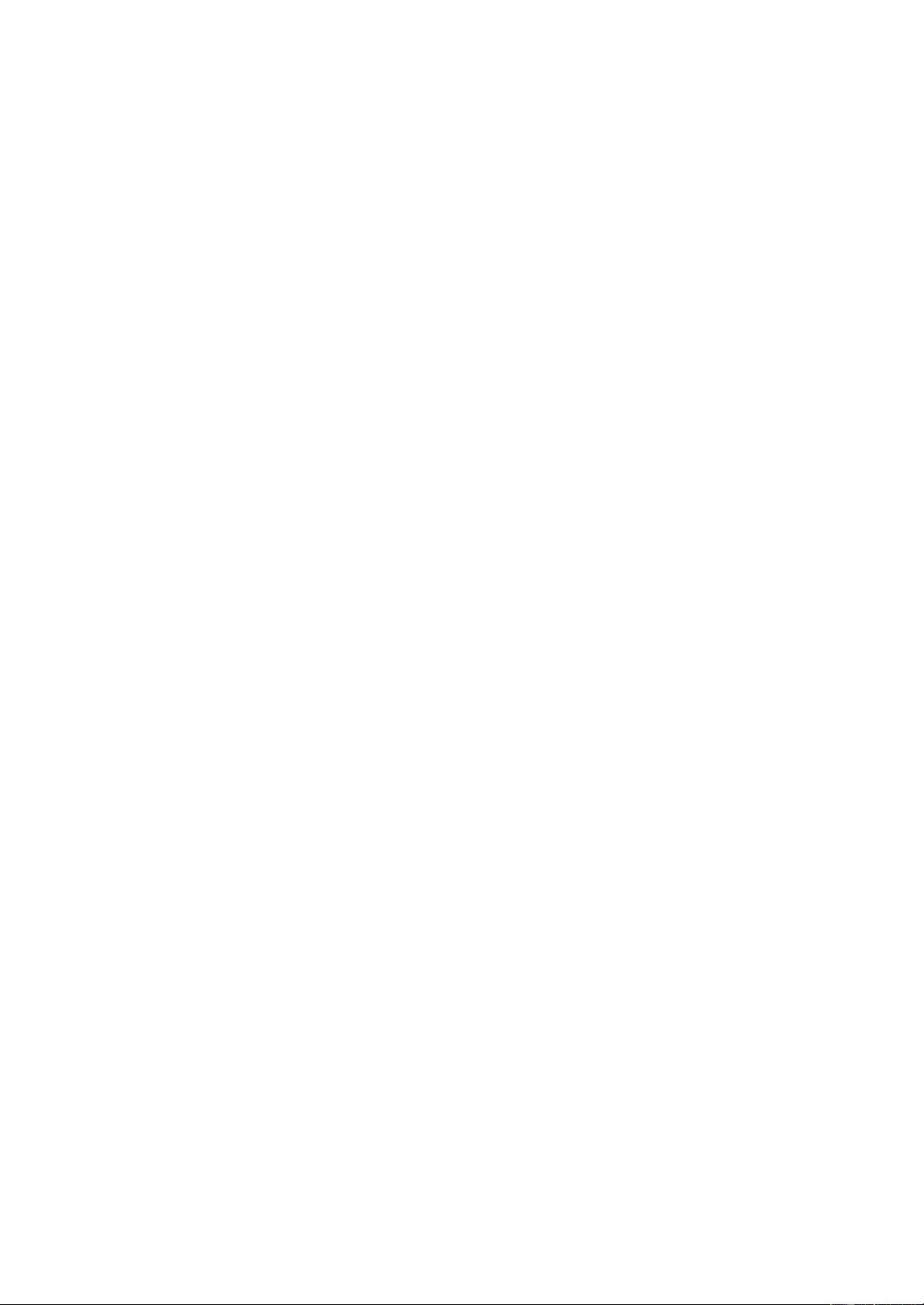
Reference manual
CvCam
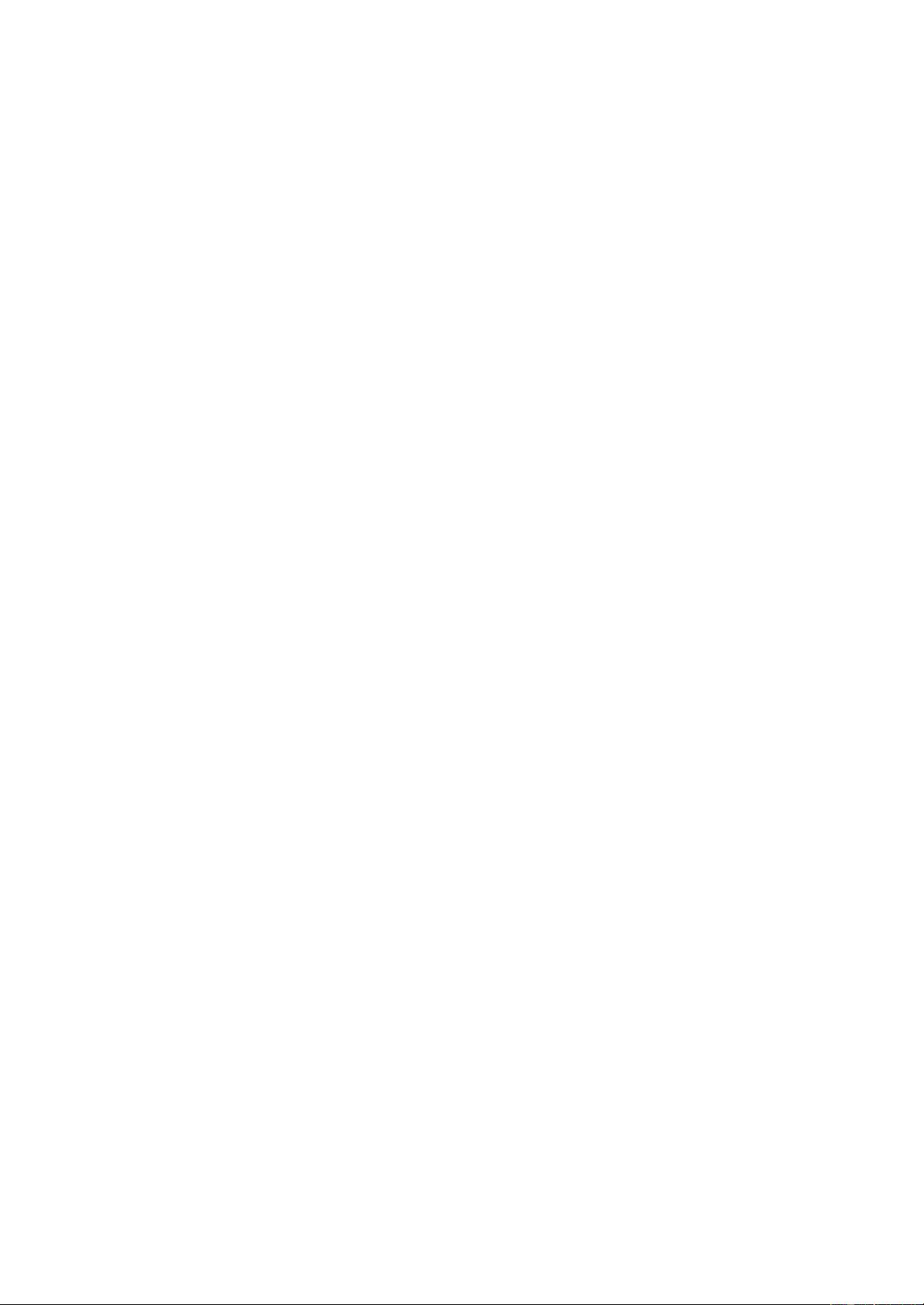
Contents
INTRODUCTION .........................................................................................................................3
COMMON USAGE .......................................................................................................................5
HOWTO: ........................................................................................................................................8
BEGIN WORK WITH CVCAM...........................................................................................................8
SELECT A CAMERA........................................................................................................................8
SET UP CAMERA(S)........................................................................................................................8
RENDER THE VIDEO STREAM.........................................................................................................8
MAKE THE SETTINGS ACTIVE........................................................................................................9
CONTROL THE VIDEO ....................................................................................................................9
DISPLAY CAMERAS PROPERTY PAGES ...........................................................................................9
PROCESS VIDEO FRAMES ...............................................................................................................9
GET THE POINTER TO LAST FRAME................................................................................................9
PLAY AN AVI FILE AND PROCESS IT’S FRAMES IF NEEDED ............................................................10
WORK WITH MULTIPLE CAMERAS UNDER LINUX.........................................................................10
WORK WITH MULTIPLE CAMERAS UNDER WINDOWS..................................................................10
FINISH WORKING WITH CVCAM...................................................................................................11
CVCAM REFERENCE ..............................................................................................................12
CVCAM API.................................................................................................................................12
cvcamExit...............................................................................................................................12
cvcamGetCamerasCount.......................................................................................................12
cvcamGetProperty.................................................................................................................12
cvcamInit................................................................................................................................13
cvcamPause...........................................................................................................................13
cvcamPlayAVI........................................................................................................................14
cvcamResume.........................................................................................................................15
cvcamSelectCamera...............................................................................................................15
cvcamSetProperty..................................................................................................................16
cvcamStart .............................................................................................................................16
cvcamStop..............................................................................................................................16
CVCAM PROPERTIES INTERFACE .................................................................................................17
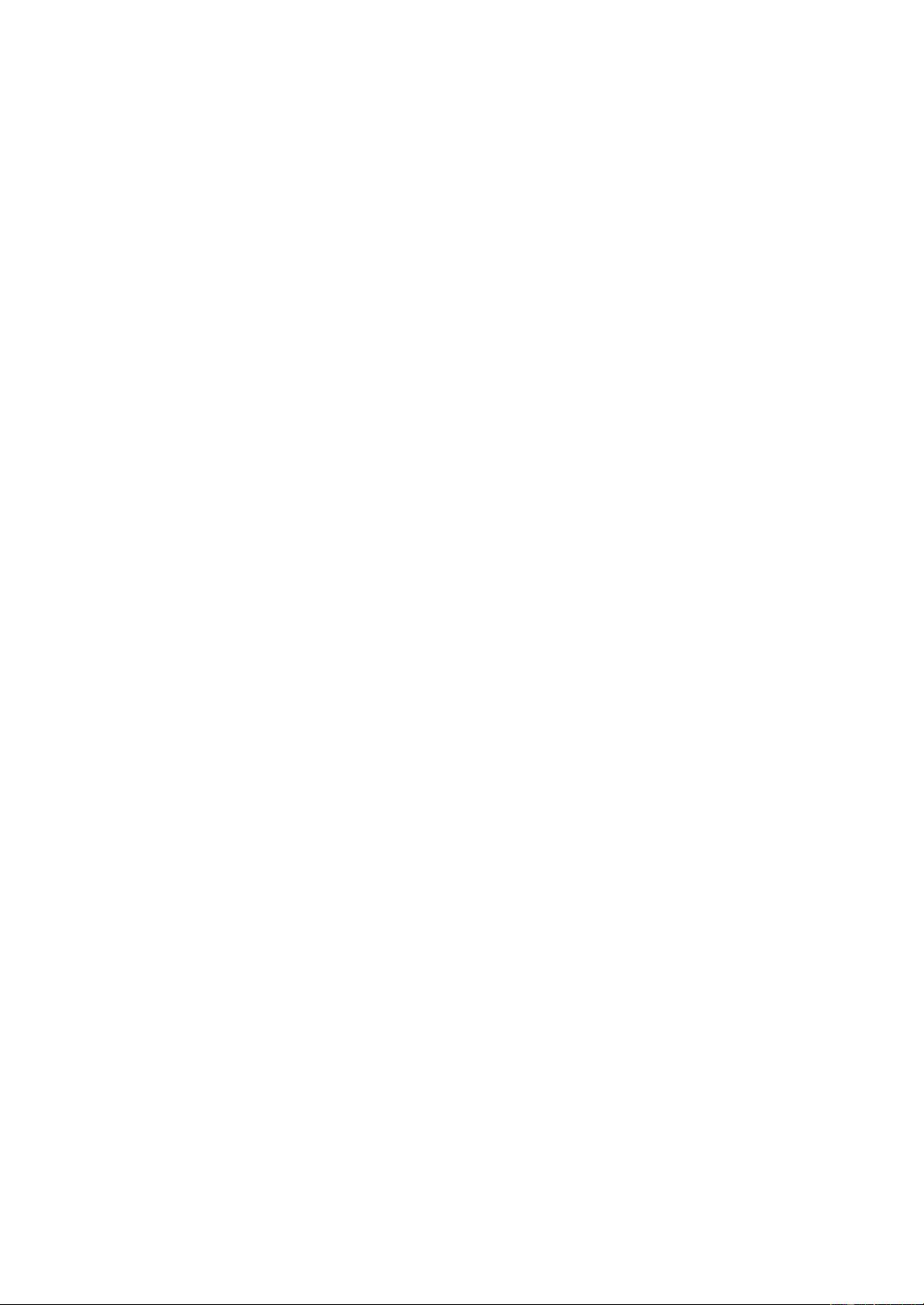
Introduction
CvCam is a universal cross-platform module for processing video stream from digital
video cameras. It is implemented as a dynamic link library (DLL) for Windows and as a shared
object library (so) for linux systems and provides a simple and convenient Application
Programming Interface (API) for reading and controlling video stream, processing its frames and
rendering the results. CvCam is distributed as a part of Intel’s OpenCV project under the same
license and uses some functionality of the Open Source Computer Vision Library.
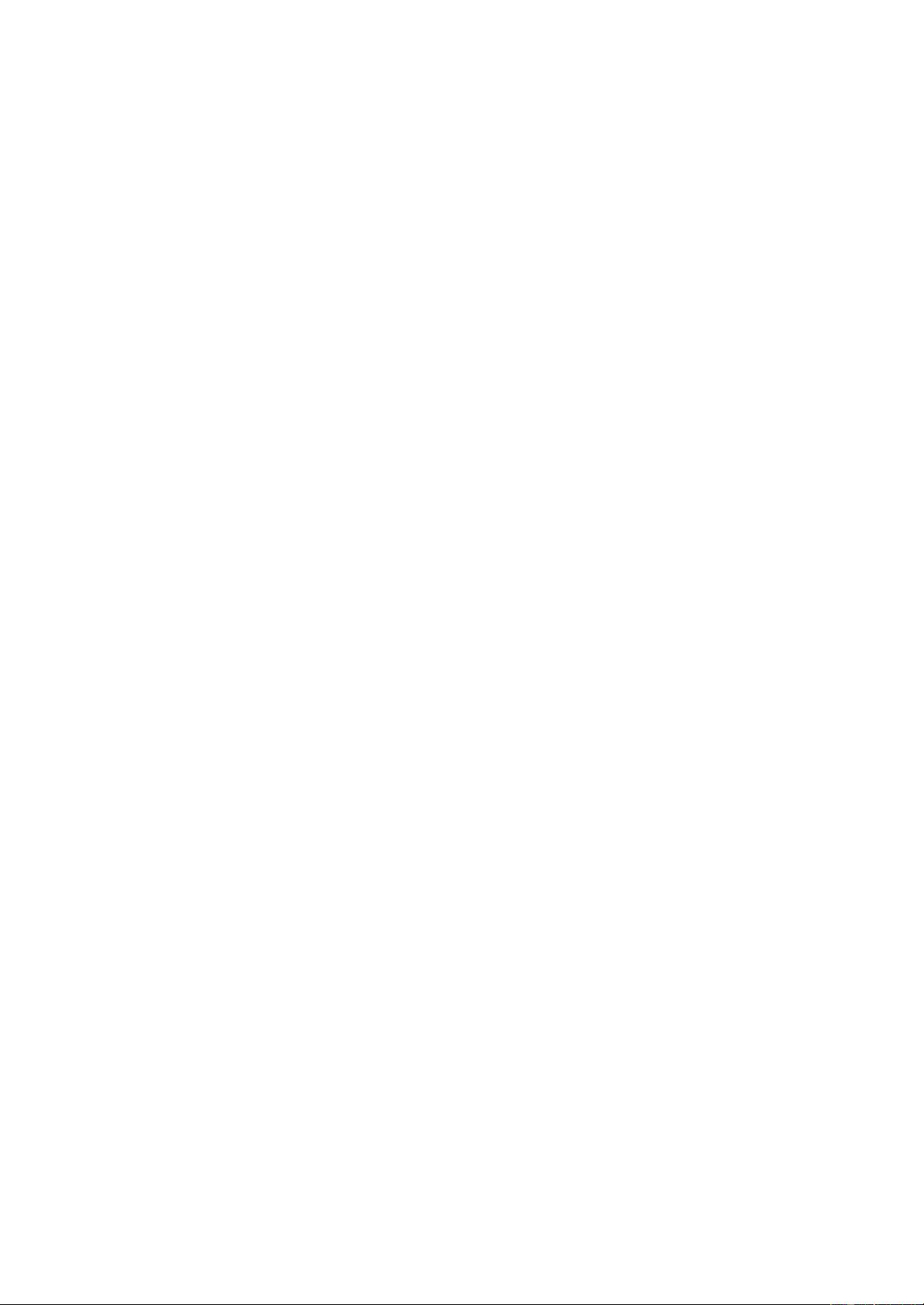
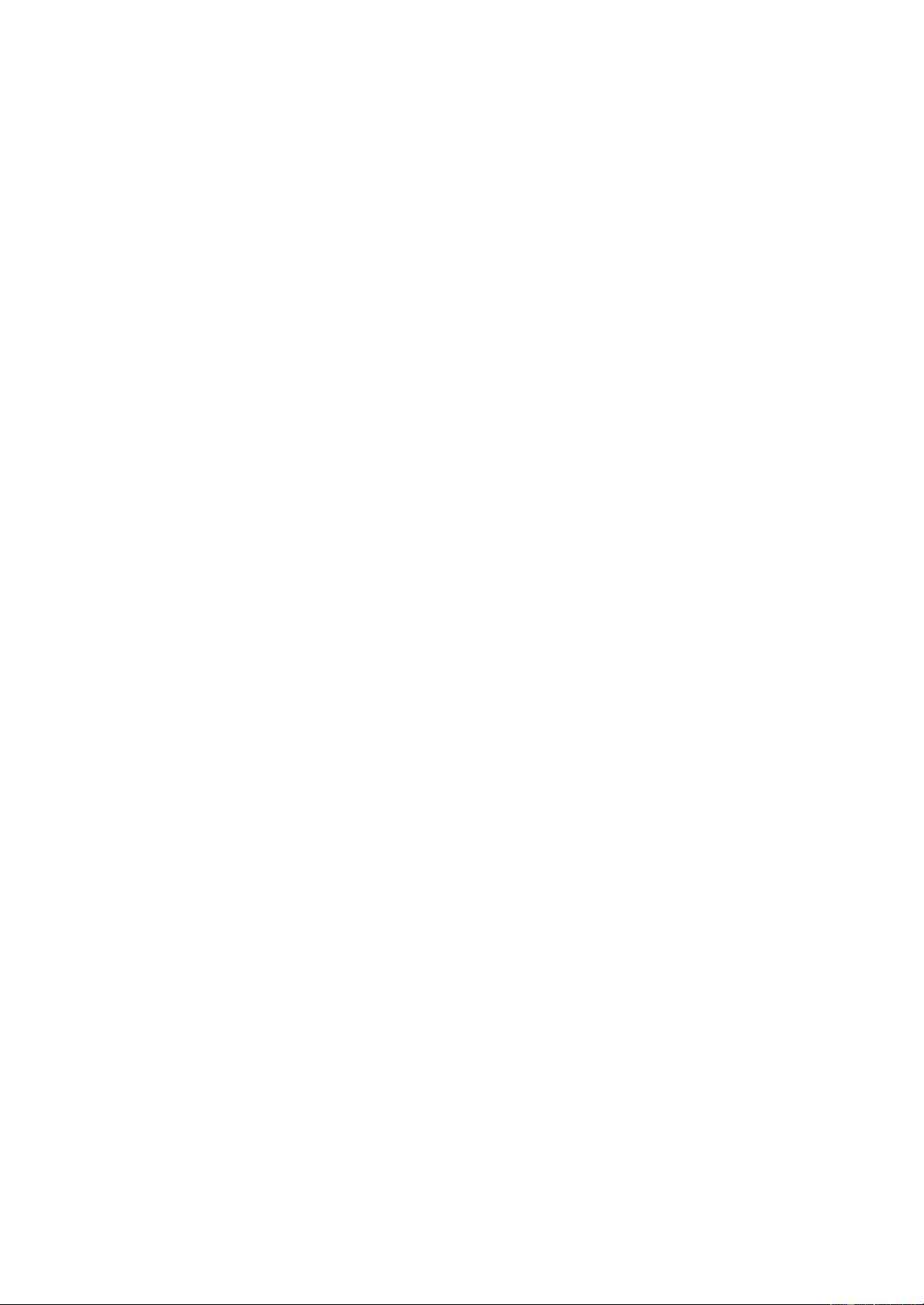
Common Usage
The simplest way to use CvCam from a C++ program for a single video source is shown
at the following listing:
#include “cvcam.h”
void callback(IplImage* image);
int main()
{
int ncams = cvcamGetCamerascount( );//returns the number of available cameras in the
//system
cvcamSetProperty(0, CVCAM_PROP_ENABLE, &cvcamone); //Selects the 1-st found
//camera
cvcamSetProperty(0, CVCAM_PROP_RENDER, &cvcamone) //We’ll render stream
//from this source
//here I assume your create a window and store it’s id in MyWin variable.
//MyWin is of type HWND on Windows and Window on linux
cvcamSetProperty(0, CVCAM_PROP_WINDOW, &MyWin); // Selects a window for
//video rendering
cvcamSetProperty(0, CVCAM_PROP_CALLBACK, callback);//this callback will
//process every frame
cvcamInit( );
cvcamStart( );
//Your app is working
cvcamStop( );
cvcamExit( );
return 0;
}
void callback(IplImage* image)//Draws blue horizontal lines across the image
{
IplImage* image1 = image;
int i,j;
assert (image);
for(i=0; i<image1->height; i+=10)
{
for(j=(image1->widthStep)*i; j<(image1->widthStep)*(i+1);
j+=image1->nChannels)
{
image1->imageData[j] = (char)255;
image1->imageData[j+1] = 0;
image1->imageData[j+2] = 0;
}
}
}
The first function to be called is cvcamGetCamerasCount( ); It prepares library and returns the
number of available cameras at the system. On Linux it’s also important to call it before your
initialize the X window system as it prepares X to work with linux threads. Your can also call
XInitThreads( ) at the beginning of your app instead.