<?php
function get_file($url, $name, $folder = './')
{
set_time_limit(24 * 60 * 60);
// 设置超时时间
$destination_folder = $folder . '/';
// 文件下载保存目录,默认为当前文件目录
if (!is_dir($destination_folder)) {
// 判断目录是否存在
mkdirs($destination_folder);
}
$newfname = $destination_folder . $name;
// 取得文件的名称
$file = fopen($url, 'rb');
// 远程下载文件,二进制模式
if ($file) {
// 如果下载成功
$newf = fopen($newfname, 'wb');
// 远在文件文件
if ($newf) {
// 如果文件保存成功
while (!feof($file)) {
// 判断附件写入是否完整
fwrite($newf, fread($file, 1024 * 8), 1024 * 8);
}
}
}
if ($file) {
fclose($file);
}
if ($newf) {
fclose($newf);
}
return true;
}
function mkdirs($path, $mode = '0777')
{
if (!is_dir($path)) {
// 判断目录是否存在
mkdirs(dirname($path), $mode);
// 循环建立目录
mkdir($path, $mode);
}
return true;
}
//循环删除目录和文件函数
function delDirAndFile($dirName)
{
if ($handle = opendir("{$dirName}")) {
while (false !== ($item = readdir($handle))) {
if ($item != "." && $item != "..") {
if (is_dir("{$dirName}/{$item}")) {
delDirAndFile("{$dirName}/{$item}");
} else {
unlink("{$dirName}/{$item}");
}
}
}
closedir($handle);
//rmdir($dirName);
}
}
// --------------------------------------------------------------------------------
// PhpConcept Library - Zip Module 2.8.2
// --------------------------------------------------------------------------------
// License GNU/LGPL - Vincent Blavet - August 2009
// http://www.phpconcept.net
// --------------------------------------------------------------------------------
//
// Presentation :
// PclZip is a PHP library that manage ZIP archives.
// So far tests show that archives generated by PclZip are readable by
// WinZip application and other tools.
//
// Description :
// See readme.txt and http://www.phpconcept.net
//
// Warning :
// This library and the associated files are non commercial, non professional
// work.
// It should not have unexpected results. However if any damage is caused by
// this software the author can not be responsible.
// The use of this software is at the risk of the user.
//
// --------------------------------------------------------------------------------
// $Id: pclzip.lib.php,v 1.60 2009/09/30 21:01:04 vblavet Exp $
// --------------------------------------------------------------------------------
// ----- Constants
if (!defined('PCLZIP_READ_BLOCK_SIZE')) {
define('PCLZIP_READ_BLOCK_SIZE', 2048);
}
// ----- File list separator
// In version 1.x of PclZip, the separator for file list is a space
// (which is not a very smart choice, specifically for windows paths !).
// A better separator should be a comma (,). This constant gives you the
// abilty to change that.
// However notice that changing this value, may have impact on existing
// scripts, using space separated filenames.
// Recommanded values for compatibility with older versions :
//define( 'PCLZIP_SEPARATOR', ' ' );
// Recommanded values for smart separation of filenames.
if (!defined('PCLZIP_SEPARATOR')) {
define('PCLZIP_SEPARATOR', ',');
}
// ----- Error configuration
// 0 : PclZip Class integrated error handling
// 1 : PclError external library error handling. By enabling this
// you must ensure that you have included PclError library.
// [2,...] : reserved for futur use
if (!defined('PCLZIP_ERROR_EXTERNAL')) {
define('PCLZIP_ERROR_EXTERNAL', 0);
}
// ----- Optional static temporary directory
// By default temporary files are generated in the script current
// path.
// If defined :
// - MUST BE terminated by a '/'.
// - MUST be a valid, already created directory
// Samples :
// define( 'PCLZIP_TEMPORARY_DIR', '/temp/' );
// define( 'PCLZIP_TEMPORARY_DIR', 'C:/Temp/' );
if (!defined('PCLZIP_TEMPORARY_DIR')) {
define('PCLZIP_TEMPORARY_DIR', '');
}
// ----- Optional threshold ratio for use of temporary files
// Pclzip sense the size of the file to add/extract and decide to
// use or not temporary file. The algorythm is looking for
// memory_limit of PHP and apply a ratio.
// threshold = memory_limit * ratio.
// Recommended values are under 0.5. Default 0.47.
// Samples :
// define( 'PCLZIP_TEMPORARY_FILE_RATIO', 0.5 );
if (!defined('PCLZIP_TEMPORARY_FILE_RATIO')) {
define('PCLZIP_TEMPORARY_FILE_RATIO', 0.47);
}
// --------------------------------------------------------------------------------
// ***** UNDER THIS LINE NOTHING NEEDS TO BE MODIFIED *****
// --------------------------------------------------------------------------------
// ----- Global variables
$g_pclzip_version = "2.8.2";
// ----- Error codes
// -1 : Unable to open file in binary write mode
// -2 : Unable to open file in binary read mode
// -3 : Invalid parameters
// -4 : File does not exist
// -5 : Filename is too long (max. 255)
// -6 : Not a valid zip file
// -7 : Invalid extracted file size
// -8 : Unable to create directory
// -9 : Invalid archive extension
// -10 : Invalid archive format
// -11 : Unable to delete file (unlink)
// -12 : Unable to rename file (rename)
// -13 : Invalid header checksum
// -14 : Invalid archive size
define('PCLZIP_ERR_USER_ABORTED', 2);
define('PCLZIP_ERR_NO_ERROR', 0);
define('PCLZIP_ERR_WRITE_OPEN_FAIL', -1);
define('PCLZIP_ERR_READ_OPEN_FAIL', -2);
define('PCLZIP_ERR_INVALID_PARAMETER', -3);
define('PCLZIP_ERR_MISSING_FILE', -4);
define('PCLZIP_ERR_FILENAME_TOO_LONG', -5);
define('PCLZIP_ERR_INVALID_ZIP', -6);
define('PCLZIP_ERR_BAD_EXTRACTED_FILE', -7);
define('PCLZIP_ERR_DIR_CREATE_FAIL', -8);
define('PCLZIP_ERR_BAD_EXTENSION', -9);
define('PCLZIP_ERR_BAD_FORMAT', -10);
define('PCLZIP_ERR_DELETE_FILE_FAIL', -11);
define('PCLZIP_ERR_RENAME_FILE_FAIL', -12);
define('PCLZIP_ERR_BAD_CHECKSUM', -13);
define('PCLZIP_ERR_INVALID_ARCHIVE_ZIP', -14);
define('PCLZIP_ERR_MISSING_OPTION_VALUE', -15);
define('PCLZIP_ERR_INVALID_OPTION_VALUE', -16);
define('PCLZIP_ERR_ALREADY_A_DIRECTORY', -17);
define('PCLZIP_ERR_UNSUPPORTED_COMPRESSION', -18);
define('PCLZIP_ERR_UNSUPPORTED_ENCRYPTION', -19);
define('PCLZIP_ERR_INVALID_ATTRIBUTE_VALUE', -20);
define('PCLZIP_ERR_DIRECTORY_RESTRICTION', -21);
// ----- Options values
define('PCLZIP_OPT_PATH', 77001);
define('PCLZIP_OPT_ADD_PATH', 77002);
define('PCLZIP_OPT_REMOVE_PATH', 77003);
define('PCLZIP_OPT_REMOVE_ALL_PATH', 77004);
define('PCLZIP_OPT_SET_CHMOD', 77005);
define('PCLZIP_OPT_EXTRACT_AS_STRING', 77006);
define('PCLZIP_OPT_NO_COMPRESSION', 77007);
define('PCLZIP_OPT_BY_NAME', 77008);
define('PCLZIP_OPT_BY_INDEX', 77009);
define('PCLZIP_OPT_BY_EREG', 77010);
define('PCLZIP_OPT_BY_PREG', 77011);
define('PCLZIP_OPT_COMMENT', 77012);
define('PCLZIP_OPT_ADD_COMMENT', 77013);
define('PCLZIP_OPT_PREPEND_COMMENT', 77014);
define('PCLZIP_OPT_EXTRACT_IN_OUTPUT', 77015);
define('PCLZIP_OPT_REPLACE_NEWER', 77016);
define('PCLZIP_OPT_STOP_ON_ERROR', 77017);
// Having big trouble with crypt. Need to multiply 2 long int
// which is not correctly supported by PHP ...
//define( 'PCLZIP_OPT_CRYPT', 77018 );
define('PCLZIP_OPT_EXTRACT_DIR_RESTRICTION', 77019);
define('PCLZIP_OPT_TEMP_FILE_THRESHOLD', 77020);
define('PCLZIP_OPT_ADD_TEMP_FILE_THRESHOLD', 77020);
// alias
define('PCLZIP_OPT_TEMP_FILE_ON', 77021);
define('PCLZIP_OPT_ADD_TEMP_FILE_ON', 77021);
// alias
define('PCLZIP_OPT_TEMP_FILE_OFF', 77022);
define('PCLZIP_OPT_ADD_TEMP_FILE_OFF', 77022);
// alias
// ----- File description attributes
define('PCLZIP_ATT_FILE_NAME', 79001);
define(
没有合适的资源?快使用搜索试试~ 我知道了~
公众号模块 在线考试2.9.1源码
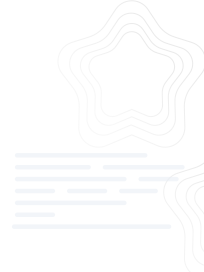
共592个文件
html:190个
php:170个
png:138个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 15 浏览量
2022-03-21
10:33:58
上传
评论
收藏 10.2MB ZIP 举报
温馨提示
1,新增自定义题型,可以根据原型添加多个自定义的试题题型。 2,新增题型排序:考试试卷的题型排序可以根据自己的喜好编排。 3,新增自定试题排序:试题的排序可以根据自己的喜好编排。 4,优化试卷选题马上显示数量特效 5,部分代码调整。
资源推荐
资源详情
资源评论
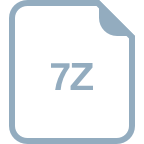
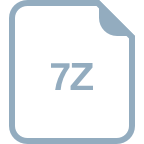
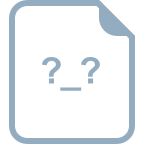
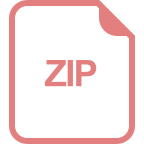
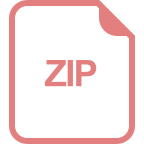
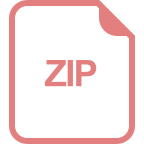
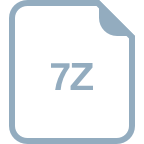
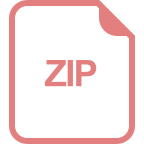
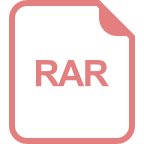
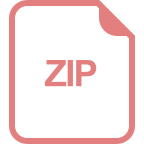
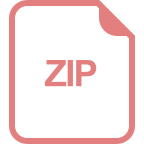
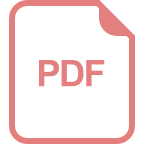
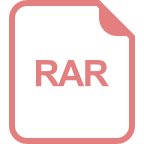
收起资源包目录

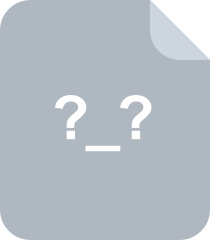
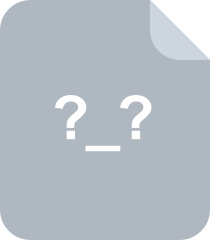
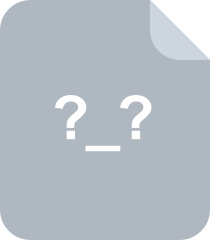
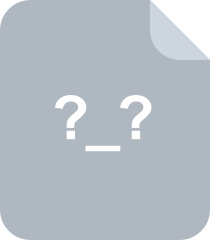
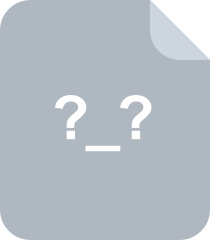
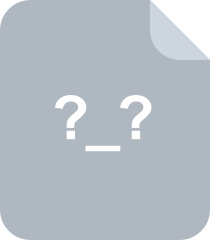
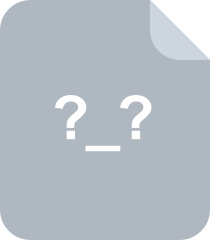
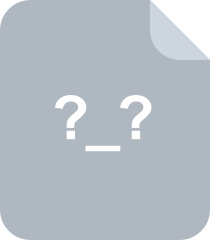
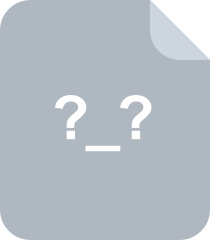
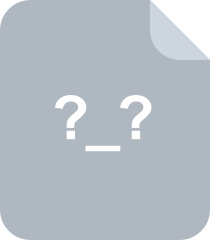
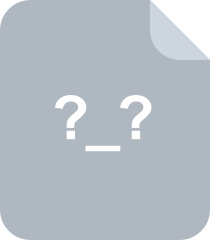
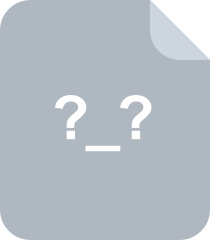
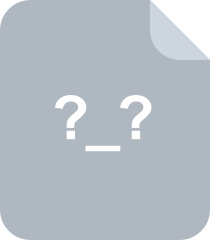
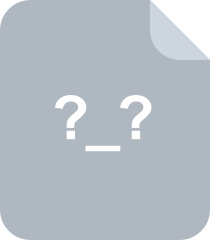
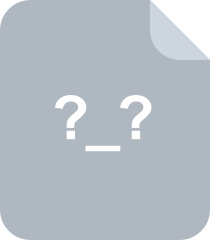
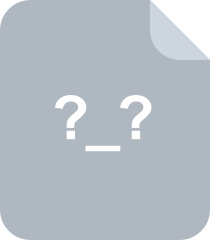
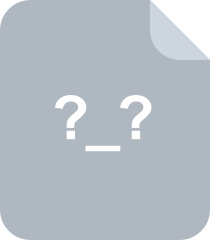
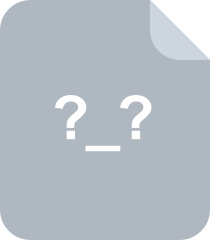
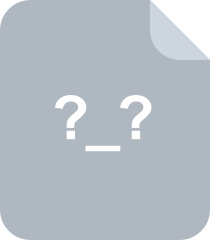
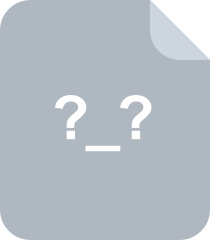
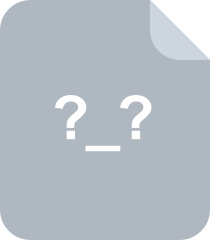
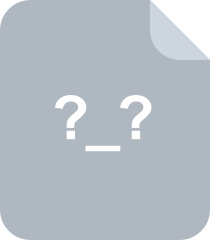
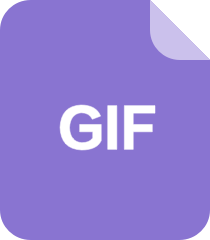
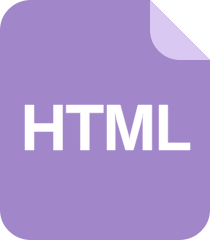
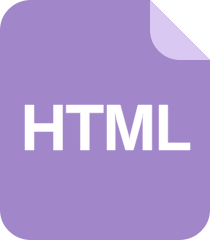
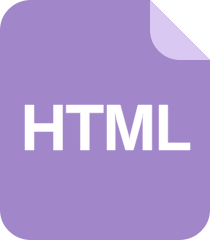
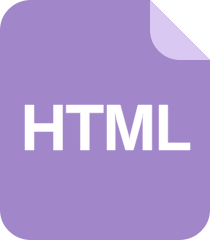
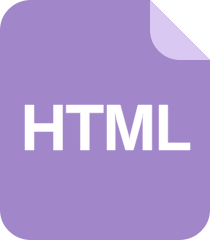
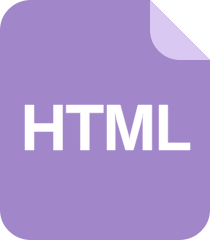
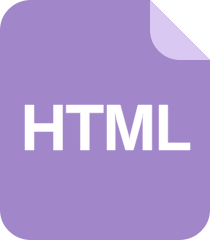
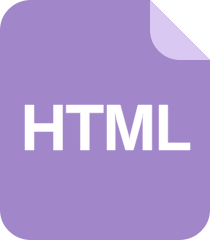
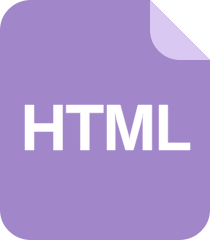
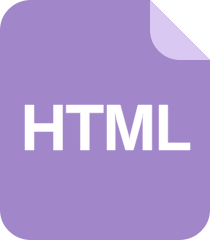
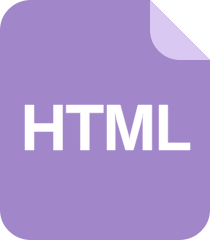
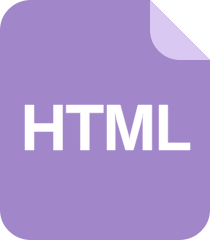
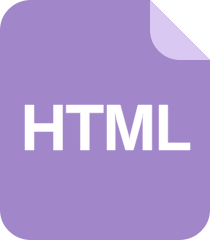
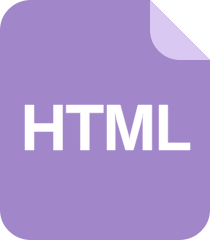
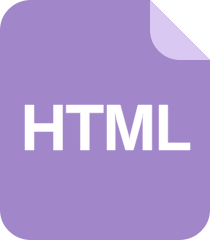
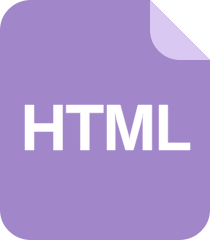
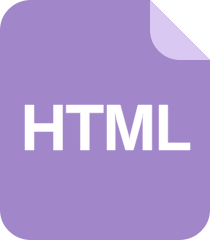
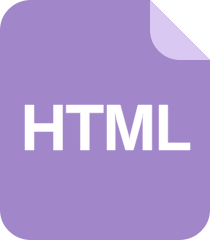
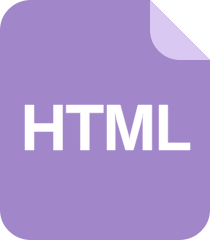
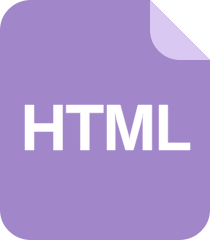
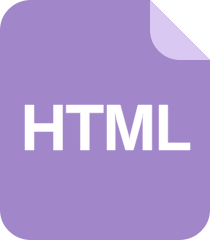
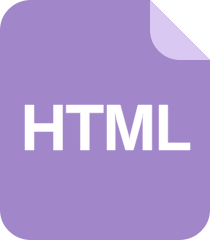
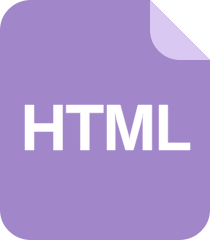
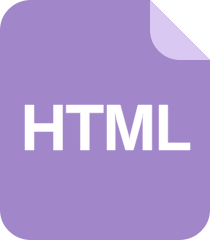
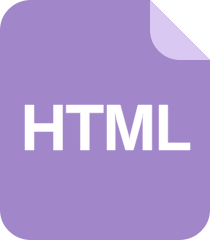
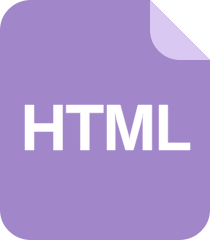
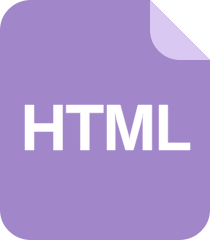
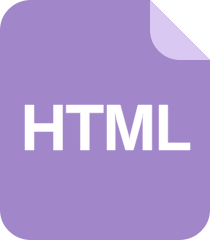
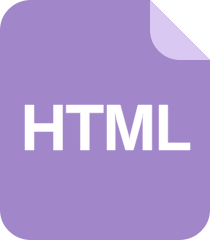
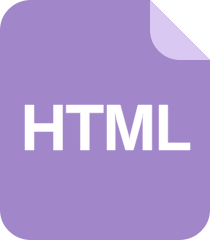
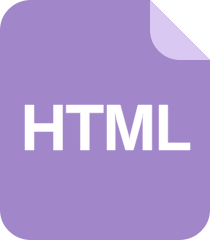
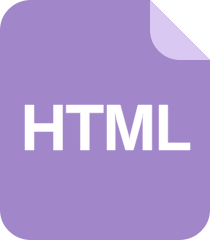
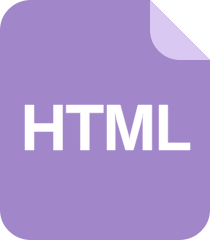
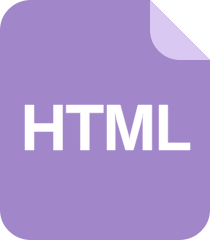
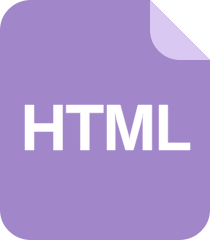
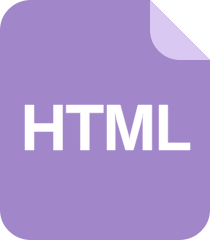
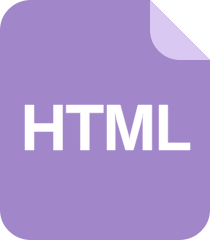
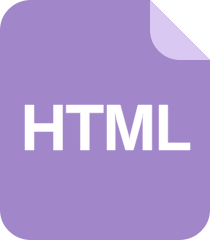
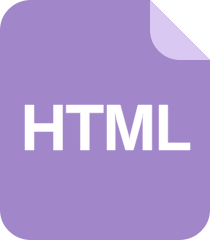
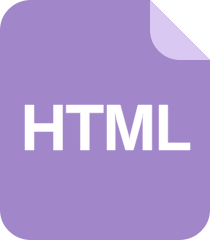
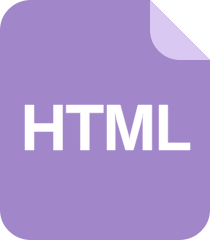
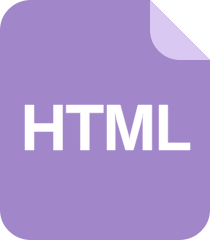
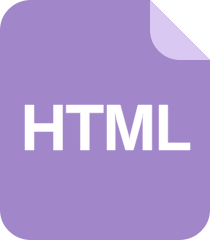
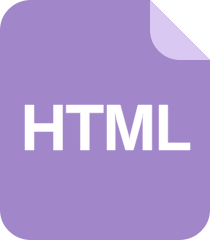
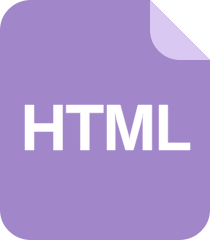
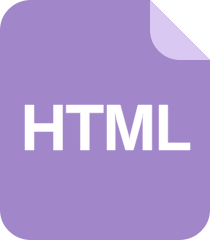
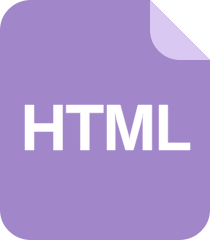
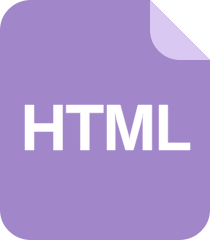
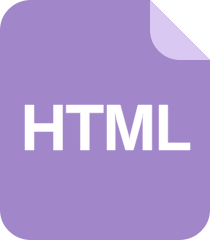
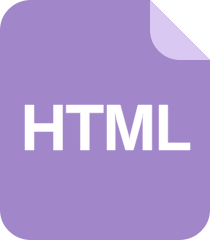
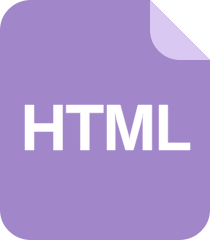
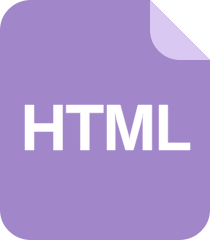
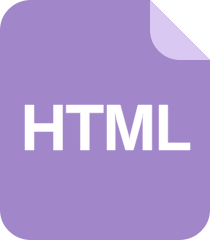
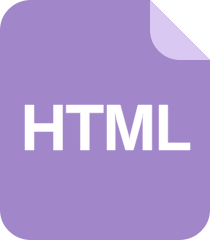
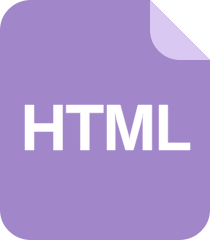
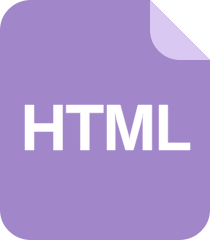
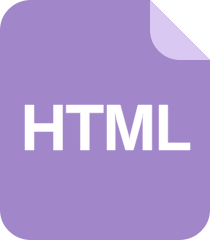
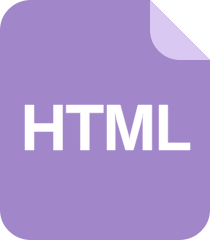
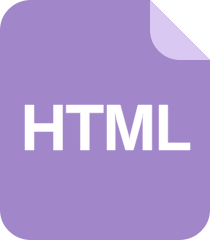
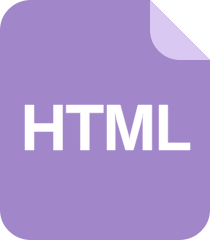
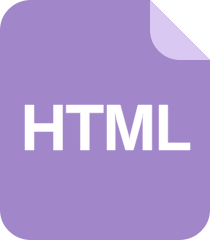
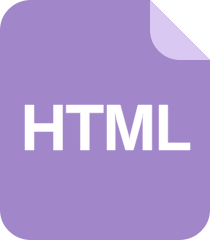
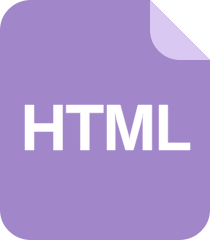
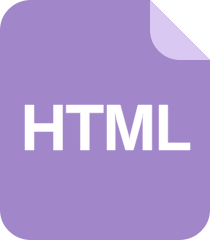
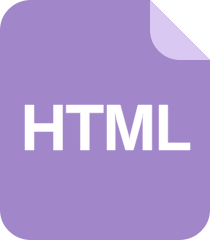
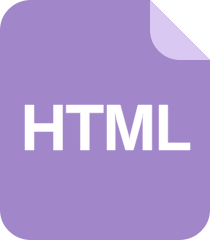
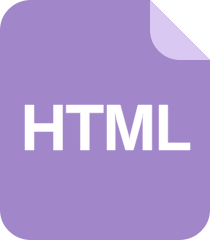
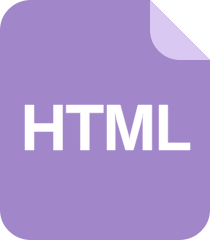
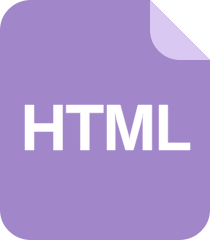
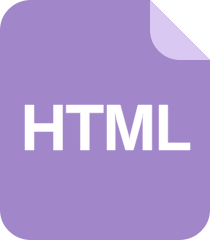
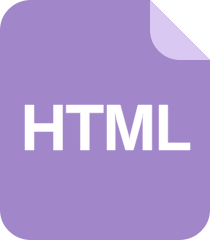
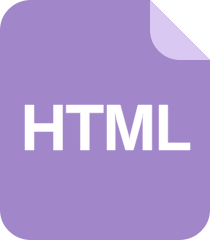
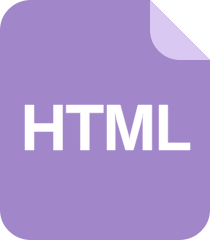
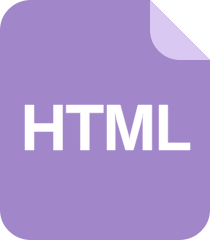
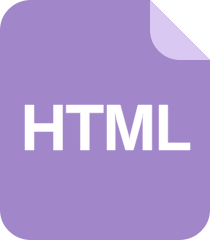
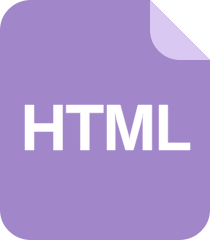
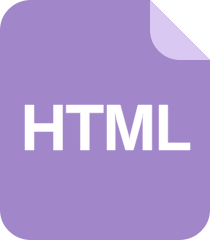
共 592 条
- 1
- 2
- 3
- 4
- 5
- 6
资源评论
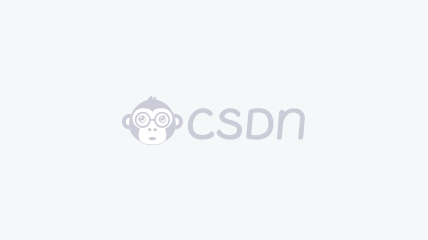

智慧浩海
- 粉丝: 1w+
- 资源: 5145
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

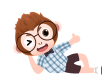
安全验证
文档复制为VIP权益,开通VIP直接复制
