Java8InAction
===============
This repository contains all the source code for the examples and quizzes in the book Java 8 in Action: Lambdas, Streams and functional-style programming.
You can purchase the book here: [http://manning.com/urma/](http://manning.com/urma/) or on Amazon
The source code for all examples can be found in the directory [src/main/java/lambdasinaction](https://github.com/java8/Java8InAction/tree/master/src/main/java/lambdasinaction)
* Chapter 1: Java 8: why should you care?
* Chapter 2: Passing code with behavior parameterization
* Chapter 3: Lambda expressions
* Chapter 4: Working with Streams
* Chapter 5: Processing data with streams
* Chapter 6: Collecting data with streams
* Chapter 7: Parallel data processing and performance
* Chapter 8: Refactoring, testing, debugging
* Chapter 9: Default methods
* Chapter 10: Using Optional as a better alternative to null
* Chapter 11: CompletableFuture: composable asynchronous programming
* Chapter 12: New Date and Time API
* Chapter 13: Thinking functionally
* Chapter 14: Functional programming techniques
* Chapter 15: Blending OOP and FP: comparing Java 8 and Scala
* Chapter 16: Conclusions and "where next" for Java
* Appendix A: Miscellaneous language updates
* Appendix B: Miscellaneous library updates
* Appendix C: Performing multiple operations in parallel on a Stream
* Appendix D: Lambdas and JVM bytecode
We will update the repository as we update the book. Stay tuned!
### Make sure to have JDK8 installed
The latest binary can be found here: http://www.oracle.com/technetwork/java/javase/overview/java8-2100321.html
$ java -version
java version "1.8.0_05"
Java(TM) SE Runtime Environment (build 1.8.0_05-b13)
Java HotSpot(TM) 64-Bit Server VM (build 25.5-b02, mixed mode)
You can download a preview version here: https://jdk8.java.net/
### Compile/Run the examples
Using maven:
$ mvn compile
$ cd target/classes
$ java lambdasinaction/chap1/FilteringApples
Alternatively you can compile the files manually inside the directory src/main/java
You can also import the project in your favorite IDE:
* In IntelliJ use "File->Open" menu and navigate to the folder where the project resides
* In Eclipse use "File->Import->Existing Maven Projects" (also modify "Reduntant super interfaces" to report as Warnings instead of Errors
* In Netbeans use "File->Open Project" menu
没有合适的资源?快使用搜索试试~ 我知道了~
java8实战的源代码
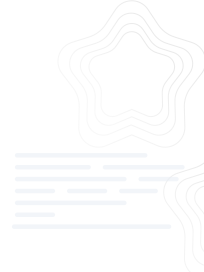
共131个文件
java:100个
sample:11个
head:4个

需积分: 16 41 下载量 48 浏览量
2018-11-18
13:05:07
上传
评论
收藏 206KB RAR 举报
温馨提示
简单地说,Java 8中的新增功能是自Java 1.0发布18年以来,Java发生的最大变化。没有去掉 任何东西,因此你现有的Java代码都能工作,但新功能提供了强大的新语汇和新设计模式,能帮 助你编写更清楚、更简洁的代码。就像遇到所有新功能时那样,你一开始可能会想:“为什么又 要去改我的语言呢?”但稍加练习之后,你就会发觉自己只用预期的一半时间,就用新功能写了更短、更清晰的代码,这时你会意识到自己永远无法返回到“旧Java”了。
资源推荐
资源详情
资源评论
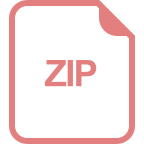
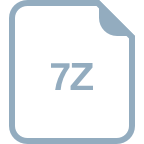
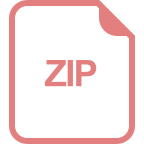
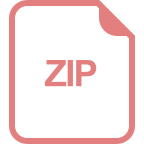
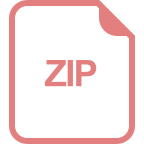
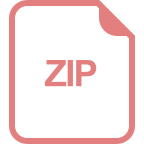
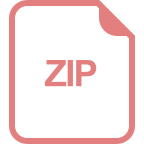
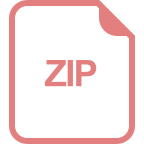
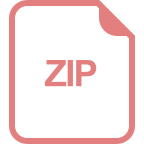
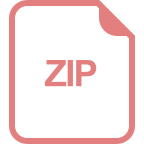
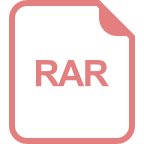
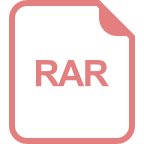
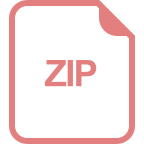
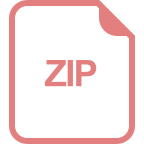
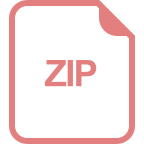
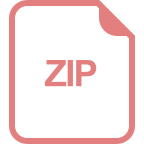
收起资源包目录

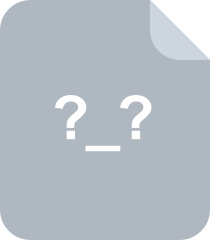
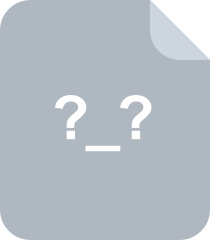
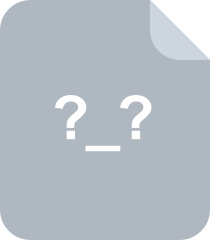
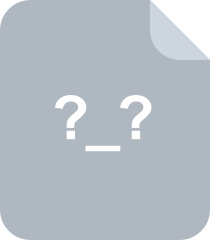
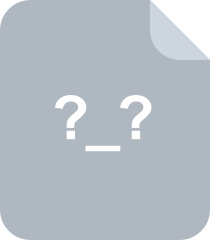
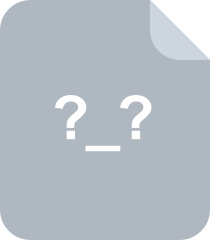
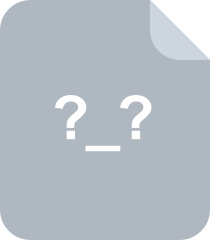
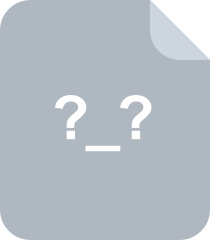
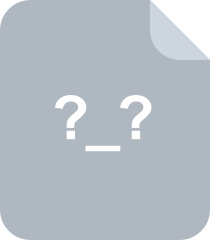
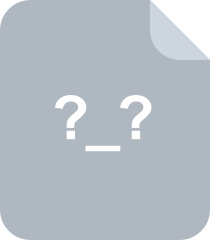
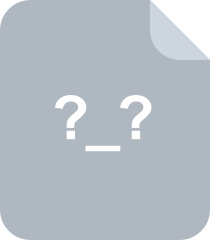
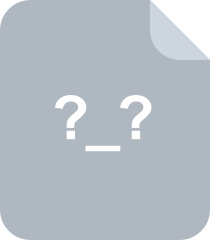
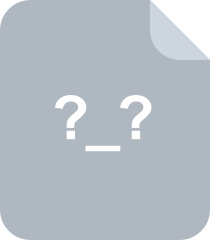
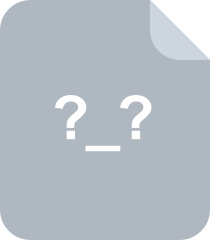
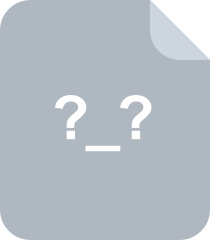
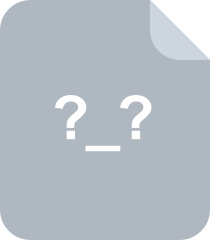
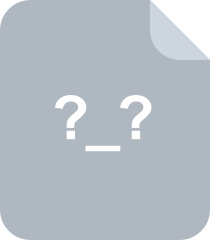
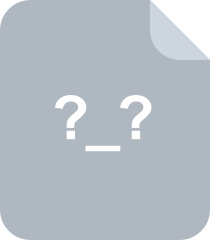
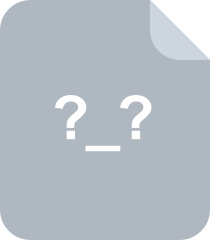
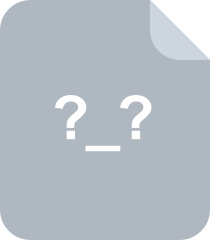
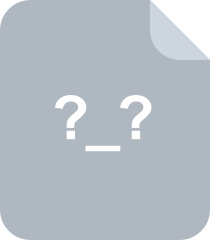
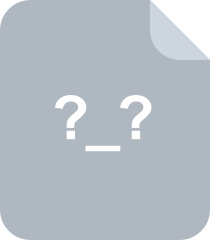
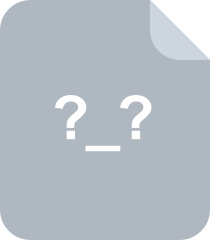
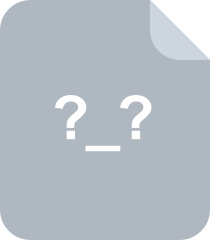
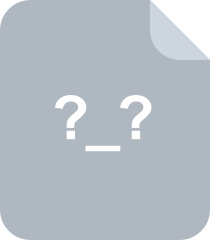
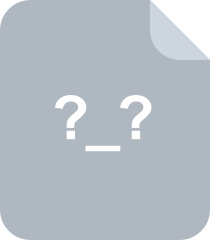
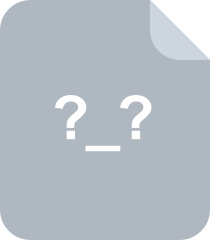
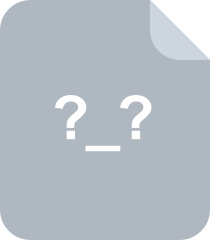
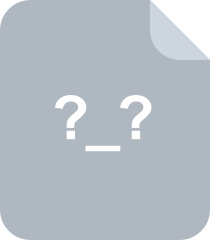
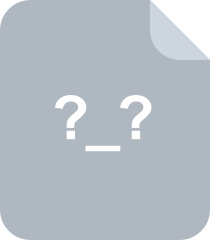
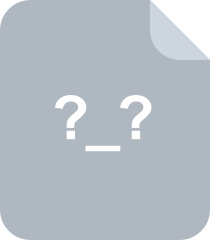
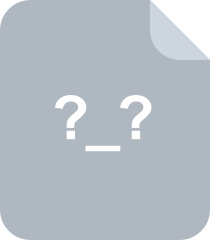
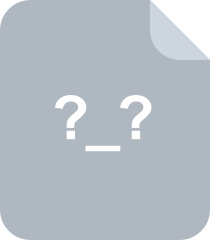
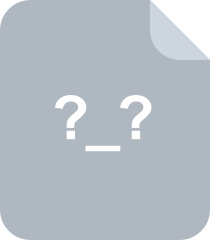
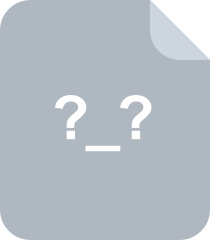
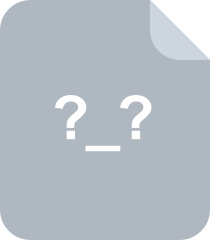
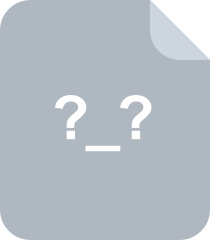
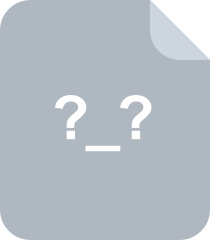
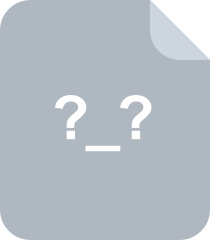
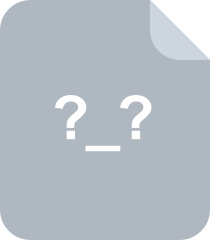
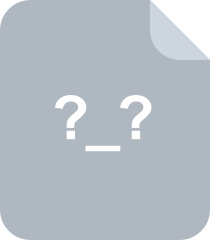
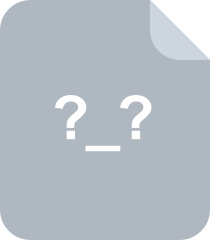
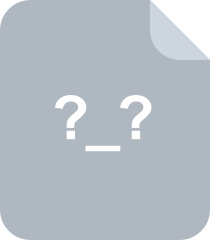
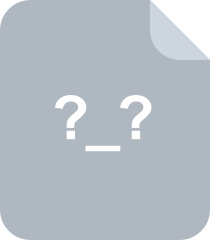
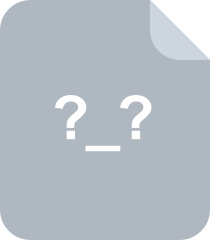
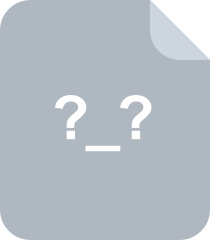
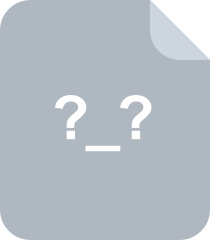
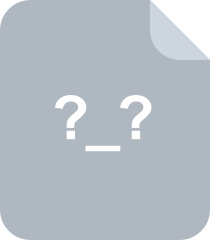
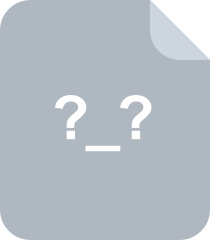
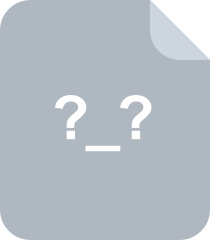
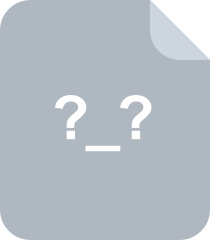
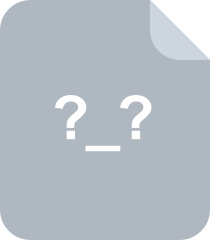
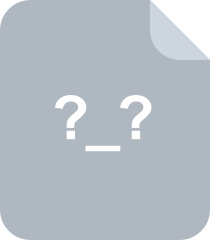
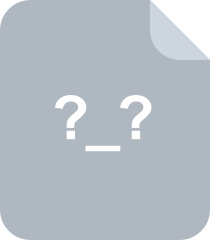
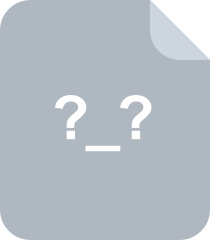
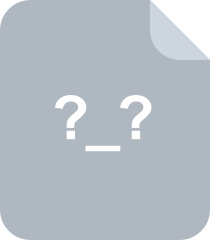
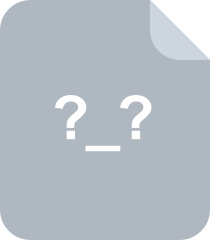
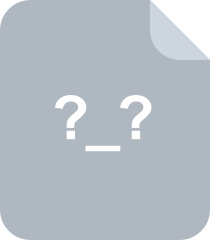
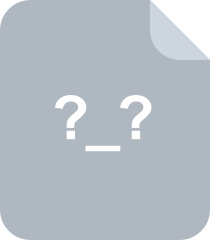
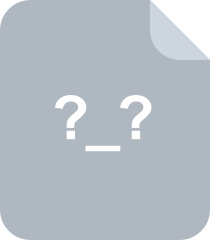
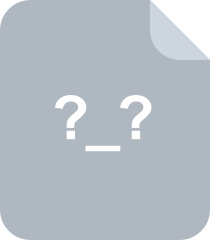
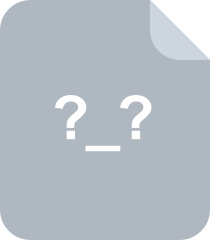
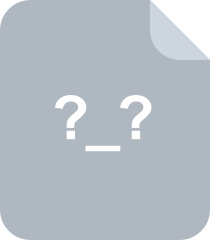
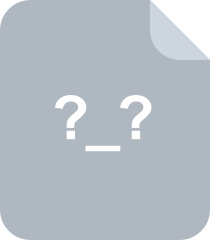
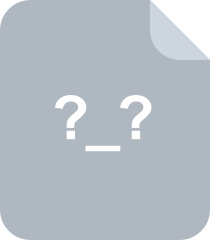
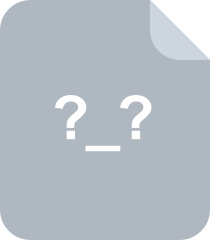
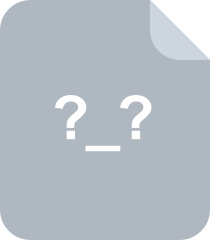
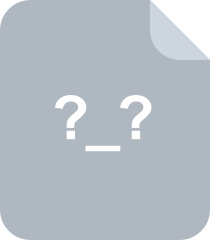
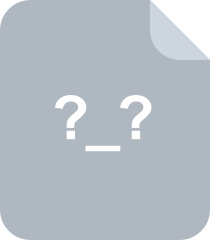
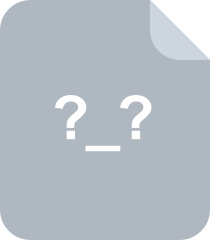
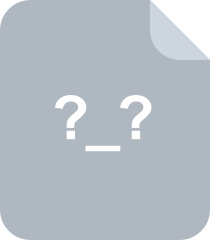
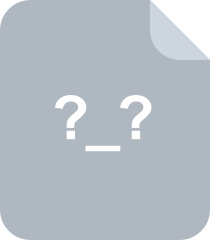
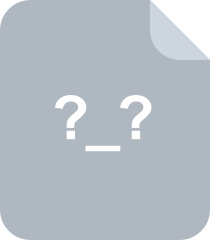
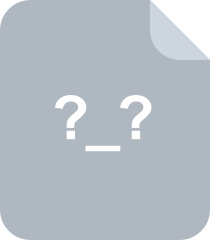
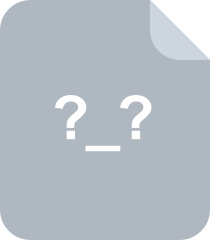
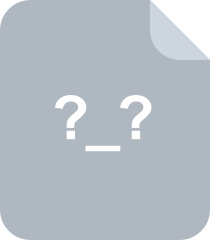
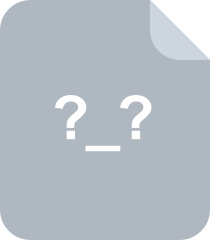
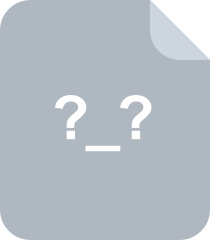
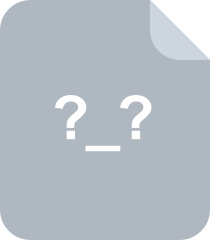
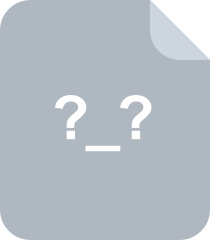
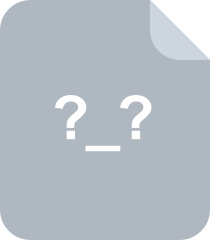
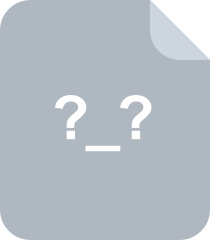
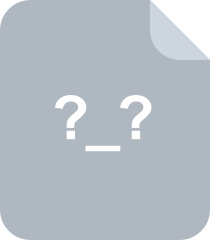
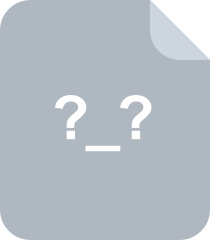
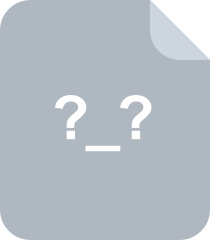
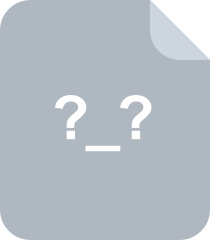
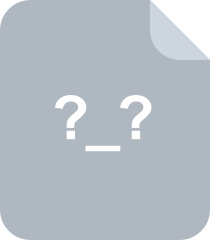
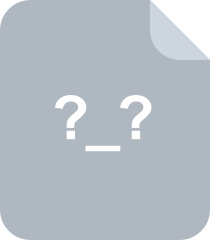
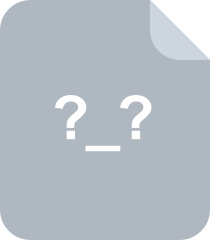
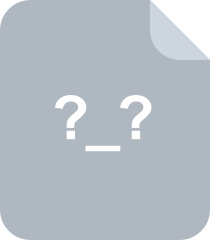
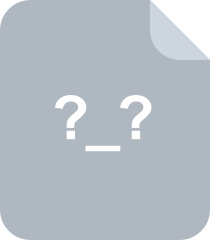
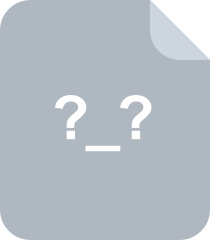
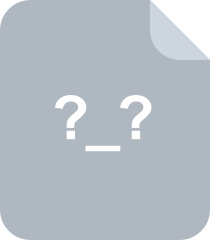
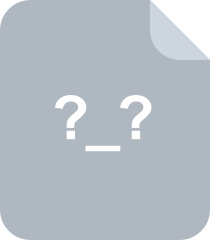
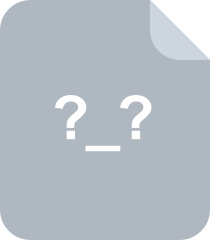
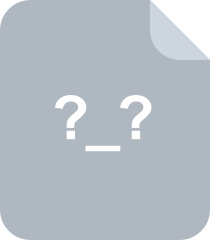
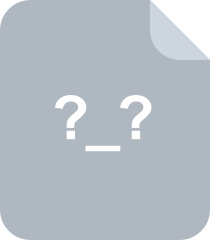
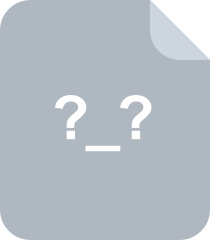
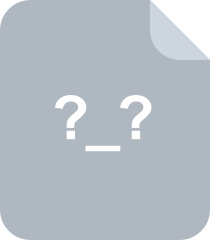
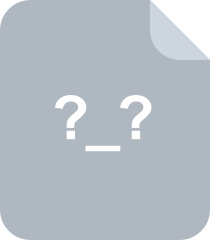
共 131 条
- 1
- 2
资源评论
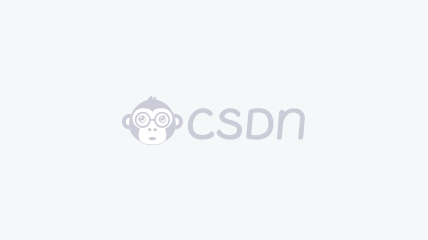

mkhsj
- 粉丝: 1
- 资源: 18
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

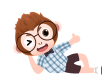
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


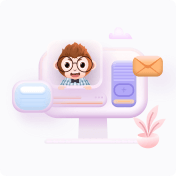
安全验证
文档复制为VIP权益,开通VIP直接复制
