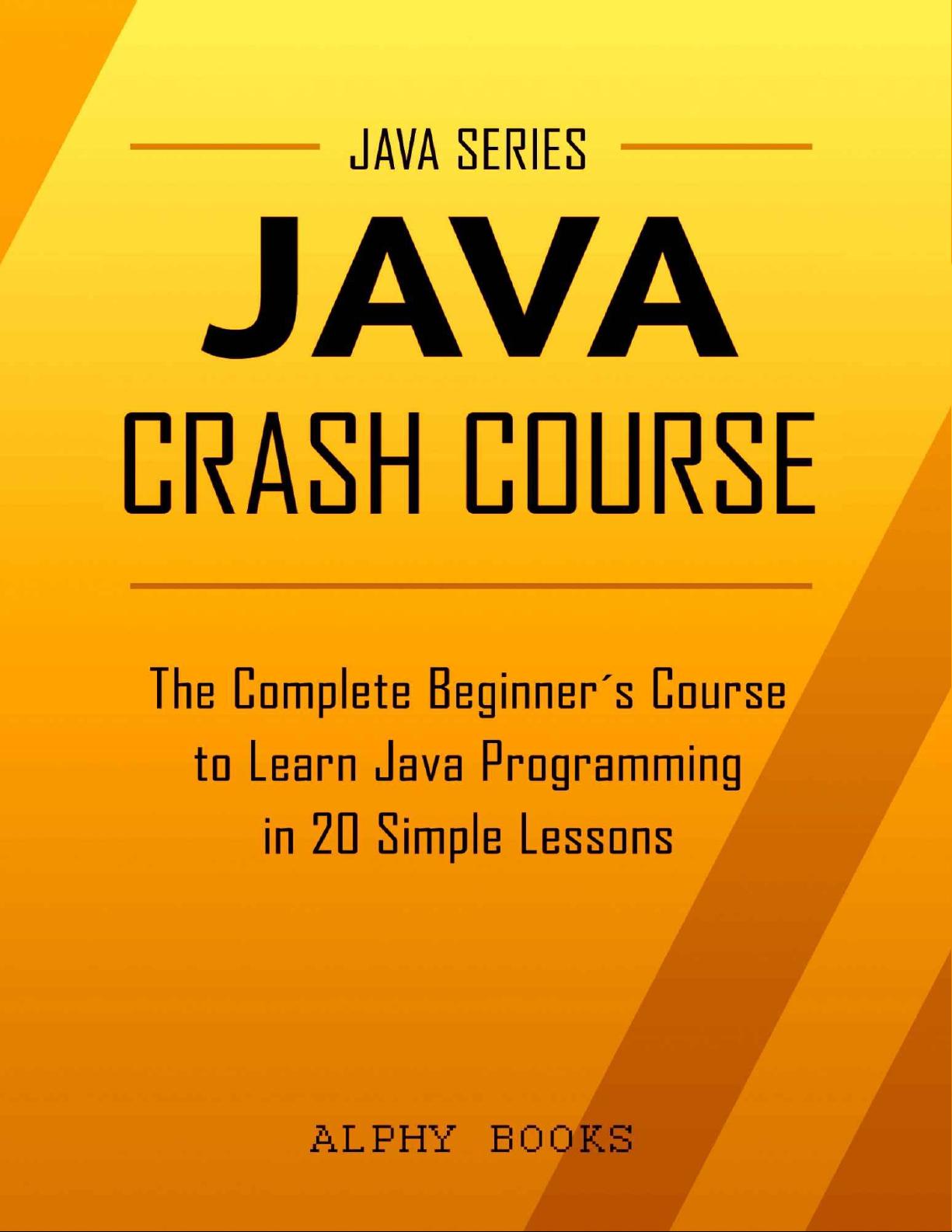
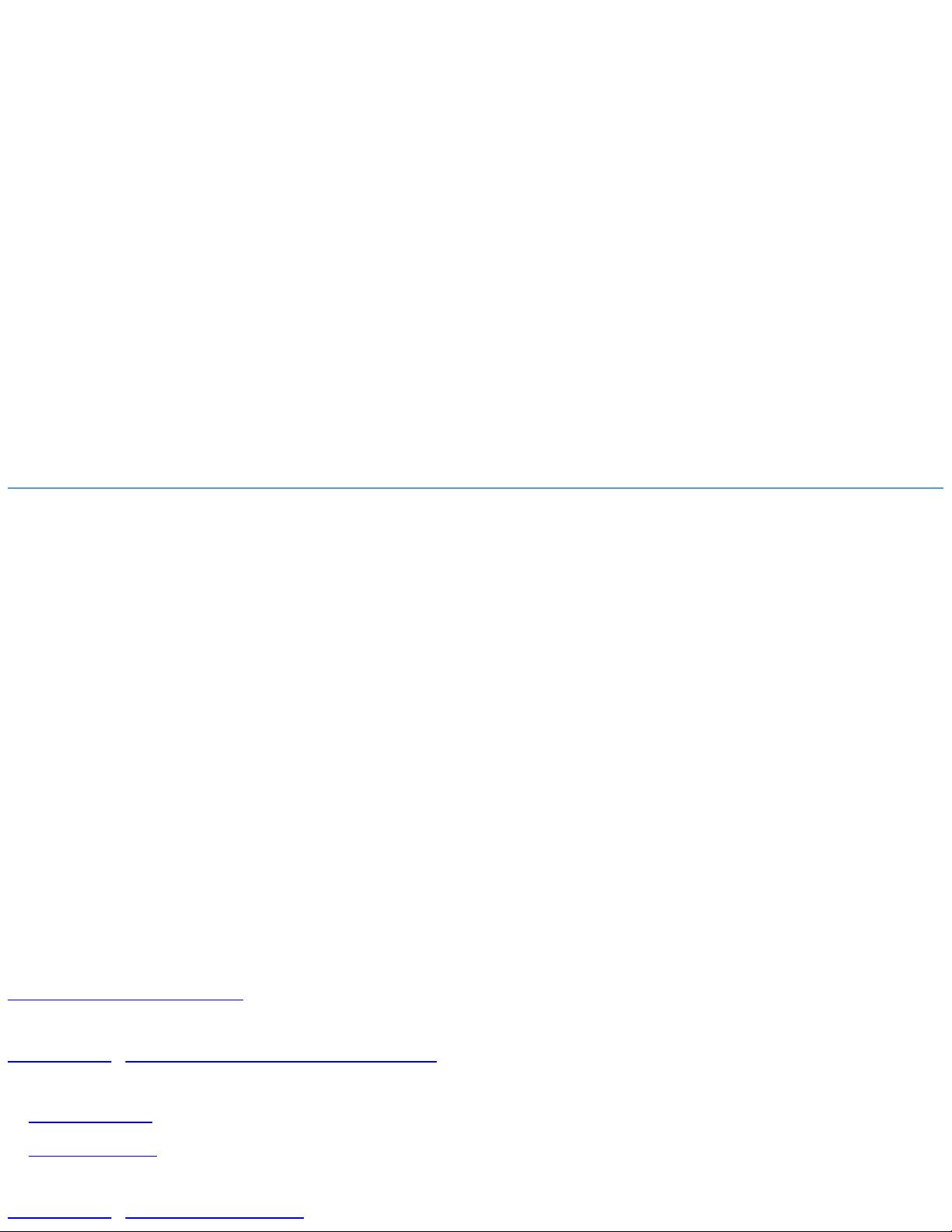
JAVA
CRASH COURSE
The Complete Beginner's Course to Learn Java
Programming in 21 Clear-Cut Lessons - Including
Dozens of Practical Examples & Exercises
By Alphy Books
Copyright © 2016
All rights reserved. No part of this publication may be reproduced, distributed or transmitted in any
form or by any means, including photocopying, recording, or other electronic or mechanical methods,
without the prior written permission of the publisher, except in the case of brief quotations embodied
in reviews and certain non-commercial uses permitted by copyright law.
Trademarked names appear throughout this book. Rather than use a trademark symbol with every
occurrence of a trademark name, names are used in an editorial fashion, with no intention of
infringement of the respective owner's trademark.
The information in this book is distributed on an "as is" basis, exclusively for educational purposes,
without warranty. Neither the author nor the publisher shall have any liability to any person or entity
with respect to any loss or damage caused or alleged to be caused directly or indirectly by the
information contained in this book.
Table of Contents
Greetings and Welcome
Chapter 1 : Let's Start From The Beginning
Short History
What is Java?
Chapter 2 : Java Environment
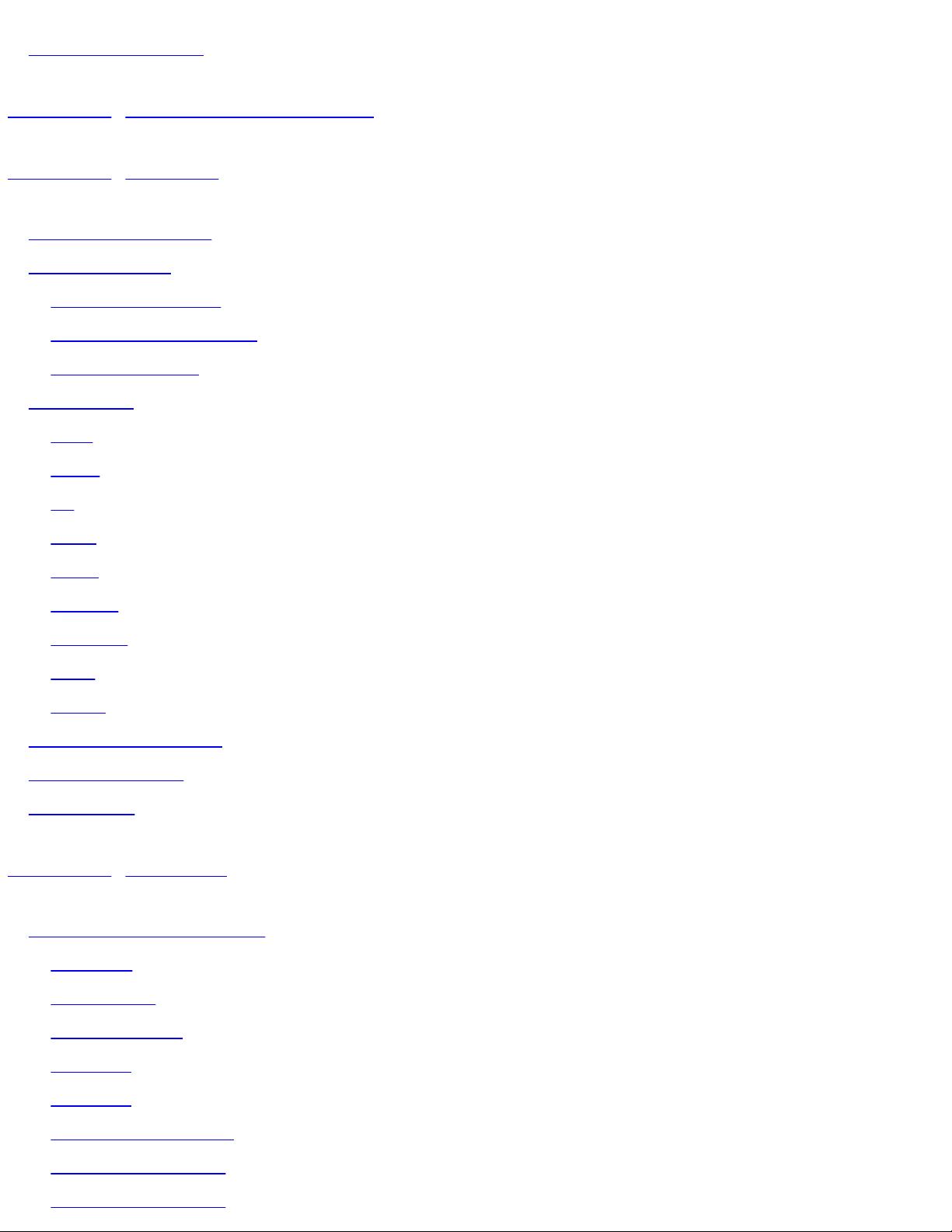
Installation of Java
Chapter 3 : Java Language Structure
Chapter 4 : Variables
What is a Variable?
Variable Types
Instance Variables
Class/Static Variables
Local Variables
Data Types
Byte
Short
Int
Long
Float
Double
Boolean
Char
String
Declaring a Variable
Using a Variable
Assignment
Chapter 5 : Operators
The Arithmetic Operators
Addition
Subtraction
Multiplication
Division
Modules
Post-Incrementation
Pre-Incrementation
Post-Decrementing
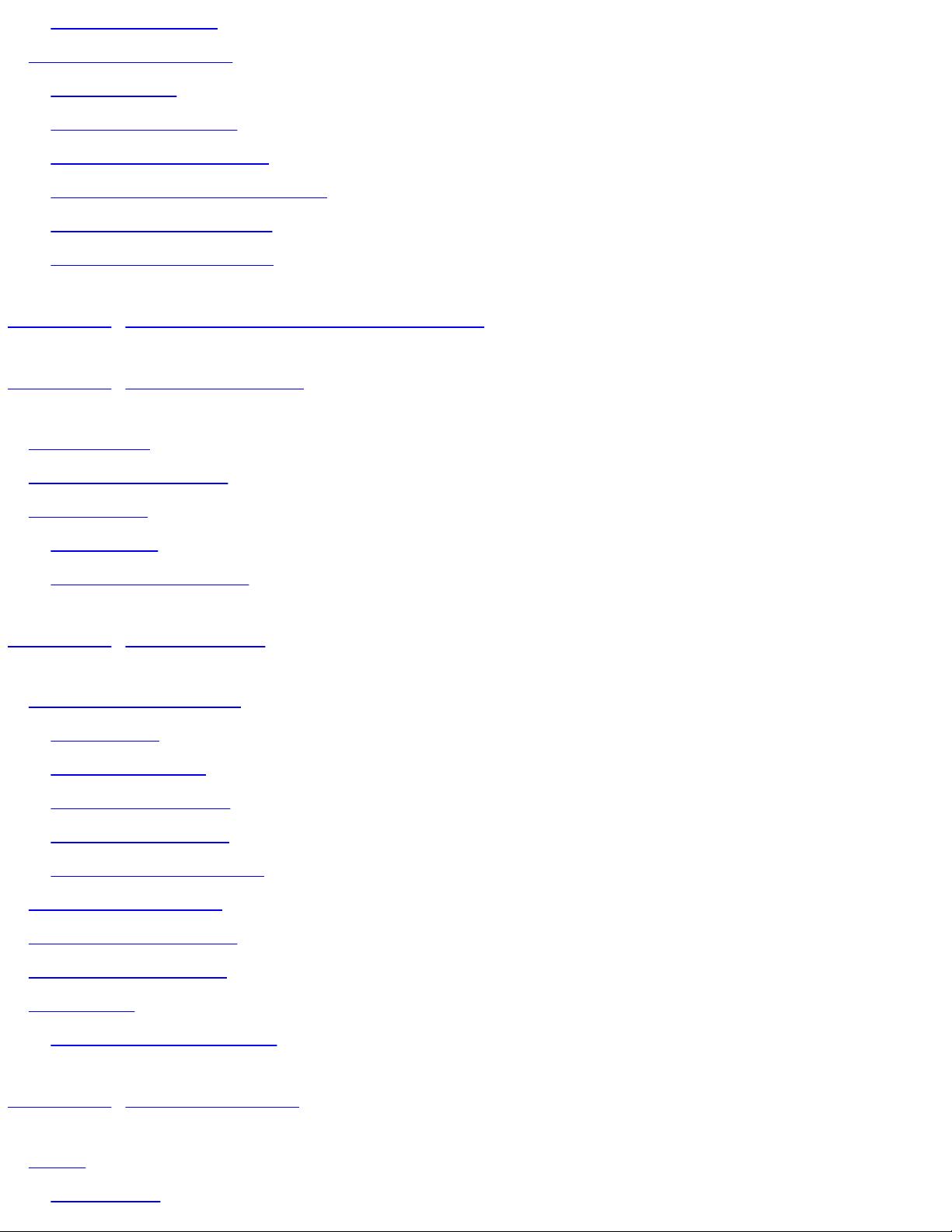
Pre-Decrementing
Assignment Operators
Equal Sign: =
Add-Equal Sign: +=
Subtract-Equal Sign: -=
Multiplication-Equal Sign: *=
Division-Equal Sign: /=
Modules-Equal Sign: -=
Chapter 6 : User Input: Getting Data in Runtime
Chapter 7 : The String Object
String Length
Concatenating Strings
String Buffer
Constructor
StringBuffer Methods
Chapter 8 : Boolean Logic
Conditional Statements
If Statement
If Else Statement
Ladder If Statement
Nested If Statement
If Statements Examples
Relational Operators
The Logical Operators
Combining Operators
Assignment
Answer and Explanation
Chapter 9 : Loops and Arrays
Loops
While Loop
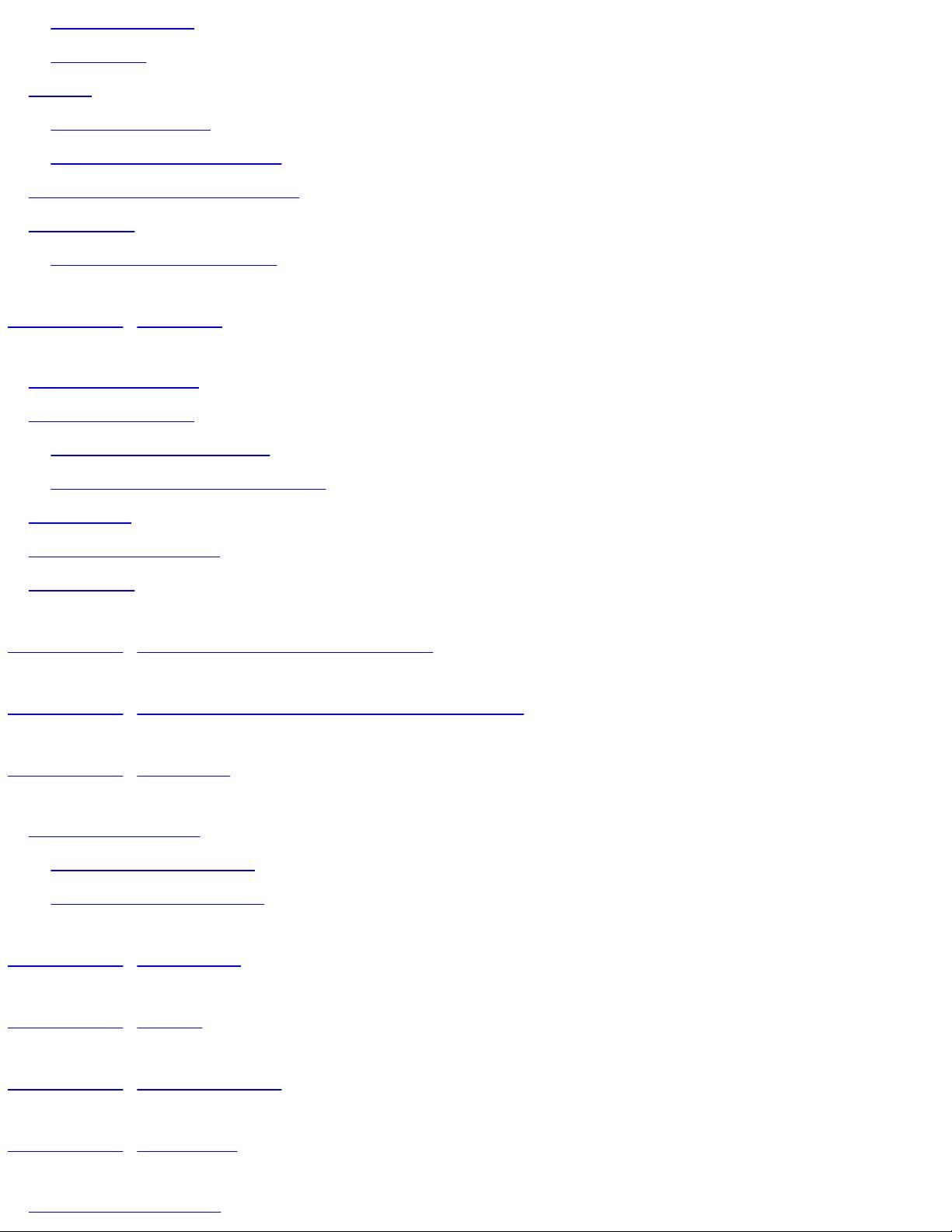
Do While Loop
For Loops
Arrays
Declaring Arrays
Multidimensional Arrays
Combining Loops and Arrays
Assignment
Answer and Explanation
Chapter 10 : Methods
Creating a Method
Types of Methods
Syntax of Static Method
Syntax of Non-Static Methods
Parameters
Method Overloading
Assignment
Chapter 11 : Inheritance and Polymorphism
Chapter 12 : Interfaces and Abstract Classes in Java
Chapter 13 : Packages
Types of Packages
Pre-Defined Packages
User Defined Packages
Chapter 14 : Debugging
Chapter 15 : Enums
Chapter 16 : Generic Types
Chapter 17 : Threading
Life Cycle of Thread