package com.controller;
import java.text.SimpleDateFormat;
import com.alibaba.fastjson.JSONObject;
import java.util.*;
import org.springframework.beans.BeanUtils;
import javax.servlet.http.HttpServletRequest;
import org.springframework.web.context.ContextLoader;
import javax.servlet.ServletContext;
import com.service.TokenService;
import com.utils.StringUtil;
import java.lang.reflect.InvocationTargetException;
import org.apache.commons.lang3.StringUtils;
import com.annotation.IgnoreAuth;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.*;
import com.baomidou.mybatisplus.mapper.EntityWrapper;
import com.baomidou.mybatisplus.mapper.Wrapper;
import com.entity.YonghuEntity;
import com.service.YonghuService;
import com.entity.view.YonghuView;
import com.utils.PageUtils;
import com.utils.R;
/**
* 学生
* 后端接口
* @author
* @email
* @date 2021-03-02
*/
@RestController
@Controller
@RequestMapping("/yonghu")
public class YonghuController {
private static final Logger logger = LoggerFactory.getLogger(YonghuController.class);
@Autowired
private YonghuService yonghuService;
@Autowired
private TokenService tokenService;
//级联表service
//字典表map
Map<String, Map<Integer, String>> dictionaryMap;
/**
* 后端列表
*/
@RequestMapping("/page")
public R page(@RequestParam Map<String, Object> params, HttpServletRequest request){
logger.debug("page方法:,,Controller:{},,params:{}",this.getClass().getName(),JSONObject.toJSONString(params));
PageUtils page = yonghuService.queryPage(params);
//字典表数据转换
List<YonghuView> list =(List<YonghuView>)page.getList();
ServletContext servletContext = ContextLoader.getCurrentWebApplicationContext().getServletContext();
dictionaryMap = (Map<String, Map<Integer, String>>) servletContext.getAttribute("dictionaryMap");
for(YonghuView c:list){
this.dictionaryConvert(c);
}
return R.ok().put("data", page);
}
/**
* 后端详情
*/
@RequestMapping("/info/{id}")
public R info(@PathVariable("id") Long id){
logger.debug("info方法:,,Controller:{},,id:{}",this.getClass().getName(),id);
YonghuEntity yonghu = yonghuService.selectById(id);
if(yonghu !=null){
//entity转view
YonghuView view = new YonghuView();
BeanUtils.copyProperties( yonghu , view );//把实体数据重构到view中
//字典表字典转换
ServletContext servletContext = ContextLoader.getCurrentWebApplicationContext().getServletContext();
dictionaryMap = (Map<String, Map<Integer, String>>) servletContext.getAttribute("dictionaryMap");
this.dictionaryConvert(view);
return R.ok().put("data", view);
}else {
return R.error(511,"查不到数据");
}
}
/**
* 后端保存
*/
@RequestMapping("/save")
public R save(@RequestBody YonghuEntity yonghu, HttpServletRequest request){
logger.debug("save方法:,,Controller:{},,yonghu:{}",this.getClass().getName(),yonghu.toString());
Wrapper<YonghuEntity> queryWrapper = new EntityWrapper<YonghuEntity>()
.eq("username", yonghu.getUsername())
.eq("password", yonghu.getPassword())
.eq("name", yonghu.getName())
.eq("phone", yonghu.getPhone())
.eq("id_number", yonghu.getIdNumber())
.eq("sex_types", yonghu.getSexTypes())
.eq("nation", yonghu.getNation())
.eq("politics_types", yonghu.getPoliticsTypes())
.eq("birthplace", yonghu.getBirthplace())
.eq("yonghu_types", yonghu.getYonghuTypes())
;
logger.info("sql语句:"+queryWrapper.getSqlSegment());
YonghuEntity yonghuEntity = yonghuService.selectOne(queryWrapper);
if(yonghuEntity==null){
yonghu.setCreateTime(new Date());
yonghu.setPassword("123456");
// String role = String.valueOf(request.getSession().getAttribute("role"));
// if("".equals(role)){
// yonghu.set
// }
yonghuService.insert(yonghu);
return R.ok();
}else {
return R.error(511,"表中有相同数据");
}
}
/**
* 修改
*/
@RequestMapping("/update")
public R update(@RequestBody YonghuEntity yonghu, HttpServletRequest request){
logger.debug("update方法:,,Controller:{},,yonghu:{}",this.getClass().getName(),yonghu.toString());
//根据字段查询是否有相同数据
Wrapper<YonghuEntity> queryWrapper = new EntityWrapper<YonghuEntity>()
.notIn("id",yonghu.getId())
.eq("username", yonghu.getUsername())
.eq("password", yonghu.getPassword())
.eq("name", yonghu.getName())
.eq("phone", yonghu.getPhone())
.eq("id_number", yonghu.getIdNumber())
.eq("sex_types", yonghu.getSexTypes())
.eq("nation", yonghu.getNation())
.eq("politics_types", yonghu.getPoliticsTypes())
.eq("birthplace", yonghu.getBirthplace())
.eq("yonghu_types", yonghu.getYonghuTypes())
;
logger.info("sql语句:"+queryWrapper.getSqlSegment());
YonghuEntity yonghuEntity = yonghuService.selectOne(queryWrapper);
if("".equals(yonghu.getMyPhoto()) || "null".equals(yonghu.getMyPhoto())){
yonghu.setMyPhoto(null);
}
if(yonghuEntity==null){
// String role = String.valueOf(request.getSession().getAttribute("role"));
// if("".equals(role)){
// yonghu.set
// }
yonghuService.updateById(yonghu);//根据id更新
return R.ok();
}else {
return R.error(511,"表中有相同数据");
}
}
/**
* 删除
*/
@RequestMapping("/delete")
public R delete(@RequestBody Integer[] ids){
logger.debug("delete:,,Controller:{},,ids:{}",this.getClass().getName(),ids.toString());
yonghuService.deleteBatchIds(Arrays.asList(ids));
return R.ok();
}
/**
*字典表数据转换
*/
public void dictionaryConvert(YonghuView yonghuView){
//当前表的字典字段
if(StringUtil.isNotEmpty(String.valueOf(yonghuView.getSexTypes()))){
yonghuView.setSexValue(dictionaryMap.get("sex_types").get(yonghuView.getSexTypes()));
}
if(StringUtil.isNotEmpty(String.valueOf(yonghuView.getPoliticsTypes()))){
yonghuView.setPoliticsValue(dictionaryMap.get("politics_types").get(yonghuView.getPoliticsTypes()));
}
if(StringUtil.isNotEmpty(String.valueOf(yonghuView.getYonghuTypes()))){
yonghuView.setYonghuValue(dictionaryMap.get("yonghu_types").get(yonghuView.getYonghuTypes()));
}
//级联表的字典字段
}
/**
* 登录
*/
@IgnoreAuth
@PostMapping(value = "/login")
public R login(String username, String password, String captcha, HttpServletRequest request) {
YonghuEntity yonghu = yonghuService.selectOne(new EntityWrapper<YonghuEntity>().eq("username", username));
if(yonghu==null || !yonghu.getPassword().equals(password)) {
return R.error("账号或密码不正确");
}
ServletContext servletContext = ContextLoader.getCurrentWebApplicationContext().getServletContext();
dictionaryMap = (Map<String, Map<Integer, String>>) servletContext.getAttribute("dictionaryMap");
String role = dictionaryMap.get("yonghu_types").get(yonghu.getYonghuTypes());
String token = tokenService.generateToken(yonghu.getId(),username, "yonghu",role );
R r = R.ok();
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
【基于SSM+Jsp的多角色学生管理系统的设计与实现】高分通过项目,已获导师指导。 本项目是一套基于SSM+Jsp的多角色学生管理系统,主要针对计算机相关专业的正在做毕设的学生和需要项目实战练习的Java学习者。也可作为课程设计、期末大作业 包含:项目源码、数据库脚本、开发说明文档、部署视频、代码讲解视频、全套软件等,该项目可以直接作为毕设使用。 项目都经过严格调试,确保可以运行!
资源推荐
资源详情
资源评论
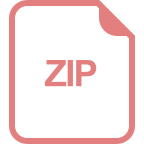
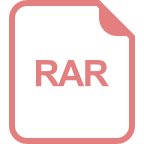
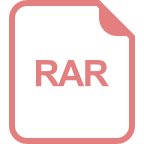
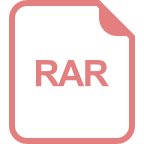
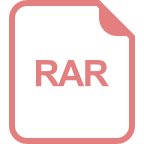
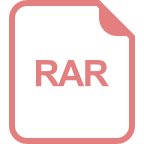
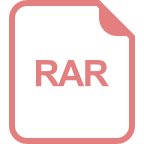
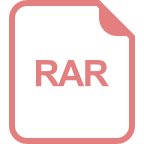
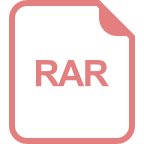
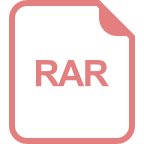
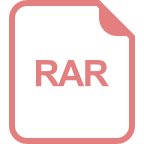
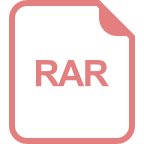
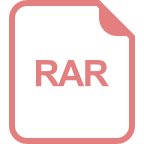
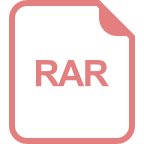
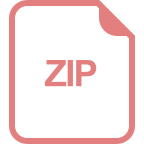
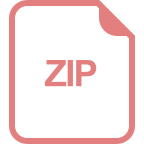
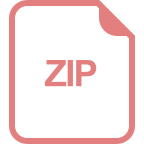
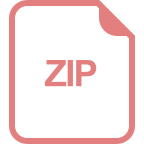
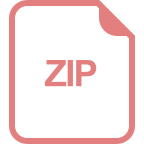
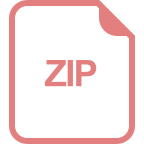
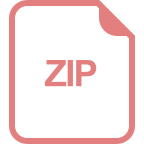
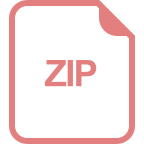
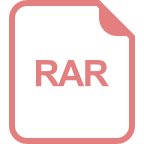
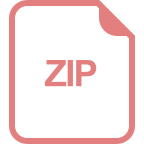
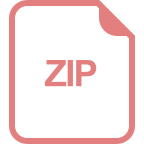
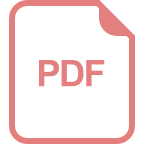
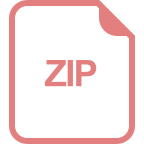
收起资源包目录

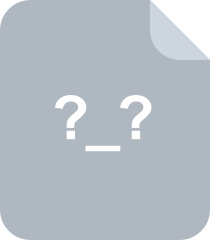
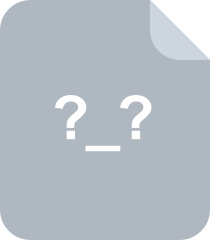
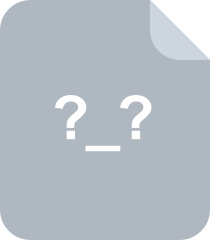
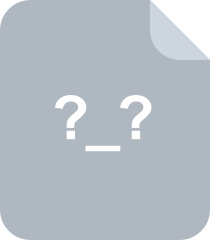
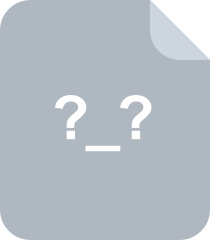
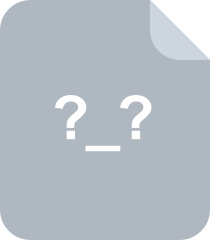
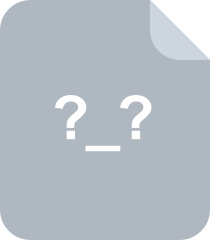
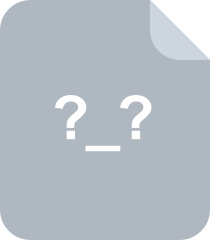
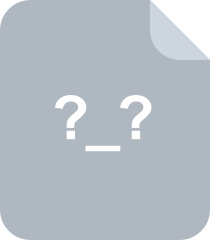
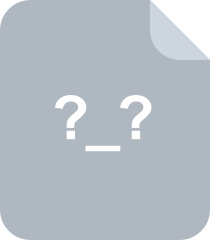
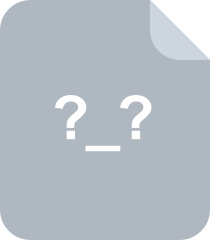
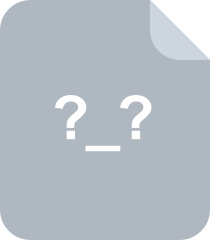
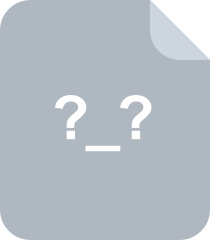
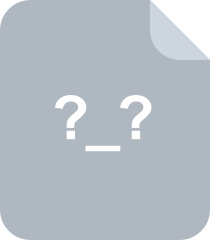
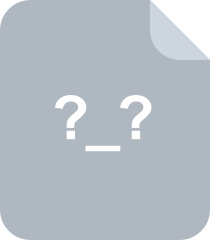
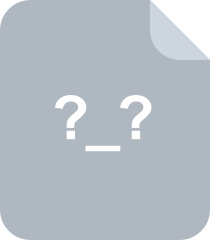
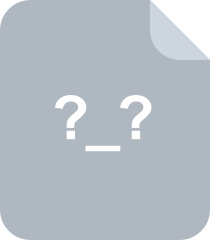
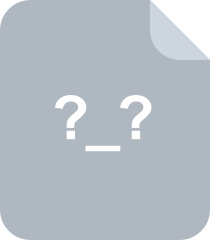
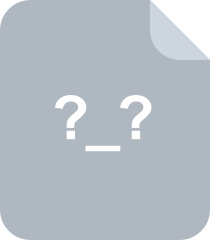
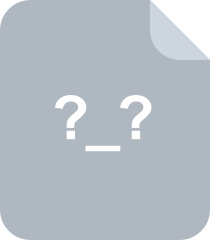
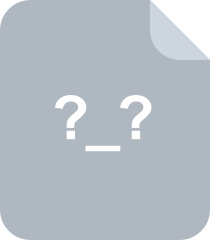
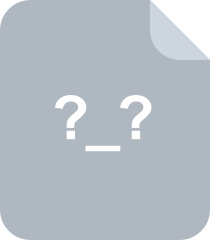
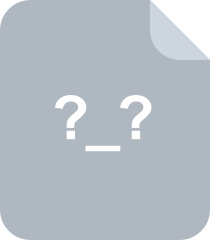
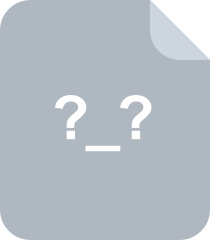
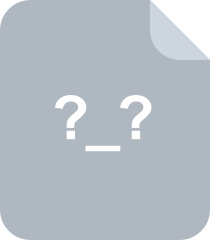
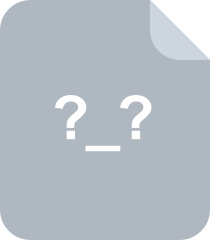
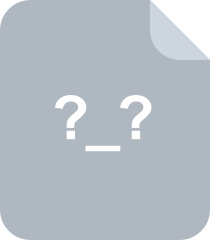
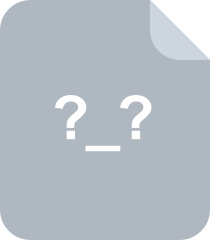
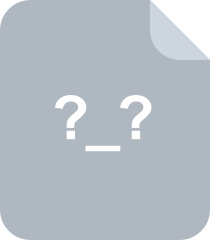
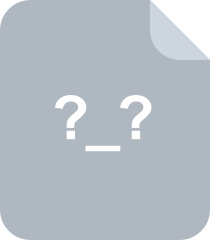
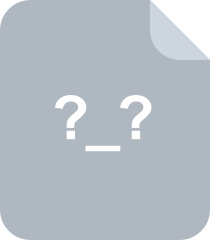
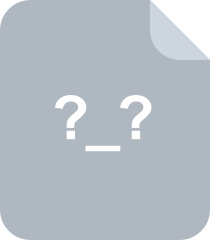
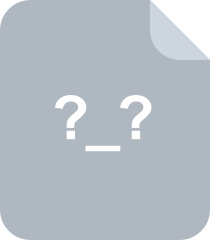
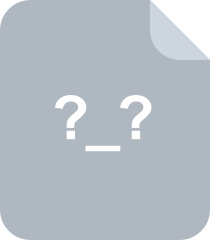
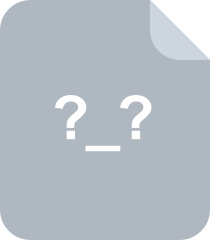
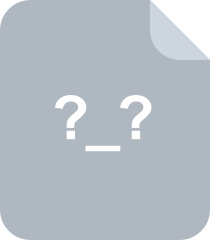
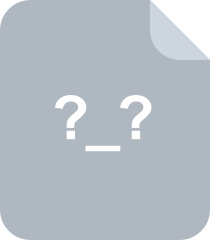
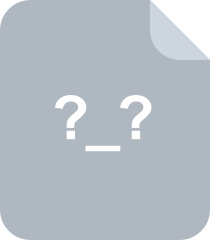
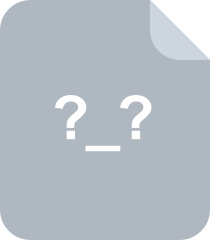
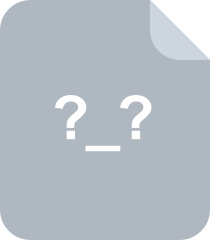
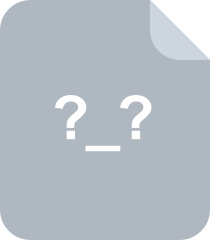
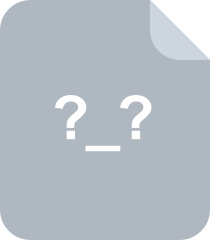
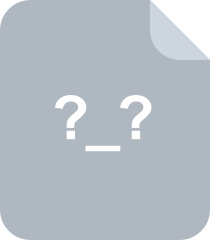
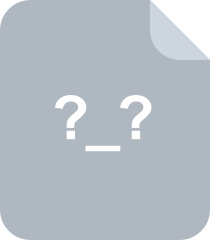
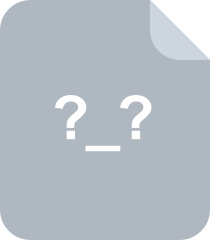
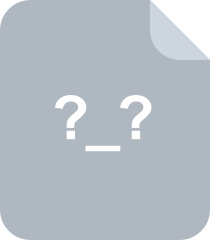
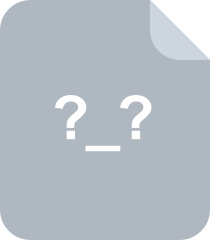
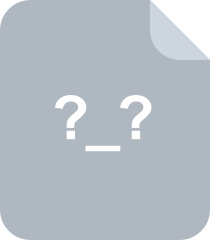
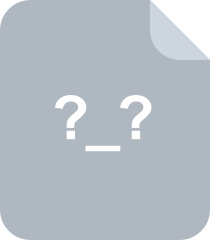
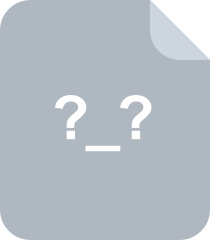
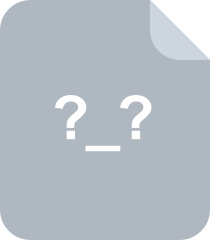
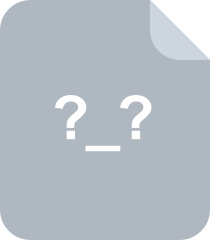
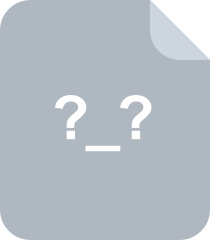
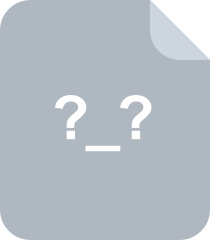
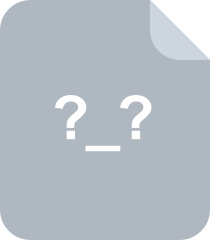
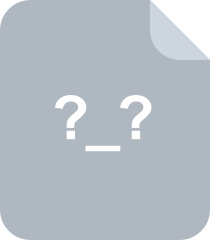
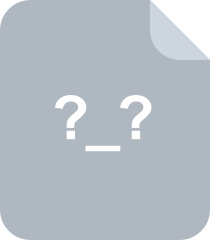
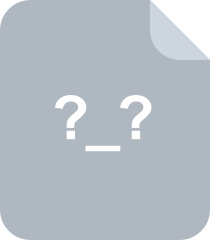
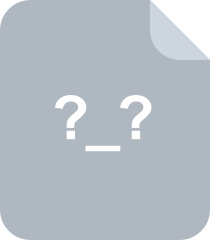
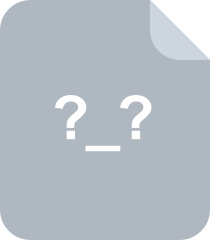
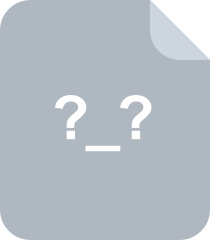
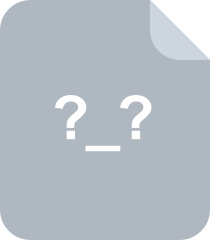
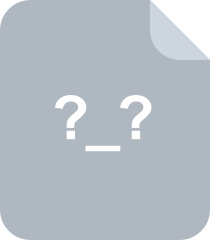
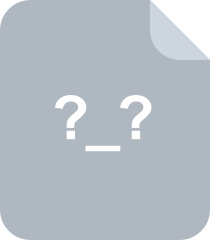
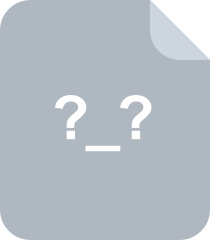
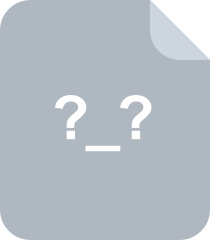
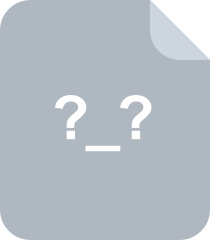
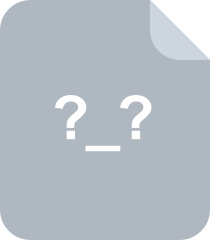
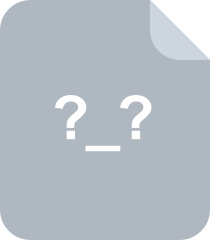
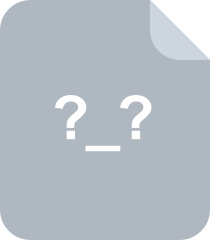
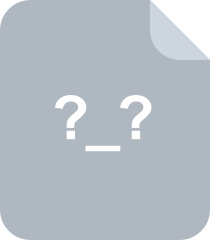
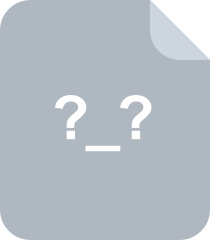
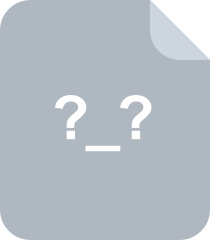
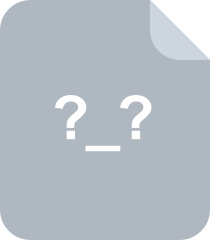
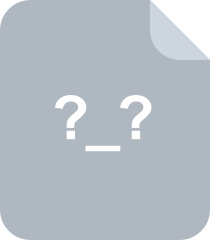
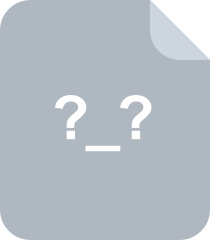
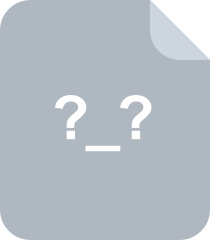
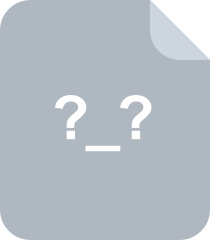
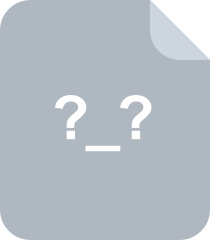
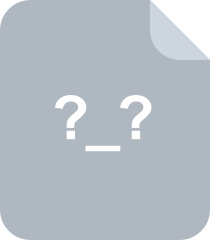
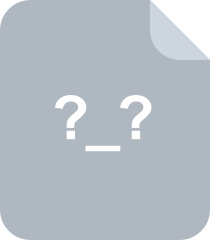
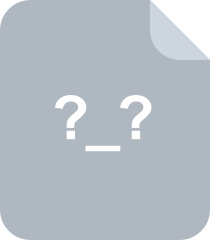
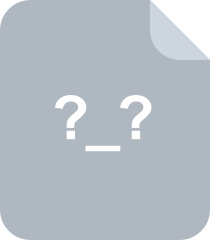
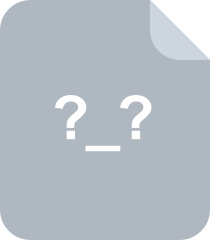
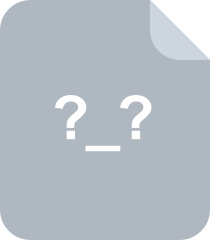
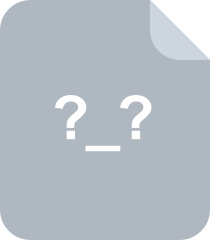
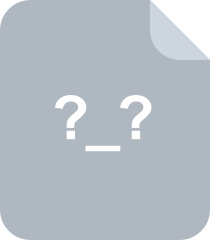
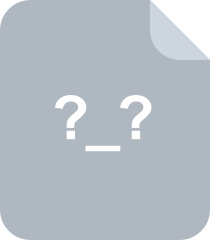
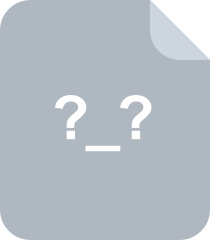
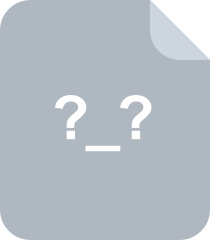
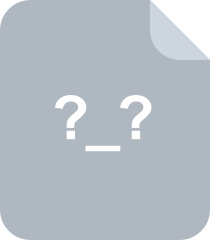
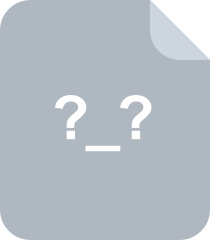
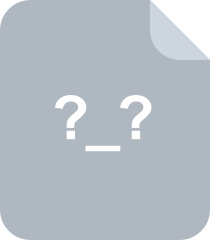
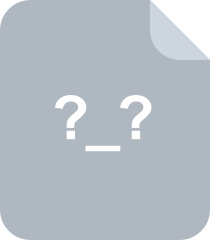
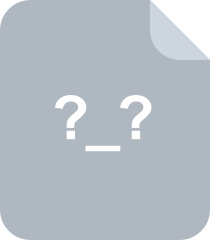
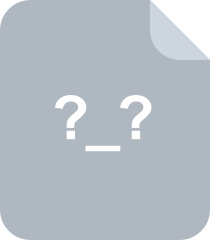
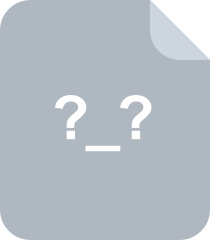
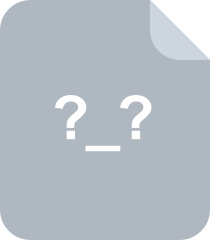
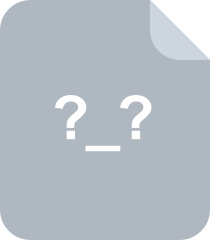
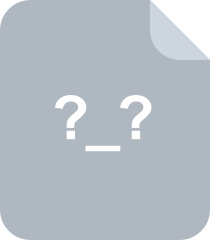
共 987 条
- 1
- 2
- 3
- 4
- 5
- 6
- 10
资源评论
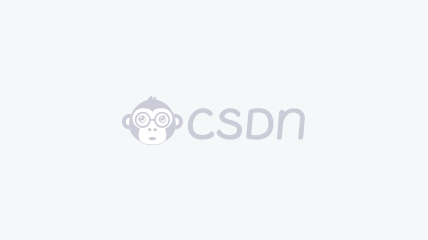

Java骨灰级码农
- 粉丝: 4616
- 资源: 928
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

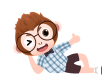
最新资源
- 【Unity遮挡剔除插件】Perfect Culling Occlusion Culling System 显著优化渲染性能
- 永磁同步电机 FOC算法电流环+速度环框架,本例中给id,iq反馈电流加了一小段延时,模拟电流采样的延时
- 【Unity语音插件】Dissonance Voice Chat 强大而灵活的实时语音通信解决方案
- 新手改善Java程序的151个建议 优化程序 良好习惯
- api-ms-win-core-path-l1-1-0.dll
- 响应式圣诞树:使用CSS的`max-width`和`min-width`属性
- 考研复试的面试中英文自我介绍及问答
- 1.FreeRTOS之任务
- cri-containerd
- Python-扫雷游戏 实战案例
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


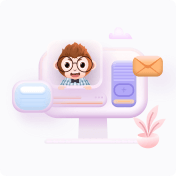
安全验证
文档复制为VIP权益,开通VIP直接复制
