package test;
import banking5.Account;
import banking5.Bank;
import banking5.CheckingAccount;
import banking5.Customer;
import banking5.SavingAccount;
/*
* This class creates the program to test the banking classes.
* It creates a new Bank, sets the Customer (with an initial balance),
* and performs a series of transactions with the Account object.
*/
public class TestBanking_5 {
public static void main(String[] args) {
Bank bank = new Bank();
Customer customer;
Account account;
//
// Create bank customers and their accounts
//
System.out.println("Creating the customer Jane Smith.");
bank.addCustomer("Jane", "Simms");
// code
System.out
.println("Creating her Savings Account with a 500.00 balance and 3% interest.");
// code
bank.getCustomer(0).setAccount(new SavingAccount(500, 0.03));
System.out.println("Creating the customer Owen Bryant.");
// code
bank.addCustomer("Owen", "Bryant");
customer = bank.getCustomer(1);
System.out
.println("Creating his Checking Account with a 500.00 balance and no overdraft protection.");
// code
customer.setAccount(new CheckingAccount(500));
System.out.println("Creating the customer Tim Soley.");
bank.addCustomer("Tim", "Soley");
customer = bank.getCustomer(2);
System.out
.println("Creating his Checking Account with a 500.00 balance and 500.00 in overdraft protection.");
// code
customer.setAccount(new CheckingAccount(500, 500));
System.out.println("Creating the customer Maria Soley.");
// code
bank.addCustomer("Maria", "Soley");
customer = bank.getCustomer(3);
System.out
.println("Maria shares her Checking Account with her husband Tim.");
customer.setAccount(bank.getCustomer(2).getAccount());
System.out.println();
//
// Demonstrate behavior of various account types
//
// Test a standard Savings Account
System.out
.println("Retrieving the customer Jane Smith with her savings account.");
customer = bank.getCustomer(0);
account = customer.getAccount();
// Perform some account transactions
System.out.println("Withdraw 150.00: " + account.withdraw(150.00));
System.out.println("Deposit 22.50: " + account.deposit(22.50));
System.out.println("Withdraw 47.62: " + account.withdraw(47.62));
System.out.println("Withdraw 400.00: " + account.withdraw(400.00));
// Print out the final account balance
System.out.println("Customer [" + customer.getLastName() + ", "
+ customer.getFirstName() + "] has a balance of "
+ account.getBalance());
System.out.println();
// Test a Checking Account w/o overdraft protection
System.out
.println("Retrieving the customer Owen Bryant with his checking account with no overdraft protection.");
customer = bank.getCustomer(1);
account = customer.getAccount();
// Perform some account transactions
System.out.println("Withdraw 150.00: " + account.withdraw(150.00));
System.out.println("Deposit 22.50: " + account.deposit(22.50));
System.out.println("Withdraw 47.62: " + account.withdraw(47.62));
System.out.println("Withdraw 400.00: " + account.withdraw(400.00));
// Print out the final account balance
System.out.println("Customer [" + customer.getLastName() + ", "
+ customer.getFirstName() + "] has a balance of "
+ account.getBalance());
System.out.println();
// Test a Checking Account with overdraft protection
System.out
.println("Retrieving the customer Tim Soley with his checking account that has overdraft protection.");
customer = bank.getCustomer(2);
account = customer.getAccount();
// Perform some account transactions
System.out.println("Withdraw 150.00: " + account.withdraw(150.00));
System.out.println("Deposit 22.50: " + account.deposit(22.50));
System.out.println("Withdraw 47.62: " + account.withdraw(47.62));
System.out.println("Withdraw 400.00: " + account.withdraw(400.00));
// Print out the final account balance
System.out.println("Customer [" + customer.getLastName() + ", "
+ customer.getFirstName() + "] has a balance of "
+ account.getBalance());
System.out.println();
// Test a Checking Account with overdraft protection
System.out
.println("Retrieving the customer Maria Soley with her joint checking account with husband Tim.");
customer = bank.getCustomer(3);
account = customer.getAccount();
// Perform some account transactions
System.out.println("Deposit 150.00: " + account.deposit(150.00));
System.out.println("Withdraw 750.00: " + account.withdraw(750.00));
// Print out the final account balance
System.out.println("Customer [" + customer.getLastName() + ", "
+ customer.getFirstName() + "] has a balance of "
+ account.getBalance());
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
Java基础实战-Bank项目-源代码
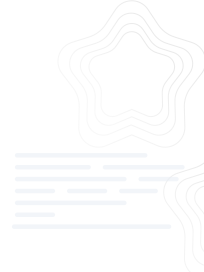
共47个文件
java:47个

0 下载量 198 浏览量
2023-06-16
16:19:55
上传
评论
收藏 44KB ZIP 举报
温馨提示
Java基础实战_银行软件项目_源代码
资源推荐
资源详情
资源评论
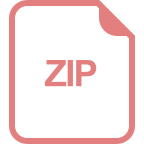
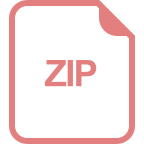
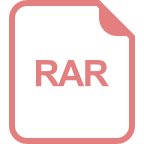
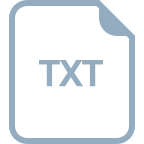
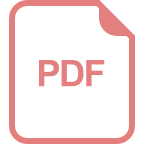
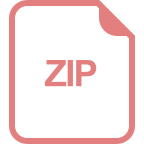
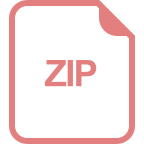
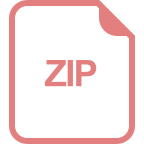
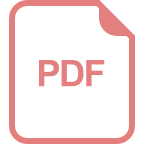
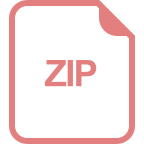
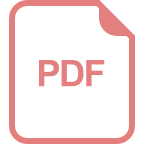
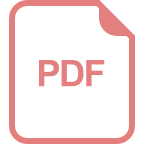
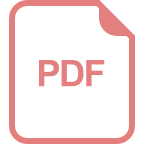
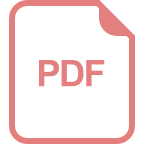
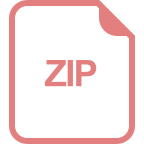
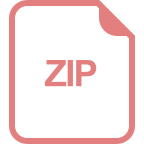
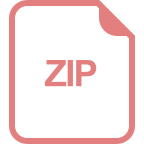
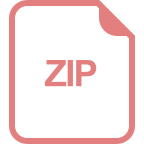
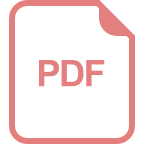
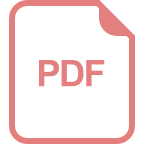
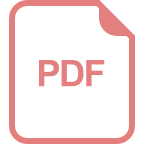
收起资源包目录



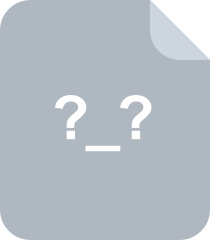
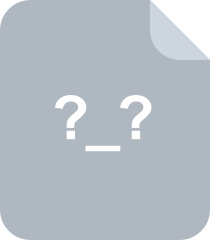
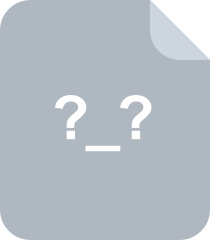
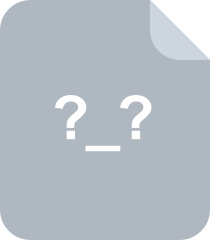
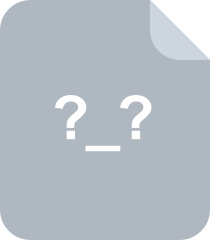
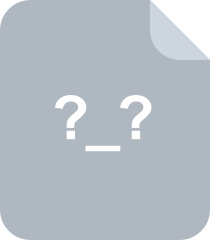
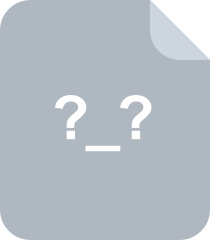

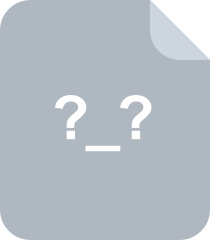
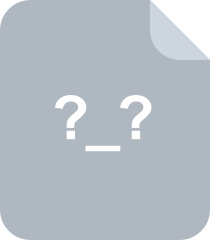

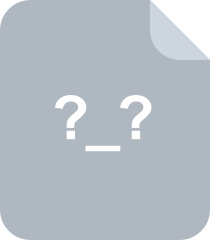
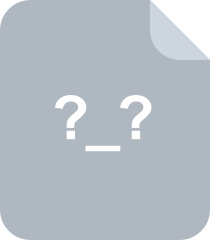
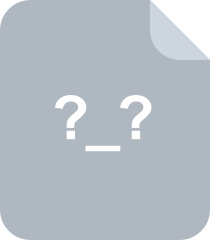
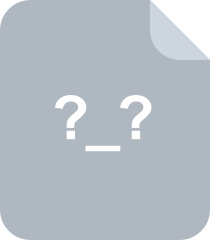

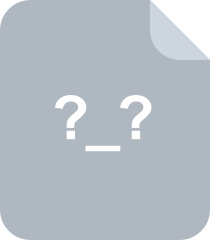
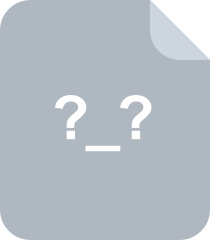
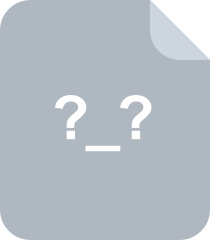
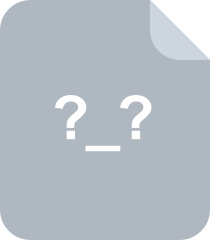
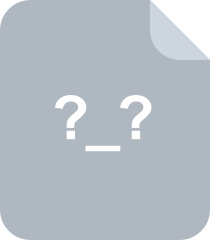
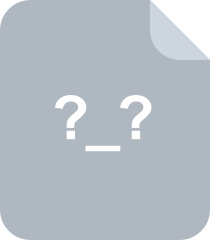
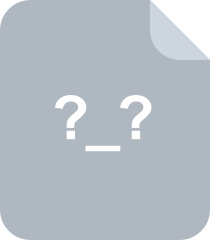

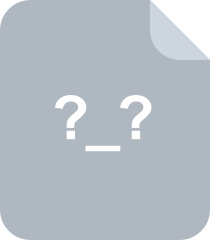

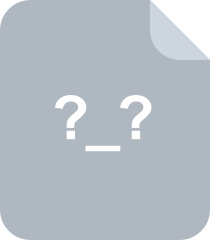
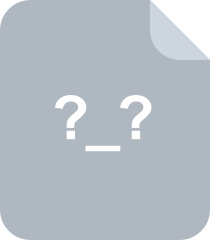
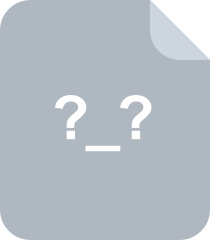

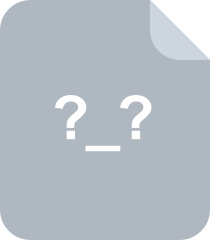
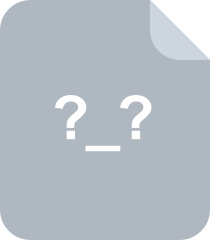
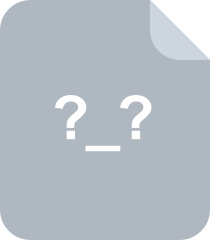
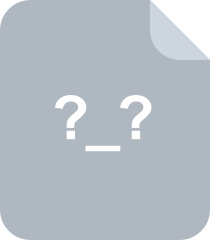
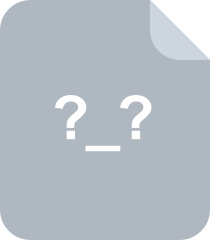
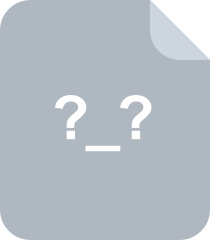

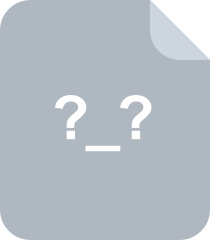
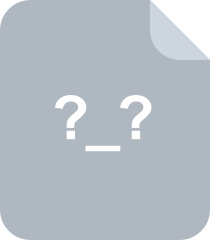
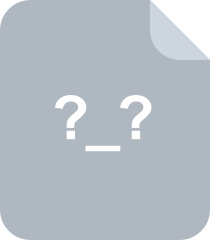
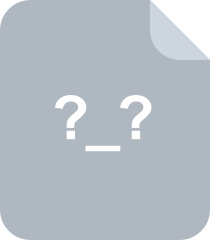
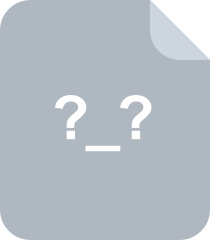
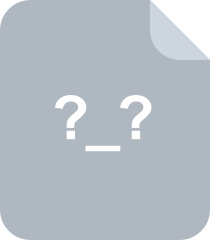

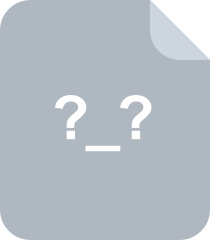
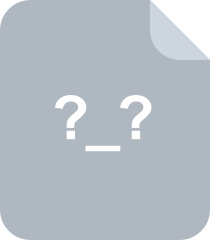
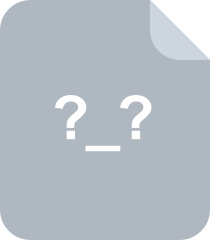
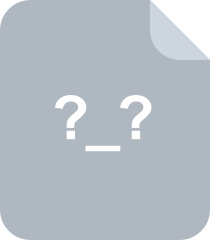
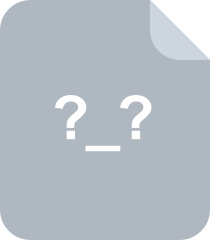
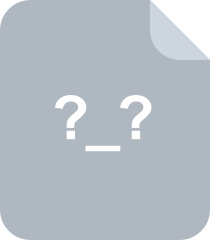
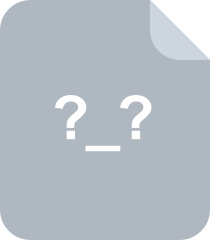
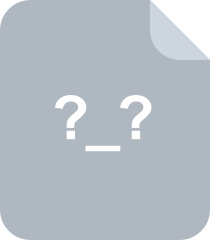

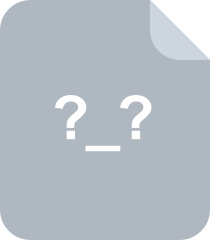
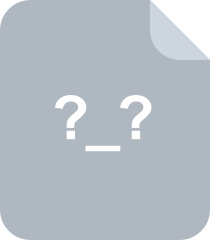
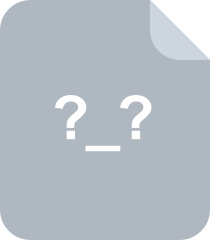
共 47 条
- 1
资源评论
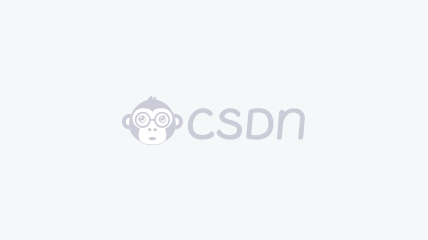
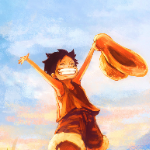

逃逸的卡路里
- 粉丝: 1w+
- 资源: 5357
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

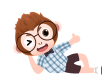
最新资源
- Java 代码覆盖率库.zip
- Java 代码和算法的存储库 也为该存储库加注星标 .zip
- 免安装Windows10/Windows11系统截图工具,无需安装第三方截图工具 双击直接使用截图即可 是一款免费可靠的截图小工具哦~
- Libero Soc v11.9的安装以及证书的获取(2021新版).zip
- BouncyCastle.Cryptography.dll
- 5.1 孤立奇点(JD).ppt
- 基于51单片机的智能交通灯控制系统的设计与实现源码+报告(高分项目)
- 什么是 SQL 注入.docx
- Windows 11上启用与禁用网络发现功能的操作指南
- Java Redis 客户端 GUI 工具.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


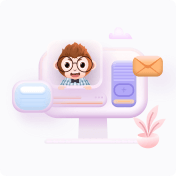
安全验证
文档复制为VIP权益,开通VIP直接复制
