没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
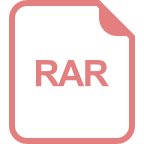
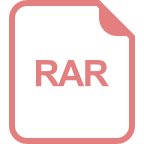
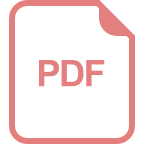
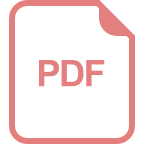
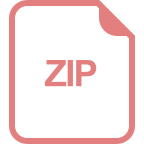
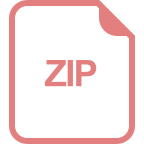
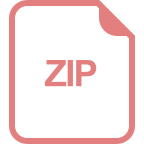
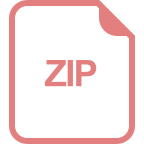
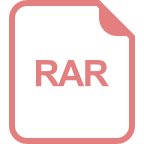
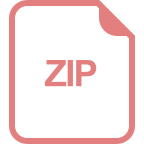
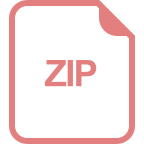
收起资源包目录



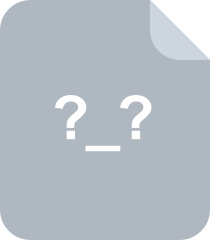



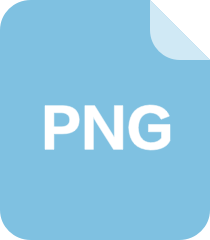
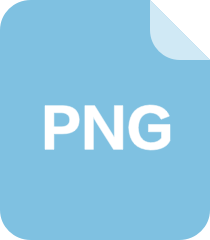
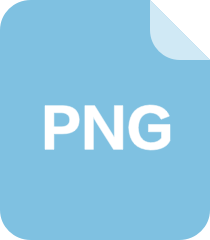
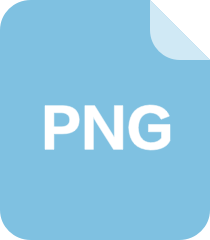

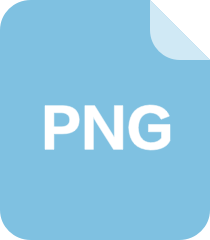

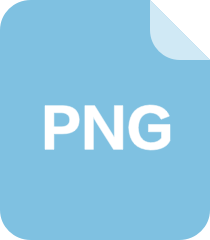

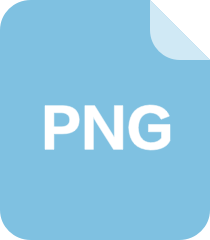
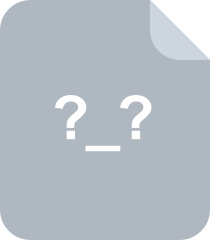

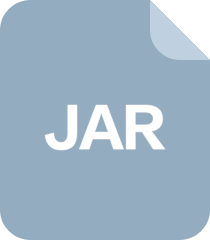




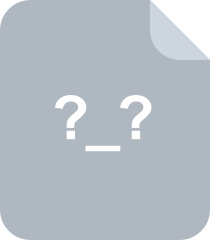
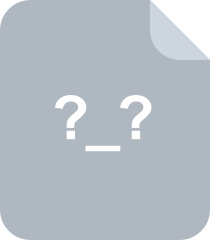
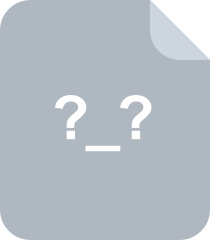
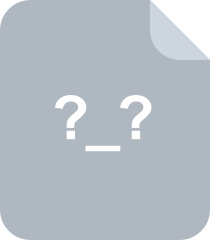
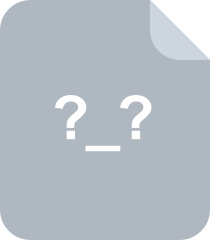
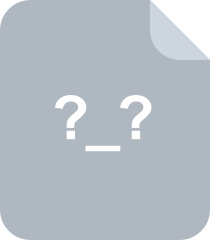
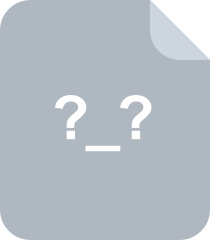
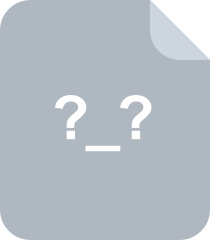
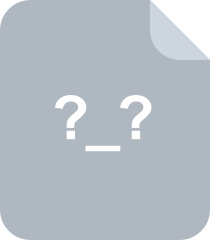
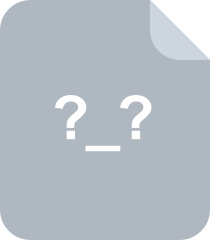
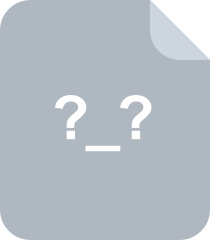
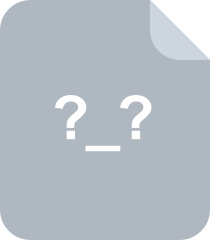
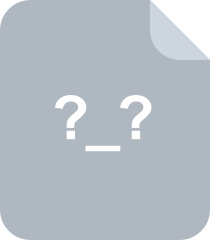
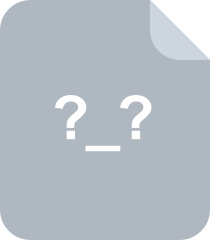
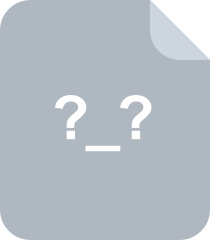
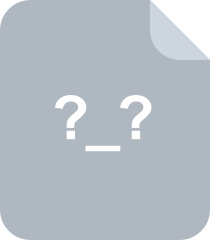
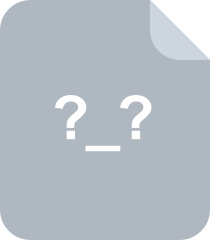
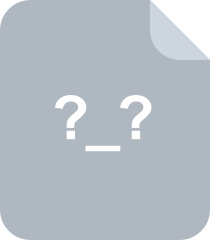
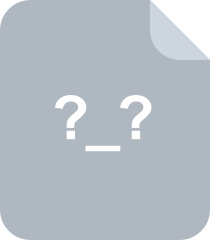
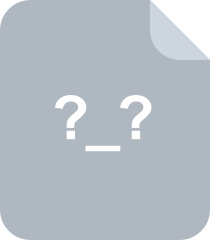
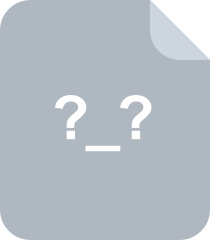
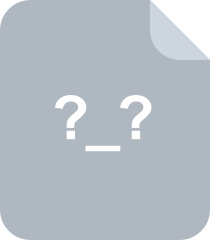



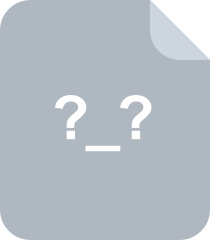

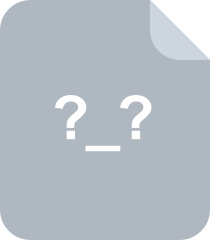

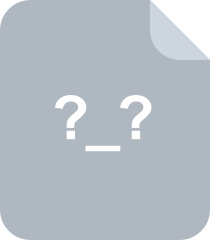

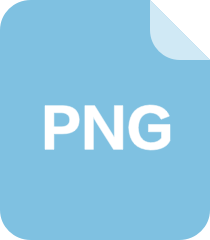
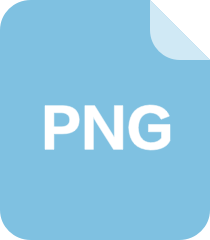
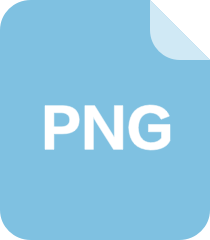
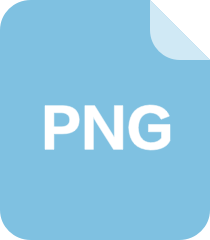

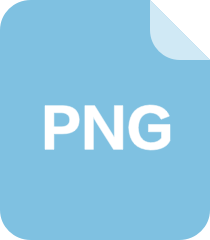

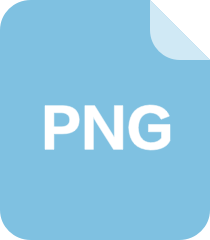

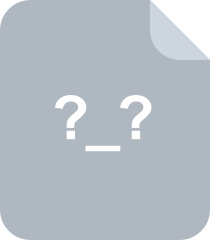
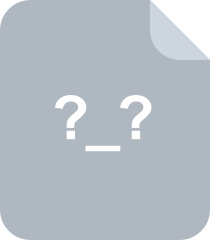
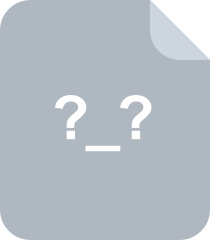

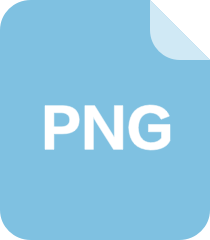

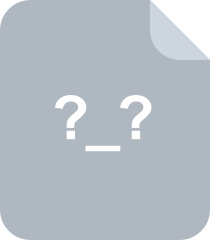
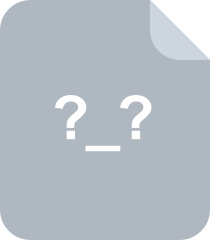

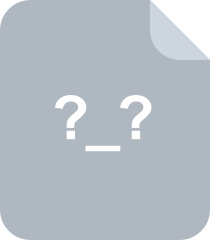
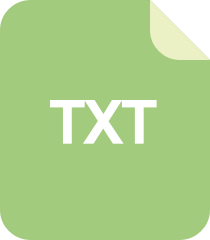
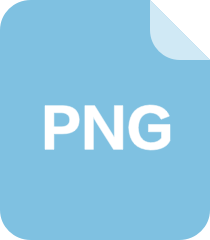





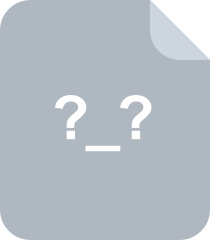
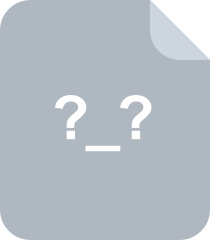




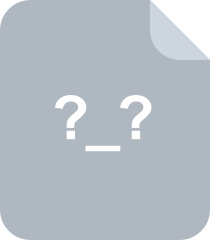
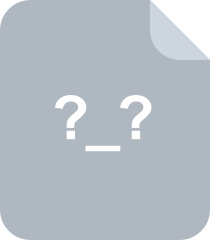
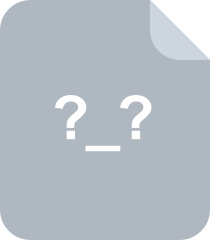
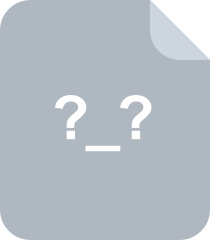
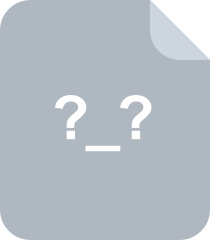
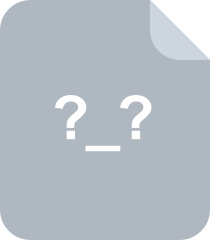

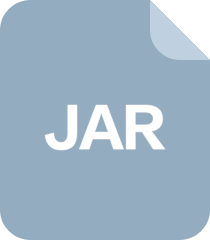
共 59 条
- 1
资源评论
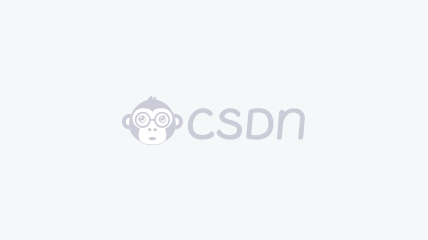

永不成熟的青春
- 粉丝: 1
- 资源: 2
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

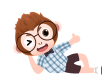
安全验证
文档复制为VIP权益,开通VIP直接复制
