没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
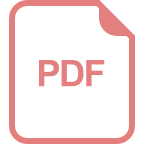
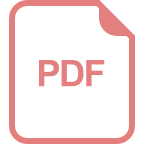
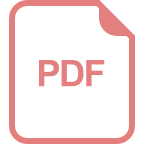
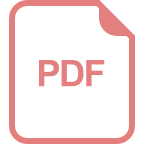
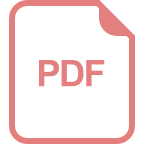
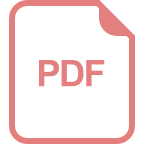
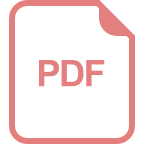
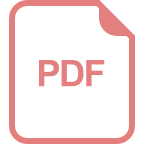
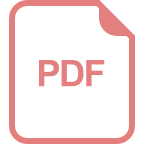
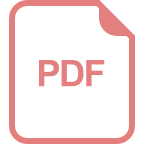
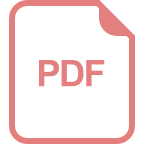
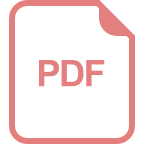
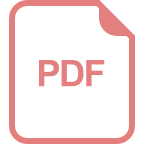
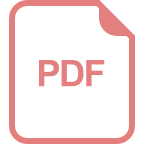
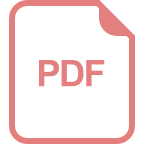
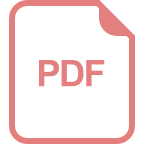
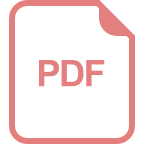
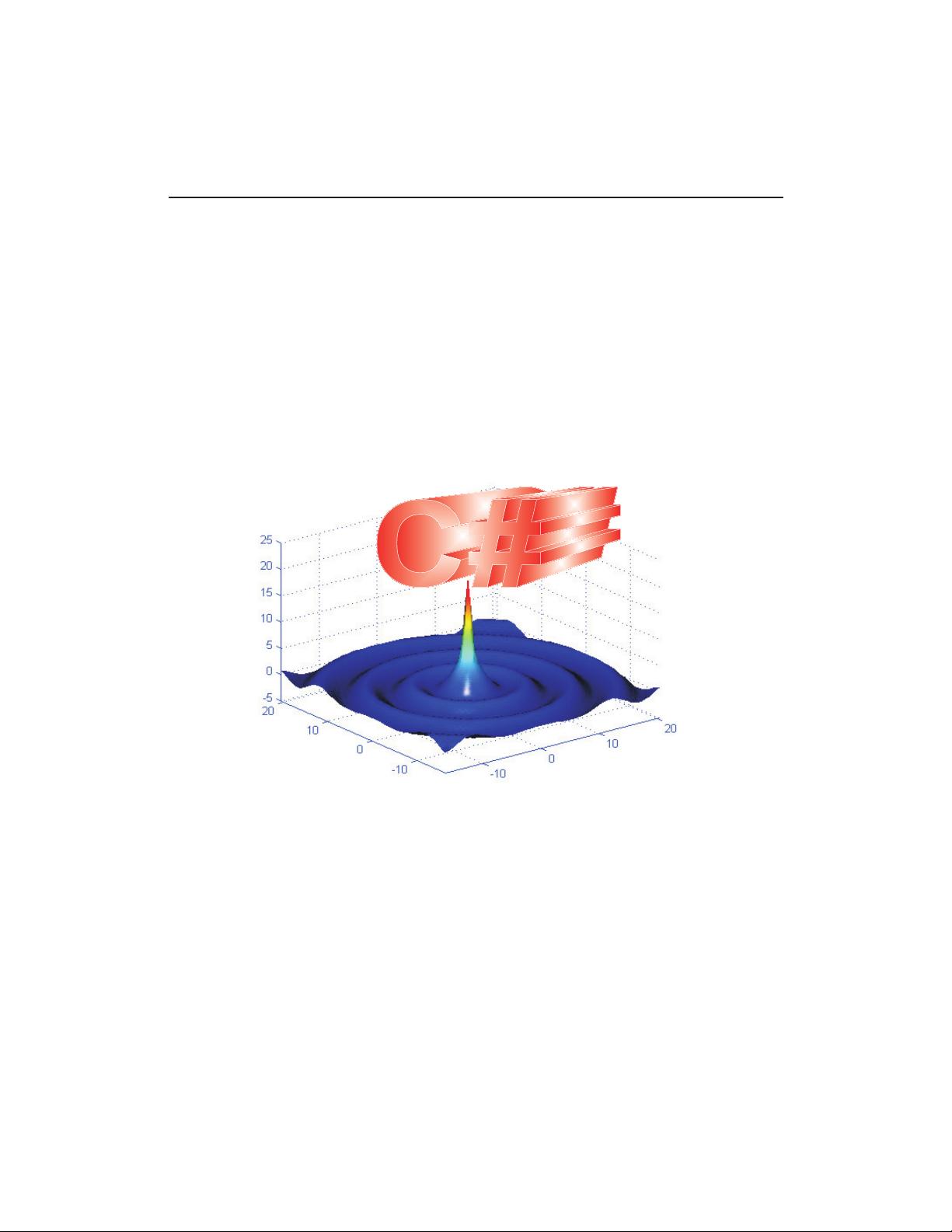
FirstEdition
ThisbookisagreattutorialforC#programmers
whouseMATLABtodevelopapplicationsandsolutions
M
ATL AB C#
® ®
Book
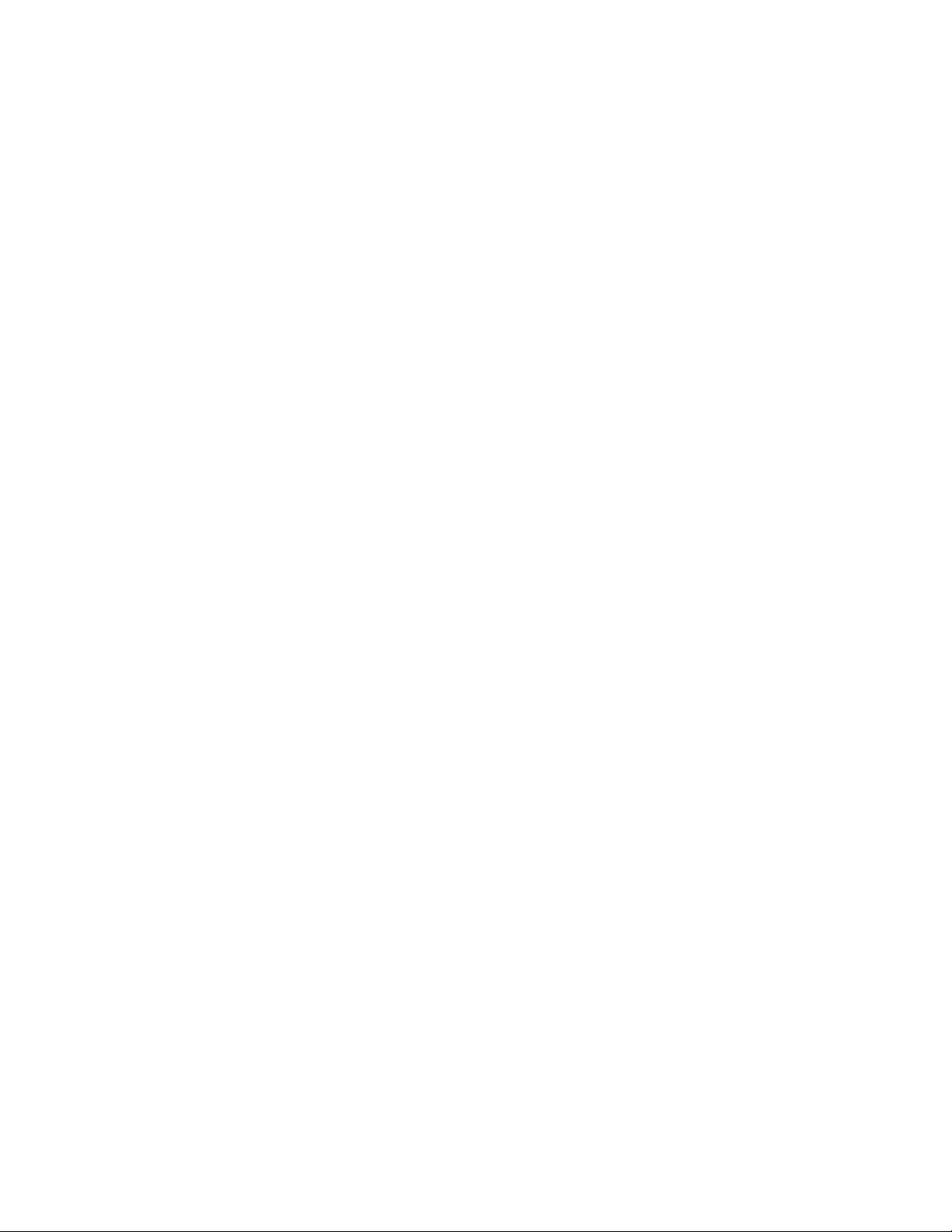
®
Allrightsreserved.NopartofthisCD-ROMshouldbereproduced,
ortransmittedbyanymeans,electronic,mechanical,photocopying,
recording,orotherwise,withoutwrittenpermissionfromthepublisher.
M C# BookATLAB
Copyright 2004byLePhanPublishing
®
MATLABisaregisteredtrademarkofTheMathWorks,Inc.
Microsoft,C#areregisteredtrademarksofMicrosoftCorporation.
Ó
Trademark
TheprogramsandapplicationsonthisCD-ROMhavebeencarefullytested,
butarenotguaranteedforanyparticularpurpose.Thepublisherdoesnot
offeranywarrantiesanddoesnotguaranteetheaccuracy,adequacy,
orcompletenessofanyinformationandisnotresponsibleforanyerrors
oromissionsortheresultsobtainedfromuseofsuchinformation.
ISBN0-9725794-4-3
Disclaimer
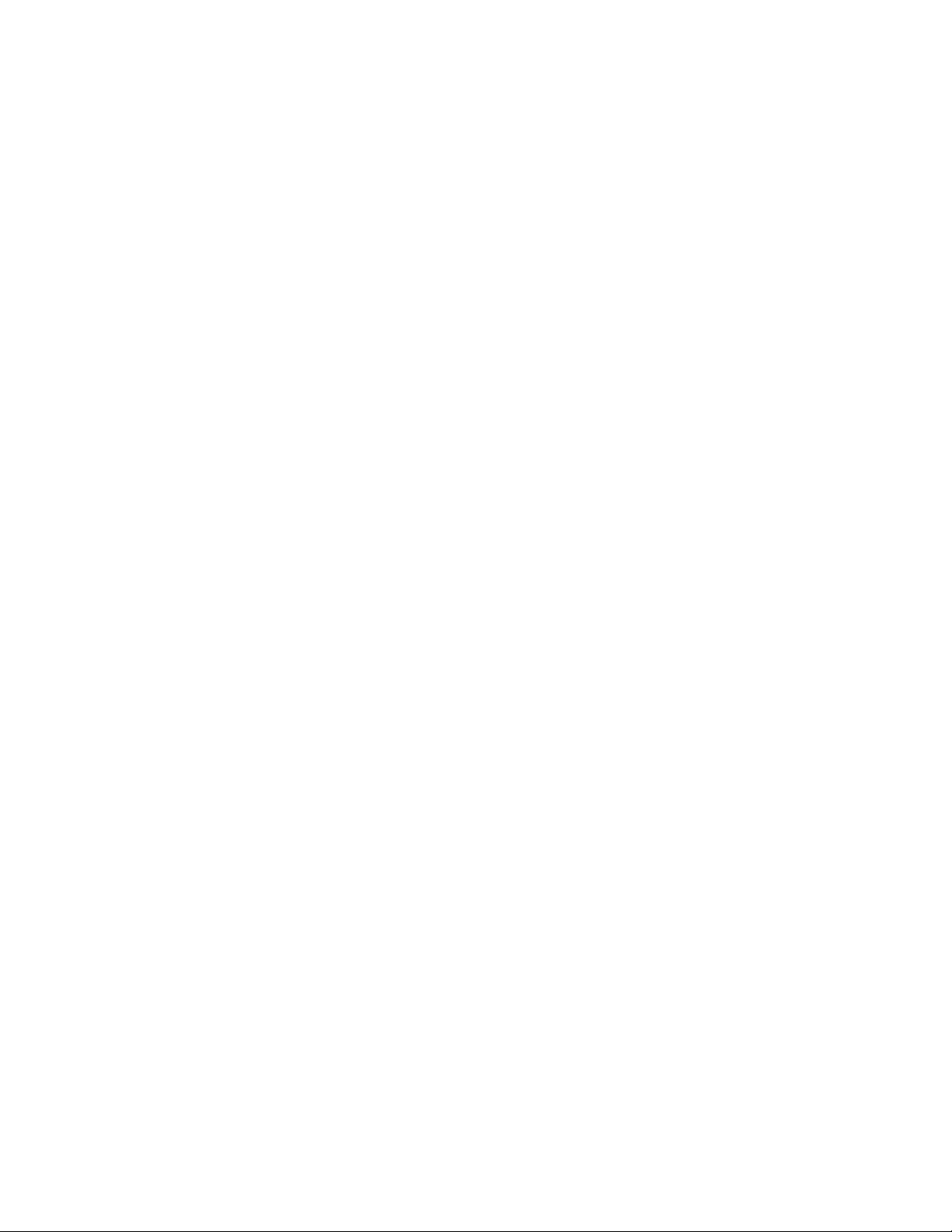
Contents
Preface v
Part I:
Creating and Using MATLAB Shared Library to Solve Mathematical
Problems In C
#
1
1 Introduction 3
1.1 Introduction . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 3
1.2 Computer Software and Book Features . . . . . . . . . . . . . . . . . . . . . . . . 4
1.3 Reference Manuals . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 4
2 Generating a C Shared Library from MATLAB M-Files To Use in Microsoft
Visual C
#
.Net
5
2.1 Generating a C Shared Library from a MATLAB M-File . . . . . . . . . . . . . . 5
2.2 Calling a C Shared Library Function in C
#
. . . . . . . . . . . . . . . . . . . . 7
2.3 Generated Functions from MATLAB Compiler 4 . . . . . . . . . . . . . . . . . . 9
2.4 Using Functions of a C shared library in C
#
. . . . . . . . . . . . . . . . . . . . 10
3 Transfer of Values between C
#
double and mxArray 11
3.1 Transfer of Values between C
#
double and mxArray . . . . . . . . . . . . . . . . 11
3.2 The Utility File CsharpMatlabCompilerVer4.cs . . . . . . . . . . . . . . . . . . . 16
4 Matrix Computations 35
4.1 Matrix Addition . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 37
4.2 Matrix Subtraction . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 39
4.3 Matrix Multiplication . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 40
4.4 Matrix Determinant . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 41
4.5 Inverse Matrix . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 42
4.6 Transpose Matrix . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 42
i
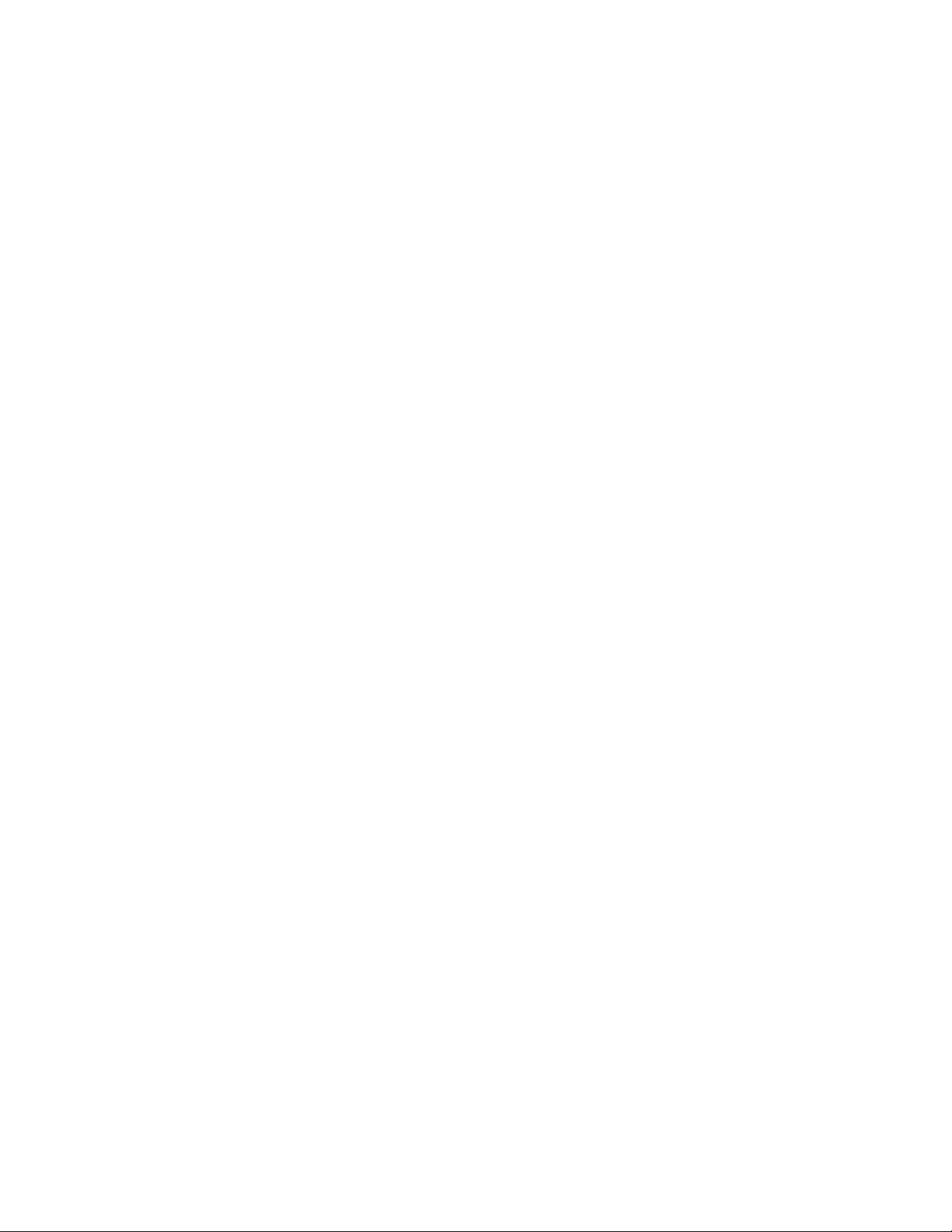
ii
5 Linear System Equations 43
5.1 Linear System Equations . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 45
5.2 Sparse Linear System . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 49
5.3 Tridiagonal System Equations . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 52
5.4 Band Diagonal System Equations . . . . . . . . . . . . . . . . . . . . . . . . . . . 56
6 Ordinary Differential Equations 61
6.1 First Order ODE . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
63
6.2 Second Order ODE . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 69
6.2.1 Analysis of second order ODE . . . . . . . . . . . . . . . . . . . . . . . . . 69
6.2.2 Using a second order ODE function . . . . . . . . . . . . . . . . . . . . . 70
7 Integration 73
7.1 Single Integration . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 74
7.2 Double-Integration . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 78
8 Curve Fitting and Interpolations 81
8.1 Polynomial Curve Fitting . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 83
8.2 One-Dimensional Polynomial Interpolation . . . . . . . . . . . . . . . . . . . . . 87
8.3 Two-Dimensional Polynomial Interpolation for Grid Points . . . . . . . . . . . . 88
9 Roots of Equations 97
9.1 Roots of Polynomials . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
98
9.2 The Root of a Nonlinear-Equation . . . . . . . . . . . . . . . . . . . . . . . . . . 101
10 Fast Fourier Transform 103
10.1 One-Dimensional Fast Fourier Transform . . . . . . . . . . . . . . . . . . . . . . 105
10.2 Two-Dimensional Fast Fourier Transform . . . . . . . . . . . . . . . . . . . . . . 109
11 Eigenvalues and Eigenvectors 113
11.1 Eigenvalues and Eigenvectors . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 114
Part II:
Calling MATLAB Workspace in C
#
Functions
Using MATLAB functions in C
#
Windows Form Applications 119
12
Calling MATLAB Workspace in C
#
Functions 121
12.1 Calling MATLAB Workspace with Input/Output as a Scalar . . . . . . . . . . . 121
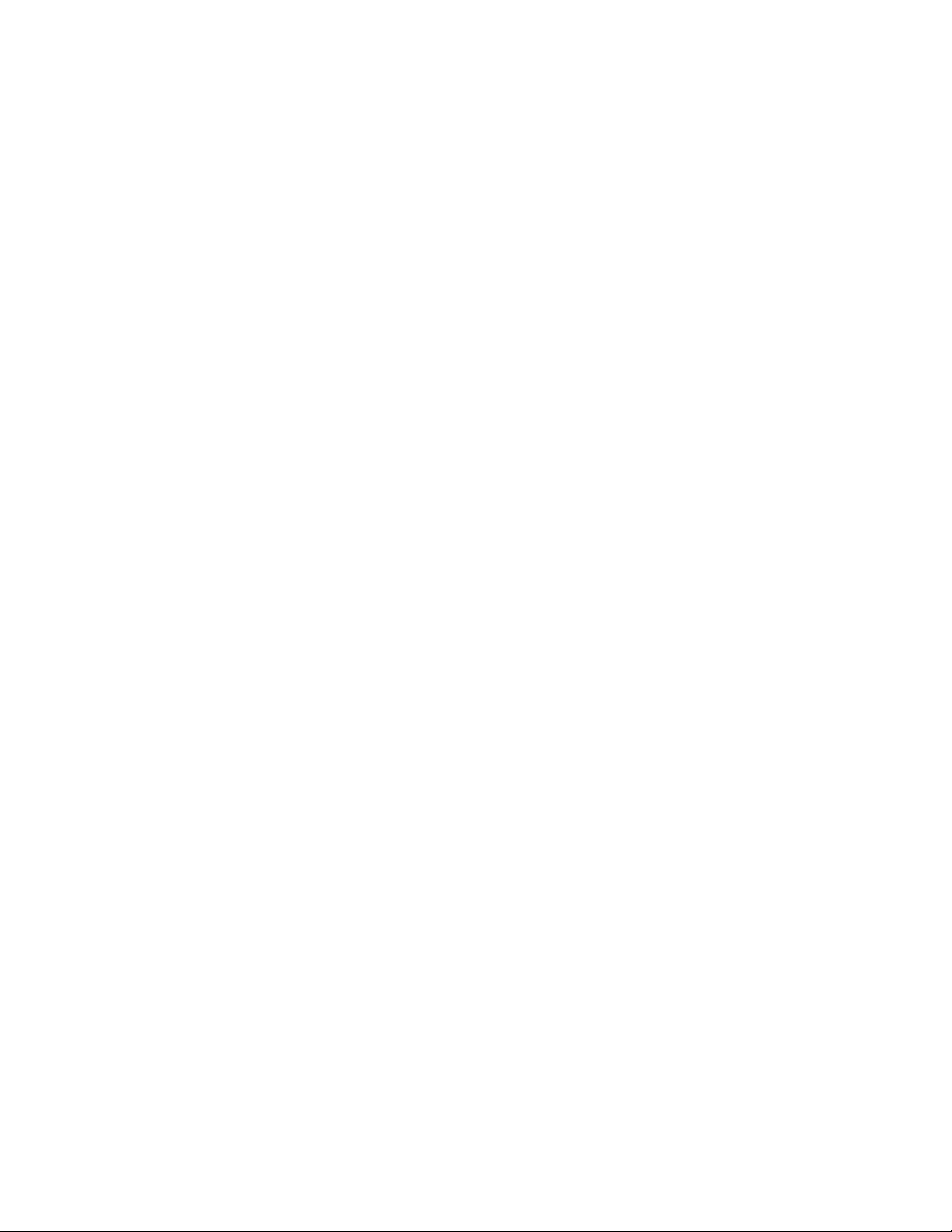
iii
12.2 Calling MATLAB Workspace with Input/Output as a Vector and a Matrix . . . 123
12.3 Generating a MATLAB Graphic from a C
#
Function . . . . . . . . . . . . . . . 125
13 Using MATLAB Functions In C
#
Windows Forms Applications 127
Part III:
Creating and Using COM From MATLAB COM Builder In C
#
131
14 Using COM Created from MATLAB COM Builder in C
#
Applications 135
14.1 Creating COM From MATLAB COM Builder . . . . . . . . . . . . . . . . . . . .
135
14.2 Using COM in C# functions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 138
15 Using COM Created from MATLAB COM Builder in C
#
Applications for
Matrix Manipulation & Linear System Equations
143
15.1 Creating COM From MATLAB COM Builder . . . . . . . . . . . . . . . . . . . . 143
15.2 Using COM From MATLAB COM Builder To Calculate Matrix Multiplication . 144
15.3 Using COM From MATLAB COM Builder To Solve Linear System Equations . . 146
References 149
Index 151
剩余158页未读,继续阅读
资源评论
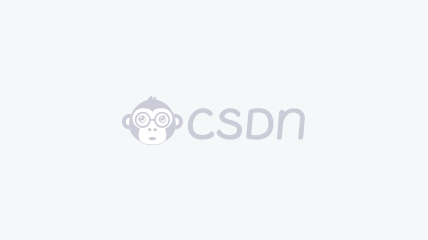

yinkaisheng-nj
- 粉丝: 763
- 资源: 6231
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

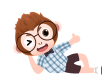
最新资源
- NetBox2及大疆智图影像缓存lrc模板
- 123456789自用解答題
- JAVA的SpringBoot个人理财系统源码数据库 MySQL源码类型 WebForm
- 全屋智能全球市场报告:2023年中国全屋智能行业市场规模已达到3705亿元
- 康复医疗全球市场报告:2023年年复合增长率高达18.19%
- 微信小程序期末大作业-商城-2024(底部导航栏,轮播图,注册登录,购物车等等)
- 碘产业全球市场报告:2023年全球碘需求量已攀升至约3.86万吨
- 基于CNN、RNN、GCN、BERT的中文文本分类源码Python高分期末大作业
- 最新源支付Ypay系统开心稳定最新免授权源码,三平台免挂免签约支付
- 6-测试安全风险知情告知书(2).docx
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


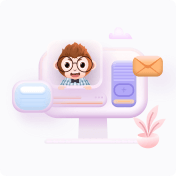
安全验证
文档复制为VIP权益,开通VIP直接复制
