# Sortable [](https://circleci.com/gh/SortableJS/Sortable) [](https://deepscan.io/dashboard#view=project&tid=3901&pid=5666&bid=43977) [](https://www.jsdelivr.com/package/npm/sortablejs) [](https://www.npmjs.com/package/sortablejs)
Sortable is a JavaScript library for reorderable drag-and-drop lists.
Demo: http://sortablejs.github.io/Sortable/
[<img width="250px" src="https://raw.githubusercontent.com/SortableJS/Sortable/HEAD/st/saucelabs.svg?sanitize=true">](https://saucelabs.com/)
## Features
* Supports touch devices and [modern](http://caniuse.com/#search=drag) browsers (including IE9)
* Can drag from one list to another or within the same list
* CSS animation when moving items
* Supports drag handles *and selectable text* (better than voidberg's html5sortable)
* Smart auto-scrolling
* Advanced swap detection
* Smooth animations
* [Multi-drag](https://github.com/SortableJS/Sortable/tree/master/plugins/MultiDrag) support
* Support for CSS transforms
* Built using native HTML5 drag and drop API
* Supports
* [Meteor](https://github.com/SortableJS/meteor-sortablejs)
* Angular
* [2.0+](https://github.com/SortableJS/angular-sortablejs)
* [1.*](https://github.com/SortableJS/angular-legacy-sortablejs)
* React
* [ES2015+](https://github.com/SortableJS/react-sortablejs)
* [Mixin](https://github.com/SortableJS/react-mixin-sortablejs)
* [Knockout](https://github.com/SortableJS/knockout-sortablejs)
* [Polymer](https://github.com/SortableJS/polymer-sortablejs)
* [Vue](https://github.com/SortableJS/Vue.Draggable)
* [Ember](https://github.com/SortableJS/ember-sortablejs)
* Supports any CSS library, e.g. [Bootstrap](#bs)
* Simple API
* Support for [plugins](#plugins)
* [CDN](#cdn)
* No jQuery required (but there is [support](https://github.com/SortableJS/jquery-sortablejs))
<br/>
### Articles
* [Dragging Multiple Items in Sortable](https://github.com/SortableJS/Sortable/wiki/Dragging-Multiple-Items-in-Sortable) (April 26, 2019)
* [Swap Thresholds and Direction](https://github.com/SortableJS/Sortable/wiki/Swap-Thresholds-and-Direction) (December 2, 2018)
* [Sortable v1.0 — New capabilities](https://github.com/SortableJS/Sortable/wiki/Sortable-v1.0-—-New-capabilities/) (December 22, 2014)
* [Sorting with the help of HTML5 Drag'n'Drop API](https://github.com/SortableJS/Sortable/wiki/Sorting-with-the-help-of-HTML5-Drag'n'Drop-API/) (December 23, 2013)
<br/>
### Getting Started
Install with NPM:
```bash
$ npm install sortablejs --save
```
Install with Bower:
```bash
$ bower install --save sortablejs
```
Import into your project:
```js
// Default SortableJS
import Sortable from 'sortablejs';
// Core SortableJS (without default plugins)
import Sortable from 'sortablejs/modular/sortable.core.esm.js';
// Complete SortableJS (with all plugins)
import Sortable from 'sortablejs/modular/sortable.complete.esm.js';
```
Cherrypick plugins:
```js
// Cherrypick extra plugins
import Sortable, { MultiDrag, Swap } from 'sortablejs';
Sortable.mount(new MultiDrag(), new Swap());
// Cherrypick default plugins
import Sortable, { AutoScroll } from 'sortablejs/modular/sortable.core.esm.js';
Sortable.mount(new AutoScroll());
```
---
### Usage
```html
<ul id="items">
<li>item 1</li>
<li>item 2</li>
<li>item 3</li>
</ul>
```
```js
var el = document.getElementById('items');
var sortable = Sortable.create(el);
```
You can use any element for the list and its elements, not just `ul`/`li`. Here is an [example with `div`s](https://jsbin.com/visimub/edit?html,js,output).
---
### Options
```js
var sortable = new Sortable(el, {
group: "name", // or { name: "...", pull: [true, false, 'clone', array], put: [true, false, array] }
sort: true, // sorting inside list
delay: 0, // time in milliseconds to define when the sorting should start
delayOnTouchOnly: false, // only delay if user is using touch
touchStartThreshold: 0, // px, how many pixels the point should move before cancelling a delayed drag event
disabled: false, // Disables the sortable if set to true.
store: null, // @see Store
animation: 150, // ms, animation speed moving items when sorting, `0` — without animation
easing: "cubic-bezier(1, 0, 0, 1)", // Easing for animation. Defaults to null. See https://easings.net/ for examples.
handle: ".my-handle", // Drag handle selector within list items
filter: ".ignore-elements", // Selectors that do not lead to dragging (String or Function)
preventOnFilter: true, // Call `event.preventDefault()` when triggered `filter`
draggable: ".item", // Specifies which items inside the element should be draggable
dataIdAttr: 'data-id',
ghostClass: "sortable-ghost", // Class name for the drop placeholder
chosenClass: "sortable-chosen", // Class name for the chosen item
dragClass: "sortable-drag", // Class name for the dragging item
swapThreshold: 1, // Threshold of the swap zone
invertSwap: false, // Will always use inverted swap zone if set to true
invertedSwapThreshold: 1, // Threshold of the inverted swap zone (will be set to swapThreshold value by default)
direction: 'horizontal', // Direction of Sortable (will be detected automatically if not given)
forceFallback: false, // ignore the HTML5 DnD behaviour and force the fallback to kick in
fallbackClass: "sortable-fallback", // Class name for the cloned DOM Element when using forceFallback
fallbackOnBody: false, // Appends the cloned DOM Element into the Document's Body
fallbackTolerance: 0, // Specify in pixels how far the mouse should move before it's considered as a drag.
dragoverBubble: false,
removeCloneOnHide: true, // Remove the clone element when it is not showing, rather than just hiding it
emptyInsertThreshold: 5, // px, distance mouse must be from empty sortable to insert drag element into it
setData: function (/** DataTransfer */dataTransfer, /** HTMLElement*/dragEl) {
dataTransfer.setData('Text', dragEl.textContent); // `dataTransfer` object of HTML5 DragEvent
},
// Element is chosen
onChoose: function (/**Event*/evt) {
evt.oldIndex; // element index within parent
},
// Element is unchosen
onUnchoose: function(/**Event*/evt) {
// same properties as onEnd
},
// Element dragging started
onStart: function (/**Event*/evt) {
evt.oldIndex; // element index within parent
},
// Element dragging ended
onEnd: function (/**Event*/evt) {
var itemEl = evt.item; // dragged HTMLElement
evt.to; // target list
evt.from; // previous list
evt.oldIndex; // element's old index within old parent
evt.newIndex; // element's new index within new parent
evt.oldDraggableIndex; // element's old index within old parent, only counting draggable elements
evt.newDraggableIndex; // element's new index within new parent, only counting draggable elements
evt.clone // the clone element
evt.pullMode; // when item is in another sortable: `"clone"` if cloning, `true` if moving
},
// Element is dropped into the list from another list
onAdd: function (/**Event*/evt) {
// same properties as onEnd
},
// Changed sorting within list
onUpdate: function (/**Event*/evt) {
// same properties as onEnd
},
// Called by any change to the list (add / update / remove)
onSort: function (/**Event*/evt) {
// same properties as onEnd
},
// Element is removed from the list into another list
onRemove: function (/**Event*/evt) {
// same properties as onEnd
},
// Attempt to drag a filtered element
onFilter: function (/**Event*/evt) {
var itemEl = evt.item; // HTMLElement receiving the `mousedown|tapstart` event.
},
// Event when you move an item in the list or between lists
onMove: function
没有合适的资源?快使用搜索试试~ 我知道了~
类似抖音小剧场短剧影视微信小程序源码 自带支付收益带会员模式用户单独购买 全开源.zip
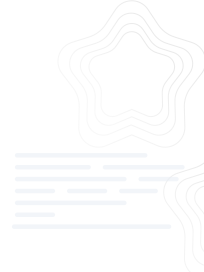
共2002个文件
js:1224个
json:216个
html:197个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉

温馨提示
经典版仿抖音滑动效果小剧场短剧影视小程序源码,带支付收益等模式、支持无限滑动;高性能滑动、预加载、视频预览,支持剧情介绍,集合壁纸; 支持会员模式,支持用户单独购买等等多功能。 丰富的后台设置,具体大家可以自行下载搭建研究。 前端支持抖音、快手、百度、H5 和微信小程序,需要哪个端就发行到哪个端即可。
资源推荐
资源详情
资源评论
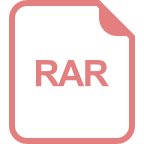
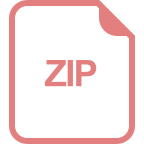
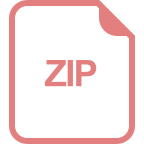
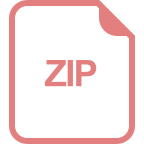
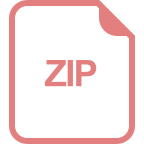
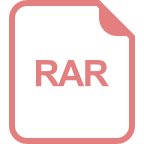
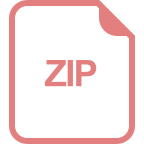
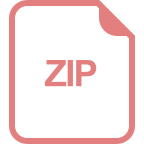
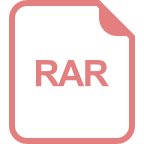
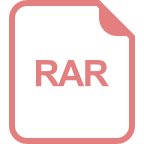
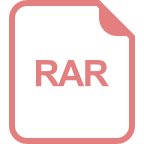
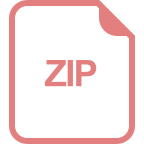
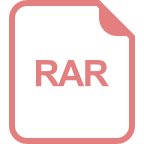
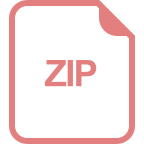
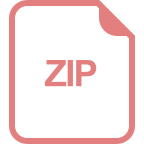
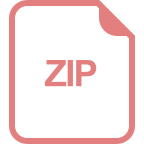
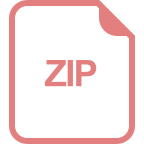
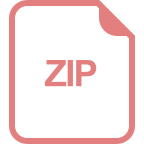
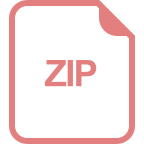
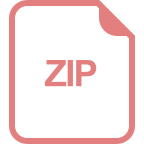
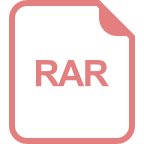
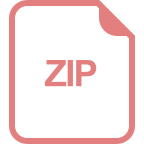
收起资源包目录

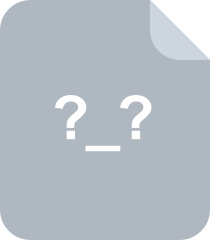
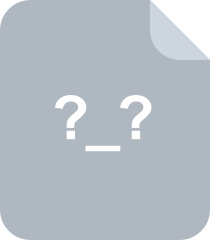
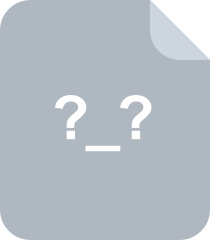
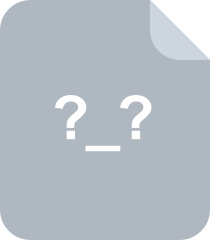
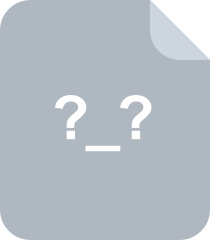
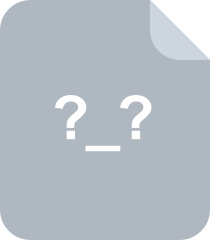
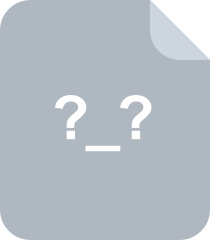
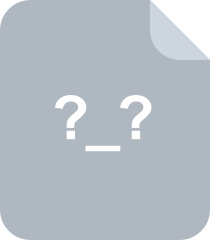
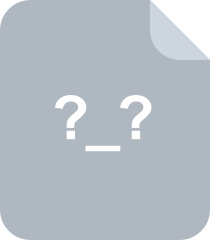
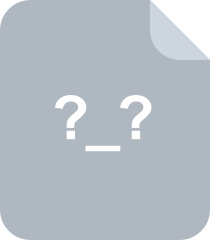
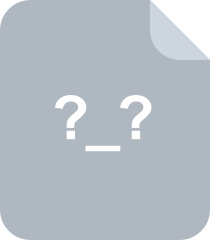
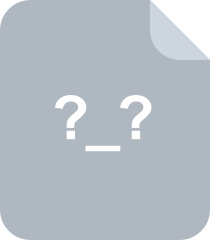
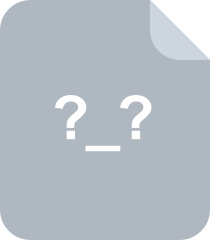
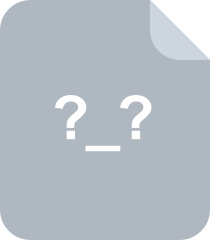
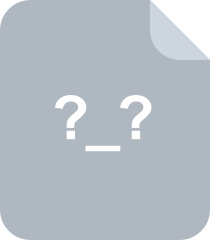
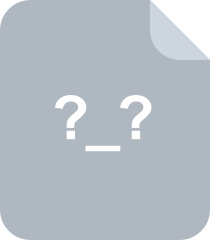
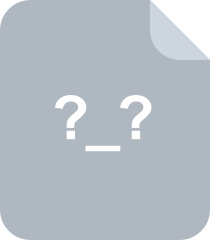
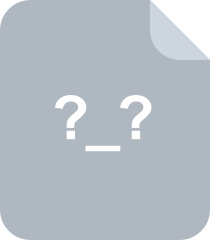
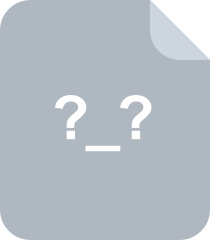
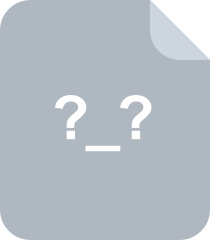
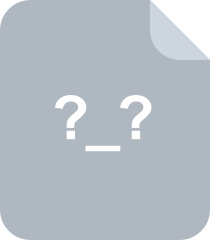
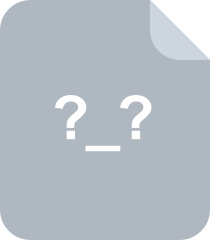
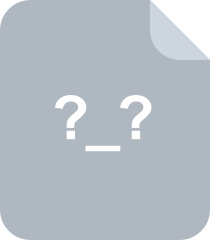
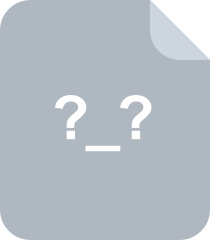
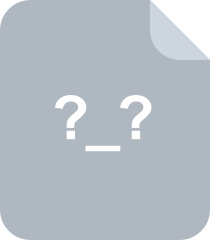
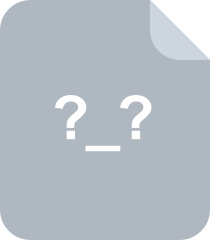
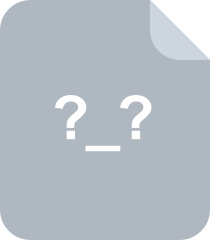
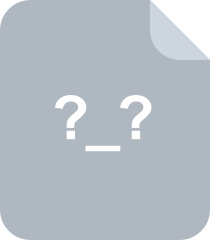
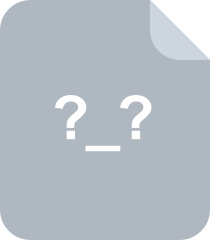
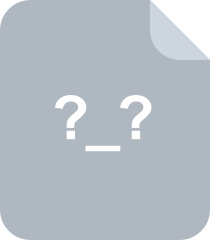
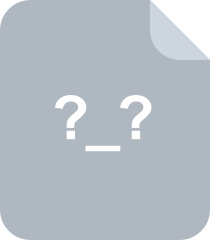
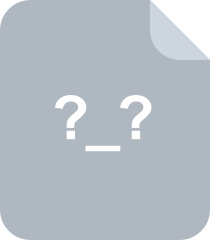
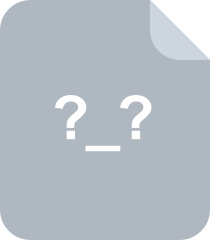
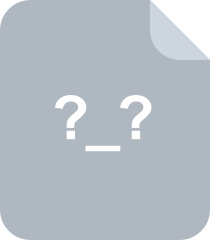
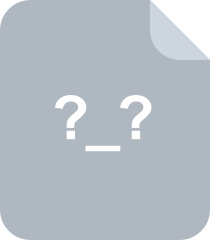
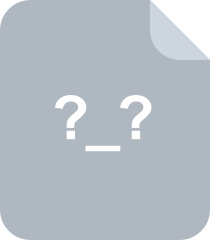
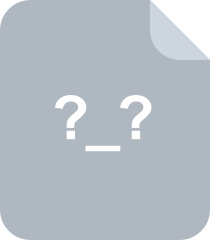
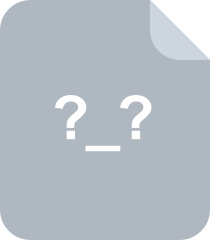
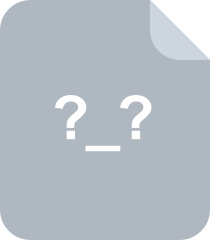
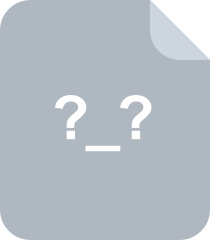
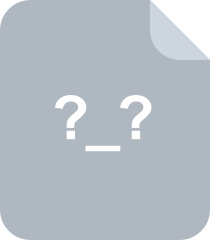
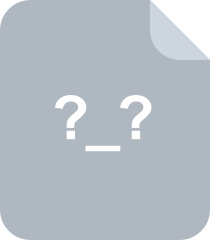
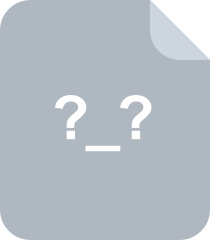
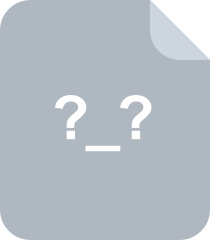
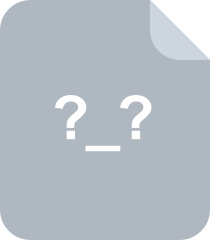
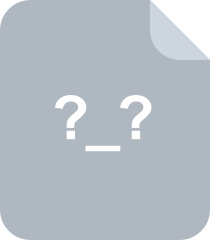
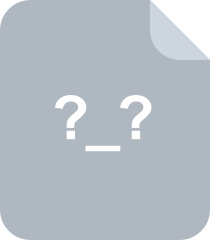
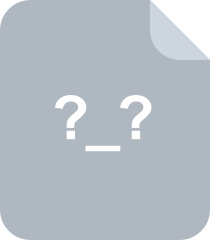
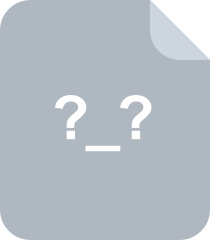
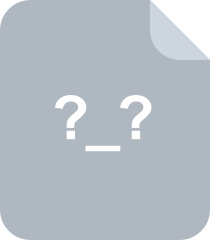
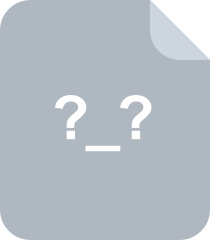
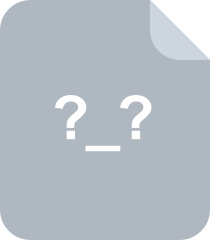
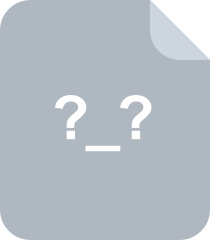
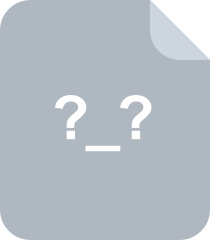
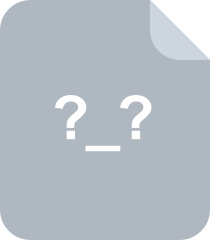
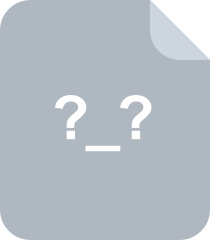
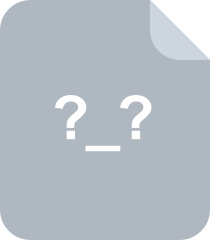
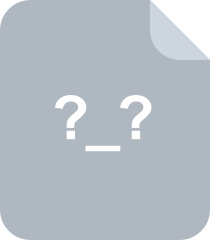
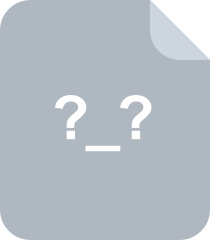
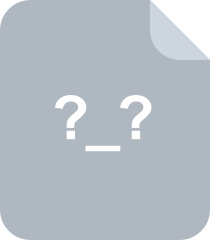
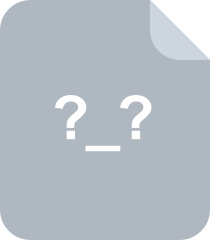
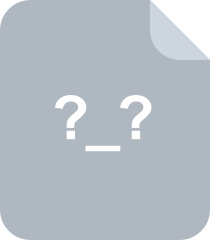
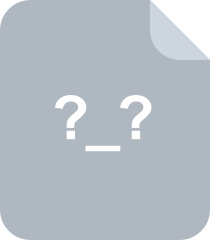
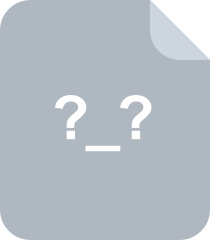
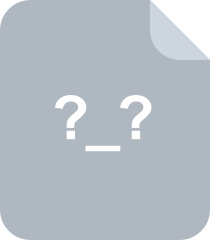
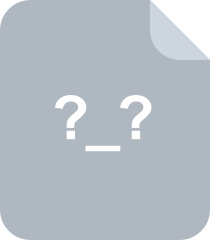
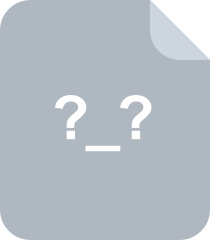
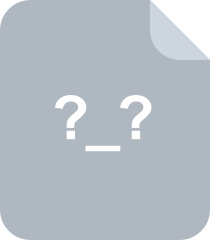
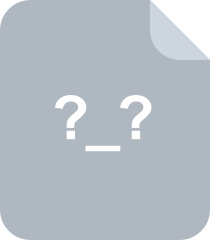
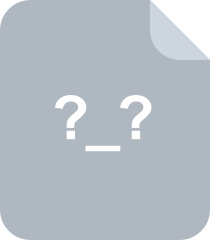
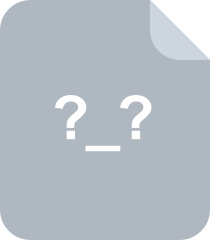
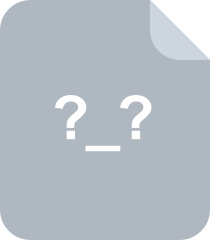
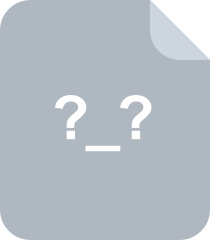
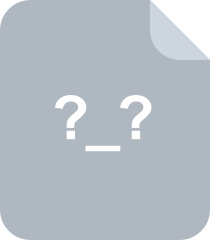
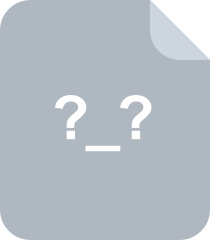
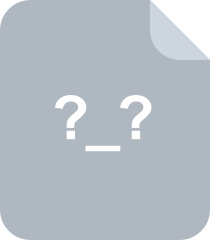
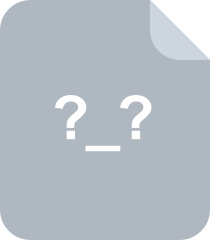
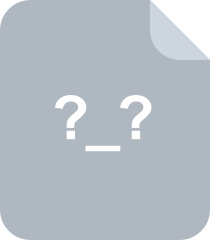
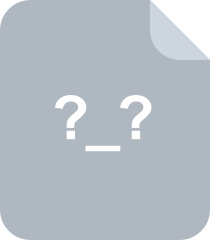
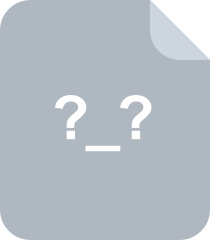
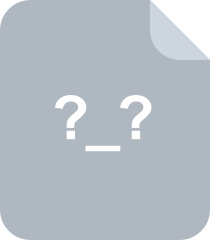
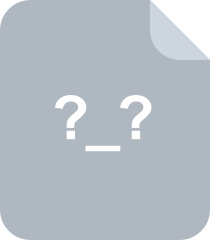
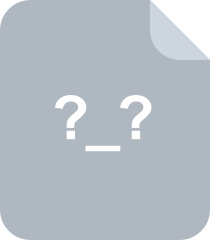
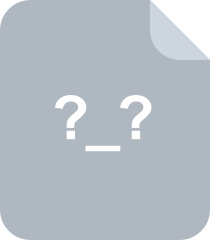
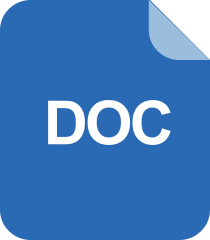
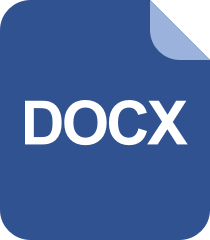
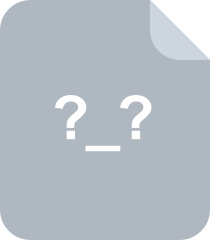
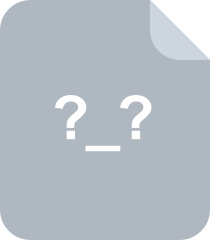
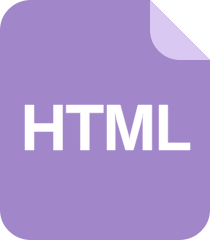
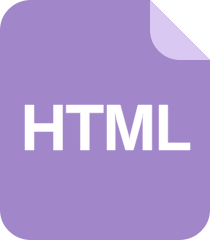
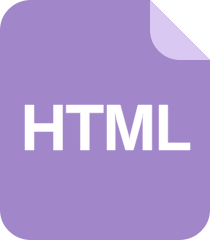
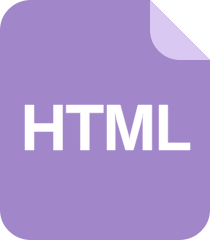
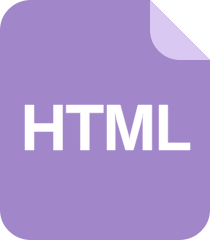
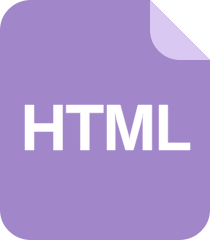
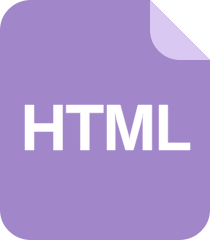
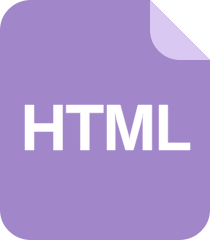
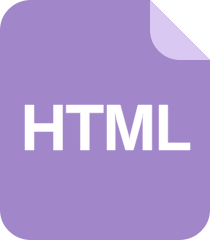
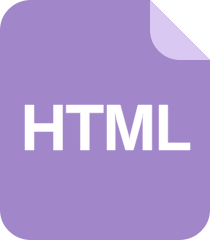
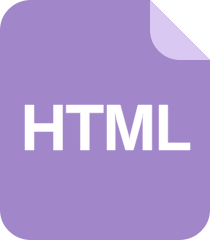
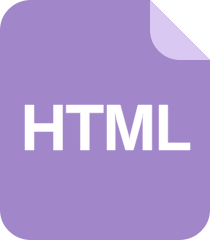
共 2002 条
- 1
- 2
- 3
- 4
- 5
- 6
- 21
资源评论
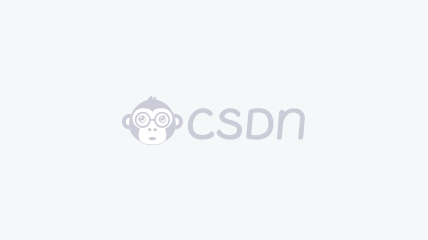
- 阿酷网络信息技术有限公司2023-10-29感谢大佬分享的资源给了我灵感,果断支持!感谢分享~

智慧浩海
- 粉丝: 1w+
- 资源: 5442
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

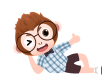
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


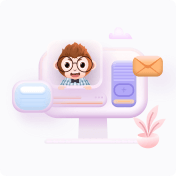
安全验证
文档复制为VIP权益,开通VIP直接复制
