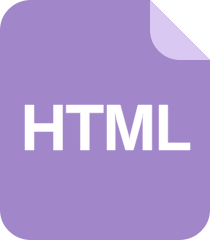
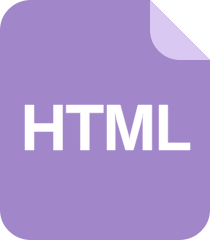
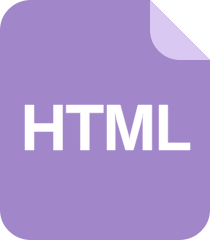
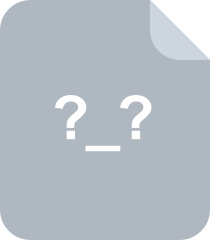
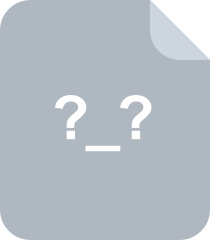
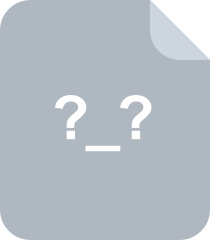
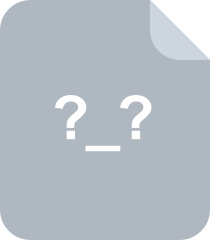
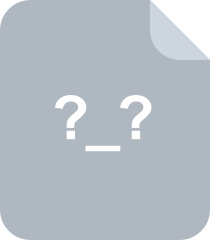
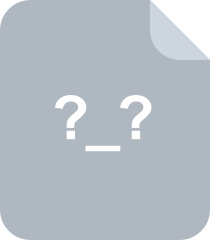
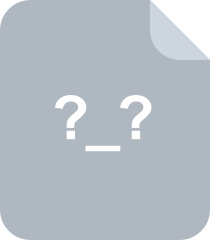
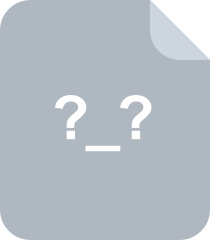
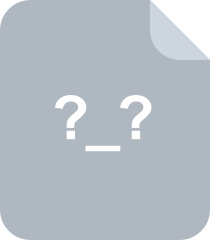
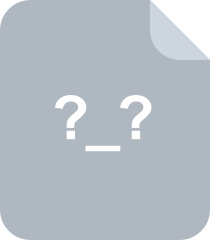
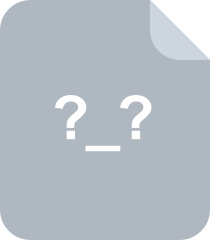
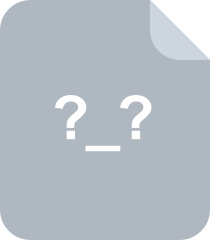
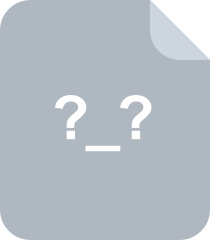
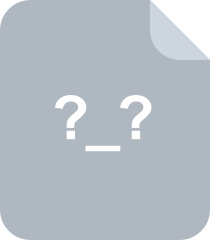
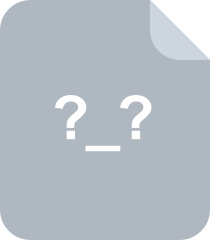
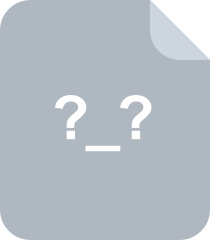
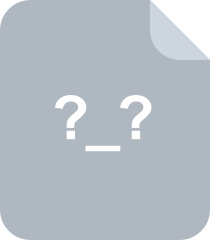
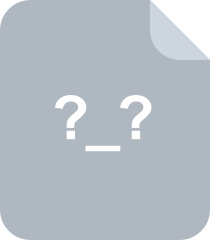
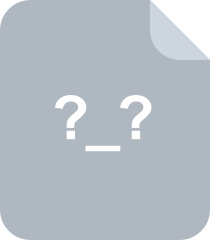
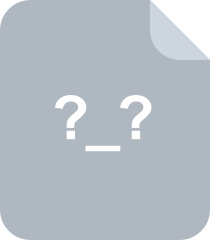
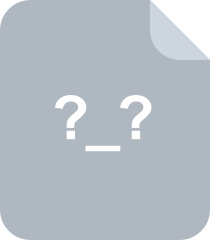
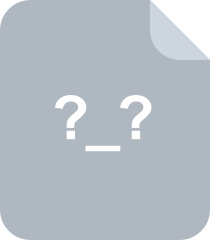
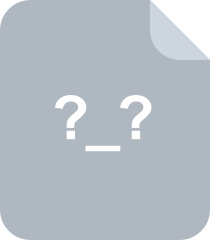
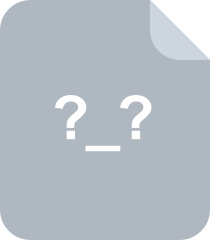
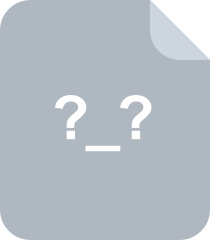
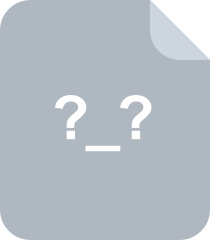
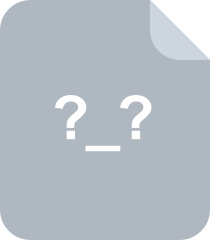
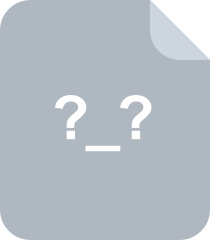
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
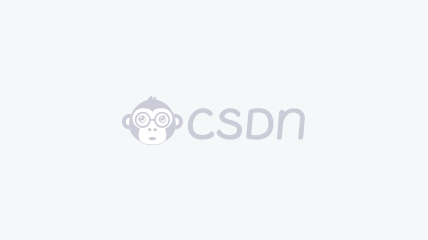

智慧浩海
- 粉丝: 1w+
- 资源: 5442

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

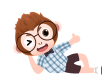
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


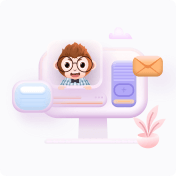
安全验证
文档复制为VIP权益,开通VIP直接复制
