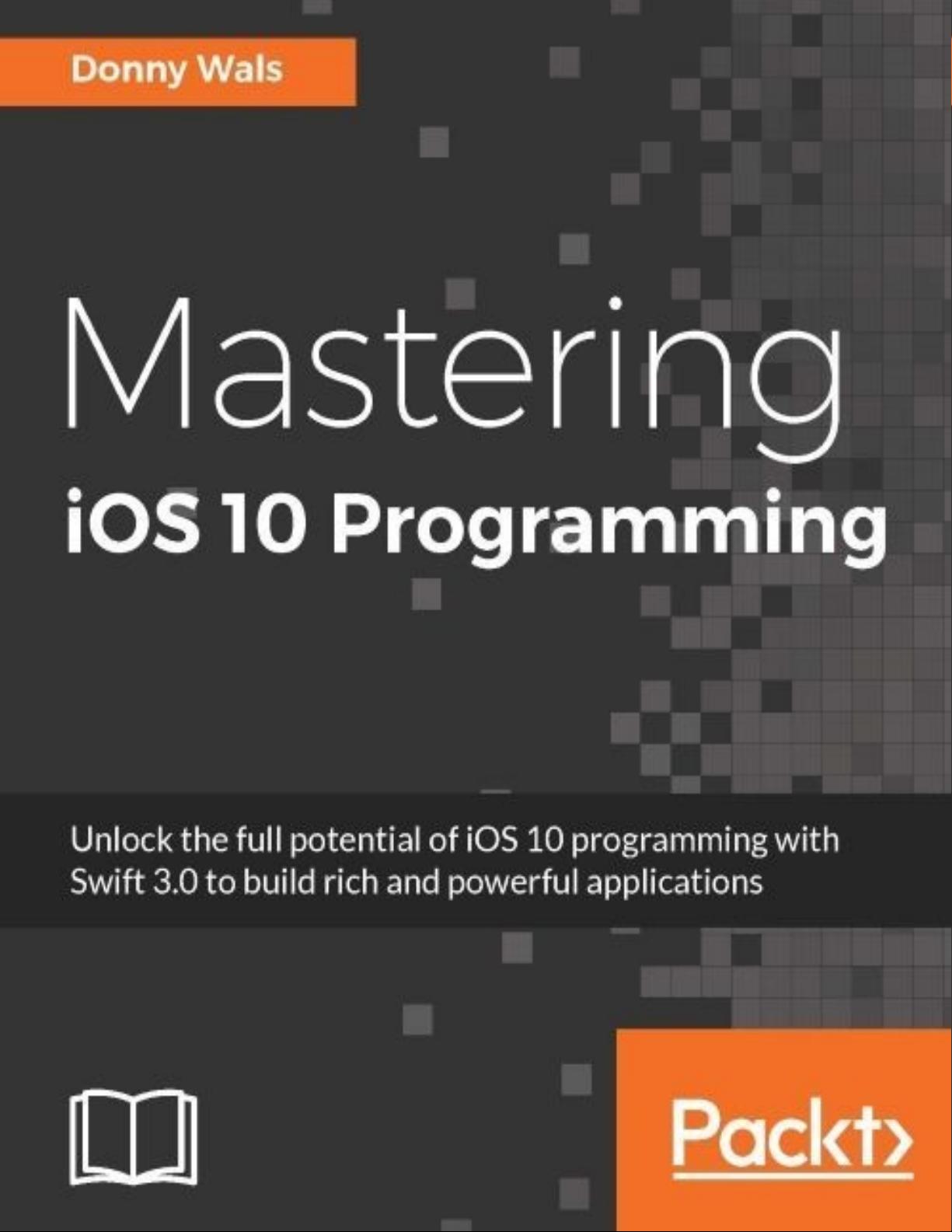
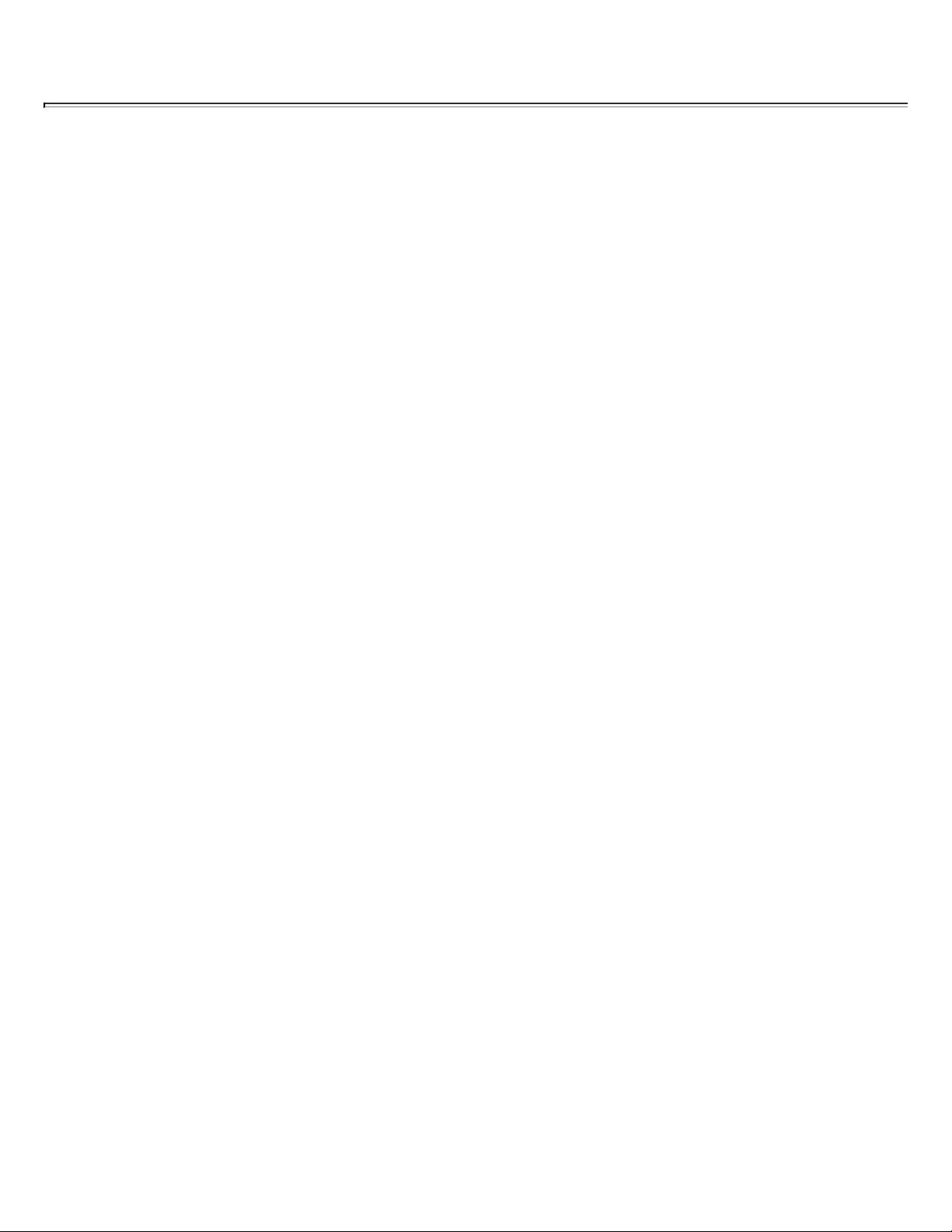
MasteringiOS10Programming
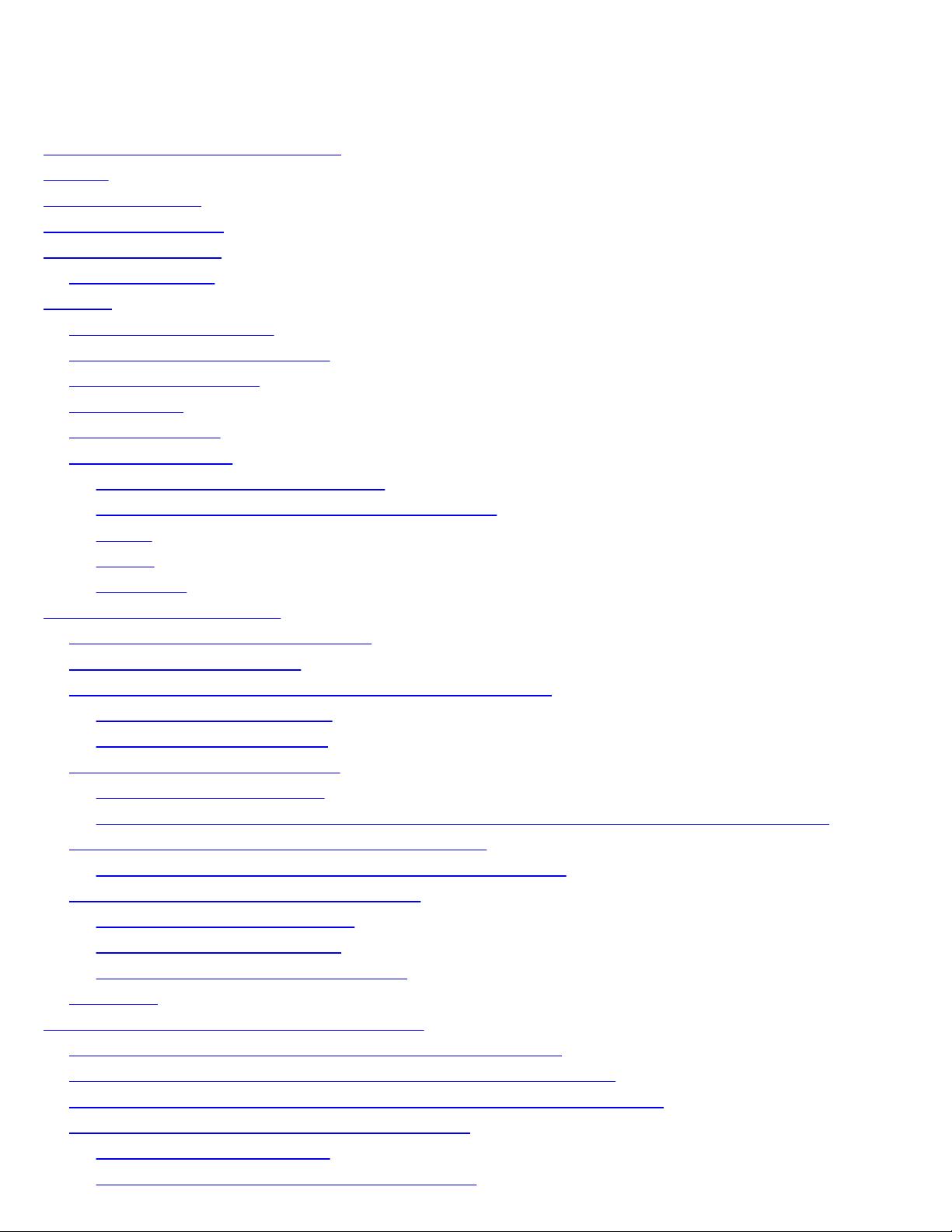
TableofContents
MasteringiOS10Programming
Credits
AbouttheAuthor
AbouttheReviewer
www.PacktPub.com
Whysubscribe?
Preface
Whatthisbookcovers
Whatyouneedforthisbook
Whothisbookisfor
Conventions
Readerfeedback
Customersupport
Downloadingtheexamplecode
Downloadingthecolorimagesofthisbook
Errata
Piracy
Questions
1.UITableViewTouchUp
SettinguptheUserInterface(UI)
Fetchingauser'scontacts
CreatingacustomUITableViewCellforourcontacts
Designingthecontactcell
Creatingthecellsubclass
Displayingthelistofcontacts
Protocolsanddelegation
ConformingtotheUITableViewDataSourceandUITableViewDelegateprotocol
UnderthehoodofUITableViewperformance
ImprovingperformancewithprefetchinginiOS10
UITableViewDelegateandinteractions
Respondingtocellselection
Implementingcelldeletion
Allowingtheusertoreordercells
Summary
2.ABetterLayoutwithUICollectionView
ConvertingfromaUITableViewtoUICollectionView
CreatingandimplementingacustomUICollectionViewCell
UnderstandingtheUICollectionViewFlowLayoutanditsdelegate
CreatingacustomUICollectionViewLayout
Pre-calculatingthelayout
ImplementingcollectionViewContentSize
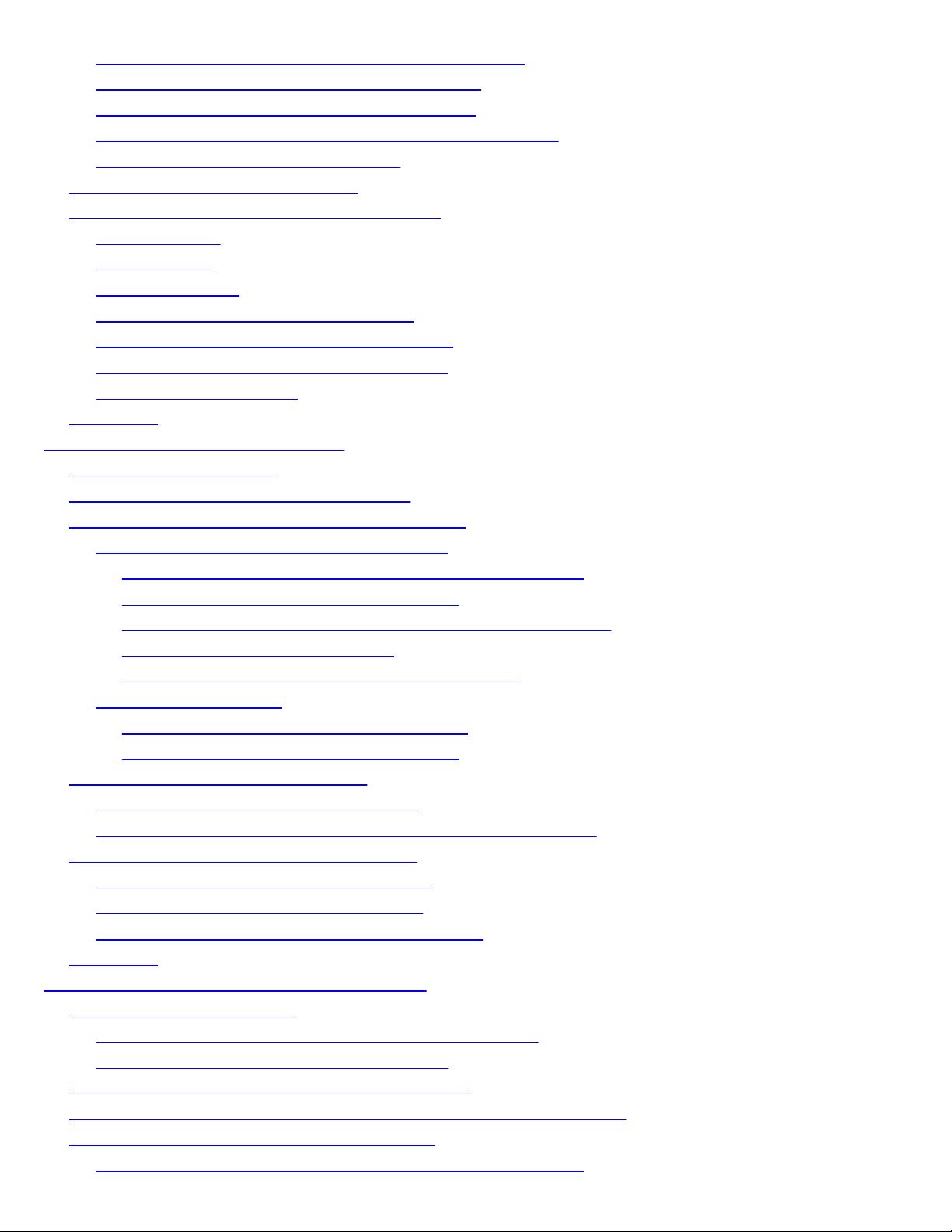
ImplementinglayoutAttributesForElements(_:)
ImplementinglayoutAttributesForItem(_:)
ImplementingshouldInvalidateLayout(_:)
Assigningthecustomlayouttothecollectionview
Finalwordsonthecustomlayout
UICollectionViewperformance
UserinteractionswithUICollectionView
Cellselection
Celldeletion
Cellreordering
Refactoringthelongpresshandler
Implementingthereordermethodcalls
Implementingthedatasourcemethods
Addingtheeditbutton
Summary
3.CreatingaContactDetailPage
Universalapplications
Implementingnavigationwithsegues
CreatingadaptivelayoutswithAutoLayout
AutoLayoutwiththeInterfaceBuilder
Addingascrollviewtomakethelayoutscrollable
Layingouttheimageandnamelabel
Adjustingtheimageandnamelabelforlargescreens
Layingoutthebottomsection
Adjustthebottomsectionforsmallscreens
AutoLayoutincode
Implementingthecompactsizelayout
Implementingtheregularsizelayout
EasierlayoutswithUIStackView
ContaininglabelsinaUIStackView
VaryingcolumnsandlayoutsfortraitsinUIStackView
Passingdatabetweenviewcontrollers
Updatingthedataloadingandmodel
Passingthemodeltothedetailpage
Implementingnewoutletsanddisplaydata
Summary
4.ImmersingYourUsersWithAnimation
UIViewanimationbasics
UsingtheUIView.animatemethodforanimation
ExploringUIView'sanimationoptions
Addingvibrancytoanimationswithsprings
TakingcontrolofanimationswithUIViewPropertyAnimator
Customizingviewcontrollertransitions
Implementingacustommodalpresentationtransition
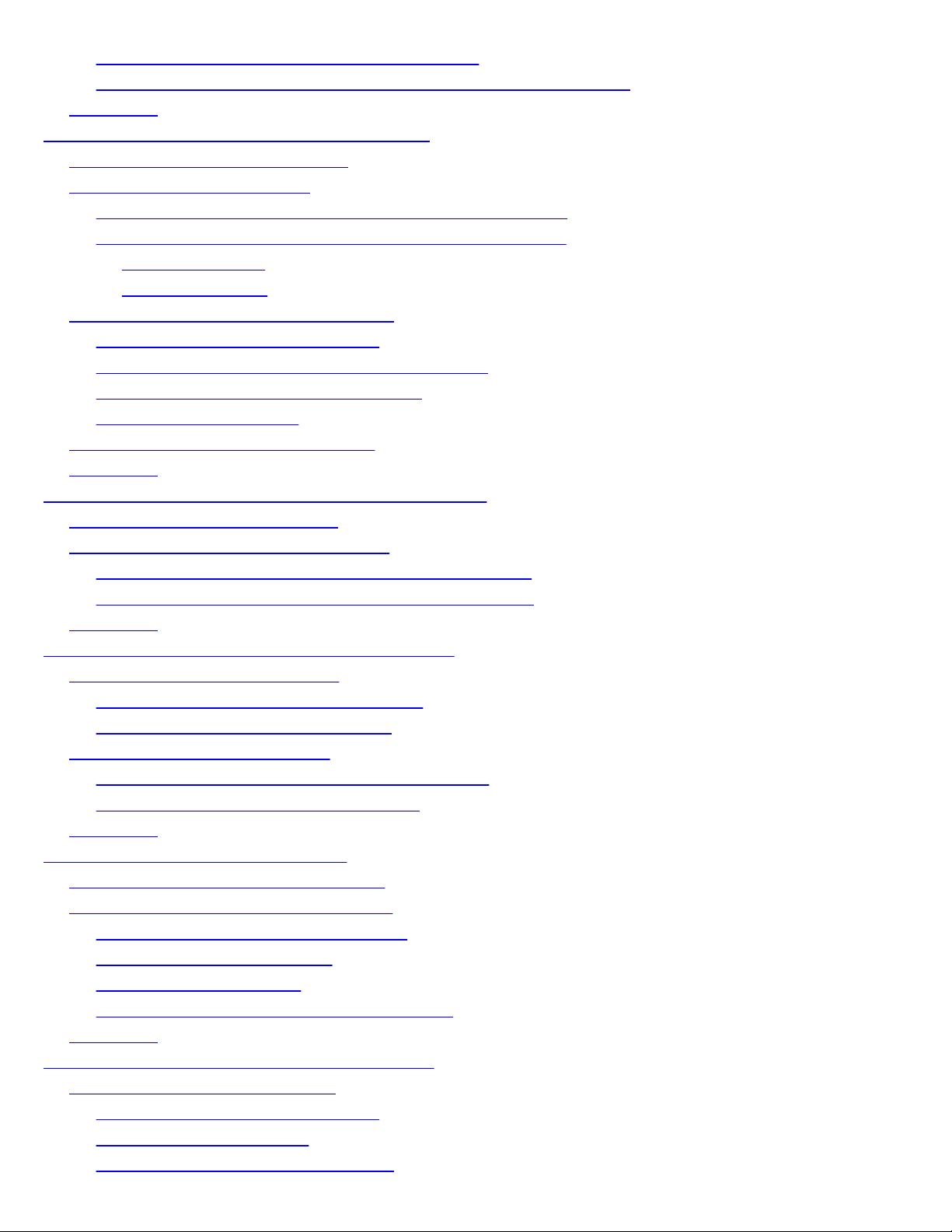
Makinganinteractivedismissaltransition
ImplementingacustomUINavigationControllertransition
Summary
5.ImprovingYourCodewithValueTypes
Understandingreferencetypes
Understandingvaluetypes
Differencesbetweenvaluesandreferencesinusage
Memorydifferencesbetweenvaluesandreferences
Heapallocation
Stackallocation
Usingstructstoimproveyourcode
Startingyourmodelsasstructs
Usingastructifyoudon'tneedinheritance
Enforcingimmutabilitywithstructs
Finalwordsonstructs
Containinginformationinenums
Summary
6.AvoidingComplexInheritancewithProtocols
Definingyourownprotocols
Checkingfortraitsinsteadoftypes
Extendingyourprotocolswithdefaultbehavior
Improvingyourprotocolswithassociatedtypes
Summary
7.RefactoringtheHelloContactsApplication
Properlyseparatingconcerns
Extractingthecontactfetchingcode
Extractingthebounceanimation
Addingprotocolsforclarity
Definingthevieweffectanimatorprotocol
Definingacontactdisplayprotocol
Summary
8.AddingCoreDatatoyourApp
UnderstandingtheCoreDatastack
AddingCoreDatatoanapplication
Modelingdatainthemodeleditor
Creatingthebasicmodels
Definingrelationships
CreatingNSManagedObjectsubclasses
Summary
9.StoringandQueryingDatainCoreData
InsertingdatawithCoreData
Understandingdatapersistence
Persistingyourmodels
Refactoringthepersistencecode