package com.controller;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Calendar;
import java.util.Map;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Date;
import java.util.List;
import javax.servlet.http.HttpServletRequest;
import com.utils.ValidatorUtils;
import org.apache.commons.lang3.StringUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.transaction.annotation.Transactional;
import org.springframework.format.annotation.DateTimeFormat;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import com.baomidou.mybatisplus.mapper.EntityWrapper;
import com.baomidou.mybatisplus.mapper.Wrapper;
import com.annotation.IgnoreAuth;
import com.entity.XueshengEntity;
import com.entity.view.XueshengView;
import com.service.XueshengService;
import com.service.TokenService;
import com.utils.PageUtils;
import com.utils.R;
import com.utils.MD5Util;
import com.utils.MPUtil;
import com.utils.CommonUtil;
import java.io.IOException;
/**
* 学生
* 后端接口
* @author
* @email
* @date 2022-05-09 16:05:16
*/
@RestController
@RequestMapping("/xuesheng")
public class XueshengController {
@Autowired
private XueshengService xueshengService;
@Autowired
private TokenService tokenService;
/**
* 登录
*/
@IgnoreAuth
@RequestMapping(value = "/login")
public R login(String username, String password, String captcha, HttpServletRequest request) {
XueshengEntity user = xueshengService.selectOne(new EntityWrapper<XueshengEntity>().eq("xuehao", username));
if(user==null || !user.getMima().equals(password)) {
return R.error("账号或密码不正确");
}
String token = tokenService.generateToken(user.getId(), username,"xuesheng", "学生" );
return R.ok().put("token", token);
}
/**
* 注册
*/
@IgnoreAuth
@RequestMapping("/register")
public R register(@RequestBody XueshengEntity xuesheng){
//ValidatorUtils.validateEntity(xuesheng);
XueshengEntity user = xueshengService.selectOne(new EntityWrapper<XueshengEntity>().eq("xuehao", xuesheng.getXuehao()));
if(user!=null) {
return R.error("注册用户已存在");
}
Long uId = new Date().getTime();
xuesheng.setId(uId);
xueshengService.insert(xuesheng);
return R.ok();
}
/**
* 退出
*/
@RequestMapping("/logout")
public R logout(HttpServletRequest request) {
request.getSession().invalidate();
return R.ok("退出成功");
}
/**
* 获取用户的session用户信息
*/
@RequestMapping("/session")
public R getCurrUser(HttpServletRequest request){
Long id = (Long)request.getSession().getAttribute("userId");
XueshengEntity user = xueshengService.selectById(id);
return R.ok().put("data", user);
}
/**
* 密码重置
*/
@IgnoreAuth
@RequestMapping(value = "/resetPass")
public R resetPass(String username, HttpServletRequest request){
XueshengEntity user = xueshengService.selectOne(new EntityWrapper<XueshengEntity>().eq("xuehao", username));
if(user==null) {
return R.error("账号不存在");
}
user.setMima("123456");
xueshengService.updateById(user);
return R.ok("密码已重置为:123456");
}
/**
* 后端列表
*/
@RequestMapping("/page")
public R page(@RequestParam Map<String, Object> params,XueshengEntity xuesheng,
HttpServletRequest request){
String tableName = request.getSession().getAttribute("tableName").toString();
if(tableName.equals("jiaoshi")) {
xuesheng.setJiaoshigonghao((String)request.getSession().getAttribute("username"));
}
if(tableName.equals("yuanxifuzeren")) {
xuesheng.setYuanxizhanghao((String)request.getSession().getAttribute("username"));
}
EntityWrapper<XueshengEntity> ew = new EntityWrapper<XueshengEntity>();
PageUtils page = xueshengService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, xuesheng), params), params));
return R.ok().put("data", page);
}
/**
* 前端列表
*/
@IgnoreAuth
@RequestMapping("/list")
public R list(@RequestParam Map<String, Object> params,XueshengEntity xuesheng,
HttpServletRequest request){
EntityWrapper<XueshengEntity> ew = new EntityWrapper<XueshengEntity>();
PageUtils page = xueshengService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, xuesheng), params), params));
return R.ok().put("data", page);
}
/**
* 列表
*/
@RequestMapping("/lists")
public R list( XueshengEntity xuesheng){
EntityWrapper<XueshengEntity> ew = new EntityWrapper<XueshengEntity>();
ew.allEq(MPUtil.allEQMapPre( xuesheng, "xuesheng"));
return R.ok().put("data", xueshengService.selectListView(ew));
}
/**
* 查询
*/
@RequestMapping("/query")
public R query(XueshengEntity xuesheng){
EntityWrapper< XueshengEntity> ew = new EntityWrapper< XueshengEntity>();
ew.allEq(MPUtil.allEQMapPre( xuesheng, "xuesheng"));
XueshengView xueshengView = xueshengService.selectView(ew);
return R.ok("查询学生成功").put("data", xueshengView);
}
/**
* 后端详情
*/
@RequestMapping("/info/{id}")
public R info(@PathVariable("id") Long id){
XueshengEntity xuesheng = xueshengService.selectById(id);
return R.ok().put("data", xuesheng);
}
/**
* 前端详情
*/
@IgnoreAuth
@RequestMapping("/detail/{id}")
public R detail(@PathVariable("id") Long id){
XueshengEntity xuesheng = xueshengService.selectById(id);
return R.ok().put("data", xuesheng);
}
/**
* 后端保存
*/
@RequestMapping("/save")
public R save(@RequestBody XueshengEntity xuesheng, HttpServletRequest request){
xuesheng.setId(new Date().getTime()+new Double(Math.floor(Math.random()*1000)).longValue());
//ValidatorUtils.validateEntity(xuesheng);
XueshengEntity user = xueshengService.selectOne(new EntityWrapper<XueshengEntity>().eq("xuehao", xuesheng.getXuehao()));
if(user!=null) {
return R.error("用户已存在");
}
xuesheng.setId(new Date().getTime());
xueshengService.insert(xuesheng);
return R.ok();
}
/**
* 前端保存
*/
@RequestMapping("/add")
public R add(@RequestBody XueshengEntity xuesheng, HttpServletRequest request){
xuesheng.setId(new Date().getTime()+new Double(Math.floor(Math.random()*1000)).longValue());
//ValidatorUtils.validateEntity(xuesheng);
XueshengEntity user = xueshengService.selectOne(new EntityWrapper<XueshengEntity>().eq("xuehao", xuesheng.getXuehao()));
if(user!=null) {
return R.error("用户已存在");
}
xuesheng.setId(new Date().getTime());
xueshengService.insert(xuesheng);
return R.ok();
}
/**
* 修改
*/
@RequestMapping("/update")
@Transactional
public R update(@RequestBody XueshengEntity xuesheng, HttpServletRequest request){
//ValidatorUtils.validateEntity(xuesheng);
xueshengService.updateById(xuesheng);//全部更新
return R.ok();
}
/**
* 删除
*/
@RequestMapping("/delete")
public R delete(@RequestBody Long[] ids){
xueshengService.deleteBatchIds(Arrays.asList(ids));
return R.ok();
}
/**
* 提醒�
没有合适的资源?快使用搜索试试~ 我知道了~
Java项目-基于springboot+Vue的高校专业实习管理系统的设计和开发(附源码,数据库,教程).zip
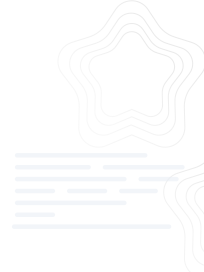
共526个文件
java:171个
svg:161个
vue:59个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 132 浏览量
2024-03-06
14:35:56
上传
评论
收藏 9.69MB ZIP 举报
温馨提示
Java 毕业设计,Java 课程设计,基于 SpringBoot 开发的,含有代码注释,新手也可看懂。毕业设计、期末大作业、课程设计、高分必看,下载下来,简单部署,就可以使用。 包含:项目源码、数据库脚本、软件工具等,前后端代码都在里面。 该系统功能完善、界面美观、操作简单、功能齐全、管理便捷,具有很高的实际应用价值。 项目都经过严格调试,确保可以运行! 1. 技术组成 前端:html/javascript 后台框架:SpringBoot 开发环境:idea 数据库:MySql(建议用 5.7 版本,8.0 有时候会有坑) 数据库工具:navicat 部署环境:Tomcat(建议用 7.x 或者 8.x 版本), maven
资源推荐
资源详情
资源评论
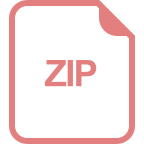
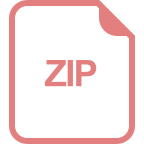
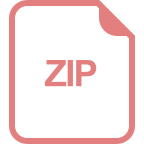
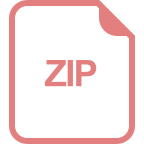
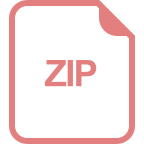
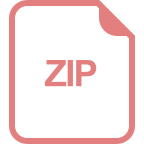
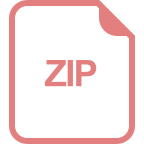
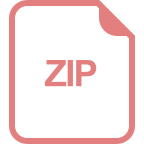
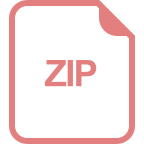
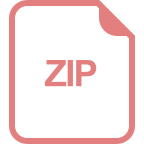
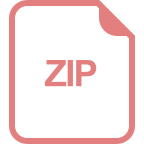
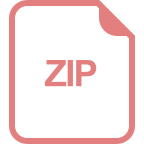
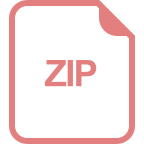
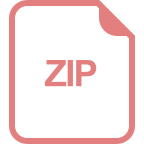
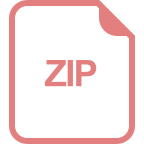
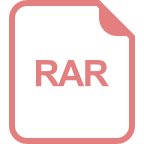
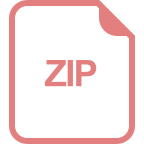
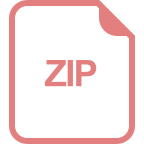
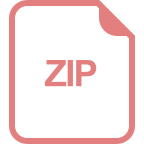
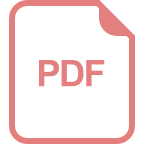
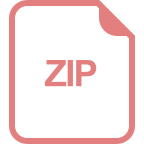
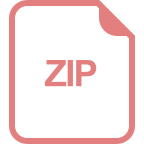
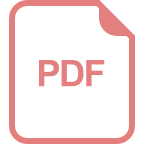
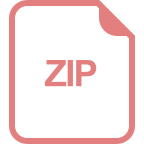
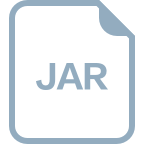
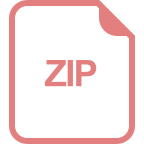
收起资源包目录

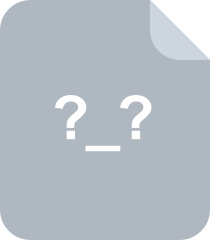
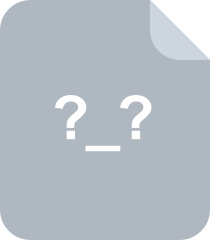
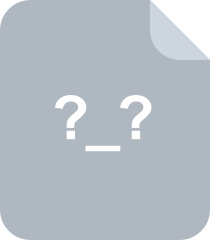
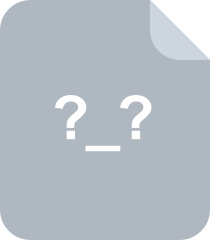
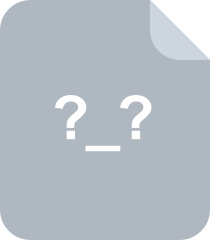
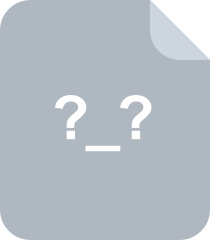
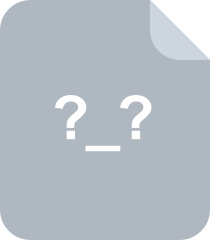
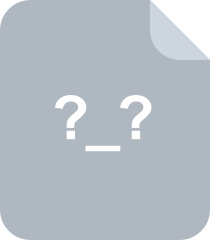
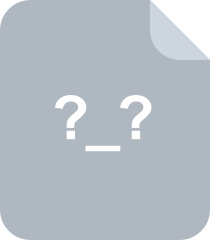
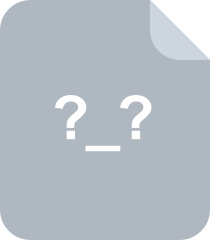
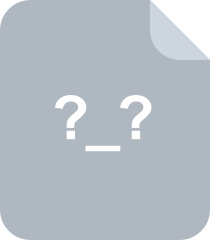
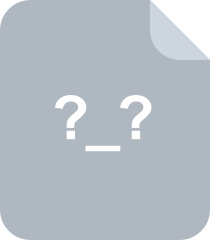
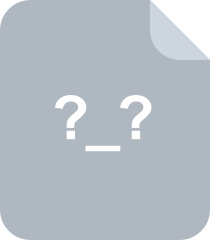
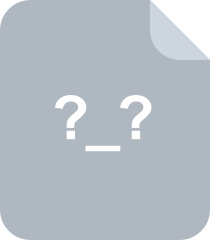
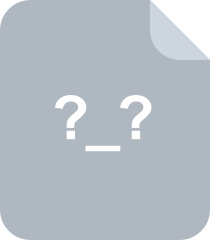
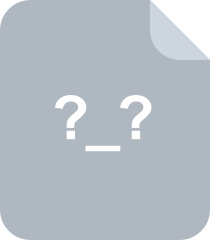
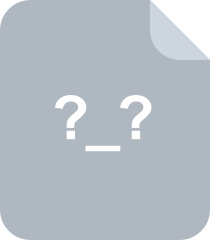
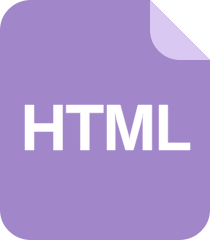
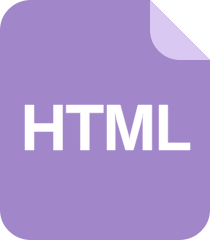
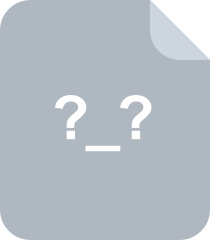
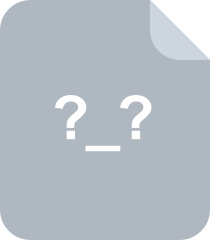
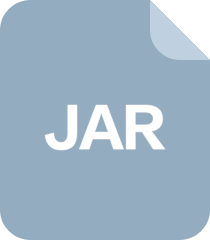
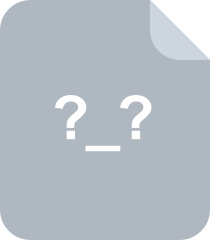
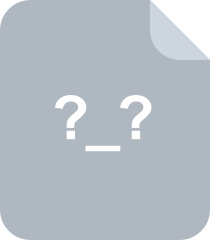
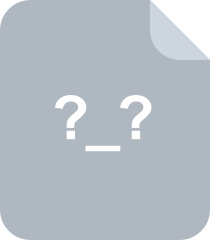
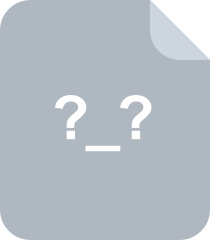
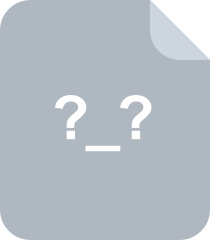
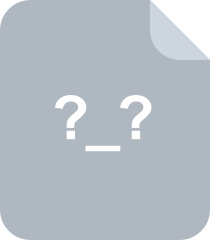
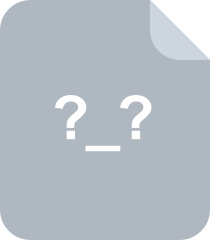
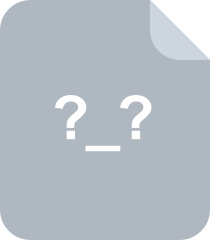
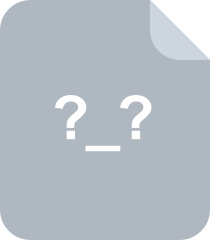
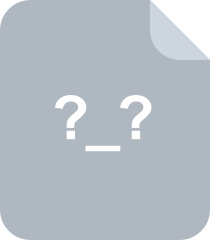
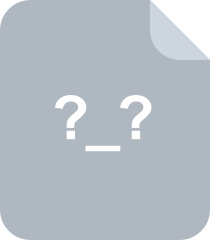
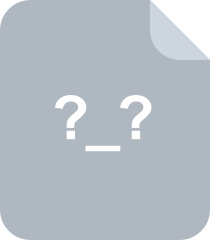
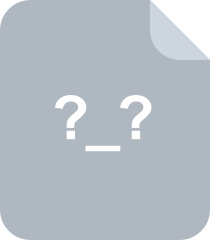
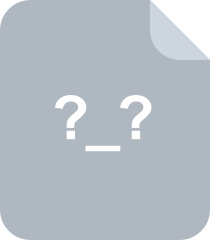
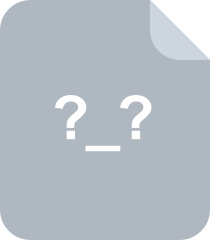
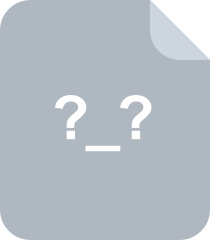
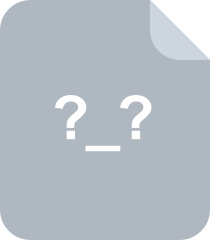
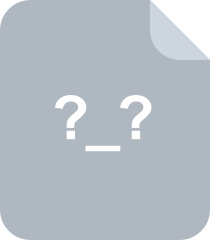
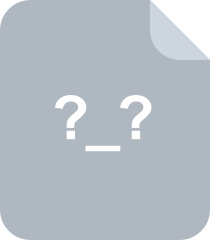
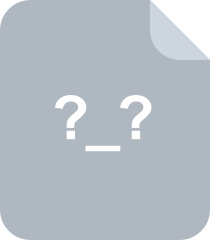
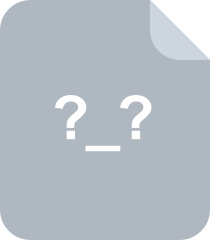
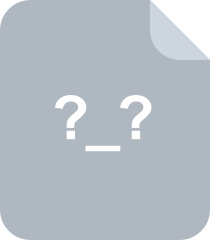
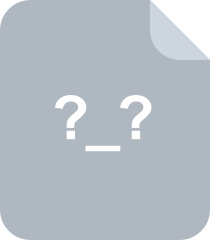
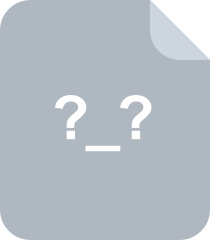
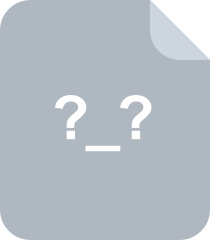
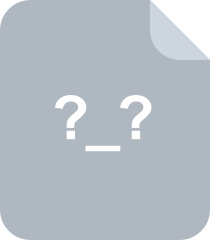
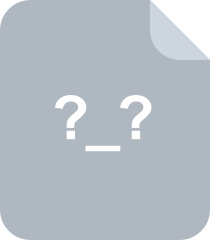
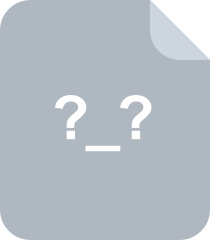
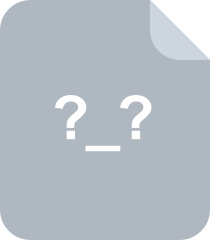
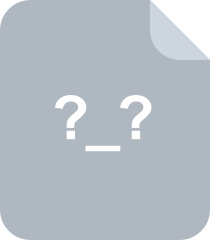
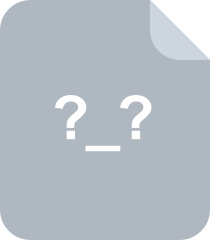
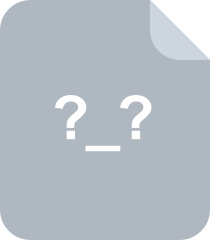
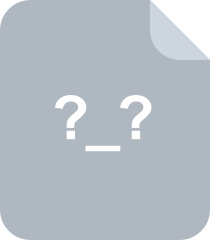
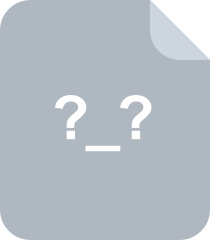
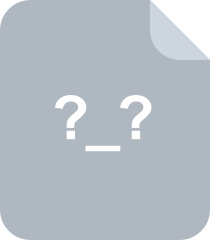
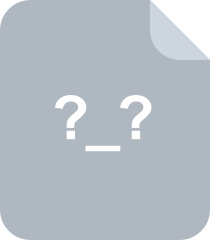
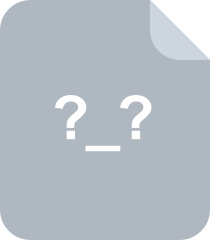
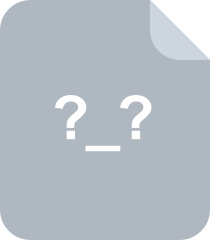
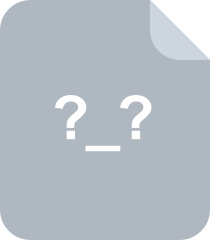
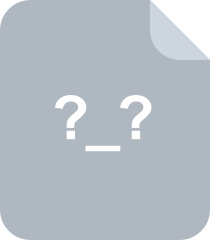
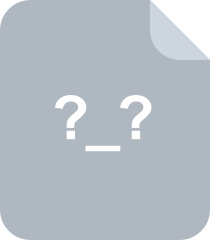
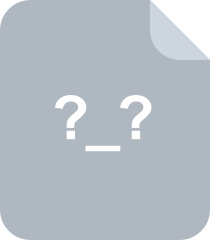
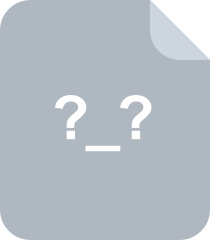
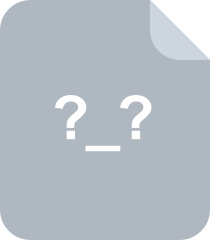
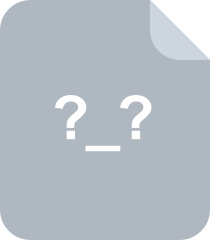
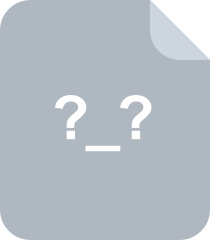
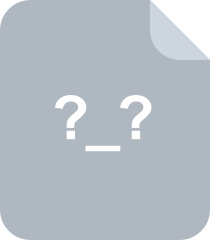
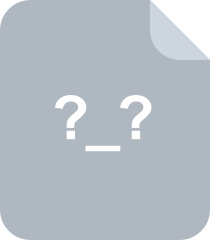
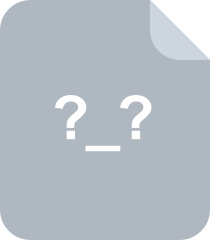
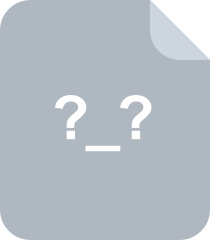
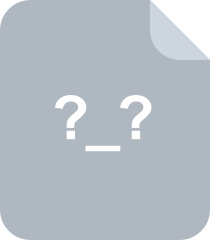
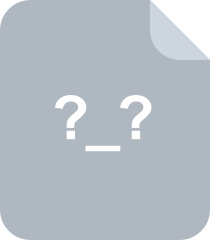
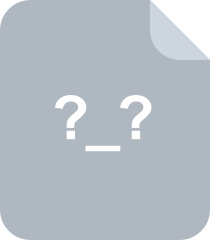
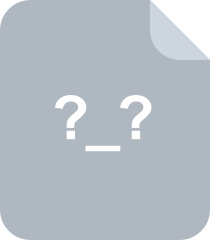
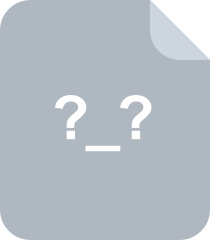
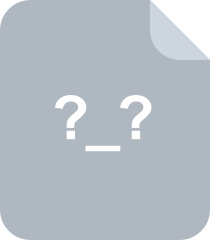
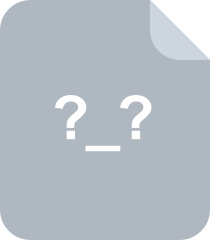
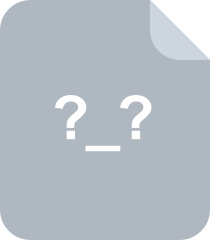
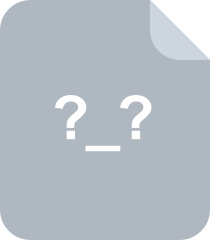
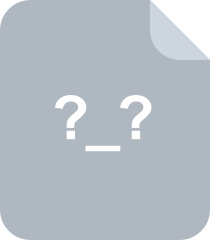
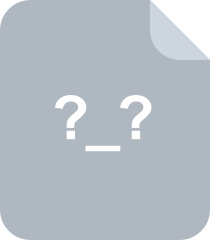
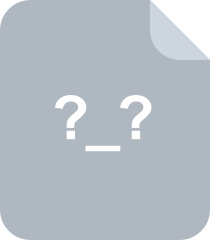
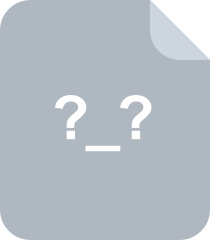
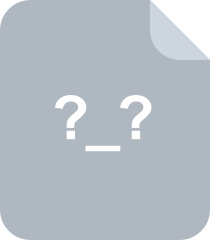
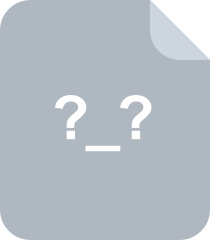
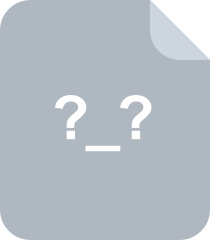
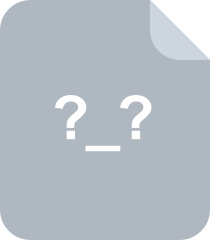
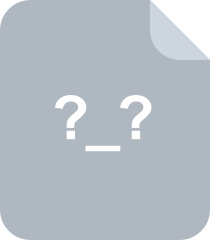
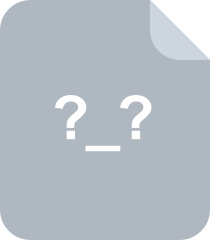
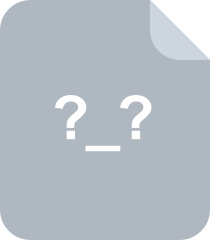
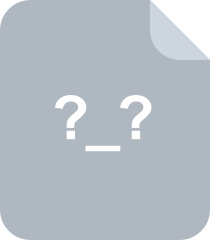
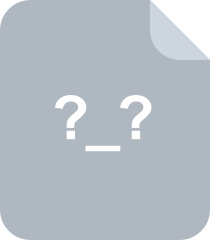
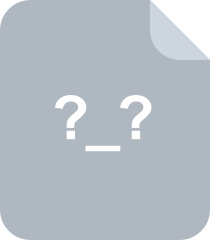
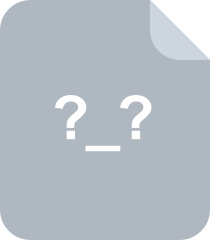
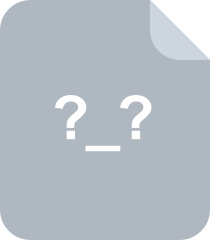
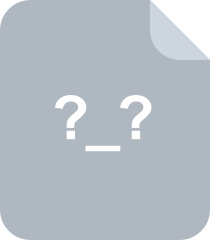
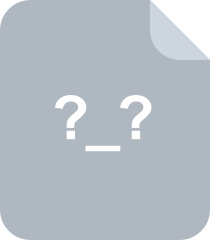
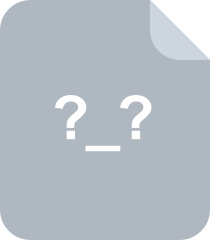
共 526 条
- 1
- 2
- 3
- 4
- 5
- 6
资源评论
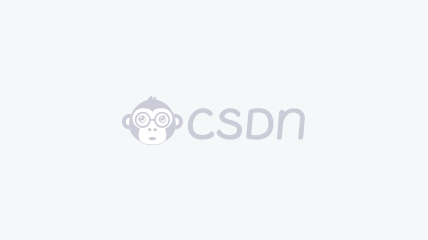

2013crazy
- 粉丝: 821
- 资源: 2236
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

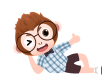
安全验证
文档复制为VIP权益,开通VIP直接复制
