import java.util.ArrayList;
public class InitPieceLocation implements java.io.Serializable{
Point [][] playPoint;
ChessPiece [] redPiece,blackPiece;
ChessBox redChessBox,blackChessBox;
public void setPoint(Point [][] p){
playPoint=p;
}
public void setRedChessPiece(ChessPiece [] redPiece){
this.redPiece=redPiece;
}
public void setBlackChessPiece(ChessPiece [] blackPiece){
this.blackPiece=blackPiece;
}
public void setRedChessBox(ChessBox redChessBox){
this.redChessBox=redChessBox;
}
public void setBlackChessBox(ChessBox blackChessBox){
this.blackChessBox=blackChessBox;
}
public void putAllPieceToPlayChessArea(){
for(int i=0;i<playPoint.length;i++){
for(int j=0;j<playPoint[i].length;j++){
playPoint[i][j].setHaveChessPiece(false);
}
}
ArrayList<ChessPiece> pieceList=new ArrayList<ChessPiece>();
for(int i=0;i<redPiece.length;i++){
pieceList.add(redPiece[i]);pieceList.add(blackPiece[i]);
}
for(int i=0;i<blackPiece.length;i++){
pieceList.add(blackPiece[i]);
}
for(int k=0;k<pieceList.size();k++){
ChessPiece piece=pieceList.get(k);
int w=piece.getBounds().width;
int h=piece.getBounds().height;
Point p=null;
p=piece.getAtPoint();
if(p!=null)
p.setHaveChessPiece(false);
String name=piece.getName();
if(name.equals("帅")&&piece.getIsRed()){
piece.setLocation(playPoint[9][4].getX()-w/2,playPoint[9][4].getY()-h/2);
piece.setAtPoint(playPoint[9][4]);
playPoint[9][4].setHaveChessPiece(true);
playPoint[9][4].setChessPiece(piece);
}
if((name.equals("士")||name.equals("仕"))&&piece.getIsRed()){
if(playPoint[9][3].isHaveChessPiece()==false){
piece.setLocation(playPoint[9][3].getX()-w/2,playPoint[9][3].getY()-h/2);
piece.setAtPoint(playPoint[9][3]);
playPoint[9][3].setHaveChessPiece(true);
playPoint[9][3].setChessPiece(piece);
}
else{
piece.setLocation(playPoint[9][5].getX()-w/2,playPoint[9][5].getY()-h/2);
piece.setAtPoint(playPoint[9][5]);
playPoint[9][5].setHaveChessPiece(true);
playPoint[9][5].setChessPiece(piece);
}
}
if(name.equals("相")&&piece.getIsRed()){
if(playPoint[9][2].isHaveChessPiece()==false){
piece.setLocation(playPoint[9][2].getX()-w/2,playPoint[9][2].getY()-h/2);
piece.setAtPoint(playPoint[9][2]);
playPoint[9][2].setHaveChessPiece(true);
playPoint[9][2].setChessPiece(piece);
}
else{
piece.setLocation(playPoint[9][6].getX()-w/2,playPoint[9][6].getY()-h/2);
piece.setAtPoint(playPoint[9][6]);
playPoint[9][6].setHaveChessPiece(true);
playPoint[9][6].setChessPiece(piece);
}
}
if((name.equals("马")||name.equals("馬"))&&piece.getIsRed()){
if(playPoint[9][1].isHaveChessPiece()==false){
piece.setLocation(playPoint[9][1].getX()-w/2,playPoint[9][1].getY()-h/2);
piece.setAtPoint(playPoint[9][1]);
playPoint[9][1].setHaveChessPiece(true);
playPoint[9][1].setChessPiece(piece);
}
else{
piece.setLocation(playPoint[9][7].getX()-w/2,playPoint[9][7].getY()-h/2);
piece.setAtPoint(playPoint[9][7]);
playPoint[9][7].setHaveChessPiece(true);
playPoint[9][7].setChessPiece(piece);
}
}
if((name.equals("车")||name.equals("車"))&&piece.getIsRed()){
if(playPoint[9][0].isHaveChessPiece()==false){
piece.setLocation(playPoint[9][0].getX()-w/2,playPoint[9][0].getY()-h/2);
piece.setAtPoint(playPoint[9][0]);
playPoint[9][0].setHaveChessPiece(true);
playPoint[9][0].setChessPiece(piece);
}
else{
piece.setLocation(playPoint[9][8].getX()-w/2,playPoint[9][7].getY()-h/2);
piece.setAtPoint(playPoint[9][8]);
playPoint[9][8].setHaveChessPiece(true);
playPoint[9][8].setChessPiece(piece);
}
}
if(name.equals("炮")&&piece.getIsRed()){
if(playPoint[7][1].isHaveChessPiece()==false){
piece.setLocation(playPoint[7][1].getX()-w/2,playPoint[7][1].getY()-h/2);
piece.setAtPoint(playPoint[7][1]);
playPoint[7][1].setHaveChessPiece(true);
playPoint[7][1].setChessPiece(piece);
}
else{
piece.setLocation(playPoint[7][7].getX()-w/2,playPoint[7][7].getY()-h/2);
piece.setAtPoint(playPoint[7][7]);
playPoint[7][7].setHaveChessPiece(true);
playPoint[7][7].setChessPiece(piece);
}
}
if(name.equals("兵")&&piece.getIsRed()){
if(playPoint[6][0].isHaveChessPiece()==false){
piece.setLocation(playPoint[6][0].getX()-w/2,playPoint[6][0].getY()-h/2);
piece.setAtPoint(playPoint[6][0]);
playPoint[6][0].setHaveChessPiece(true);
playPoint[6][0].setChessPiece(piece);
}
else if(playPoint[6][2].isHaveChessPiece()==false){
piece.setLocation(playPoint[6][2].getX()-w/2,playPoint[6][2].getY()-h/2);
piece.setAtPoint(playPoint[6][2]);
playPoint[6][2].setHaveChessPiece(true);
playPoint[6][2].setChessPiece(piece);
}
else if(playPoint[6][4].isHaveChessPiece()==false){
piece.setLocation(playPoint[6][4].getX()-w/2,playPoint[6][4].getY()-h/2);
piece.setAtPoint(playPoint[6][4]);
playPoint[6][4].setHaveChessPiece(true);
playPoint[6][4].setChessPiece(piece);
}
else if(playPoint[6][6].isHaveChessPiece()==false){
piece.setLocation(playPoint[6][6].getX()-w/2,playPoint[6][6].getY()-h/2);
piece.setAtPoint(playPoint[6][6]);
playPoint[6][6].setHaveChessPiece(true);
playPoint[6][6].setChessPiece(piece);
}
else{
piece.setLocation(playPoint[6][8].getX()-w/2,playPoint[6][8].getY()-h/2);
piece.setAtPoint(playPoint[6][8]);
playPoint[6][8].setHaveChessPiece(true);
playPoint[6][8].setChessPiece(piece);
}
}
if(name.equals("将")&&piece.getIsBlack()){
piece.setLocation(playPoint[0][4].getX()-w/2,playPoint[0][4].getY()-h/2);
piece.setAtPoint(playPoint[0][4]);
playPoint[0][4].setHaveChessPiece(true);
playPoint[0][4].setChessPiece(piece);
}
if((name.equals("士")||name.equals("仕"))&&piece.getIsBlack()){
if(playPoint[0][3].isHaveChessPiece()==false){
piece.setLocation(playPoint[0][3].getX()-w/2,playPoint[0][3].getY()-h/2);
piece.setAtPoint(playPoint[0][3]);
playPoint[0][3].setHaveChessPiece(true);
playPoint[0][3].setChessPiece(piece);
}
else{
piece.setLocation(playPoint[0][5].getX()-w/2,playPoint[0][5].getY()-h/2);
piece.setAtPoint(playPoint[0][5]);
playPoint[0][5].setHaveChessPiece(true);

仰望IT星空
- 粉丝: 0
- 资源: 1
最新资源
- stylus-chrome-mv2-2.0.8-id.zip
- maven地配置文件,可以找文章自己配置
- 123sdqwdqdwdqdqd
- 游戏人物检测32-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- 数控稳压直流电源课程设计报告
- python爬虫中国日报爬虫,按关键词爬取中国日报新闻
- 正则表达式的基本语法及其在文本处理中的应用
- order_system.c
- oracle sqlplus arm版本和linux版本
- MATLAB代码:基于主从博弈的电热综合能源系统动态定价与能量管理 关键词:主从博弈 电热综合能源 动态定价 能量管理 仿真平台:MATLAB 平台 优势:代码具有一定的深度和创新性,注释清晰
- SQL Server Native Client 10 64位驱动
- infobright-4.0.7-0-x86-64-ice.rpm
- 棉花病害图像分类数据集8类别:健康棉花叶、蚜虫、粘虫、白粉病、斑点病、细菌性叶枯病、健康棉花球、棉花球红腐病(6000多张图片).rar
- 永磁同步电机pmsm无感foc驱动代码,启动为高频注入,平滑切入观测器高速控制,代码全部手写开源,可以移植到各类mcu上 附赠高频注入仿真模型
- 游戏人物检测33-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- relation-GDP-life - GDP与幸福感指数关系 数据分析项目
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


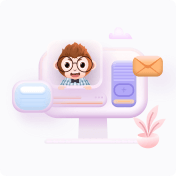