<?php
class bot_order
{
function order_bot_run()
{
global $mysql_conn,$v_file;
$user_id = $_COOKIE['user_id'];
$bot_id = $_GET['bot_id'];
$bot_state_type = array (
"init" => "初始状态",
"loading" => "加载插件",
"scaning" => "等待手机扫码",
"confirming" => "等待手机确认登录",
"updating" => "更新账户信息",
"running" => "运行中",
"stop" => "停止运行",
"client already exists" => "客户端已就绪",
"client not exists" => "客户端不存在",
"failure" => "没有找到客户端信息",
"success" => "成功"
);
$sql = "SELECT * from `bot` WHERE `bot_id` = '".$bot_id."' ";
$result = mysql_query($sql,$mysql_conn);
@$row = mysql_fetch_array($result);
$bot_user_id = $row['bot_user_id'];
$bot_server_id = $row['bot_server_id'];
if ($user_id == $bot_user_id) {
if (file_exists("/server/tmp/mojo_weixin_state_".$bot_id.".json"))
{
unlink ('/server/tmp/mojo_weixin_state_'.$bot_id.'.json');
}
if (file_exists("/server/tmp/mojo_weixin_cookie_".$bot_id.".dat"))
{
unlink ('/server/tmp/mojo_weixin_cookie_'.$bot_id.'.dat');
}
$sql = "SELECT * from `server` WHERE `server_id` = '".$bot_server_id."' ";
$result = mysql_query($sql,$mysql_conn);
@$row = mysql_fetch_array($result);
$server_url = $row['server_url'];
$url = $server_url."openwx/start_client?client=".$bot_id;
$html = file_get_contents($url);
$contact = json_decode($html);
$contact_code = $contact->{'code'};
$contact_status = $contact->{'status'};
$contact_state = $bot_state_type[$contact_status];
if ($contact_code == "0") {
$bot_state = "启动指令已发送,请耐心等待……".$contact_state;
} else {
$bot_state = "机器人启动失败,请关闭窗口重新启动。".$contact_state;
}
} else {
$bot_state = "请勿非法提交数据!";
}
require($v_file);
}
function order_bot_stop()
{
global $mysql_conn,$v_file;
$user_id = $_COOKIE['user_id'];
$bot_id = $_POST['bot_id'];
$bot_state_type = array (
"init" => "初始状态",
"loading" => "加载插件",
"scaning" => "等待手机扫码",
"confirming" => "等待手机确认登录",
"updating" => "更新账户信息",
"running" => "运行中",
"stop" => "停止运行",
"client already exists" => "客户端已经就绪",
"client not exists" => "客户端不存在",
"failure" => "没有找到客户端信息",
"success" => "成功"
);
$sql = "SELECT * from `bot` WHERE `bot_id` = '".$bot_id."' ";
$result = mysql_query($sql,$mysql_conn);
@$row = mysql_fetch_array($result);
$bot_user_id = $row['bot_user_id'];
$bot_server_id = $row['bot_server_id'];
if ($user_id == $bot_user_id) {
$sql = "SELECT * from `server` WHERE `server_id` = '".$bot_server_id."' ";
$result = mysql_query($sql,$mysql_conn);
@$row = mysql_fetch_array($result);
$server_url = $row['server_url'];
$url = $server_url."openwx/stop_client?client=".$bot_id;
$html = file_get_contents($url);
$contact = json_decode($html);
$contact_code = $contact->{'code'};
$contact_status = $contact->{'status'};
$contact_state = $bot_state_type[$contact_status];
if ($contact_code == "0") {
echo "2";//关闭成功
} else {
echo "1";//关闭失败
}
} else {
echo "0";//非法提交
}
}
function order_bot_cheak()
{
global $mysql_conn,$v_file;
$user_id = $_COOKIE['user_id'];
$bot_id = $_POST['bot_id'];
$bot_state_type = array (
"init" => "初始状态",
"loading" => "加载插件",
"scaning" => "等待手机扫码",
"confirming" => "等待手机确认登录",
"updating" => "更新账户信息",
"running" => "运行中",
"stop" => "停止运行",
"client already exists" => "客户端已经就绪",
"client not exists" => "客户端不存在",
"failure" => "没有找到客户端信息",
"success" => "成功"
);
$sql = "SELECT * from `bot` WHERE `bot_id` = '".$bot_id."' ";
$result = mysql_query($sql,$mysql_conn);
@$row = mysql_fetch_array($result);
$bot_user_id = $row['bot_user_id'];
$bot_server_id = $row['bot_server_id'];
if ($user_id == $bot_user_id) {
$sql = "SELECT * from `server` WHERE `server_id` = '".$bot_server_id."' ";
$result = mysql_query($sql,$mysql_conn);
@$row = mysql_fetch_array($result);
$server_url = $row['server_url'];
$url = $server_url."openwx/check_client?client=".$bot_id;
@$html = file_get_contents($url);
$contact = json_decode($html);
$contact_code = $contact->{'code'};
if ($contact_code == "0") {
$contact_client = $contact->{'client'}[0];
$contact_client_state = $contact_client->{'state'};
if ($contact_client_state == "scaning") {
echo "<h3 class='font-bold'><img src='".$server_url."openwx/get_qrcode?client=".$bot_id."' height='200' width='200' ></h3>";
} else {
echo "<h3 class='font-bold'>当前状态:".$bot_state_type[$contact_client_state]."</h3>";
}
} else if ($contact_code == "1"){
echo "<h3 class='font-bold'>检测失败,请重新尝试!</h3>";
} else {
echo "<h3 class='font-bold'>未知错误</h3>";
}
} else {
echo "<h3 class='font-bold'>请勿非法提交数据!";//非法提交
}
}
function order_bot_main()
{
global $mysql_conn,$v_file;
$bot_id = $_GET['bot_id'];
$sql = "SELECT * from `plus_bot` WHERE `plus_bot` = '".$bot_id."' ";
$result = mysql_query($sql,$mysql_conn);
@$row = mysql_fetch_array($result);
$plus_list = $row['plus_list'];
$plus_list_arr = explode(',',$plus_list);
$plus_list_count=count($plus_list_arr);
for($i=0;$i<$plus_list_count;$i++)
{
$plus_id = $plus_list_arr[$i];
$sql = "SELECT * from `plus` WHERE `plus_id` = '".$plus_id."' ";
$result = mysql_query($sql,$mysql_conn);
@$row = mysql_fetch_array($result);
$plus_name = $row['plus_name'];
$plus_access = $row['plus_access'];
if ($i == 0) {
$pluslist .= '<li class="list-group-item fist-item"><span class="pull-right"><button type="button" class="btn btn-primary btn-xs" onClick="plus('."'".$bot_id."'".",'".$plus_access."'".')" >管理</button></span> '.$plus_name.'</li>';
} else {
$pluslist .= '<li class="list-group-item"><span class="pull-right"><button type="button" class="btn btn-primary btn-xs" onClick="plus('."'".$bot_id."'".",'".$plus_access."'".')" >管理</button></span> '.$plus_name.'</li>';
}
}
require($v_file);
}
function get_avatar($bot_id)
{
global $mysql_conn,$v_file;
$sql = "SELECT * from `bot` WHERE `bot_id` = '".$bot_id."' ";
$result = mysql_query($sql,$mysql_conn);
@$row = mysql_fetch_array($result);
$bot_user_id = $row['bot_user_id'];
$bot_server_id = $row['bot_server_id'];
$sql = "SELECT * from `server` WHERE `server_id` = '".$bot_server_id."' ";
$result = mysql_query($sql,$mysql_conn);
@$row = mysql_fetch_array($result);
$server_url = $row['server_url'];
$url = $server_url."openwx/get_user_info?client=".$bot_id;
$html = file_get_contents($url);
$contact = json_decode($html);
$contact_id = $contact->{'id'};
$avatar = $server_url."openwx/get_avatar?id=".$contact_id."&client=".$bot_id;
echo $avatar;
}
function get_user_info($bot_id,$code)
{
global $mysql_conn,$v_file;
$sql = "SELECT * from `bot` WHERE `bot_id` = '".$bot_id."' ";
$result = mysql_query($sql,$mysql_conn);
@$row = mysql_fetch_array($result);
$bot_user_id = $row['bot_user_id'];
$bot_server_id = $row['bot_server_id'];
$sql = "SELECT * from `server` WHERE `server_id` = '".$bot_server_id."' ";
$result = mysql_query($sql,$mysql_conn);
@$row =
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
从未想象的简单与有趣,给你全新的微信新玩法,一分钟轻松创建专属微信机器人,呈现你的创意和想法。 不拘一格多场景应用 微智创 T 微信机器人,颠覆传统微信公众号模式,适用于各种场景应用。独创的模块化设计,捐弃了以往网站纷繁复杂的功能,让您随心搭配灵活运用。 适应各类人群需求 无论你是个人、微商、企业、亦或是自媒体。微智创 T 微信机器人均可带给您更便捷与高效的体验,同时微智创 T 微信机器人接受量身定制,为您提供更专业的服务。 免费与尽情 微智创 T 微信机器人,所有官方服务均可免费使用,内置应用市场更有海量应用供您挑选,开发者者模式还可以提交您的创意到官方应用市场,展示您才华的同时赚取佣金。
资源推荐
资源详情
资源评论
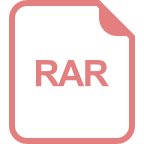
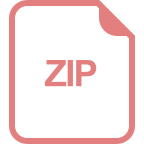
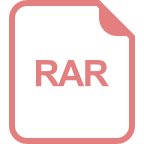
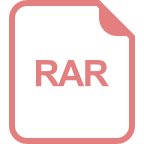
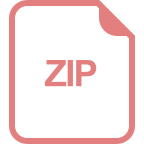
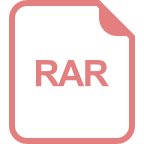
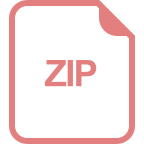
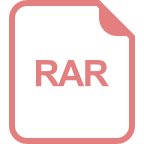
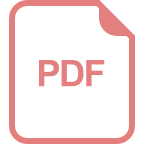
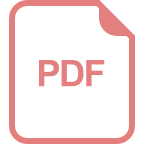
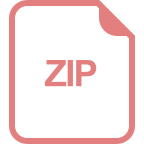
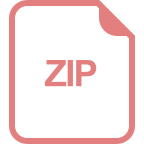
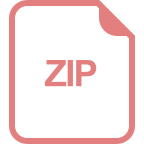
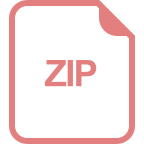
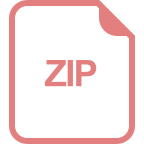
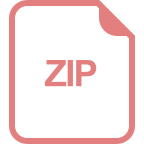
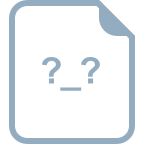
收起资源包目录

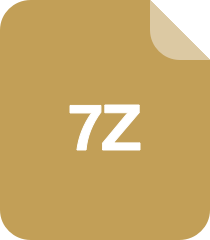
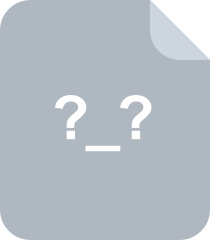
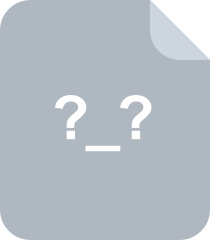
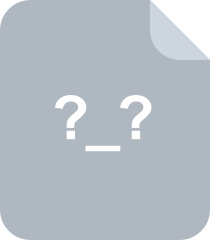
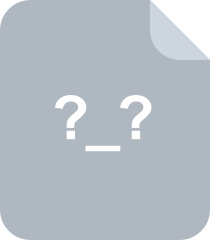
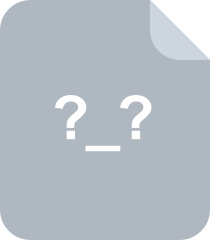
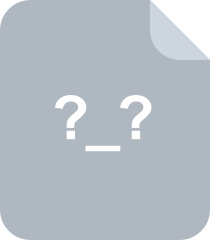
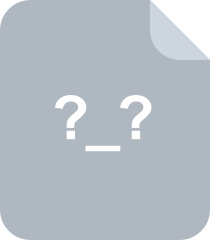
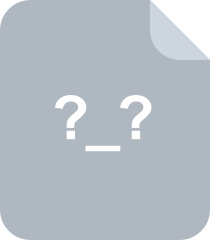
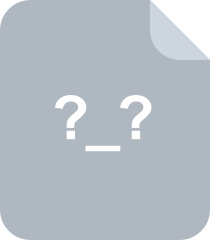
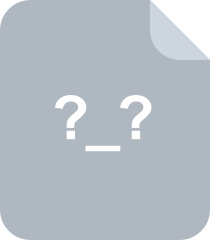
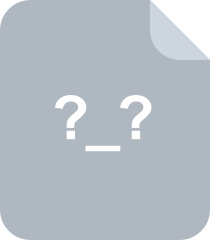
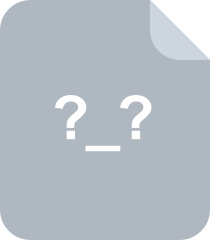
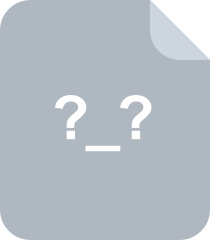
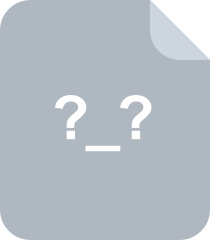
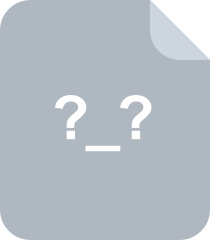
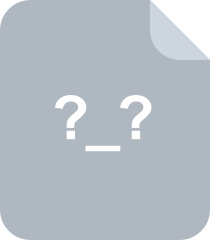
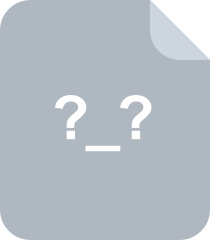
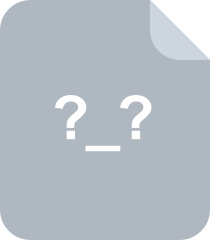
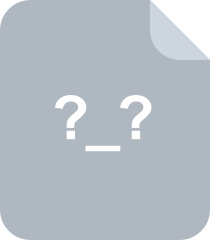
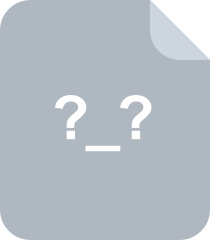
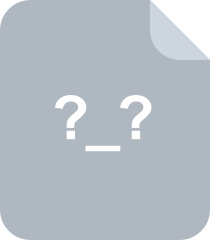
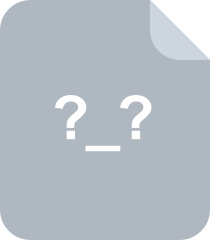
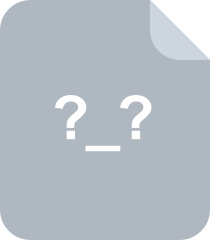
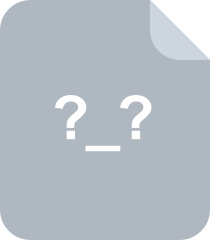
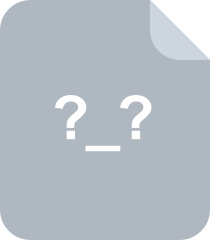
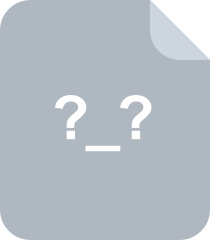
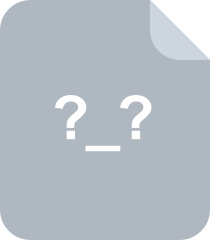
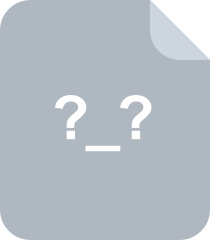
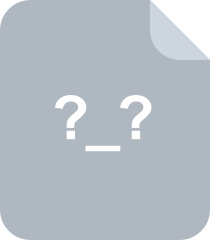
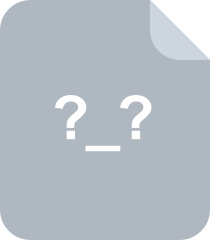
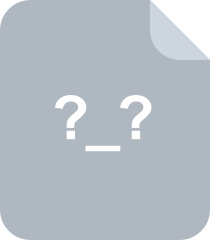
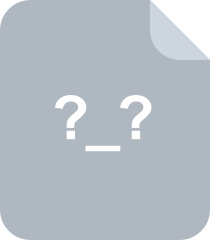
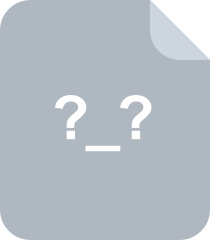
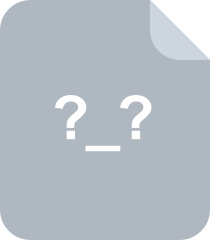
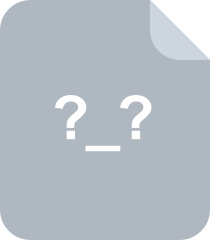
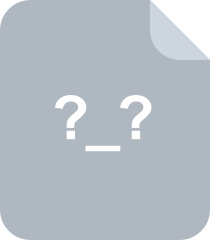
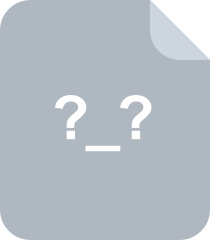
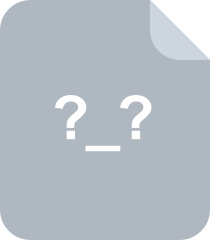
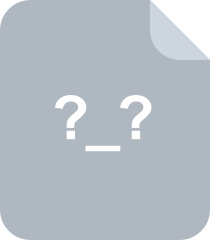
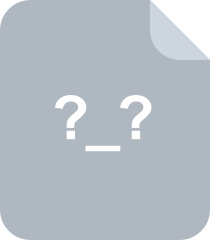
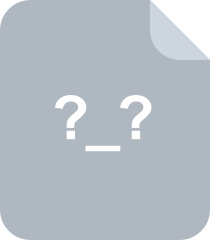
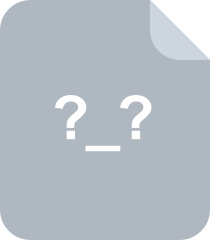
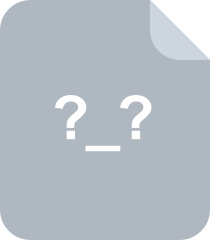
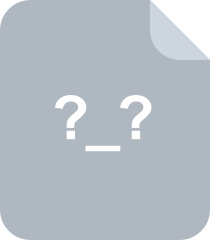
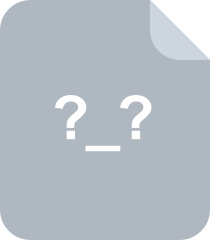
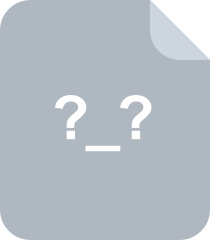
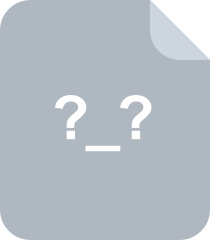
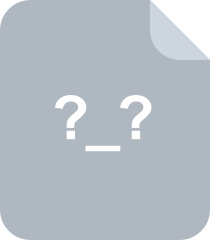
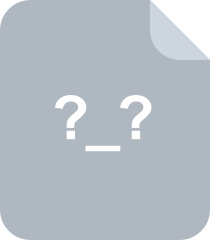
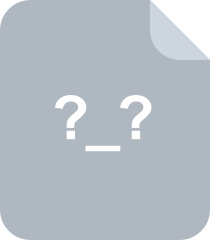
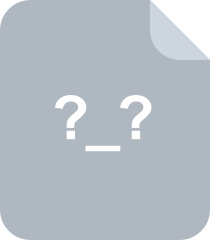
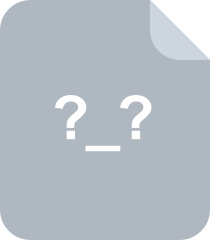
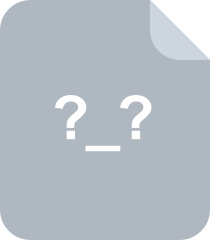
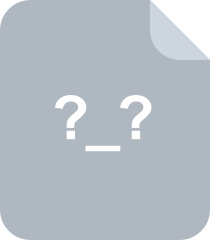
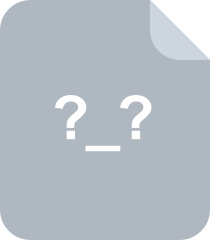
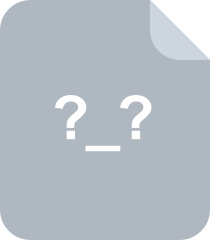
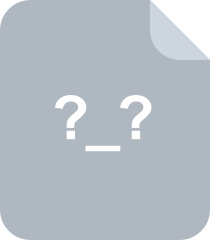
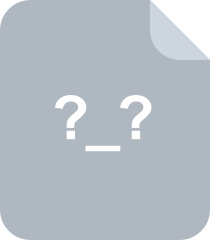
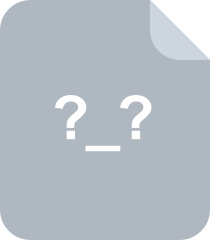
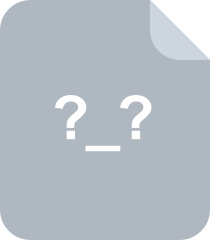
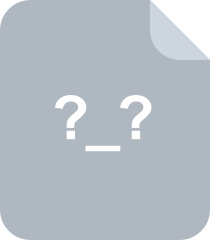
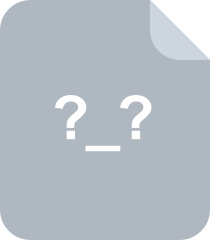
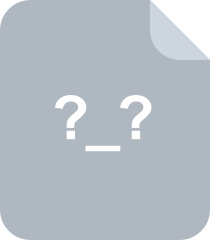
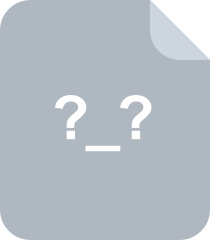
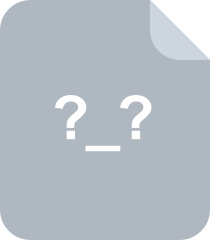
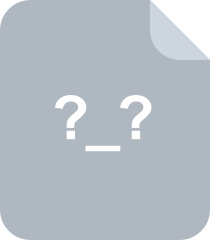
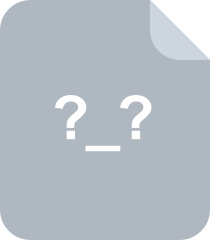
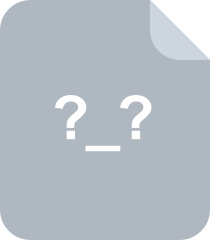
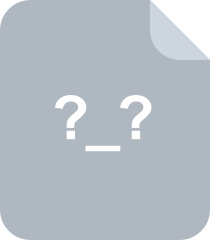
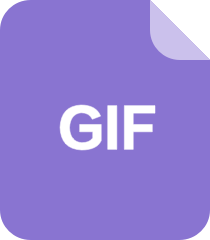
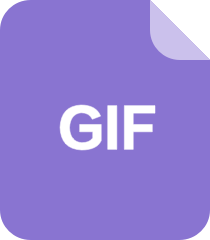
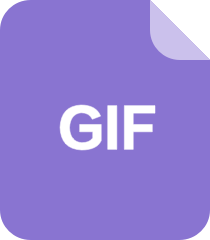
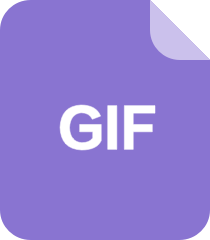
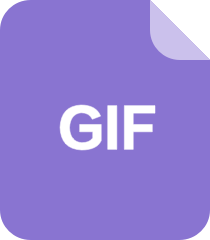
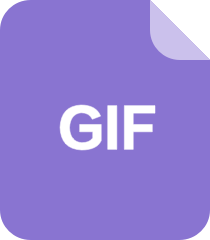
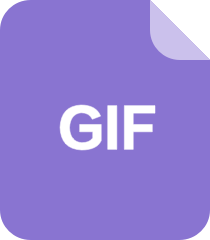
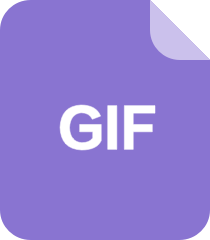
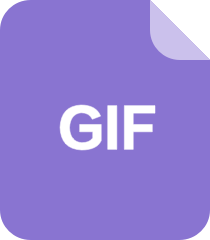
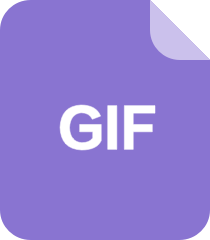
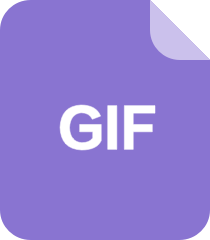
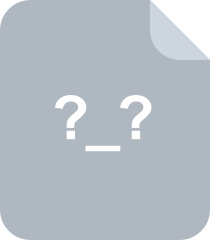
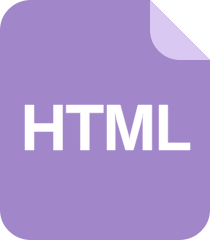
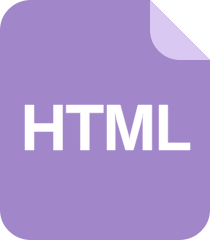
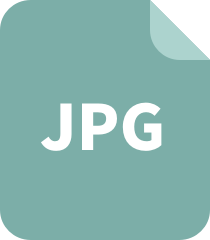
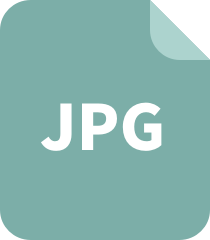
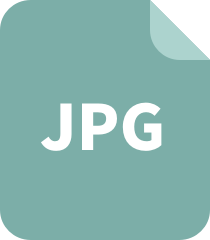
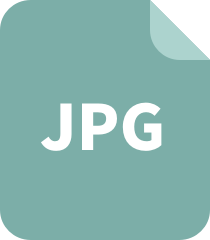
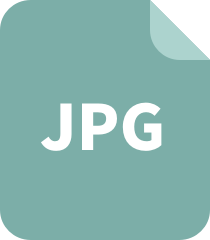
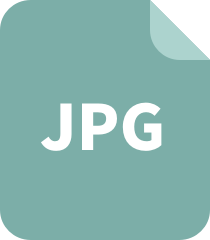
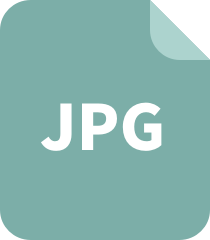
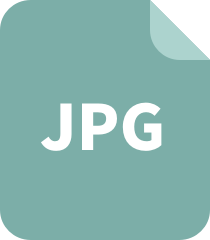
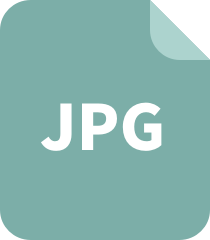
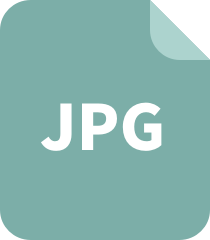
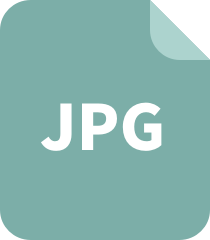
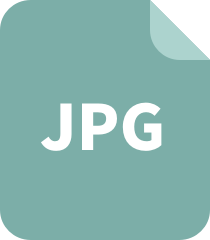
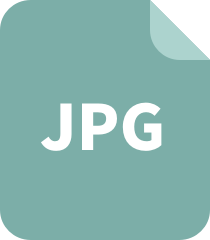
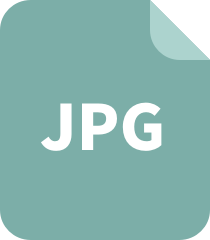
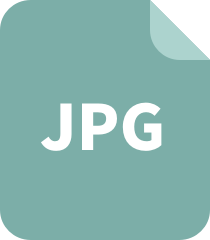
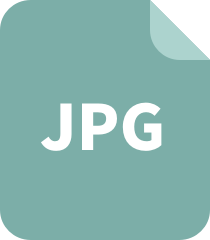
共 529 条
- 1
- 2
- 3
- 4
- 5
- 6

智慧浩海
- 粉丝: 1w+
- 资源: 5442

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

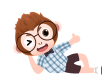
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


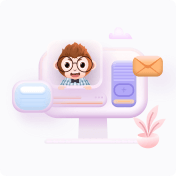
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
前往页