<?php
/*~ class.phpmailer.php
.---------------------------------------------------------------------------.
| Software: PHPMailer - PHP email class |
| Version: 5.1 |
| Contact: via sourceforge.net support pages (also www.worxware.com) |
| Info: http://phpmailer.sourceforge.net |
| Support: http://sourceforge.net/projects/phpmailer/ |
| ------------------------------------------------------------------------- |
| Admin: Andy Prevost (project admininistrator) |
| Authors: Andy Prevost (codeworxtech) codeworxtech@users.sourceforge.net |
| : Marcus Bointon (coolbru) coolbru@users.sourceforge.net |
| Founder: Brent R. Matzelle (original founder) |
| Copyright (c) 2004-2009, Andy Prevost. All Rights Reserved. |
| Copyright (c) 2001-2003, Brent R. Matzelle |
| ------------------------------------------------------------------------- |
| License: Distributed under the Lesser General Public License (LGPL) |
| http://www.gnu.org/copyleft/lesser.html |
| This program is distributed in the hope that it will be useful - WITHOUT |
| ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or |
| FITNESS FOR A PARTICULAR PURPOSE. |
| ------------------------------------------------------------------------- |
| We offer a number of paid services (www.worxware.com): |
| - Web Hosting on highly optimized fast and secure servers |
| - Technology Consulting |
| - Oursourcing (highly qualified programmers and graphic designers) |
'---------------------------------------------------------------------------'
*/
/**
* PHPMailer - PHP email transport class
* NOTE: Requires PHP version 5 or later
* @package PHPMailer
* @author Andy Prevost
* @author Marcus Bointon
* @copyright 2004 - 2009 Andy Prevost
* @version $Id: class.phpmailer.php 447 2009-05-25 01:36:38Z codeworxtech $
* @license http://www.gnu.org/copyleft/lesser.html GNU Lesser General Public License
*/
if (version_compare(PHP_VERSION, '5.0.0', '<') ) exit("Sorry, this version of PHPMailer will only run on PHP version 5 or greater!\n");
class PHPMailer {
/////////////////////////////////////////////////
// PROPERTIES, PUBLIC
/////////////////////////////////////////////////
/**
* Email priority (1 = High, 3 = Normal, 5 = low).
* @var int
*/
public $Priority = 3;
/**
* Sets the CharSet of the message.
* @var string
*/
public $CharSet = 'iso-8859-1';
/**
* Sets the Content-type of the message.
* @var string
*/
public $ContentType = 'text/plain';
/**
* Sets the Encoding of the message. Options for this are
* "8bit", "7bit", "binary", "base64", and "quoted-printable".
* @var string
*/
public $Encoding = '8bit';
/**
* Holds the most recent mailer error message.
* @var string
*/
public $ErrorInfo = '';
/**
* Sets the From email address for the message.
* @var string
*/
public $From = 'root@localhost';
/**
* Sets the From name of the message.
* @var string
*/
public $FromName = 'Root User';
/**
* Sets the Sender email (Return-Path) of the message. If not empty,
* will be sent via -f to sendmail or as 'MAIL FROM' in smtp mode.
* @var string
*/
public $Sender = '';
/**
* Sets the Subject of the message.
* @var string
*/
public $Subject = '';
/**
* Sets the Body of the message. This can be either an HTML or text body.
* If HTML then run IsHTML(true).
* @var string
*/
public $Body = '';
/**
* Sets the text-only body of the message. This automatically sets the
* email to multipart/alternative. This body can be read by mail
* clients that do not have HTML email capability such as mutt. Clients
* that can read HTML will view the normal Body.
* @var string
*/
public $AltBody = '';
/**
* Sets word wrapping on the body of the message to a given number of
* characters.
* @var int
*/
public $WordWrap = 0;
/**
* Method to send mail: ("mail", "sendmail", or "smtp").
* @var string
*/
public $Mailer = 'mail';
/**
* Sets the path of the sendmail program.
* @var string
*/
public $Sendmail = '/usr/sbin/sendmail';
/**
* Path to PHPMailer plugins. Useful if the SMTP class
* is in a different directory than the PHP include path.
* @var string
*/
public $PluginDir = '';
/**
* Sets the email address that a reading confirmation will be sent.
* @var string
*/
public $ConfirmReadingTo = '';
/**
* Sets the hostname to use in Message-Id and Received headers
* and as default HELO string. If empty, the value returned
* by SERVER_NAME is used or 'localhost.localdomain'.
* @var string
*/
public $Hostname = '';
/**
* Sets the message ID to be used in the Message-Id header.
* If empty, a unique id will be generated.
* @var string
*/
public $MessageID = '';
/////////////////////////////////////////////////
// PROPERTIES FOR SMTP
/////////////////////////////////////////////////
/**
* Sets the SMTP hosts. All hosts must be separated by a
* semicolon. You can also specify a different port
* for each host by using this format: [hostname:port]
* (e.g. "smtp1.example.com:25;smtp2.example.com").
* Hosts will be tried in order.
* @var string
*/
public $Host = 'localhost';
/**
* Sets the default SMTP server port.
* @var int
*/
public $Port = 25;
/**
* Sets the SMTP HELO of the message (Default is $Hostname).
* @var string
*/
public $Helo = '';
/**
* Sets connection prefix.
* Options are "", "ssl" or "tls"
* @var string
*/
public $SMTPSecure = '';
/**
* Sets SMTP authentication. Utilizes the Username and Password variables.
* @var bool
*/
public $SMTPAuth = false;
/**
* Sets SMTP username.
* @var string
*/
public $Username = '';
/**
* Sets SMTP password.
* @var string
*/
public $Password = '';
/**
* Sets the SMTP server timeout in seconds.
* This function will not work with the win32 version.
* @var int
*/
public $Timeout = 10;
/**
* Sets SMTP class debugging on or off.
* @var bool
*/
public $SMTPDebug = false;
/**
* Prevents the SMTP connection from being closed after each mail
* sending. If this is set to true then to close the connection
* requires an explicit call to SmtpClose().
* @var bool
*/
public $SMTPKeepAlive = false;
/**
* Provides the ability to have the TO field process individual
* emails, instead of sending to entire TO addresses
* @var bool
*/
public $SingleTo = false;
/**
* If SingleTo is true, this provides the array to hold the email addresses
* @var bool
*/
public $SingleToArray = array();
/**
* Provides the ability to change the line ending
* @var string
*/
public $LE = "\n";
/**
* Used with DKIM DNS Resource Record
* @var string
*/
public $DKIM_selector = 'phpmailer';
/**
* Used with DKIM DNS Resource Record
* optional, in format of email address 'y
没有合适的资源?快使用搜索试试~ 我知道了~
防乐享微盟源码(PHP源码)
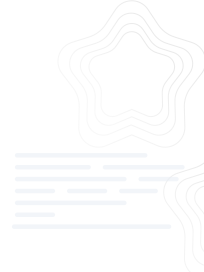
共2000个文件
png:610个
gif:494个
jpg:307个


温馨提示
微CMS平台的管理界面简单易用、操作方便,大量人性化设计,一分钟开启微信营销,无需安装任何软件,全自动“云”平台,多账号管理,只需要简单的设置即可完成复杂的微信营销推广功能。 功能强大 通过微CMS平台,用户可以轻松管理自己的微信各类信息,对微信公众账号进行维护、开展智能机器人、在线发优惠劵、抽奖、刮奖、派发会员卡、打造微官网、开启微团购等多种活动,对微信营销实现有效监控,在线优惠劵、转盘抽奖、微信会员卡等推广服务更是让微信成为商家推广的利器,智能客服的可调教功能让用户真正从微信繁琐的日常客服工作中解脱出来,真正成为商家便利的新营销渠道,极大扩展潜在客户群和实现企业的运营目标。 专业团队 一站式管理,平台支持,拥有多年网络营销经验的管理团队,强大的技术支持,专注于社会化媒体的研究,自有全国百万订阅量公众账号、全国主要城市区域号,以及微信电商团队实际操作,运营上海本地公众账号用户过万,熟练掌握获取本地用户的方法,不做整合营销,我们只专注于微信。
资源推荐
资源详情
资源评论
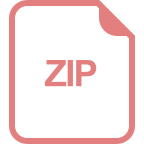
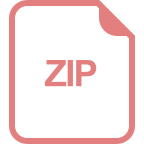
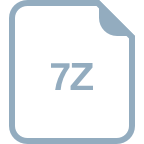
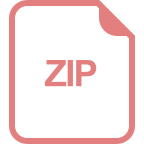
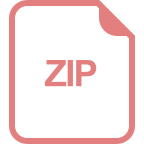
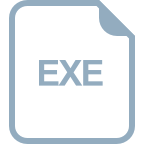
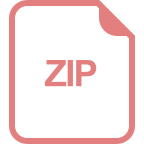
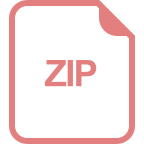
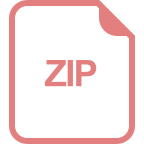
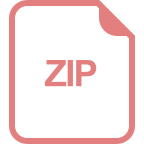
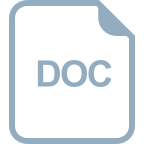
收起资源包目录

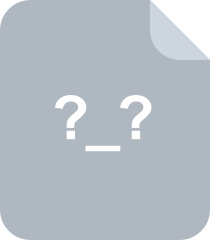
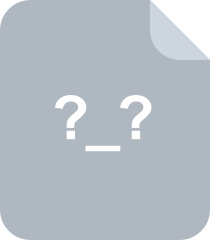
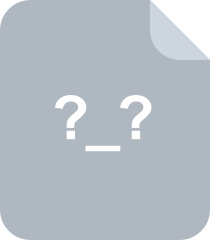
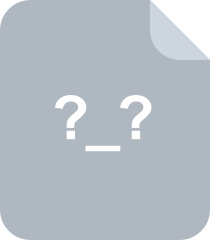
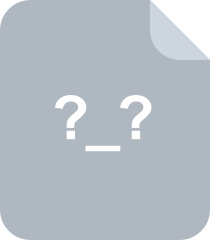
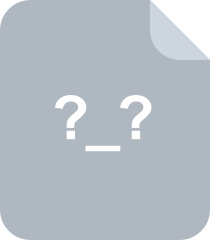
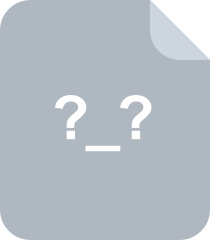
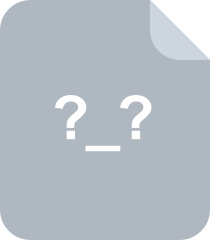
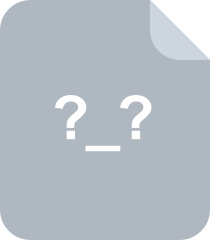
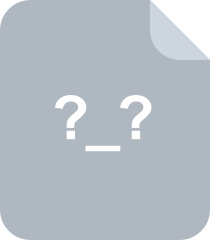
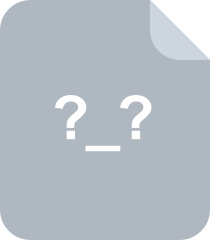
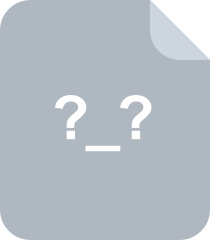
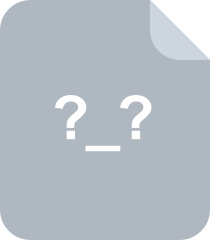
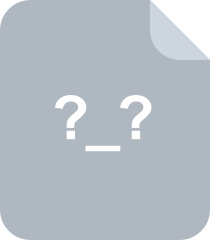
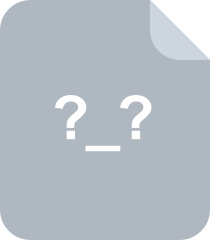
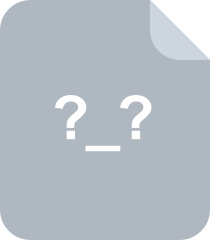
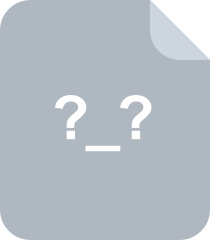
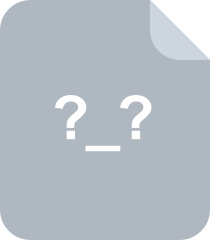
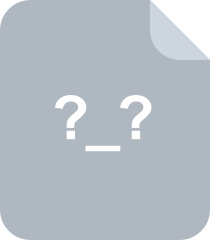
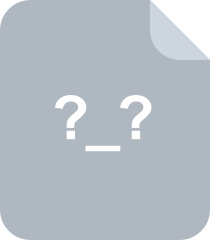
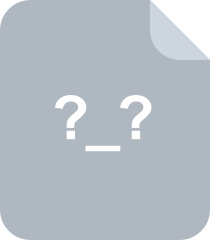
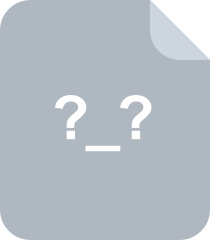
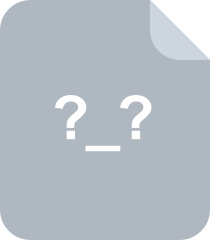
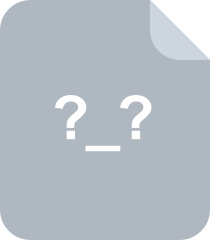
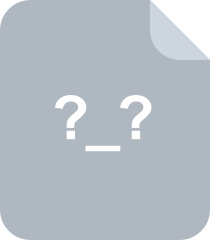
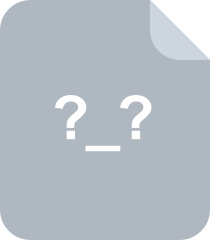
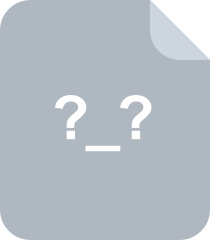
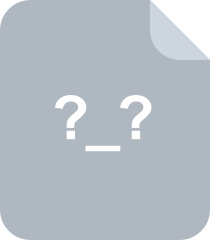
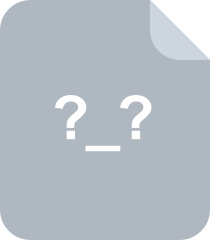
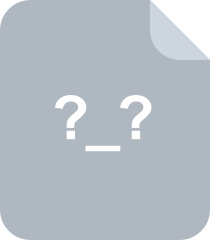
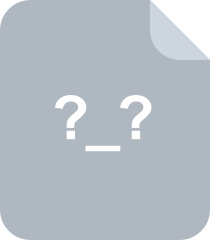
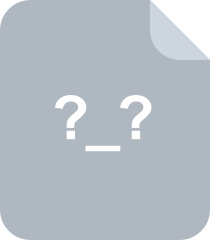
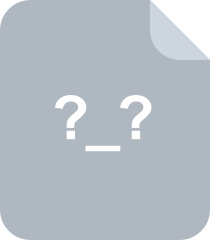
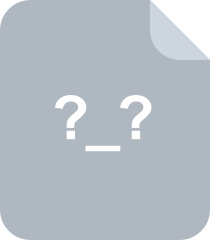
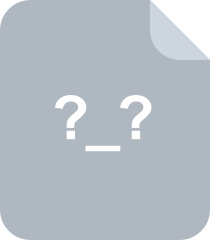
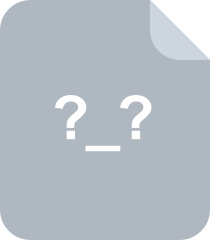
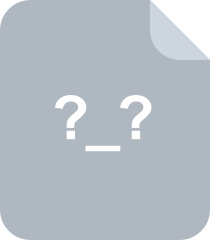
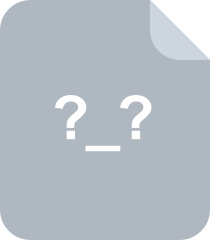
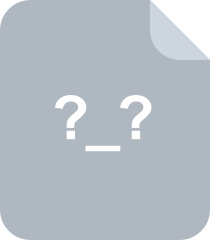
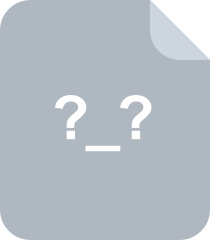
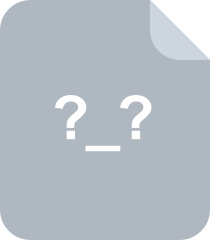
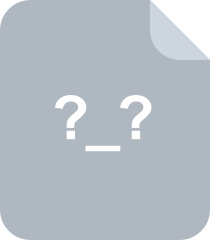
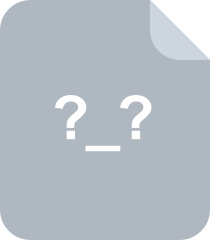
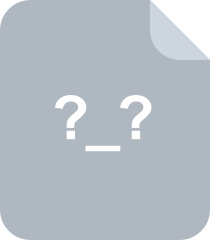
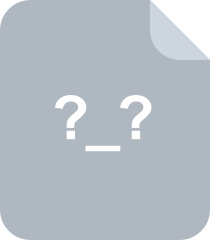
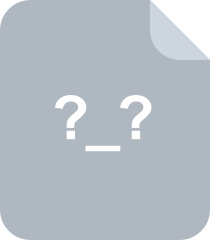
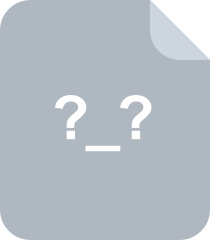
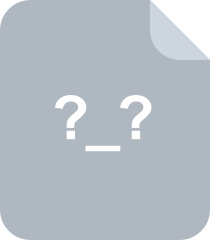
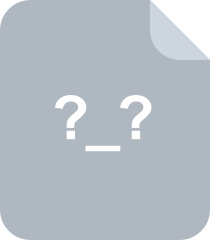
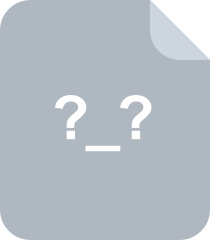
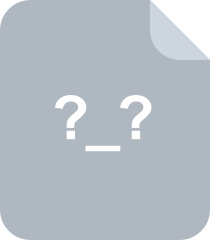
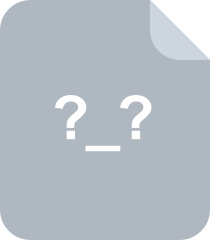
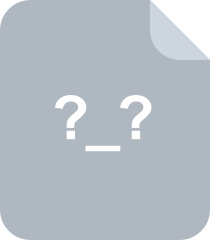
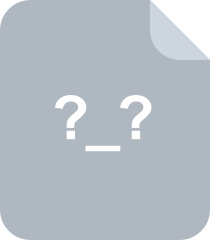
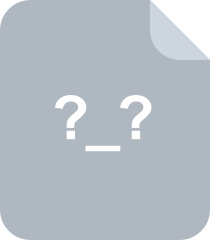
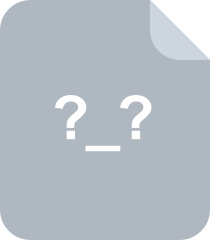
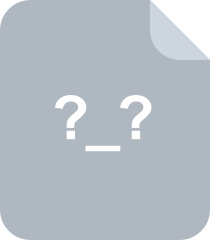
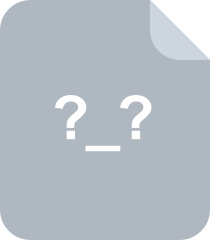
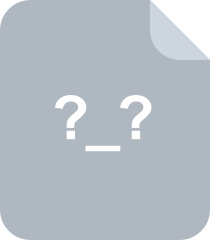
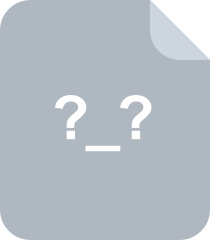
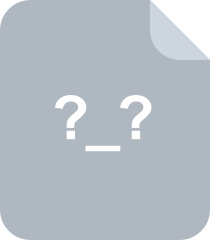
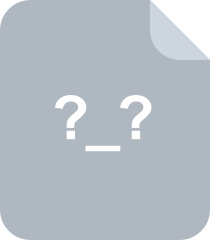
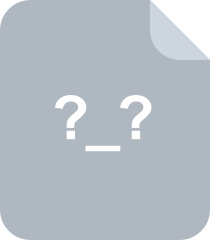
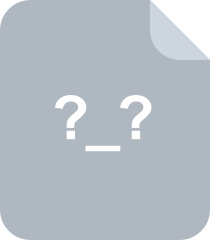
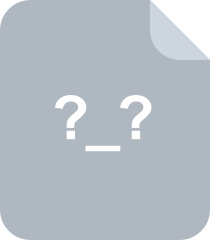
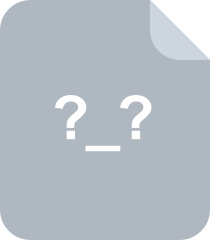
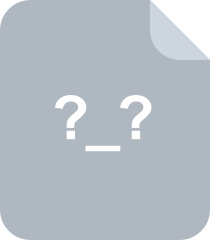
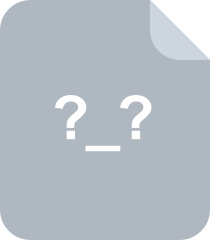
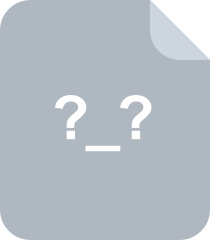
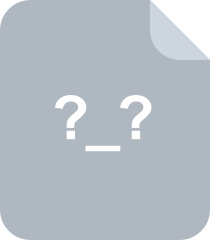
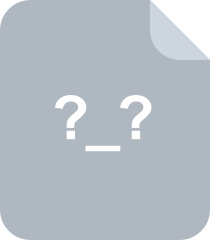
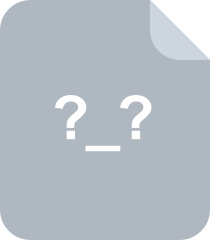
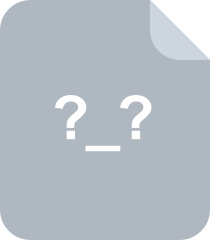
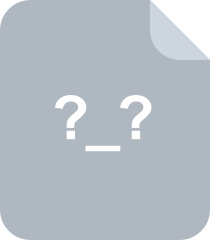
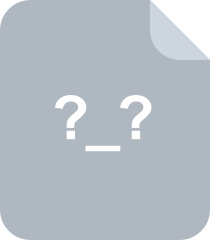
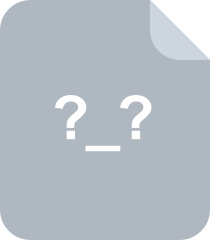
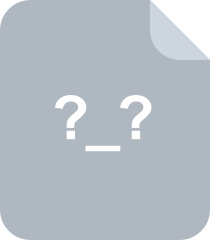
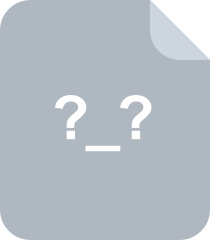
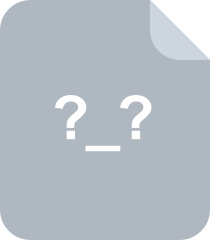
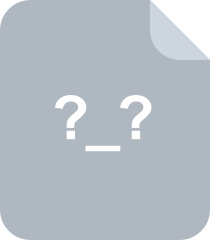
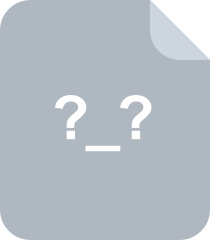
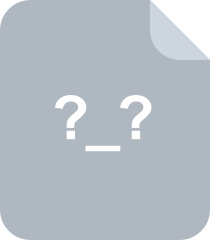
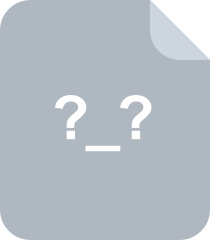
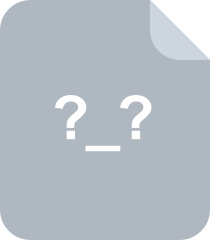
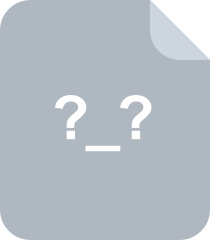
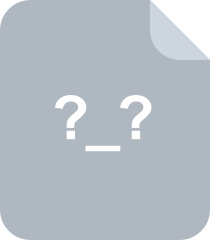
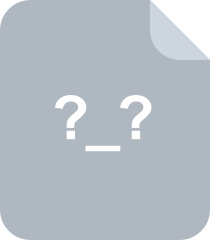
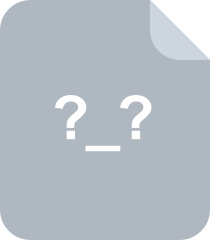
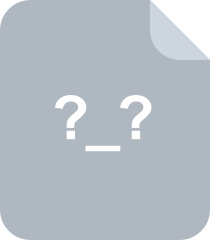
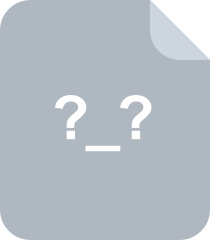
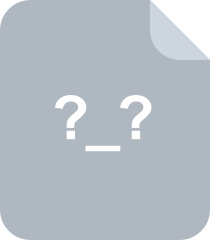
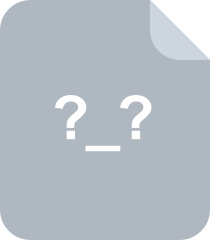
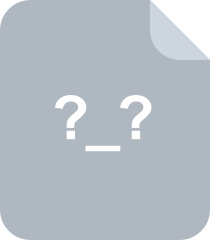
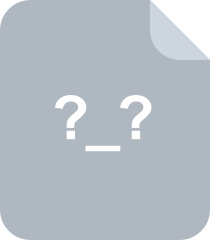
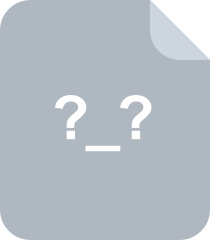
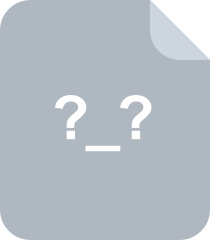
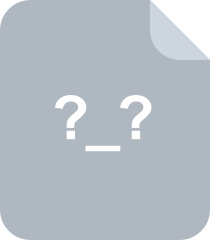
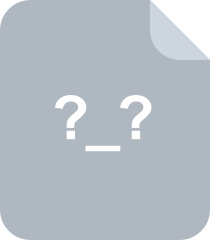
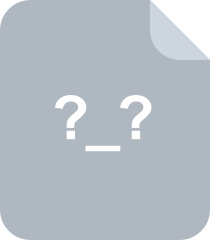
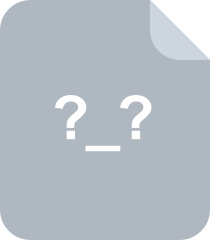
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20

usktwxdi
- 粉丝: 0
- 资源: 5
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

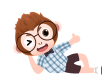
最新资源
- R语言机器学习指南PPT 44页
- 【java毕业设计】医院打卡挂号系统源码(ssm+jsp+mysql+说明文档+LW).zip
- 【java毕业设计】雅博书城在线系统源码(ssm+jsp+mysql+说明文档+LW).zip
- 基于spring+Sql server实现的题库及试卷管理系统模块的设计与开发(源码+数据库+毕业论文)
- 【java毕业设计】学生综合考评管理系统源码(ssm+jsp+mysql+说明文档+LW).zip
- 鸢尾花数据-数据集(文件)
- 俄罗斯方块游戏的C++源代码
- CIFAR10-数据集
- Kaggle生物信息学挑战:酶稳定性预测大赛
- 基于Servlet+jsp+Sql server实现的学校教务管理系统(源码+数据库+开题报告+程序使用说明书)
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


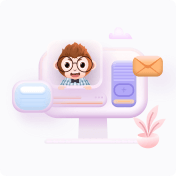
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
前往页