#include "stdio.h"
#define MAX_BUF_LEN 512
char buf[MAX_BUF_LEN];
void set_buf()
{
int bufpoint=0;
while(1)
{
char c=get_the_char();
if(c=='\b')
{
if(bufpoint==0)
continue;
putch('\b');
putch(' ');
putch('\b');
bufpoint--;
}
else if(c==10||c==13)
{
putch(10);
buf[bufpoint]=0;
bufpoint++;
break;
}
else
{
putch(c);
buf[bufpoint]=c;
bufpoint++;
}
if(bufpoint==MAX_BUF_LEN-1)
{
buf[bufpoint]=0;
break;
}
}
}
void putch(char c)
{
int position=get_cursor();
if(c==13)
c=10;
if(c==10)
{
int currow=position/80;
position=80*currow;
position+=80;
}
else
{
put_the_char(c,position);
position++;
}
if(position>1999)
{
roll_screen();
position=1920;
}
set_curson(position);
}
int get_cursor()
{
int position;
asm push ax
asm push dx
asm mov dx,3d4h
asm mov al,0eh
asm out dx,al
asm mov dx,3d5h
asm in al,dx
asm mov ah,al
asm mov dx,3d4h
asm mov al,0fh
asm out dx,al
asm mov dx,3d5h
asm in al,dx
asm mov position,ax
asm pop dx
asm pop ax
return position;
}
void set_curson(int position)
{
asm push ax
asm push bx
asm push dx
asm mov bx,position
asm mov dx,3d4h
asm mov al,0eh
asm out dx,al
asm mov dx,3d5h
asm mov al,bh
asm out dx,al
asm mov dx,3d4h
asm mov al,0fh
asm out dx,al
asm mov dx,3d5h
asm mov al,bl
asm out dx,al
asm pop dx
asm pop bx
asm pop ax
}
void put_the_char(char c,int position)
{
position*=2;
asm push ax
asm push cx
asm push bx
asm push ds
asm push si
asm mov cl,c
asm mov ax,0b800h
asm mov ds,ax
asm mov bx,position
asm mov si,bx
asm mov byte ptr [si],cl
asm pop si
asm pop ds
asm pop bx
asm pop cx
asm pop ax
}
void roll_screen()
{
asm push ax
asm push ds
asm push es
asm push si
asm push di
asm push cx
asm push bx
asm mov ax,0xb800
asm mov ds,ax
asm mov es,ax
asm cld
asm mov si,0xa0
asm mov di,0x00
asm mov cx,1920
asm rep movsw
asm mov bx,3840
asm mov cx,80
last_row_cls:
asm mov word ptr [bx],0x0720
asm add bx,2
asm loop last_row_cls
asm pop bx
asm pop cx
asm pop di
asm pop si
asm pop es
asm pop ds
asm pop ax
}
void puts(char* str)
{
while(*str)
{
putch(*str);
str++;
}
}
char getch()
{
char c;
char curchar;
while(1)
{
curchar=get_the_char();
if(curchar==32||curchar==10||curchar==13)
{
putch(curchar);
continue;
}
else
{
putch(curchar);
c=curchar;
break;
}
}
return c;
}
char get_the_char()
{
char the_char;
asm mov ah,0
asm int 16h
asm mov the_char, al
return the_char;
}
void gets(char* str)
{
char curchar;
while(1)
{
curchar=get_the_char();
putch(curchar);
if(curchar!=32&&curchar!=10&&curchar!=13)
break;
}
(*str)=curchar;
str++;
while(1)
{
curchar=get_the_char();
putch(curchar);
if(curchar!=10&&curchar!=13)
{
(*str)=curchar;
str++;
}
else
break;
}
(*str)=0;
}
void printfint(int num)
{
char buffer[8];
int point=0;
if(num==0)
{
putch('0');
}
else
{
while(num)
{
buffer[point]=(num%10)+'0';
num/=10;
point++;
}
while(point)
{
putch(buffer[point-1]);
point--;
}
}
}
void printf(char* format, ...)
{
int stackptr =(&format);
int data;
while(*format)
{
if((*format)=='%')
{
format++;
stackptr+=2;
asm push ax
asm push bp
asm mov ax,word ptr stackptr
asm mov bp,ax
asm mov ax,word ptr [bp]
asm pop bp
asm mov word ptr data,ax
asm pop ax
if((*format)=='c')
{
char c=(char )data;
putch(c);
}
else if((*format)=='d')
{
printfint(data);
}
else if((*format)=='s')
{
char* s=(char* )data;
puts(s);
}
else
{
format--;
stackptr-=2;
}
}
else
{
putch(*format);
}
format++;
}
}
void scanf(char* format, ...)
{
int stackptr=(&format);
int* data;
char* temp;
int pos;
pos=0;
set_buf();
while((*format))
{
if((*format)=='%')
{
format++;
stackptr+=2;
asm push ax
asm push bp
asm mov ax,word ptr stackptr
asm mov bp,ax
asm mov ax,word ptr [bp]
asm pop bp
asm mov word ptr data,ax
asm pop ax
if((*format)=='c')
{
while(pos<MAX_BUF_LEN&&buf[pos])
{
if(buf[pos]!=32&&buf[pos]!=10&&buf[pos]!=13)
break;
pos++;
}
temp=(char* )data;
(*temp)=buf[pos];
pos++;
}
else if((*format)=='d')
{
int num=0;
while(buf[pos]>'9'||buf[pos]<'0')
{
pos++;
}
while(buf[pos]>='0'&&buf[pos]<='9')
{
num=num*10+buf[pos]-'0';
pos++;
}
(*data)=num;
}
else if((*format)=='s')
{
while(buf[pos]==32||buf[pos]==10||buf[pos]==13)
{
pos++;
}
temp=(char* )data;
while(pos<MAX_BUF_LEN&&buf[pos]!=0&&buf[pos]!=32&&buf[pos]!=10&&buf[pos]!=13)
{
(*temp)=buf[pos];
temp++;
pos++;
}
(*temp)=0;
}
else
{
format--;
stackptr-=2;
}
}
format++;
}
}

log16
- 粉丝: 17
- 资源: 12
最新资源
- Cocos2d-x 2.2.1 版本资源
- 关于DeepSeek的几点思考.pdf
- 基于ISODATA算法优化的负荷场景曲线聚类方法(包含K-means、L-ISODATA及K-L-ISODATA算法,聚类效果评价与风光场景应用),基于ISODATA改进算法的负荷场景曲线聚类:多方法
- qpress的二进制命令
- 基于Matlab Simulink的风光储并网双闭环控制策略及单极调制协同运行模型研究,基于Matlab Simulink的风光储并网双闭环控制策略及其单极调制协同运行模型研究,风光储并网协同运行模型
- deepseek详细对话
- 遗传算法与动态窗口法DWA融合优化:全局路径规划与动态环境适应的算法创新,遗传算法优化与动态窗口法融合:全局最优路径规划与动态环境适应策略,遗传算法(GA)优化与动态窗口法dwa融合,效果极佳,算法新
- COMSOL仿真揭示变压器磁致伸缩现象:电路磁场分布、振动噪声及受力和噪声分布研究,COMSOL仿真揭示变压器磁致伸缩机理:电路磁场分布、振动数据与噪声分布综合分析,COMSOL仿真,变压器磁致伸缩
- 数据科学相关人员的岗位及薪资数据.zip
- 零基础入门转录组下游分析-机器学习算法之xgboost(筛选特征基因)教程配套资源
- 基于相量模型的UPFC在500kV与230kV输电系统中的功率流控仿真研究,基于相量模型的UPFC在500kV与230kV输电系统中的功率流控仿真研究,UPFC(相量模型)控制500 kV 230
- 中秋节静态网站网页设计(HTML+CSS+JS)期末大作业.zip
- C#开发TCP通信demo
- 基于Electron+HTML+CSS+Node.js开发的的中国象棋游戏,支持人机对大模型AI对战 (源码)
- 基于粒子群算法的地表水源热泵系统建模与最佳制冷制热量求解研究,水源热泵系统建模与粒子群优化:求解热泵机组最佳制冷制热量算法研究,matlab代码 从水源热泵机组角度对地表水源热泵系统建模,并采用粒子群
- COCOS2DX 2.2.2 引擎下载
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


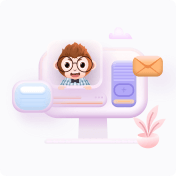