/*
* Copyright (c) 2013 - 2015, Freescale Semiconductor, Inc.
* Copyright 2016-2017 NXP
* All rights reserved.
*
* THIS SOFTWARE IS PROVIDED BY NXP "AS IS" AND ANY EXPRESSED OR
* IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES
* OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED.
* IN NO EVENT SHALL NXP OR ITS CONTRIBUTORS BE LIABLE FOR ANY DIRECT,
* INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
* (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR
* SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION)
* HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT,
* STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING
* IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF
* THE POSSIBILITY OF SUCH DAMAGE.
*/
/**
* @page misra_violations MISRA-C:2012 violations
*
* @section [global]
* Violates MISRA 2012 Required Rule 1.3, There shall be no occurrence of
* undefined or critical unspecified behaviour.
* The addresses of the stack variables are only used at local scope.
*
* @section [global]
* Violates MISRA 2012 Required Rule 10.3, Expression assigned to a narrower or different essential type.
* The cast is required to perform a conversion between an unsigned integer and an enum type.
*
* @section [global]
* Violates MISRA 2012 Required Rule 11.6, A cast shall not be performed
* between pointer to void and an arithmetic type.
* The base address parameter from HAL functions is provided as integer so
* it needs to be cast to pointer.
*
* @section [global]
* Violates MISRA 2012 Advisory Rule 11.4, A conversion should not be performed
* between a pointer to object and an integer type.
* The base address parameter from HAL functions is provided as integer so
* a conversion between a pointer and an integer has to be performed
*
* @section [global]
* Violates MISRA 2012 Advisory Rule 8.7, External could be made static.
* Function is defined for usage by application code.
*
* @section [global]
* Violates MISRA 2012 Required Rule 8.4, external symbol defined without a prior
* declaration.
* It's temporary until CLOCK_DRV_Init function will be declared
*/
#include "device_registers.h"
#include "sim_hw_access.h"
#include "scg_hw_access.h"
#include "pcc_hw_access.h"
#include "pmc_hw_access.h"
#include "smc_hw_access.h"
#include "clock_manager.h"
#include <stddef.h> /* This header is included for bool type */
/*
* README:
* This file provides these APIs:
* 1. APIs to get the frequency of output clocks in Reference Manual ->
* Chapter Clock Distribution -> Figure Clocking diagram.
* 2. APIs for IP modules listed in Reference Manual -> Chapter Clock Distribution
* -> Module clocks.
*/
/*******************************************************************************
* Definitions
******************************************************************************/
/* This frequency values should be set by different boards. */
/* SIM */
uint32_t g_TClkFreq[NUMBER_OF_TCLK_INPUTS]; /* TCLKx clocks */
/* RTC */
uint32_t g_RtcClkInFreq; /* RTC CLKIN clock */
/* SCG */
uint32_t g_xtal0ClkFreq; /* EXTAL0 clock */
/* @brief System PLL base multiplier value, it is the multiplier value when SCG_SPLLCFG[MULT]=0. */
#define SCG_SPLL_MULT_BASE 16U
/*
* @brief System PLL base divider value, it is the PLL reference clock divider value when
* SCG_SPLLCFG[PREDIV]=0.
*/
#define SCG_SPLL_PREDIV_BASE 1U
/*
* @brief System PLL reference clock after SCG_SPLLCFG[PREDIV] should be in the range of
* SCG_SPLL_REF_MIN to SCG_SPLL_REF_MAX.
*/
#define SCG_SPLL_REF_MIN 8000000U
/*
* @brief System PLL reference clock after SCG_SPLLCFG[PREDIV] should be in the range of
* SCG_SPLL_REF_MIN to SCG_SPLL_REF_MAX.
*/
#define SCG_SPLL_REF_MAX 32000000U
/*
* @brief LPO 128K fixed clock frequency.
*/
#define LPO_128K_FREQUENCY 128000UL
/*
* @brief LPO 32K fixed clock frequency.
*/
#define LPO_32K_FREQUENCY 32000UL
/*
* @brief LPO 1K fixed clock frequency.
*/
#define LPO_1K_FREQUENCY 1000UL
/*
* @brief Running modes.
*/
#define HIGH_SPEED_RUNNING_MODE (1UL << 7U)
#define RUN_SPEED_RUNNING_MODE (1UL << 0U)
#define VLPR_SPEED_RUNNING_MODE (1UL << 2U)
/*
* @brief Number of peripheral clocks.
*/
#if defined(PCC_FTFC_INDEX)
#define TMP_FTFC 1U
#else
#define TMP_FTFC 0U
#endif
#if defined(PCC_DMAMUX_INDEX)
#define TMP_DMAMUX 1U
#else
#define TMP_DMAMUX 0U
#endif
#if defined(PCC_FlexCAN0_INDEX)
#define TMP_FlexCAN0 1U
#else
#define TMP_FlexCAN0 0U
#endif
#if defined(PCC_FlexCAN1_INDEX)
#define TMP_FlexCAN1 1U
#else
#define TMP_FlexCAN1 0U
#endif
#if defined(PCC_FTM3_INDEX)
#define TMP_FTM3 1U
#else
#define TMP_FTM3 0U
#endif
#if defined(PCC_ADC1_INDEX)
#define TMP_ADC1 1U
#else
#define TMP_ADC1 0U
#endif
#if defined(PCC_FlexCAN2_INDEX)
#define TMP_FlexCAN2 1U
#else
#define TMP_FlexCAN2 0U
#endif
#if defined(PCC_LPSPI0_INDEX)
#define TMP_LPSPI0 1U
#else
#define TMP_LPSPI0 0U
#endif
#if defined(PCC_LPSPI1_INDEX)
#define TMP_LPSPI1 1U
#else
#define TMP_LPSPI1 0U
#endif
#if defined(PCC_LPSPI2_INDEX)
#define TMP_LPSPI2 1U
#else
#define TMP_LPSPI2 0U
#endif
#if defined(PCC_PDB1_INDEX)
#define TMP_PDB1 1U
#else
#define TMP_PDB1 0U
#endif
#if defined(PCC_CRC_INDEX)
#define TMP_CRC 1U
#else
#define TMP_CRC 0U
#endif
#if defined(PCC_PDB0_INDEX)
#define TMP_PDB0 1U
#else
#define TMP_PDB0 0U
#endif
#if defined(PCC_LPIT_INDEX)
#define TMP_LPIT 1U
#else
#define TMP_LPIT 0U
#endif
#if defined(PCC_FTM0_INDEX)
#define TMP_FTM0 1U
#else
#define TMP_FTM0 0U
#endif
#if defined(PCC_FTM1_INDEX)
#define TMP_FTM1 1U
#else
#define TMP_FTM1 0U
#endif
#if defined(PCC_FTM2_INDEX)
#define TMP_FTM2 1U
#else
#define TMP_FTM2 0U
#endif
#if defined(PCC_ADC0_INDEX)
#define TMP_ADC0 1U
#else
#define TMP_ADC0 0U
#endif
#if defined(PCC_RTC_INDEX)
#define TMP_RTC 1U
#else
#define TMP_RTC 0U
#endif
#if defined(PCC_LPTMR0_INDEX)
#define TMP_LPTMR0 1U
#else
#define TMP_LPTMR0 0U
#endif
#if defined(PCC_PORTA_INDEX)
#define TMP_PORTA 1U
#else
#define TMP_PORTA 0U
#endif
#if defined(PCC_PORTB_INDEX)
#define TMP_PORTB 1U
#else
#define TMP_PORTB 0U
#endif
#if defined(PCC_PORTC_INDEX)
#define TMP_PORTC 1U
#else
#define TMP_PORTC 0U
#endif
#if defined(PCC_PORTD_INDEX)
#define TMP_PORTD 1U
#else
#define TMP_PORTD 0U
#endif
#if defined(PCC_PORTE_INDEX)
#define TMP_PORTE 1U
#else
#define TMP_PORTE 0U
#endif
#if defined(PCC_SAI0_INDEX)
#define TMP_SAI0 1U
#else
#define TMP_SAI0 0U
#endif
#if defined(PCC_SAI1_INDEX)
#define TMP_SAI1 1U
#else
#define TMP_SAI1 0U
#endif
#if defined(PCC_FlexIO_INDEX)
#define TMP_FlexIO 1U
#else
#define TMP_FlexIO 0U
#endif
#if defined(PCC_EWM_INDEX)
#define TMP_EWM 1U
#else
#define TMP_EWM 0U
#endif
#if defined(PCC_LPI2C0_INDEX)
#define TMP_LPI2C0 1U
#else
#define TMP_LPI2C0 0U
#endif
#if defined(PCC_LPI2C1_INDEX)
#define TMP_LPI2C1 1U
#else
#define TMP_LPI2C1 0U
#endif
#if defined(PCC_LPUART0_INDEX)
#define TMP_LPUART0 1U
#else
#define TMP_LPUART0 0U
#endif
#if defined(PCC_LPUART1_INDEX)
#define TMP_LPUART1 1U
#else
#define TMP_LPUART1 0U
#endif
#if defined(PCC_LPUART2_INDEX)
#define TMP_LPUART2 1U
#else
#define TMP_LPUART2 0U
#endif
#if defined(PCC_FTM4_INDEX)
#define TMP_FTM4 1U
#else
#define TMP_FTM4 0U
#endif
#if defined(PCC_FTM5_INDEX)
#define TMP_FTM5 1U
#else
#define TMP_FTM5 0U
#endif
#if defined(PCC_FTM6_INDEX)
#define TMP_FTM6 1U
#else
#define TMP_FTM6 0U
#endif
#if defined(PCC_FTM7_INDEX)
#define TMP_FTM7 1U
#else
#define TMP_FTM7 0U
#endif
#if defined(PCC_CMP0_INDEX)
#define TMP_CMP0 1U
#else
#define TMP_CMP0 0U
#endif
#if defined(PCC_QSPI_INDEX)
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
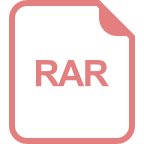
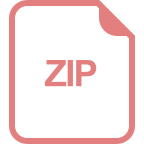
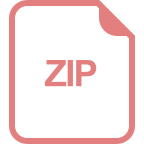
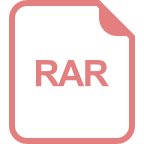
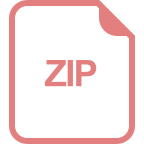
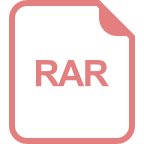
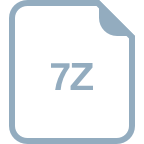
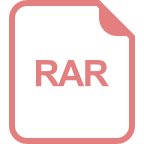
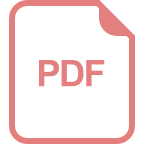
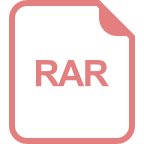
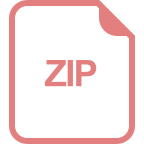
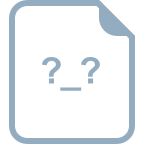
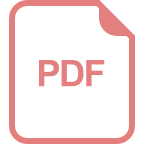
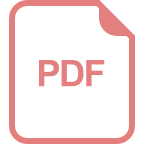
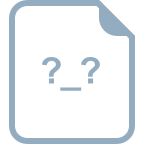
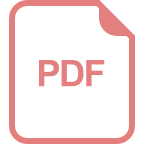
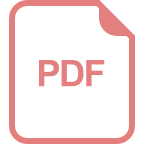
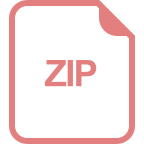
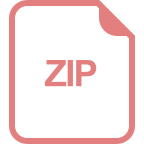
收起资源包目录

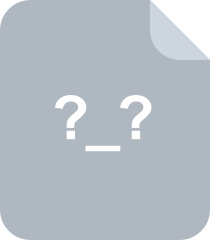
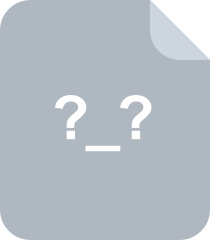
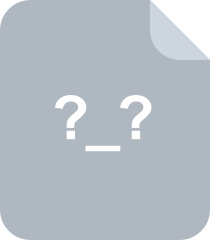
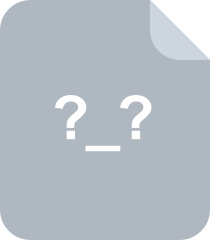
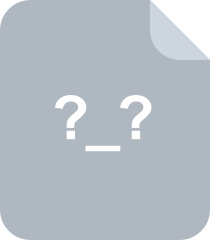
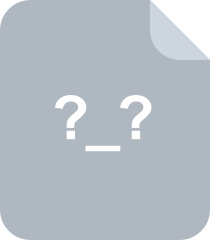
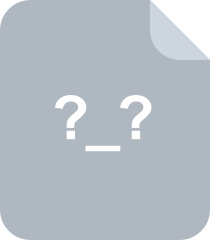
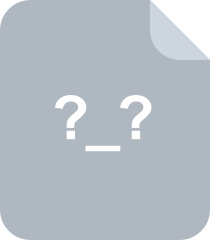
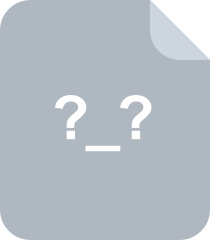
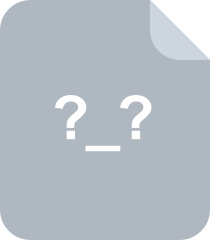
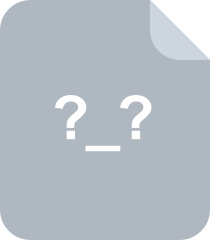
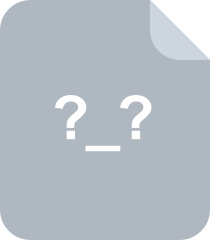
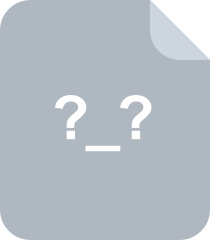
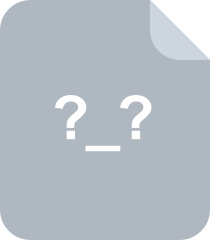
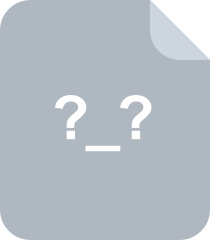
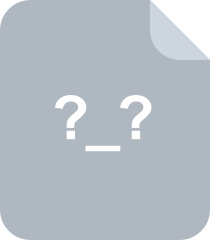
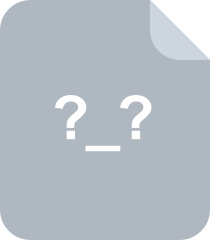
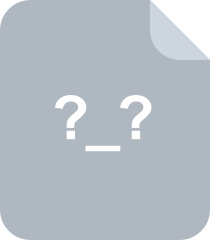
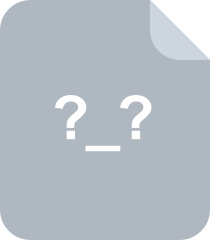
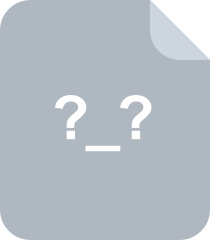
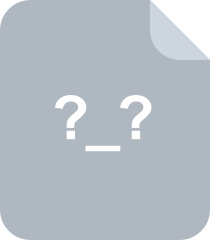
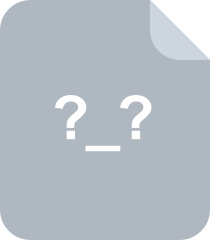
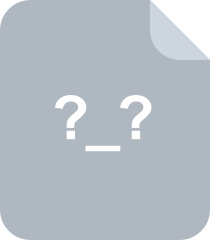
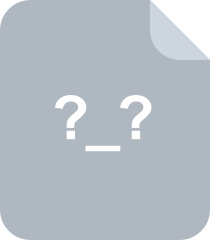
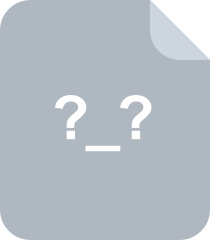
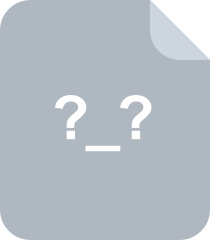
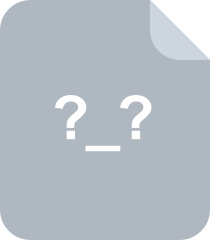
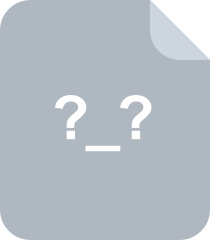
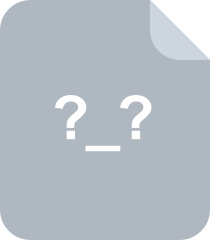
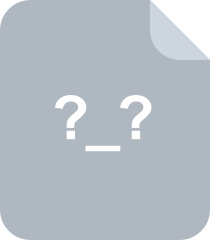
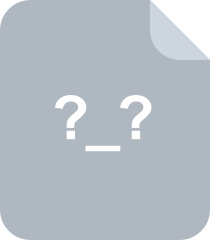
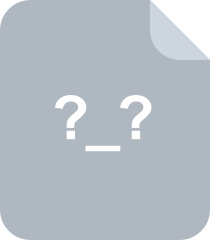
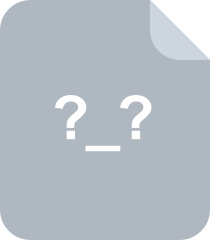
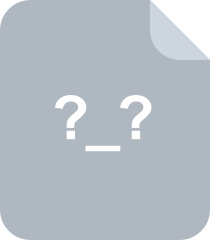
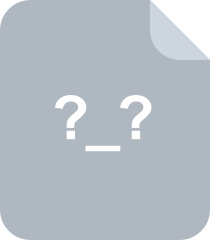
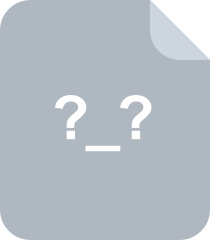
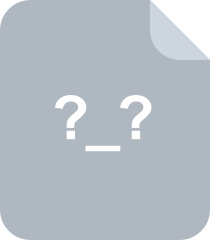
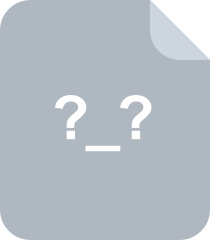
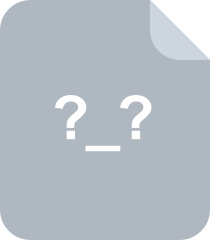
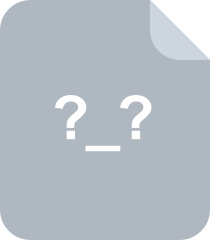
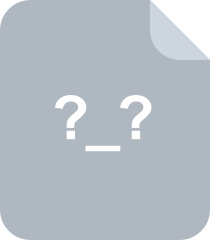
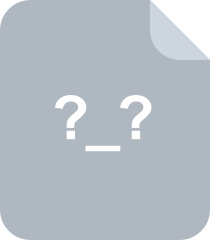
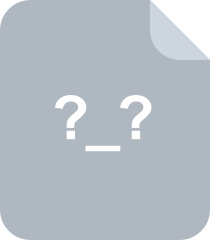
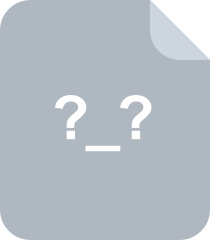
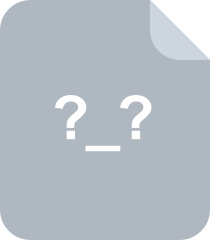
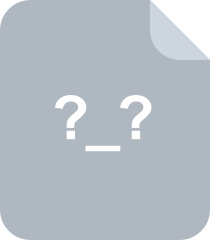
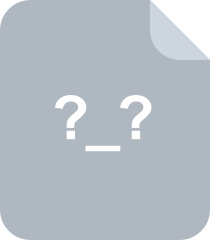
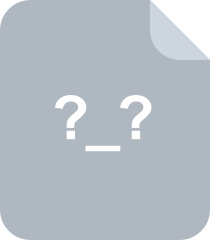
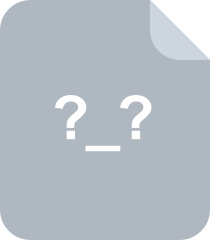
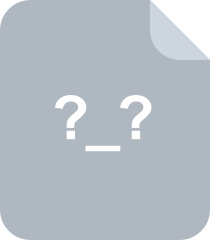
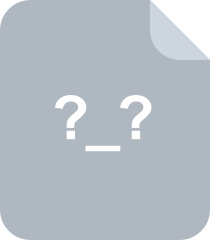
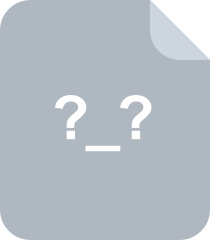
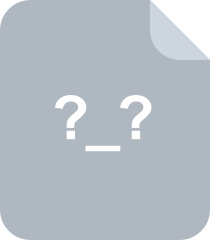
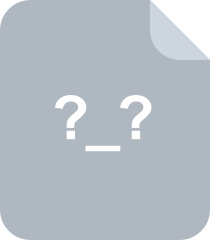
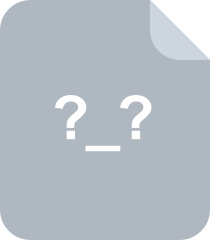
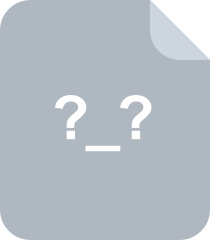
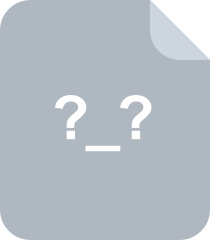
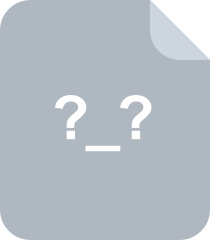
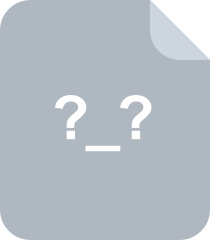
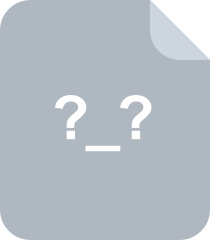
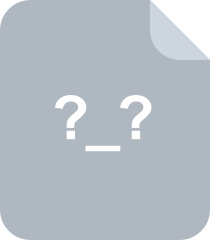
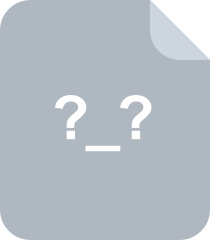
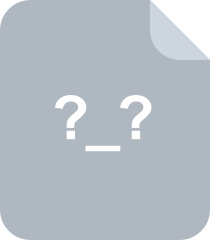
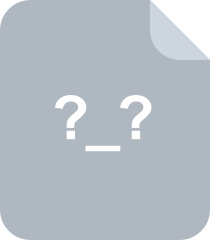
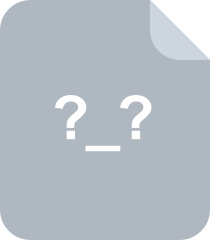
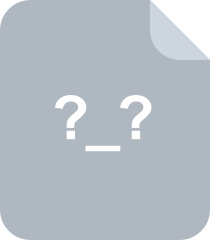
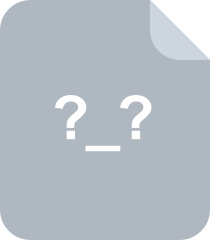
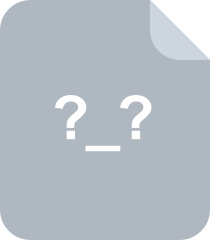
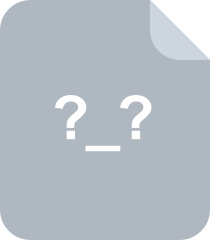
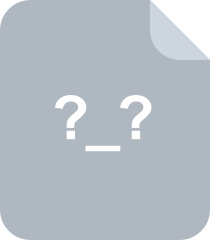
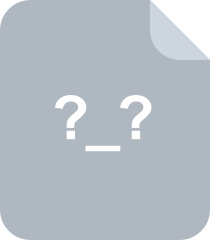
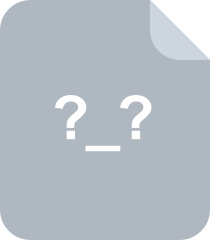
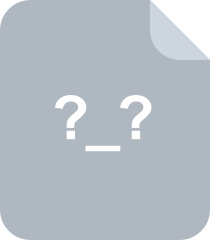
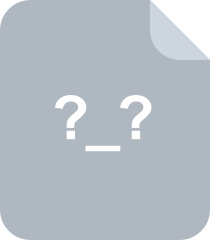
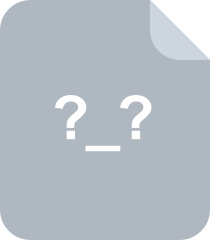
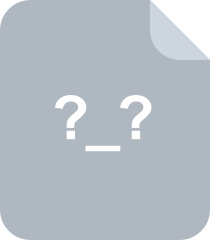
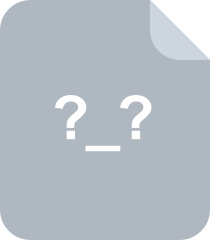
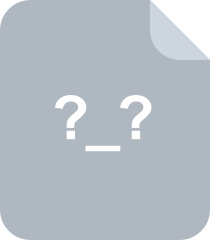
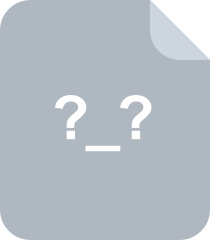
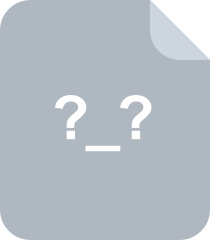
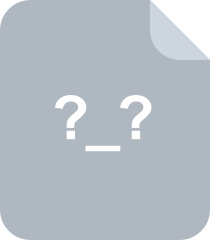
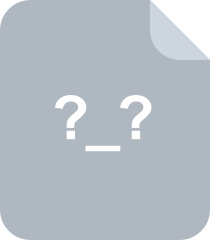
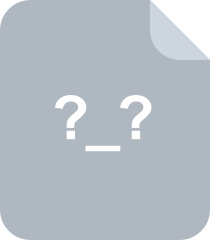
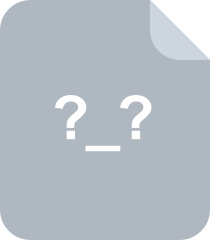
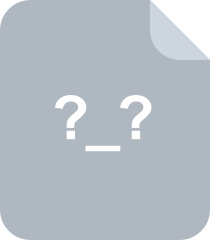
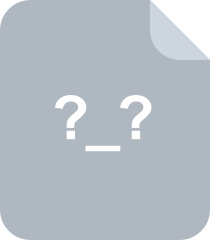
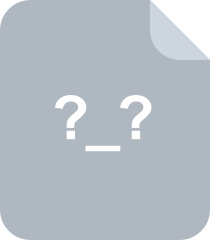
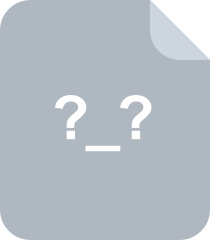
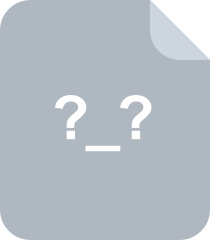
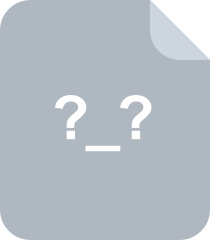
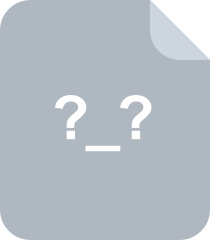
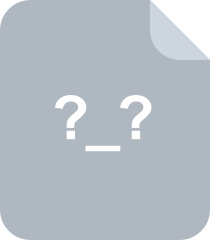
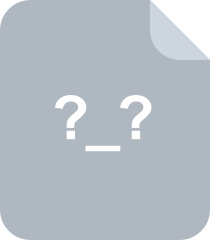
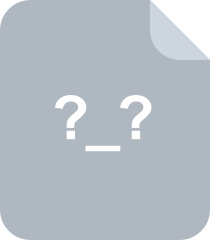
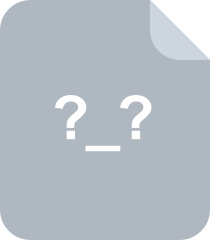
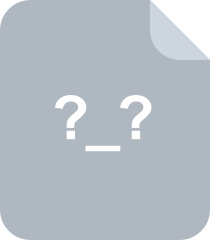
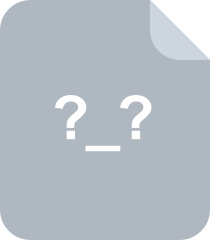
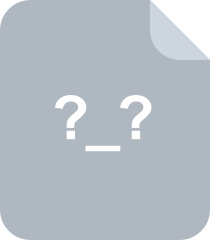
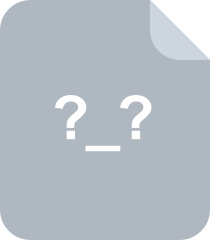
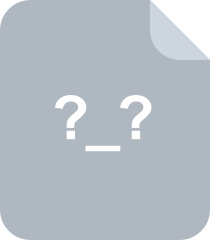
共 376 条
- 1
- 2
- 3
- 4
资源评论
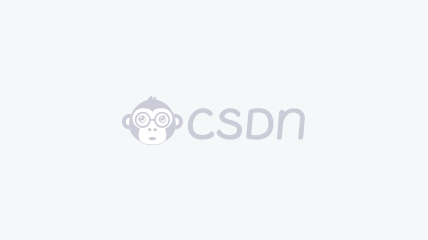

jorelxu
- 粉丝: 3
- 资源: 38
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

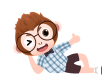
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


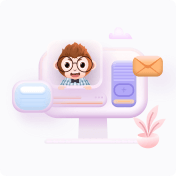
安全验证
文档复制为VIP权益,开通VIP直接复制
