/*
* This file is part of Pimple.
*
* Copyright (c) 2014 Fabien Potencier
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is furnished
* to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
#ifdef HAVE_CONFIG_H
#include "config.h"
#endif
#include "php.h"
#include "php_ini.h"
#include "ext/standard/info.h"
#include "php_pimple.h"
#include "pimple_compat.h"
#include "zend_interfaces.h"
#include "zend.h"
#include "Zend/zend_closures.h"
#include "ext/spl/spl_exceptions.h"
#include "Zend/zend_exceptions.h"
#include "main/php_output.h"
#include "SAPI.h"
static zend_class_entry *pimple_ce_PsrContainerInterface;
static zend_class_entry *pimple_ce_PsrContainerExceptionInterface;
static zend_class_entry *pimple_ce_PsrNotFoundExceptionInterface;
static zend_class_entry *pimple_ce_ExpectedInvokableException;
static zend_class_entry *pimple_ce_FrozenServiceException;
static zend_class_entry *pimple_ce_InvalidServiceIdentifierException;
static zend_class_entry *pimple_ce_UnknownIdentifierException;
static zend_class_entry *pimple_ce;
static zend_object_handlers pimple_object_handlers;
static zend_class_entry *pimple_closure_ce;
static zend_class_entry *pimple_serviceprovider_ce;
static zend_object_handlers pimple_closure_object_handlers;
static zend_internal_function pimple_closure_invoker_function;
#define FETCH_DIM_HANDLERS_VARS pimple_object *pimple_obj = NULL; \
ulong index; \
pimple_obj = (pimple_object *)zend_object_store_get_object(object TSRMLS_CC); \
#define PIMPLE_OBJECT_HANDLE_INHERITANCE_OBJECT_HANDLERS do { \
if (ce != pimple_ce) { \
zend_hash_find(&ce->function_table, ZEND_STRS("offsetget"), (void **)&function); \
if (function->common.scope != ce) { /* if the function is not defined in this actual class */ \
pimple_object_handlers.read_dimension = pimple_object_read_dimension; /* then overwrite the handler to use custom one */ \
} \
zend_hash_find(&ce->function_table, ZEND_STRS("offsetset"), (void **)&function); \
if (function->common.scope != ce) { \
pimple_object_handlers.write_dimension = pimple_object_write_dimension; \
} \
zend_hash_find(&ce->function_table, ZEND_STRS("offsetexists"), (void **)&function); \
if (function->common.scope != ce) { \
pimple_object_handlers.has_dimension = pimple_object_has_dimension; \
} \
zend_hash_find(&ce->function_table, ZEND_STRS("offsetunset"), (void **)&function); \
if (function->common.scope != ce) { \
pimple_object_handlers.unset_dimension = pimple_object_unset_dimension; \
} \
} else { \
pimple_object_handlers.read_dimension = pimple_object_read_dimension; \
pimple_object_handlers.write_dimension = pimple_object_write_dimension; \
pimple_object_handlers.has_dimension = pimple_object_has_dimension; \
pimple_object_handlers.unset_dimension = pimple_object_unset_dimension; \
}\
} while(0);
#define PIMPLE_CALL_CB do { \
zend_fcall_info_argn(&fci TSRMLS_CC, 1, &object); \
fci.size = sizeof(fci); \
fci.object_ptr = retval->fcc.object_ptr; \
fci.function_name = retval->value; \
fci.no_separation = 1; \
fci.retval_ptr_ptr = &retval_ptr_ptr; \
\
zend_call_function(&fci, &retval->fcc TSRMLS_CC); \
efree(fci.params); \
if (EG(exception)) { \
return EG(uninitialized_zval_ptr); \
} \
} while(0);
/* Psr\Container\ContainerInterface */
ZEND_BEGIN_ARG_INFO_EX(arginfo_pimple_PsrContainerInterface_get, 0, 0, 1)
ZEND_ARG_INFO(0, id)
ZEND_END_ARG_INFO()
ZEND_BEGIN_ARG_INFO_EX(arginfo_pimple_PsrContainerInterface_has, 0, 0, 1)
ZEND_ARG_INFO(0, id)
ZEND_END_ARG_INFO()
static const zend_function_entry pimple_ce_PsrContainerInterface_functions[] = {
PHP_ABSTRACT_ME(ContainerInterface, get, arginfo_pimple_PsrContainerInterface_get)
PHP_ABSTRACT_ME(ContainerInterface, has, arginfo_pimple_PsrContainerInterface_has)
PHP_FE_END
};
/* Psr\Container\ContainerExceptionInterface */
static const zend_function_entry pimple_ce_PsrContainerExceptionInterface_functions[] = {
PHP_FE_END
};
/* Psr\Container\NotFoundExceptionInterface */
static const zend_function_entry pimple_ce_PsrNotFoundExceptionInterface_functions[] = {
PHP_FE_END
};
/* Pimple\Exception\FrozenServiceException */
ZEND_BEGIN_ARG_INFO_EX(arginfo_FrozenServiceException___construct, 0, 0, 1)
ZEND_ARG_INFO(0, id)
ZEND_END_ARG_INFO()
static const zend_function_entry pimple_ce_FrozenServiceException_functions[] = {
PHP_ME(FrozenServiceException, __construct, arginfo_FrozenServiceException___construct, ZEND_ACC_PUBLIC)
PHP_FE_END
};
/* Pimple\Exception\InvalidServiceIdentifierException */
ZEND_BEGIN_ARG_INFO_EX(arginfo_InvalidServiceIdentifierException___construct, 0, 0, 1)
ZEND_ARG_INFO(0, id)
ZEND_END_ARG_INFO()
static const zend_function_entry pimple_ce_InvalidServiceIdentifierException_functions[] = {
PHP_ME(InvalidServiceIdentifierException, __construct, arginfo_InvalidServiceIdentifierException___construct, ZEND_ACC_PUBLIC)
PHP_FE_END
};
/* Pimple\Exception\UnknownIdentifierException */
ZEND_BEGIN_ARG_INFO_EX(arginfo_UnknownIdentifierException___construct, 0, 0, 1)
ZEND_ARG_INFO(0, id)
ZEND_END_ARG_INFO()
static const zend_function_entry pimple_ce_UnknownIdentifierException_functions[] = {
PHP_ME(UnknownIdentifierException, __construct, arginfo_UnknownIdentifierException___construct, ZEND_ACC_PUBLIC)
PHP_FE_END
};
/* Pimple\Container */
ZEND_BEGIN_ARG_INFO_EX(arginfo___construct, 0, 0, 0)
ZEND_ARG_ARRAY_INFO(0, value, 0)
ZEND_END_ARG_INFO()
ZEND_BEGIN_ARG_INFO_EX(arginfo_offsetset, 0, 0, 2)
ZEND_ARG_INFO(0, offset)
ZEND_ARG_INFO(0, value)
ZEND_END_ARG_INFO()
ZEND_BEGIN_ARG_INFO_EX(arginfo_offsetget, 0, 0, 1)
ZEND_ARG_INFO(0, offset)
ZEND_END_ARG_INFO()
ZEND_BEGIN_ARG_INFO_EX(arginfo_offsetexists, 0, 0, 1)
ZEND_ARG_INFO(0, offset)
ZEND_END_ARG_INFO()
ZEND_BEGIN_ARG_INFO_EX(arginfo_offsetunset, 0, 0, 1)
ZEND_ARG_INFO(0, offset)
ZEND_END_ARG_INFO()
ZEND_BEGIN_ARG_INFO_EX(arginfo_factory, 0, 0, 1)
ZEND_ARG_INFO(0, callable)
ZEND_END_ARG_INFO()
ZEND_BEGIN_ARG_INFO_EX(arginfo_protect, 0, 0, 1)
ZEND_ARG_INFO(0, callable)
ZEND_END_ARG_INFO()
ZEND_BEGIN_ARG_INFO_EX(arginfo_raw, 0, 0, 1)
ZEND_ARG_INFO(0, id)
ZEND_END_ARG_INFO()
ZEND_BEGIN_ARG_INFO_EX(arginfo_extend, 0, 0, 2)
ZEND_ARG_INFO(0, id)
ZEND_ARG_INFO(0, callable)
ZEND_END_ARG_INFO()
ZEND_BEGIN_ARG_INFO_EX(arginfo_keys, 0, 0, 0)
ZEND_END_ARG_INFO()
ZEND_BEGIN_ARG_INFO_EX(arginfo_register, 0, 0, 1)
ZEND_ARG_OBJ_INFO(0, provider, Pimple\\ServiceProviderInterface, 0)
ZEND_ARG_ARRAY_INFO(0, values, 1)
ZEND_END_ARG_INFO()
static const zend_function_entry pimple_ce_functions[] = {
PHP_ME(Pimple, __construct, arginfo___construct, ZEND_ACC_PUBLIC)
PHP_ME(Pimple, factory, arginfo_factory, ZEND_ACC_PUBLIC)
PHP_ME(Pimple, protect, arginfo_protect, ZEND_ACC_PUBLIC)
PHP_ME(Pimple, raw, arginfo_raw, ZEND_ACC_PUBLIC)
PHP_ME(Pimple, extend, arginfo_extend, ZEND_ACC_PUBLIC)
PHP_ME(Pimple, keys, arginfo_keys, ZEND_ACC_PUBLIC)
没有合适的资源?快使用搜索试试~ 我知道了~
php客服在线IM源码 网页在线客服软件代码.zip
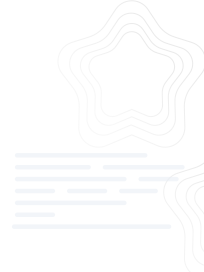
共6323个文件
php:2979个
png:1159个
js:418个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 78 浏览量
2023-01-16
14:21:30
上传
评论
收藏 26.39MB ZIP 举报
温馨提示
PHP在线客服系统源码是一款PHP开发的在线客服系统源码,网站在线客服系统,网页在线客服软件代码, 免费在线客服系统源码,支持多商家多客服,客服系统源码支持二开,客服同时支持手机移动端和PC网页客服。 php客服在线IM源码,支持自动刷新(网页即时接收消息)+自动回复+可生成接入+手机版管理后台:弹窗接入,微信公众号接入,网页链接接入。 目前第三方在线客服系统,基本都是需要年费(几千一年),受到运营方各种限制, 严重影响流量转化订单成交。本在线客服系统解决这个问题,可选择安装在你自己的服务器想怎么用都行! 不会搭建也没关系,本站站长配有安装搭建教程,轻松使用! 为了更好的发挥网站系统性能推荐Linux服务器 推荐配置2核4G 5M带宽
资源推荐
资源详情
资源评论
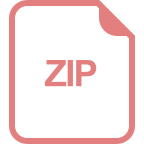
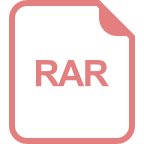
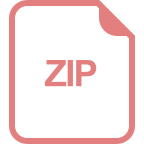
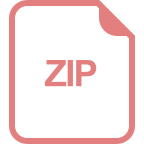
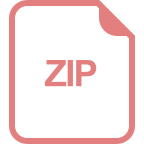
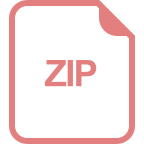
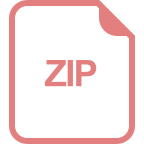
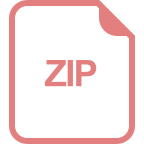
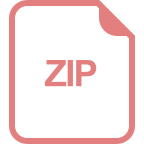
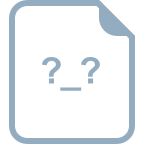
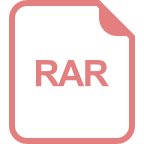
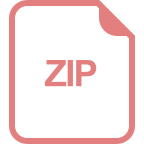
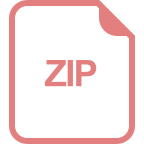
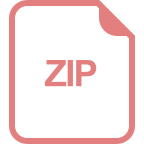
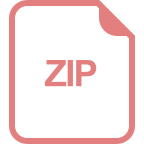
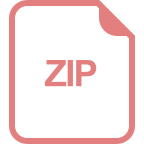
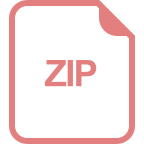
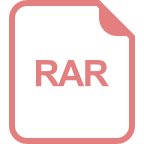
收起资源包目录

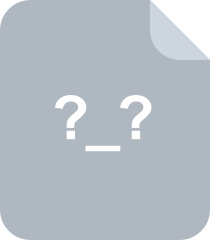
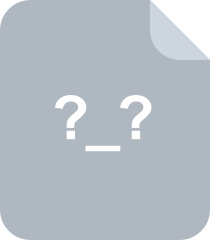
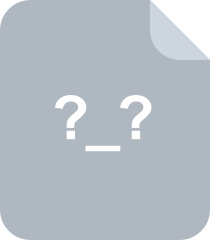
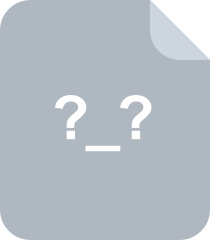
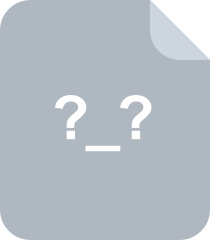
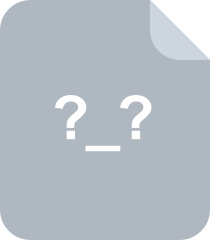
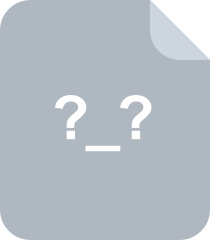
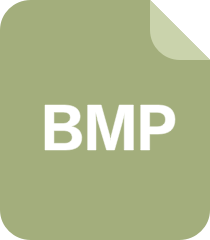
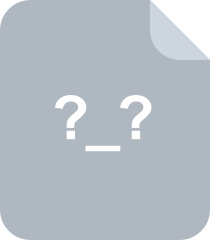
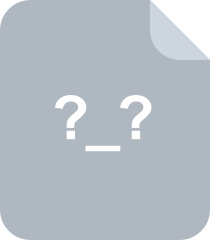
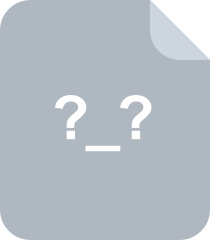
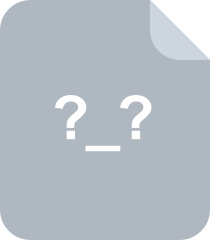
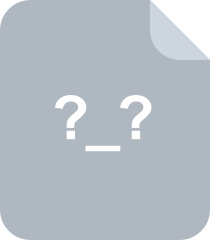
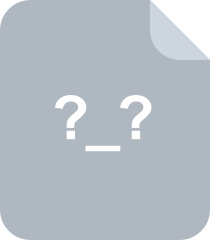
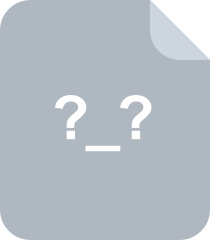
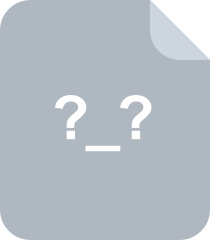
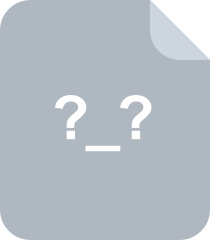
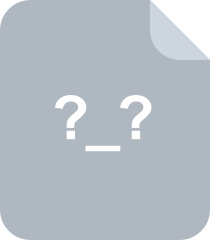
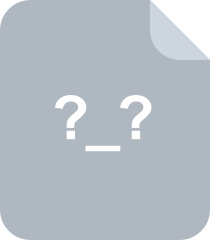
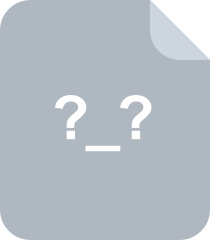
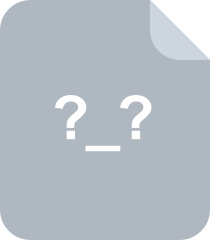
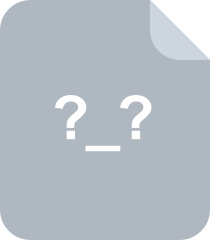
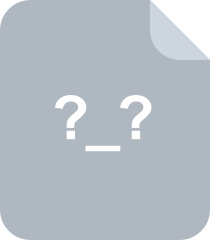
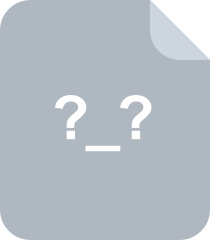
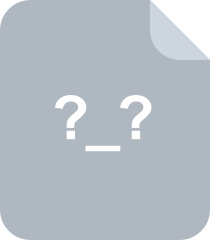
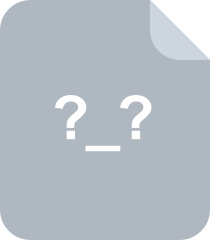
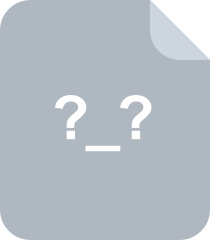
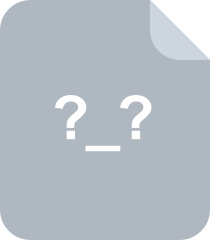
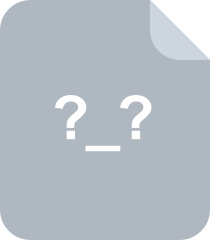
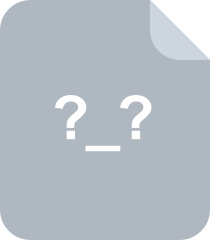
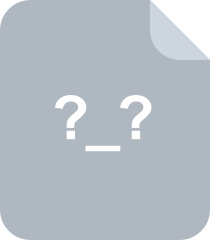
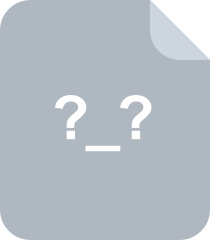
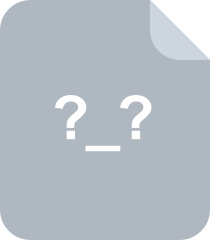
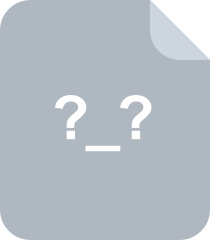
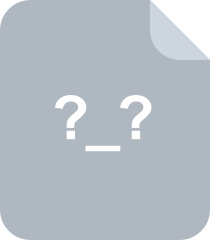
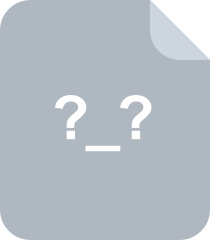
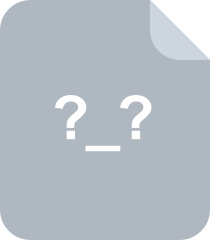
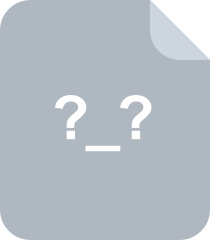
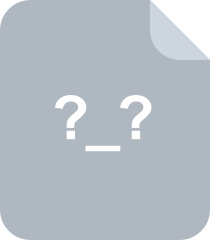
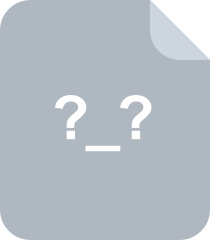
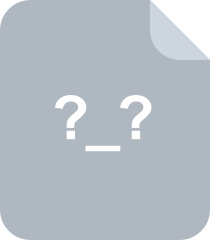
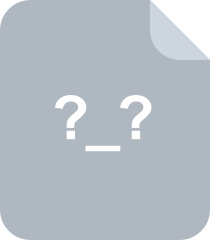
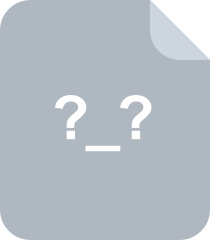
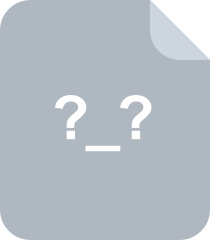
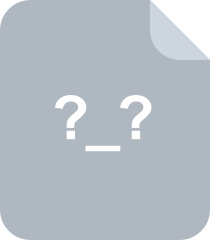
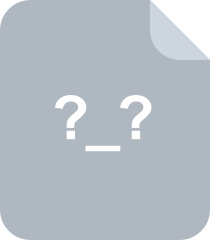
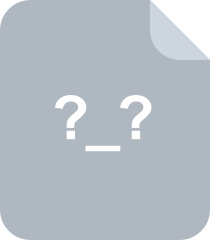
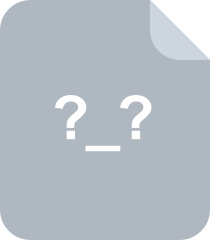
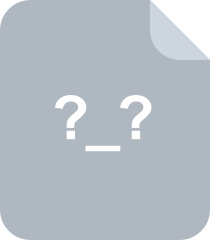
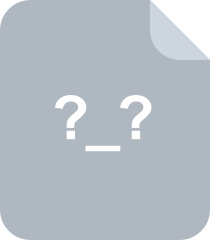
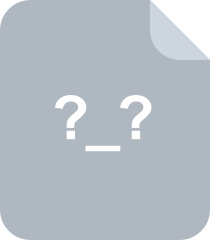
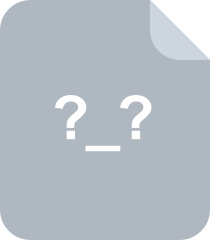
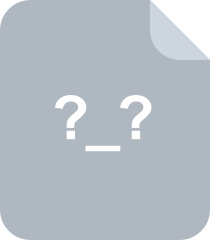
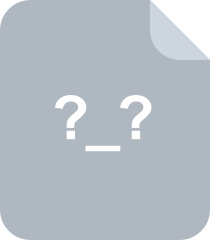
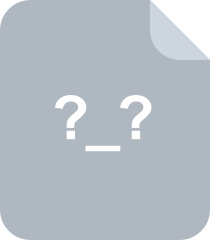
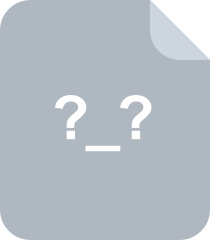
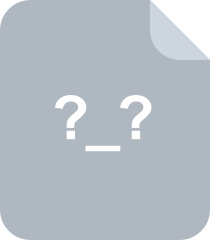
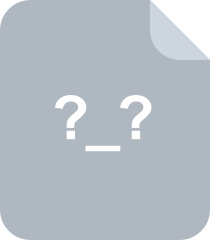
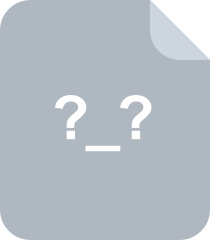
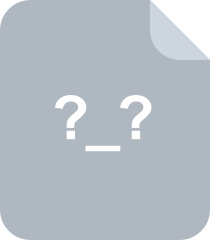
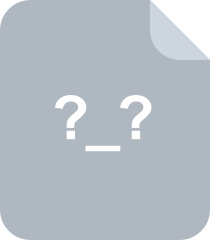
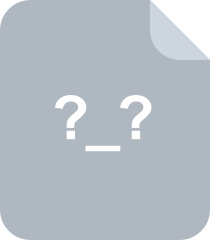
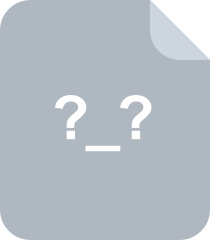
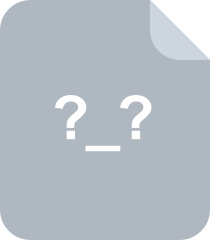
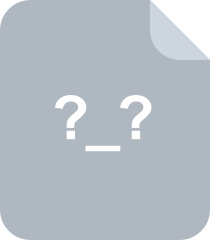
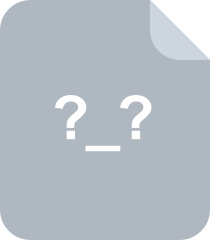
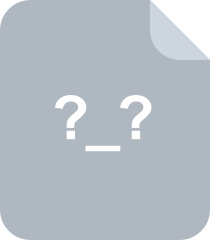
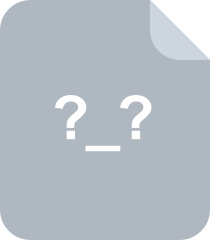
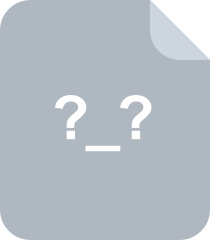
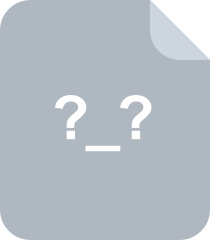
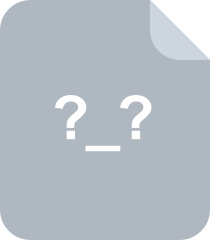
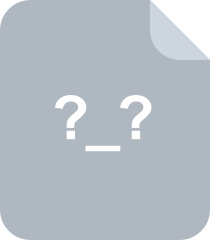
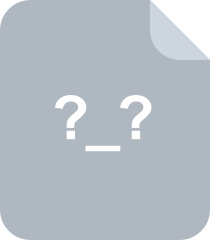
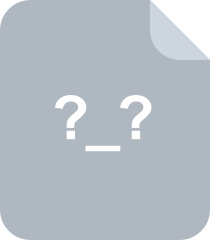
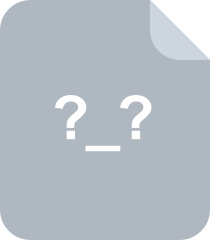
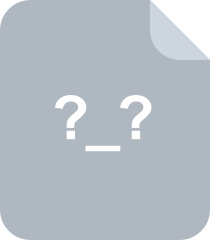
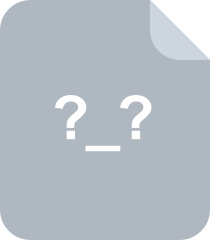
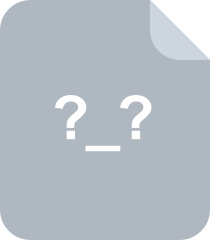
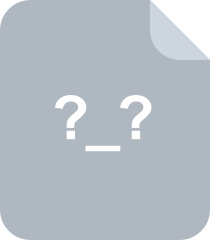
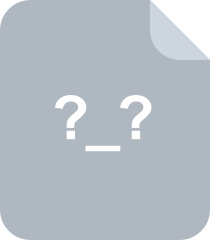
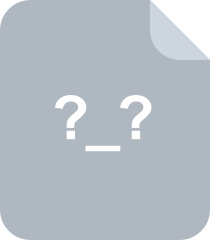
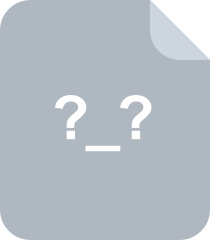
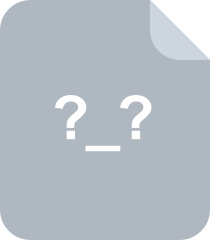
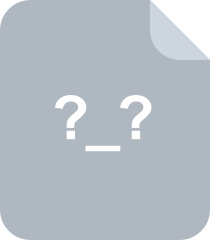
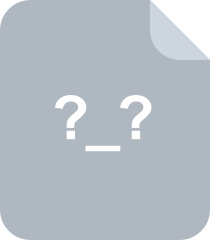
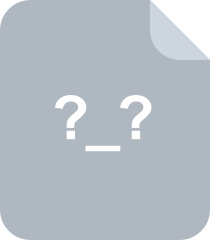
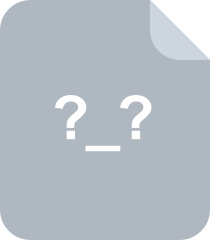
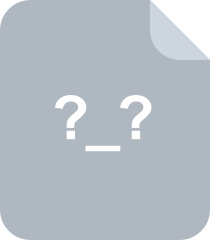
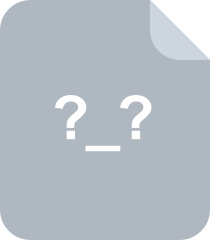
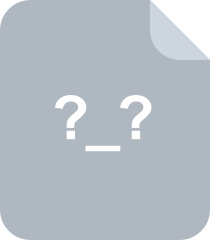
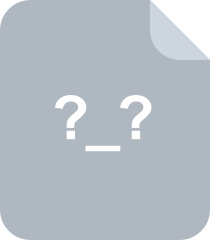
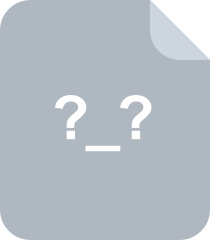
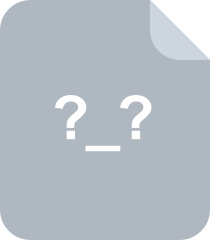
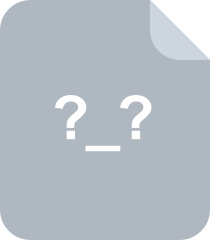
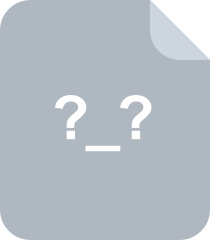
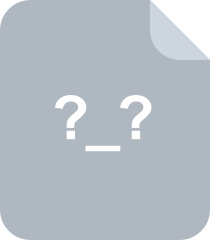
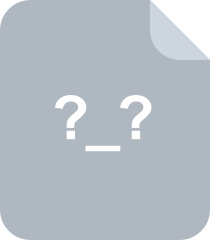
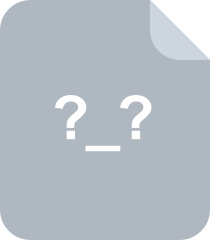
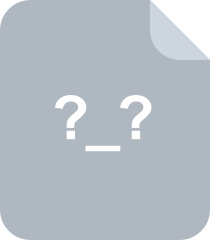
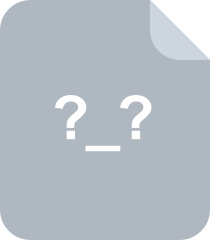
共 6323 条
- 1
- 2
- 3
- 4
- 5
- 6
- 64
资源评论
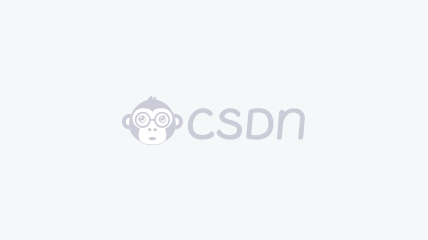

智慧浩海
- 粉丝: 1w+
- 资源: 5459

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

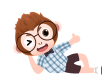
最新资源
- 俞敏洪:企业的文化基因需要一开始就注入.docx
- 政钧企业文化诊断学之 :企业家个人文化力不足.docx
- STM32串口下载软件(FLYMCU)
- 红枣疏花机(含工程图sw18可编辑+cad)全套技术开发资料100%好用.zip
- 基于web的网上演唱会票务管理系统.doc
- Python面向对象.xmind
- 中职学校《Windows Server网络操作系统》课程标准及教学指导(2024年版)
- 基于java的物流信息网的设计与实现论文.doc
- 中职学校《Java程序设计》课程标准及教学指导(2024年版)
- Python面向对象进阶.xmind
- 中职学校《Android Studio程序设计》课程标准及教学指导(2024年版)
- ReST-MCTS∗: LLM Self-Training via Process Reward Guided Tree Search
- 基于java的音乐交流平台论文.doc
- pptssssssss
- 中职学校《物联网网关Qt程序编写》课程标准及教学指导(2024年版)
- 基于web的银行业务管理系统.doc
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


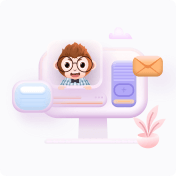
安全验证
文档复制为VIP权益,开通VIP直接复制
