package com.example.demo.utils;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.net.MalformedURLException;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.zip.ZipEntry;
import java.util.zip.ZipOutputStream;
import com.itextpdf.text.*;
import com.itextpdf.text.pdf.BaseFont;
import com.itextpdf.text.pdf.ColumnText;
import com.itextpdf.text.pdf.PdfAnnotation;
import com.itextpdf.text.pdf.PdfContentByte;
import com.itextpdf.text.pdf.PdfImportedPage;
import com.itextpdf.text.pdf.PdfPTable;
import com.itextpdf.text.pdf.PdfPageEventHelper;
import com.itextpdf.text.pdf.PdfReader;
import com.itextpdf.text.pdf.PdfStamper;
import com.itextpdf.text.pdf.PdfTransition;
import com.itextpdf.text.pdf.PdfWriter;
import com.itextpdf.text.pdf.draw.DottedLineSeparator;
import com.itextpdf.text.pdf.draw.LineSeparator;
import com.itextpdf.text.pdf.draw.VerticalPositionMark;
public class PDFUtils {
public static final String FILE_DIR = "E:\\home\\";
public static final String const_JPG_JAVA = FILE_DIR + "danren.jpg";
public static final String const_JPG_NGINX = FILE_DIR + "hongse.jpg";
private static final String const_NEZHA = "哪吒编程";
private static final String const_NEZHA_PROGRAM = "获取Java学习资料请关注公众号:哪吒编程";
private static final String const_BIBIDONG = "比比东";
private static final String const_YUNYUN = "云韵";
private static final String const_BAIDU = "百度一下 你就知道";
private static final String const_BAIDU_URL = "https://www.baidu.com";
private static final String const_PAGE_FIRST = "第一页";
private static final String const_PAGE_SECOND = "第二页";
private static final String const_PAGE_THIRD = "第三页";
private static final String const_PAGE_FOUR = "第四页";
private static final String const_PAGE_FIVE = "第五页";
private static final String const_TITLE_FIRST = "一级标题";
private static final String const_TITLE_SECOND = "二级标题";
private static final String const_CONTENT = "内容";
// 普通中文字体
public static Font static_FONT_CHINESE = null;
// 超链字体
public static Font static_FONT_LINK = null;
private static void pdfFontInit() throws IOException, DocumentException {
// 微软雅黑
BaseFont chinese = BaseFont.createFont("STSong-Light", "UniGB-UCS2-H", BaseFont.NOT_EMBEDDED);
// 普通中文字体
static_FONT_CHINESE = new Font(chinese, 12, Font.NORMAL);// Font.BOLD为加粗
// 超链字体
static_FONT_LINK = new Font(chinese, 12, Font.NORMAL, BaseColor.BLUE);
}
public static Document document;
public void createPDFFile() throws Exception {
pdfFontInit();
//createPDF();// 生成一个 PDF 文件
///createPDFWithColor();// 设置PDF的页面大小和背景颜色
//createPDFWithPassWord();// 创建带密码的PDF
//createPDFWithNewPages();// 为PDF添加页
//createPDFWithWaterMark();// 为PDF文件添加水印,背景图
//createPDFWithContent();//插入块Chunk, 内容Phrase, 段落Paragraph, List
//createPDFWithExtraContent();//插入Anchor, Image, Chapter, Section
draw();//画图
createPDFWithAlignment();//设置段落
//createPDFToDeletePage();//删除 page
//insertPage();// 插入 page
//splitPDF();//分割 page
//mergePDF();// 合并 PDF 文件
//sortpage();// 排序page
//setHeaderFooter();// 页眉,页脚
//addColumnText();// 左右文字
//setView();// 文档视图
//pdfToZip();// 压缩PDF到Zip
//addAnnotation();// 注释
}
/**
* 创建一个 PDF 文件,并添加文本
*/
public static void createPDF() throws IOException, DocumentException {
// 实例化 document
document = new Document();
// 生成文件
String path = FILE_DIR + "createPDF.pdf";
File file = new File(path);
if(!file.exists()){
file.createNewFile();
}
PdfWriter.getInstance(document, new FileOutputStream(FILE_DIR + "createPDF.pdf"));
// 打开 document
document.open();
// 添加文本 此处无法写入中文 TODO
document.add(new Paragraph(const_NEZHA));
document.add(new Paragraph("什么情况,我这是第一个PDF文件!", static_FONT_CHINESE));
document.add(new Paragraph(const_NEZHA, static_FONT_CHINESE));
// 关闭 document
document.close();
}
/**
* 创建PDF文件,修改文件的属性
*/
public static void createPDFWithColor() throws FileNotFoundException, DocumentException {
// 页面大小 PageSize.A5.rotate()
Rectangle rect = new Rectangle(PageSize.A4.rotate());
// 页面背景色
rect.setBackgroundColor(BaseColor.YELLOW);
document = new Document(rect);
PdfWriter writer = PdfWriter.getInstance(document, new FileOutputStream(FILE_DIR + "createPDFWithColor.pdf"));
// PDF版本(默认1.4)
writer.setPdfVersion(PdfWriter.VERSION_1_6);
// 文档属性
document.addAuthor(const_NEZHA);
document.addTitle("我的第一个pdf");
// 页边空白
document.setMargins(10, 10, 10, 10);
// 打开
document.open();
document.add(new Paragraph("重新打开文件并进行填写信息!", static_FONT_CHINESE));
// 关闭
document.close();
}
/**
* 创建带密码的PDF
*/
public static void createPDFWithPassWord() throws FileNotFoundException, DocumentException {
// 页面大小
Rectangle rect = new Rectangle(PageSize.A5.rotate());
// 页面背景色
rect.setBackgroundColor(BaseColor.YELLOW);
document = new Document(rect);
PdfWriter writer = PdfWriter.getInstance(document, new FileOutputStream(FILE_DIR + "createPDFWithPassWord.pdf"));
// userPassword打开密码:"123"
// ownerPassword编辑密码: "123456"
writer.setEncryption("123".getBytes(), "123456".getBytes(), PdfWriter.ALLOW_SCREENREADERS, PdfWriter.STANDARD_ENCRYPTION_128);
document.open();
document.add(new Paragraph(const_NEZHA, static_FONT_CHINESE));
document.close();
}
/**
* 为PDF添加页
*/
public static void createPDFWithNewPages() throws FileNotFoundException, DocumentException {
document = new Document();
PdfWriter writer = PdfWriter.getInstance(document, new FileOutputStream(FILE_DIR + "createPDFAddNewPages.pdf"));
document.open();
document.add(new Paragraph(const_PAGE_FIRST, static_FONT_CHINESE));
document.newPage();
document.add(new Paragraph(const_PAGE_SECOND, static_FONT_CHINESE));
writer.setPageEmpty(true);
document.newPage();
document.add(new Paragraph(const_PAGE_THIRD, static_FONT_CHINESE));
document.close();
}
/**
* 为PDF文件添加水印,背景图
*/
public static void createPDFWithWaterMark() throws IOException, DocumentException {
FileOutputStream out = new FileOutputStream(FILE_DIR + "createPDFWithWaterMark.pdf");
document = new Document();
PdfWriter.getInstance(document, out);
document.open();
document.add(new Paragraph(const_PAGE_FIRST, static_FONT_CHINESE));
document.newPage();
document.add(new Paragraph(const_PAGE_SECOND, static_FONT_CHINESE));
document.newPage();
document.add(new Paragraph(const_PAGE_THIRD, static_FONT_CH
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
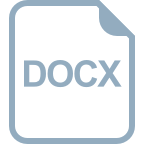
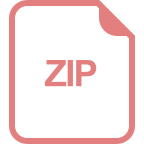
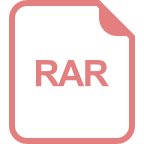
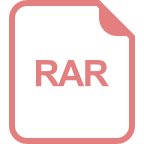
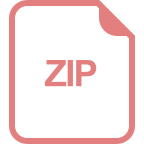
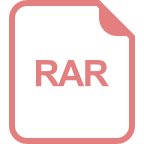
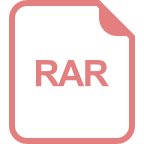
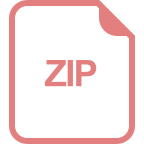
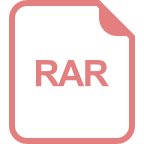
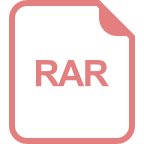
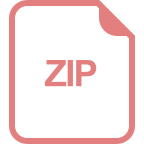
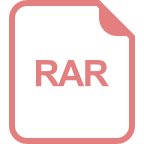
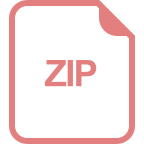
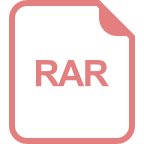
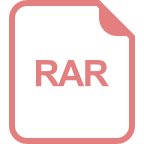
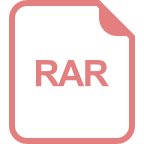
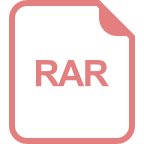
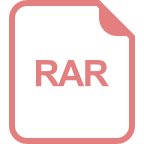
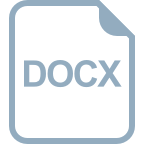
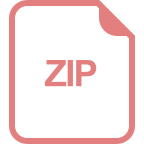
收起资源包目录

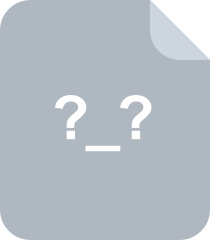
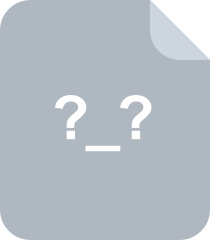
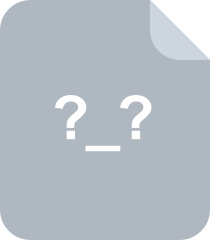
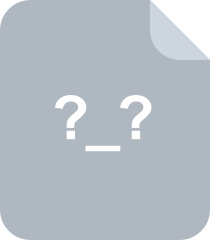
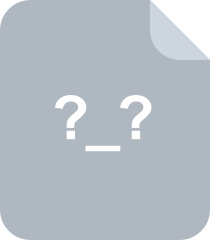
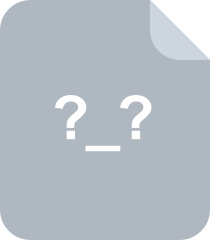
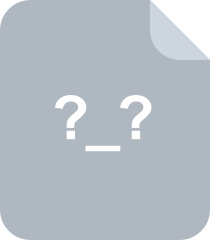
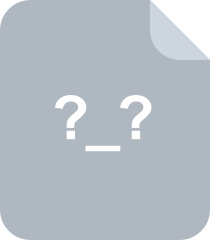
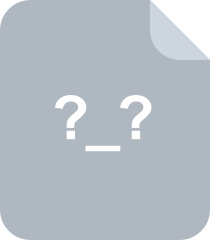
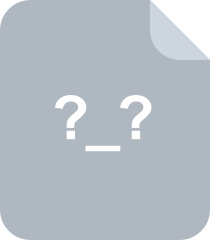
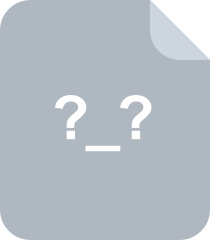
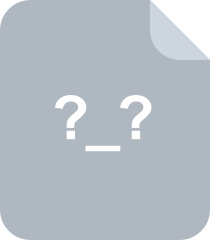
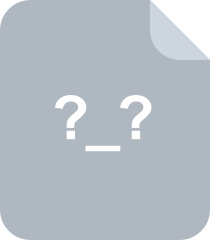
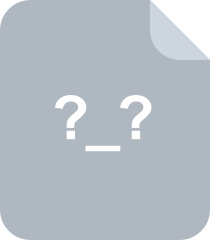
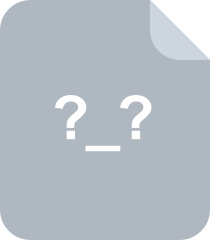
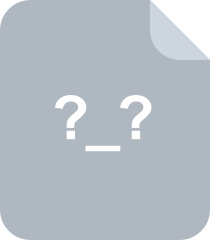
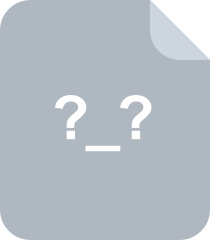
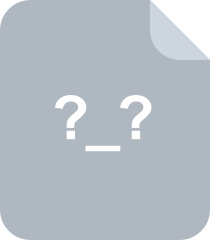
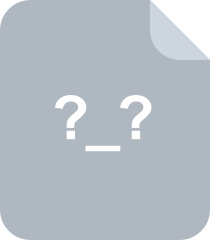
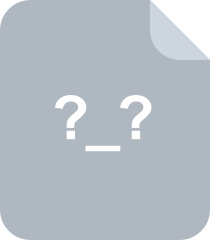
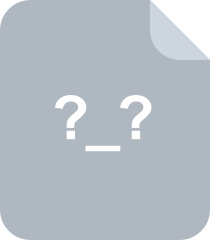
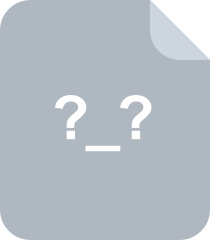
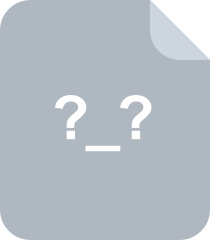
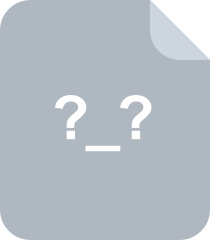
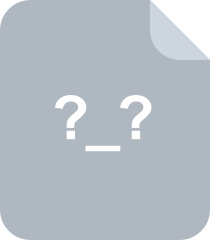
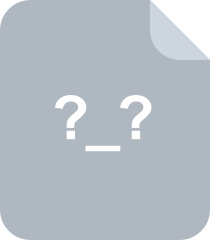
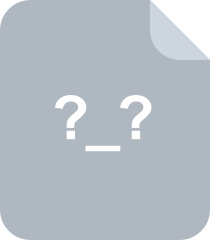
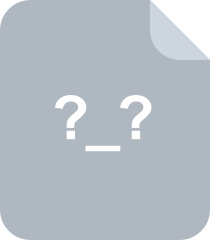
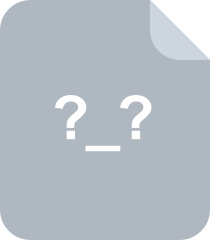
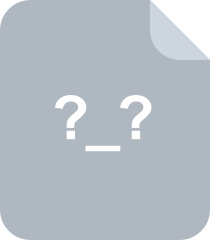
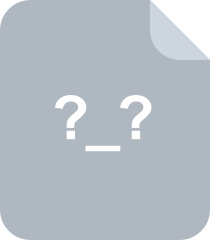
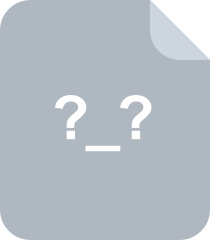
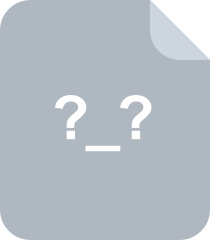
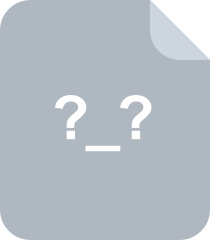
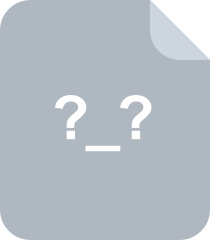
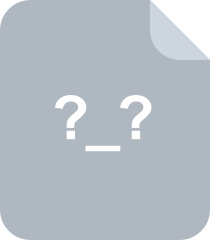
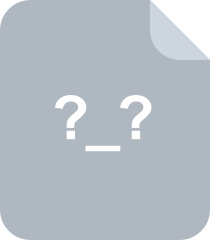
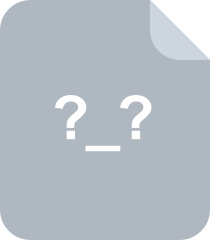
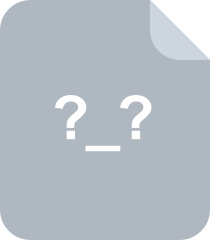
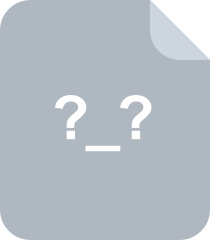
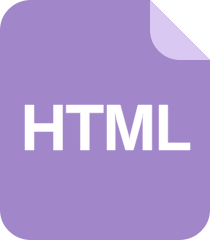
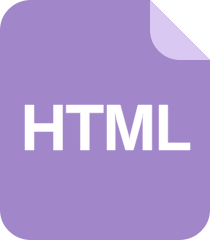
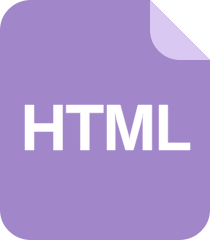
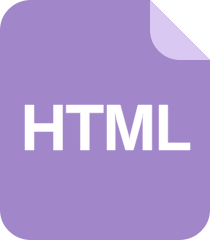
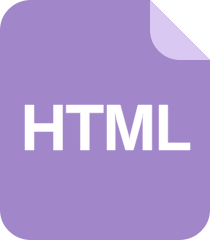
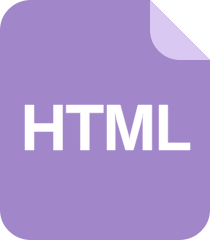
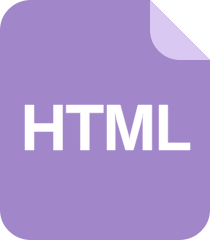
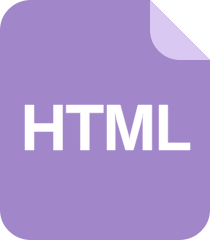
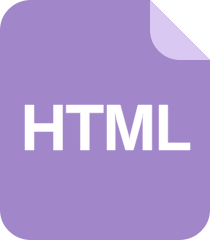
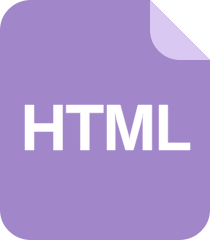
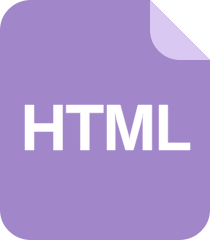
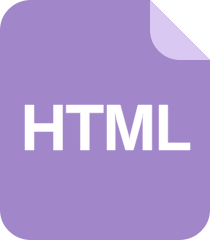
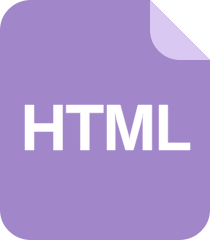
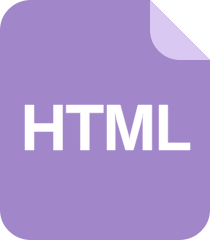
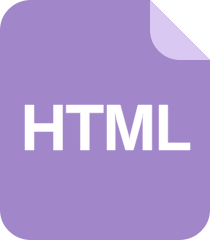
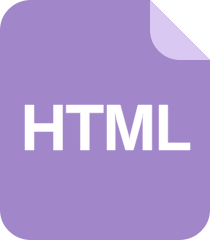
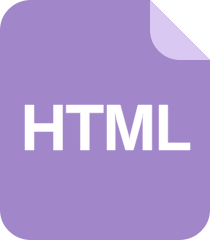
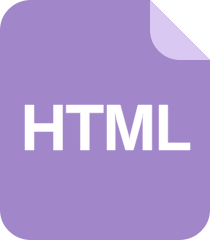
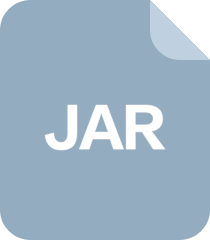
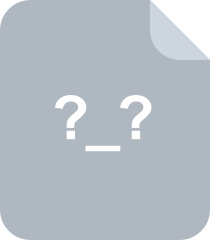
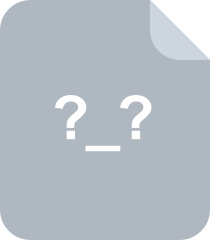
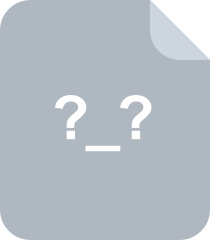
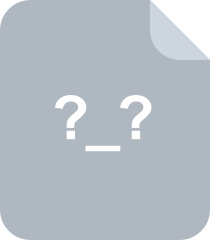
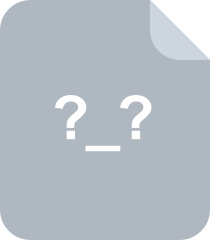
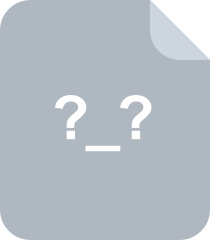
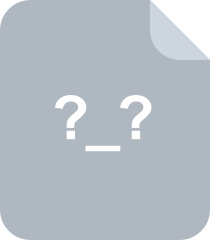
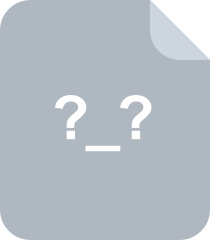
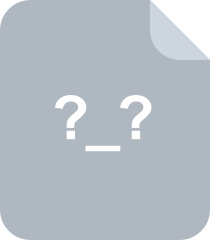
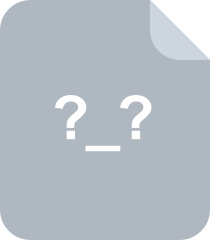
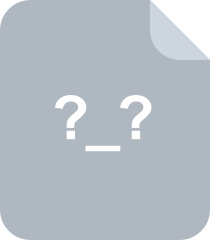
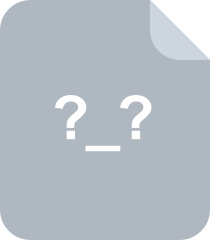
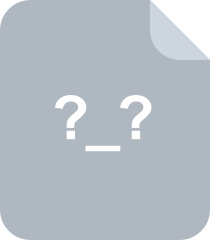
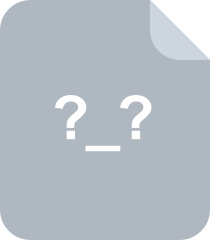
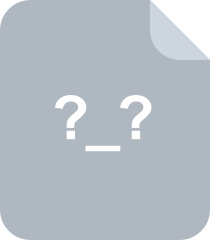
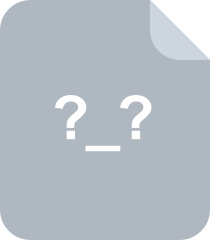
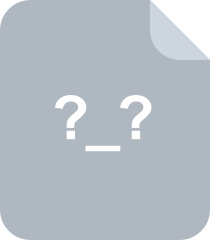
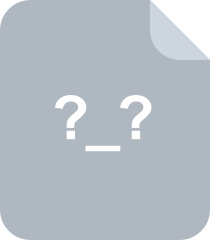
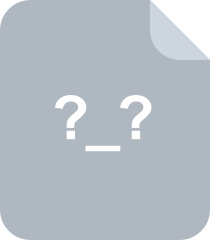
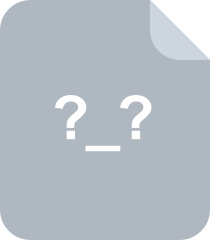
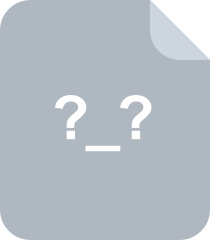
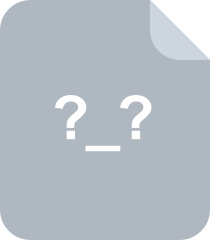
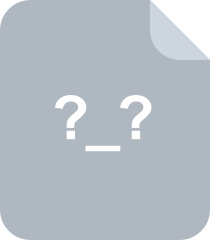
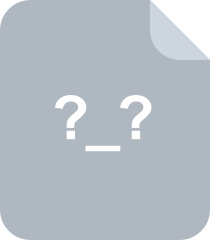
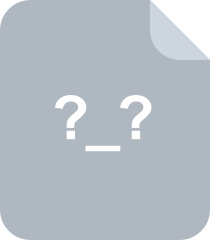
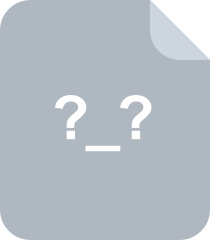
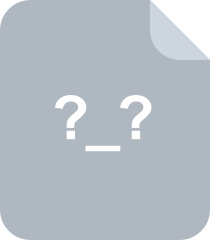
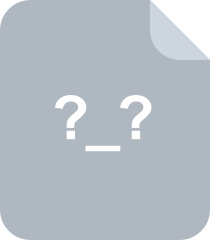
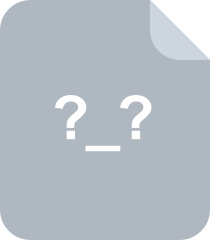
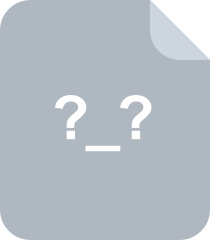
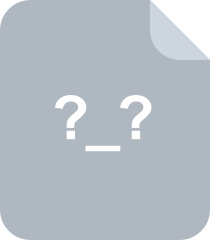
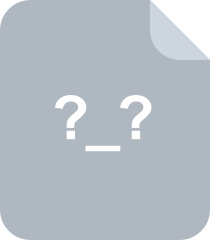
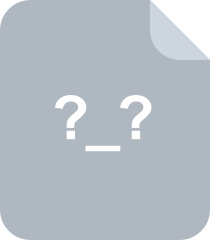
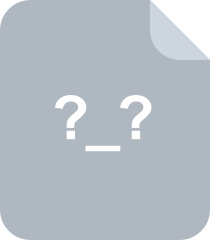
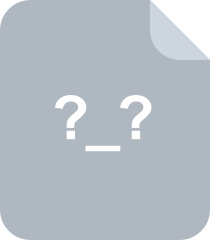
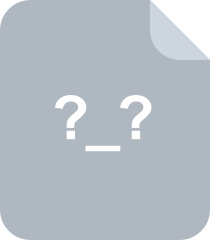
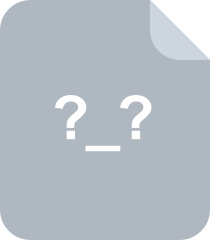
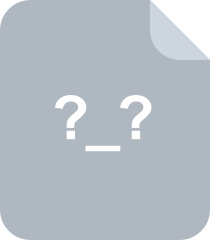
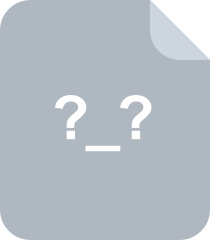
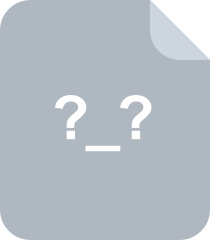
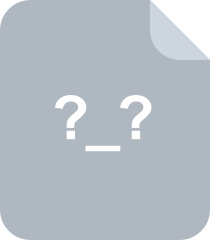
共 114 条
- 1
- 2
资源评论
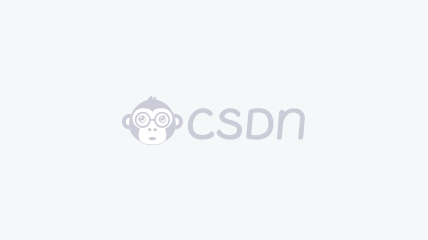

涛声露语
- 粉丝: 1
- 资源: 17
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

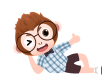
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


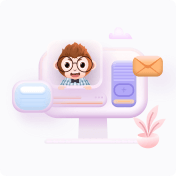
安全验证
文档复制为VIP权益,开通VIP直接复制
