ServiceDemo项目中任意界面控制service的开启与停止
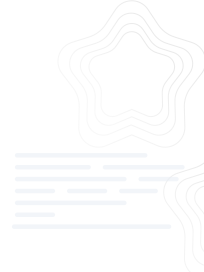

在Android应用开发中,Service是实现后台长时间运行任务的关键组件,它可以脱离用户界面独立执行。在"ServiceDemo"项目中,我们关注的核心是如何在任意界面控制Service的启动和停止,这是提升用户体验和优化应用程序资源管理的重要技能。下面将详细介绍如何实现这一功能。 创建一个Service。在Android Studio中,右键点击`app/src/main/java/your_package_name`,选择New -> Service -> Service,命名为`MyService`。在`MyService.java`中,我们需要重写`onStartCommand()`方法,这是Service生命周期中的关键方法,它会接收`Intent`并返回一个标志,表明Service应该如何响应。 ```java public class MyService extends Service { @Override public int onStartCommand(Intent intent, int flags, int startId) { // 在这里执行你的后台任务 return super.onStartCommand(intent, flags, startId); } @Override public void onDestroy() { // 当Service被停止时,这里可以进行清理工作 super.onDestroy(); } // 必须提供默认的Binder实例,以便其他组件(如Activity)能与Service交互 @Nullable @Override public IBinder onBind(Intent intent) { return null; } } ``` 接下来,我们需要在任意界面启动和停止Service。在Activity中,我们可以通过`startService()`和`stopService()`方法来实现。 ```java // 启动Service Intent serviceIntent = new Intent(this, MyService.class); startService(serviceIntent); // 停止Service Intent stopIntent = new Intent(this, MyService.class); stopService(stopIntent); ``` 为了能在任何界面控制Service,我们需要创建一个BroadcastReceiver。这个BroadcastReceiver会在接收到特定广播时启动或停止Service。创建一个新的BroadcastReceiver类,如`ServiceControlReceiver.java`: ```java public class ServiceControlReceiver extends BroadcastReceiver { @Override public void onReceive(Context context, Intent intent) { String action = intent.getAction(); if (action.equals("START_SERVICE")) { Intent serviceIntent = new Intent(context, MyService.class); context.startService(serviceIntent); } else if (action.equals("STOP_SERVICE")) { Intent serviceIntent = new Intent(context, MyService.class); context.stopService(serviceIntent); } } } ``` 然后,在任意界面发送广播以控制Service: ```java // 开启Service Intent startIntent = new Intent(); startIntent.setAction("START_SERVICE"); sendBroadcast(startIntent); // 停止Service Intent stopIntent = new Intent(); stopIntent.setAction("STOP_SERVICE"); sendBroadcast(stopIntent); ``` 别忘了在`AndroidManifest.xml`中注册Service和BroadcastReceiver: ```xml <service android:name=".MyService" /> <receiver android:name=".ServiceControlReceiver"> <intent-filter> <action android:name="START_SERVICE" /> <action android:name="STOP_SERVICE" /> </intent-filter> </receiver> ``` 此外,为了在任意界面都能够方便地启动和停止Service,可以考虑创建一个工具类`ServiceUtils`,提供静态方法来封装这些操作。这将使代码更整洁,更易于维护。 通过以上步骤,我们就能够在"ServiceDemo"项目中的任意界面控制Service的启动和停止。理解并熟练掌握这一技巧,对于开发高效、响应迅速的Android应用至关重要。同时,合理使用Service可以避免因应用被系统杀死而导致的后台任务中断,提高用户体验。
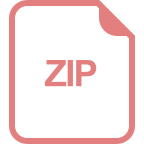
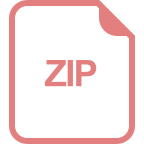
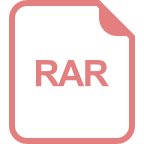
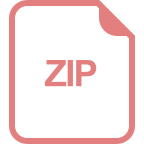
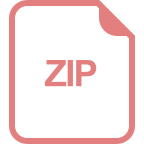
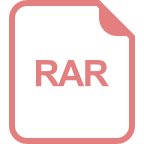
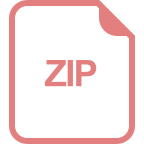
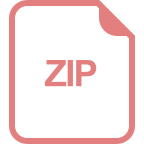
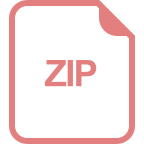
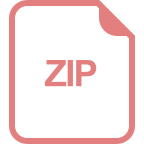
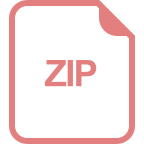
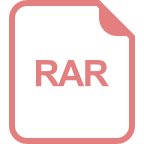
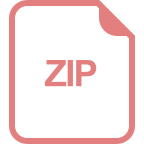
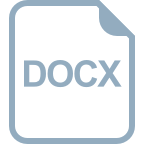
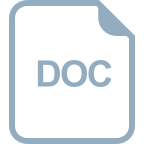


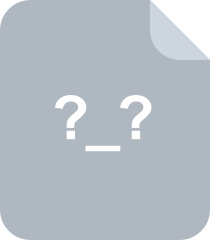
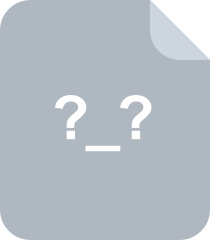
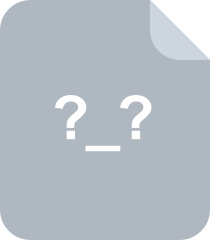




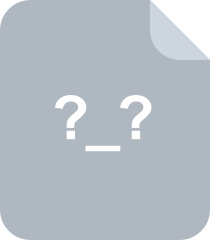
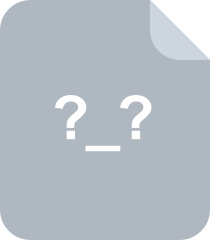
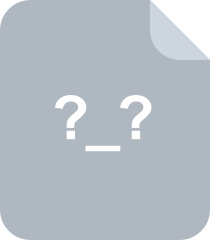
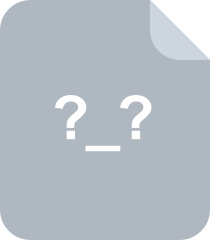
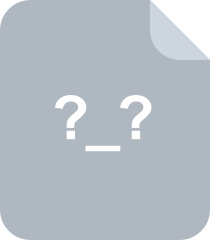



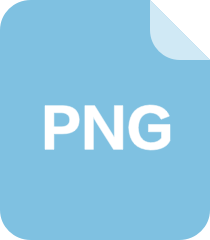

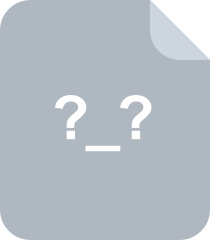

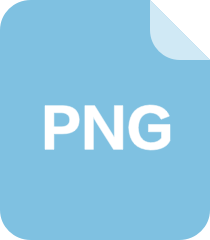

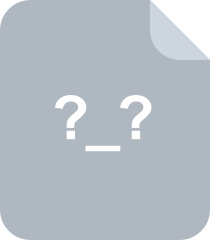
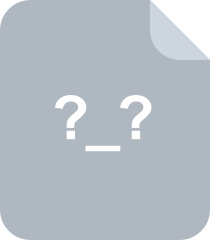

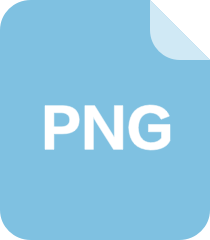

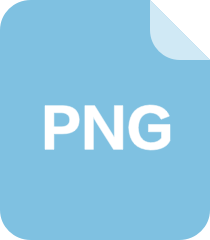

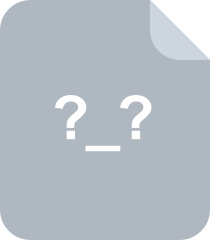
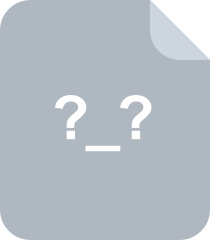

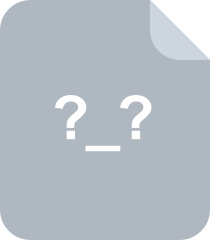





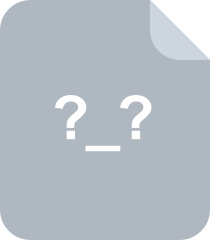
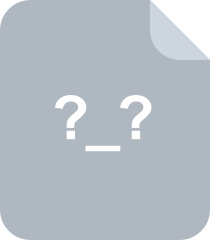

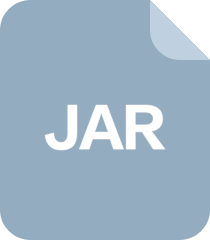
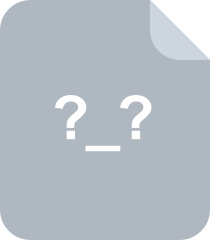
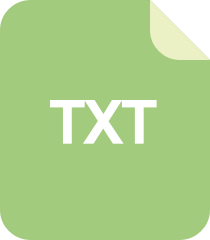
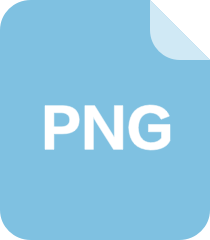





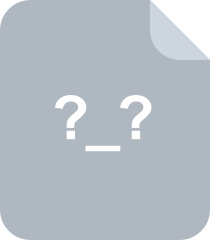
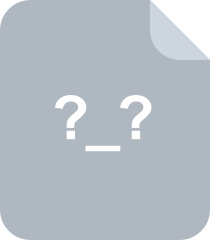
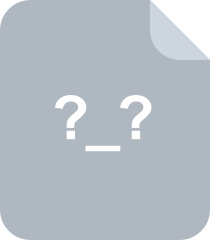
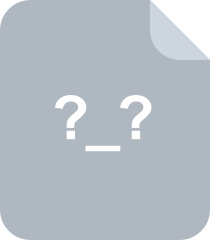
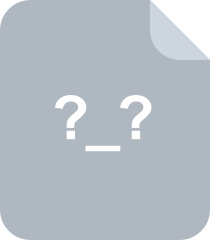
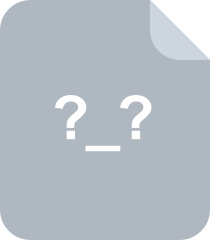
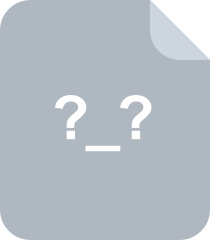
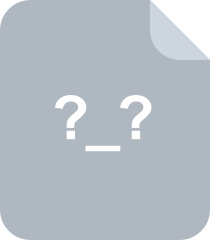
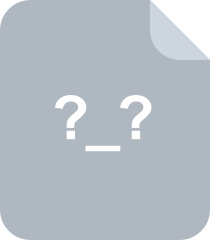
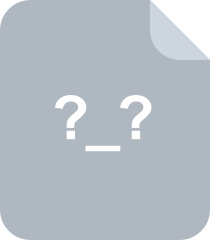
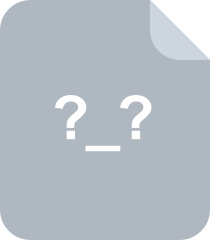
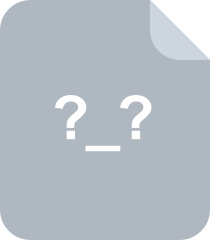
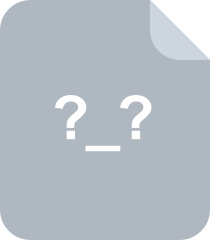
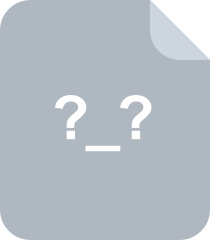
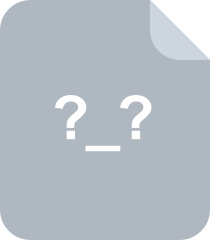
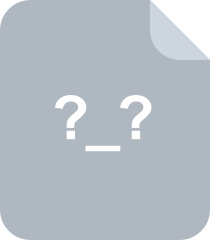
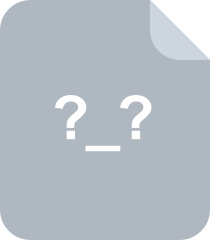

- 1
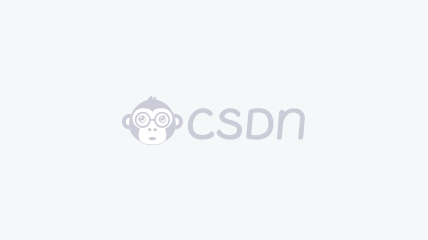

- 粉丝: 0
- 资源: 4
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

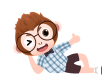
最新资源

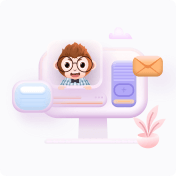
